How Can You Effectively Clear Variables in Python?
In the world of programming, managing memory and resources efficiently is crucial for optimizing performance and ensuring smooth execution. Python, a language celebrated for its simplicity and readability, provides developers with a variety of tools to handle variables and their lifecycles. However, as your code grows in complexity, you may find yourself needing to clear variables to free up memory or reset your environment. Understanding how to clear variables in Python not only enhances your coding practices but also empowers you to write cleaner, more efficient code.
Clearing variables in Python can be a straightforward process, yet it involves a nuanced understanding of the language’s memory management and variable scope. Whether you’re working in an interactive environment like Jupyter Notebook or developing a larger application, knowing when and how to clear variables can help prevent memory leaks and improve performance. Python offers several methods to achieve this, each with its own use cases and implications.
From using the `del` statement to remove specific variables, to leveraging garbage collection for automatic memory management, there are multiple strategies at your disposal. As we delve deeper into the topic, we will explore these methods, their advantages, and the best practices for managing your variables effectively, ensuring that you can maintain a clean and efficient coding environment.
Using the `del` Statement
The `del` statement in Python is a straightforward way to remove variables from the local or global namespace. When you use `del`, the variable is deleted, and any reference to it afterward will raise a `NameError`. Here’s how to use it:
“`python
x = 10
del x x is now deleted
“`
You can also delete multiple variables at once by passing them as a comma-separated list:
“`python
a, b, c = 1, 2, 3
del a, b a and b are deleted, but c remains
“`
Setting Variables to `None`
Another common practice is to set variables to `None`. This does not remove the variable, but it clears its value, which can be useful for indicating that a variable is no longer needed or is in an uninitialized state.
“`python
x = 10
x = None x is now None
“`
This method allows you to maintain the variable name while essentially resetting its value.
Using the `locals()` Function
In more complex scenarios, especially when dealing with a large number of variables, you can use the `locals()` function in combination with `del` to clear multiple variables. `locals()` returns a dictionary of the current local symbol table, which you can manipulate.
“`python
for var in list(locals().keys()):
if var.startswith(‘temp_’): Example condition to match variable names
del locals()[var]
“`
This method provides a programmatic way to clear variables based on specific criteria.
Clearing All Variables in a Namespace
To clear all variables from a namespace, particularly in an interactive environment such as Jupyter Notebook, you can use the following command:
“`python
%reset
“`
This command will prompt you to confirm that you want to delete all variables in the current namespace.
Alternatively, in a script, you can use:
“`python
globals().clear()
“`
However, this approach will remove all global variables, including built-in ones, which can lead to errors if not handled carefully.
Method | Description | Use Case |
---|---|---|
del | Removes the variable from the namespace. | When you no longer need a variable. |
None | Resets the variable’s value to None. | To indicate a variable is uninitialized. |
locals() | Allows deletion of variables based on conditions. | When handling multiple variables dynamically. |
%reset | Clears all variables in interactive environments. | In Jupyter, when starting fresh. |
globals().clear() | Removes all global variables. | In scripts, with caution required. |
These methods provide flexibility depending on your specific requirements for managing variables in Python.
Clearing Variables in Python
In Python, there are several methods to clear or delete variables. The approach you choose may depend on your specific use case and the scope of the variables you wish to clear.
Using the `del` Statement
The `del` statement is a straightforward way to remove a variable from the namespace. Once a variable is deleted, it is no longer accessible, and attempting to use it will result in a `NameError`.
“`python
x = 10
print(x) Output: 10
del x
print(x) Raises NameError: name ‘x’ is not defined
“`
Clearing Variables in a Dictionary
If variables are stored in a dictionary, you can clear them by using the `clear()` method or by deleting specific keys.
- Using `clear()`:
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.clear()
print(my_dict) Output: {}
“`
- Deleting specific keys:
“`python
my_dict = {‘a’: 1, ‘b’: 2}
del my_dict[‘a’]
print(my_dict) Output: {‘b’: 2}
“`
Resetting Variables
To reset a variable while retaining its name, you can simply assign it a new value or use `None`.
“`python
y = 5
y = None
print(y) Output: None
“`
This method is useful when you want to indicate that the variable is no longer holding a meaningful value but still want to keep its reference for potential future use.
Clearing All Variables in a Session
To clear all variables in an interactive session, such as IPython or Jupyter Notebook, you can use the `%reset` magic command. This command will prompt you for confirmation before deleting all variables.
“`python
In a Jupyter Notebook cell
%reset
“`
Alternatively, in a script, you can restart the kernel or the Python interpreter to achieve the same effect.
Using the `globals()` Function
You can access and modify global variables using the `globals()` function, which returns a dictionary of the current global symbol table. You can delete specific variables or clear them all.
“`python
a = 1
b = 2
print(globals()) Shows all global variables
Deleting a specific variable
del globals()[‘a’]
print(globals()) ‘a’ is removed
Clearing all global variables (not recommended in practice)
for key in list(globals().keys()):
if key not in [‘__name__’, ‘__doc__’, ‘__package__’]:
del globals()[key]
“`
Best Practices for Variable Management
To maintain clean and efficient code, consider the following best practices:
- Use descriptive variable names: This reduces confusion about variable purpose.
- Limit variable scope: Define variables within the smallest scope necessary.
- Avoid using `del` in large scripts: It may lead to unexpected behavior; instead, use context management or function scopes.
- Regularly review and refactor code: This helps in identifying unused variables that can be safely removed.
By employing these techniques, you can effectively manage variable lifecycle and memory usage in Python.
Expert Insights on Clearing Variables in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively clear variables in Python, one can use the `del` statement, which removes the variable from the local or global namespace. This is particularly useful in long-running applications to free up memory.”
Michael Chen (Data Scientist, Analytics Hub). “In data-heavy applications, clearing variables not only helps manage memory but also prevents unintended data leakage. Using `gc.collect()` after deleting variables can ensure that any unreferenced memory is also freed.”
Sarah Thompson (Python Developer, CodeCraft Solutions). “It’s important to note that simply assigning `None` to a variable does not free up the memory immediately. Instead, using `del` is the best practice for clearing variables, especially in iterative processes.”
Frequently Asked Questions (FAQs)
How do I delete a variable in Python?
You can delete a variable in Python using the `del` statement followed by the variable name, for example, `del variable_name`.
What happens to a variable when it is deleted in Python?
When a variable is deleted, it is removed from the local or global namespace, and its memory is freed if no other references to the object exist.
Can I clear all variables in a Python script?
Yes, you can clear all variables in a script by using the `globals()` function along with `del` in a loop, or by restarting the Python interpreter.
Is there a way to clear variables in an interactive Python session?
In an interactive session, you can use the `reset` command in IPython or Jupyter Notebook to clear all variables.
How can I check if a variable exists before deleting it?
You can check if a variable exists using the `in` keyword with `locals()` or `globals()`, for example, `if ‘variable_name’ in locals(): del variable_name`.
What is the difference between deleting a variable and clearing its value?
Deleting a variable removes it entirely from the namespace, while clearing its value (e.g., setting it to `None`) keeps the variable in the namespace but removes its assigned value.
In Python, clearing variables can be accomplished through various methods, depending on the context and the desired outcome. One common approach is to use the `del` statement, which removes a variable from the local or global namespace. This effectively frees up the memory associated with that variable, making it unavailable for further use. Additionally, reassigning a variable to `None` can also indicate that it is no longer needed, although the variable itself remains in the namespace.
Another method to clear all variables in a specific scope, such as within a Jupyter Notebook, is to use the `%reset` magic command. This command clears all variables in the current interactive session, allowing for a fresh start without the need to restart the kernel. It is important to note that this action cannot be undone, so users should ensure they have saved any necessary data before executing it.
For those working within a script or module, it is generally advisable to manage variable scope carefully and avoid unnecessary variable persistence. Utilizing functions and classes can help encapsulate variables, making it easier to control their lifecycle. In summary, understanding how to effectively clear variables in Python is essential for memory management and maintaining clean code practices.
Author Profile
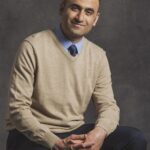
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?