How Can You Easily Combine Two Dictionaries in Python?
In the world of Python programming, dictionaries are a fundamental data structure that allows developers to store and manipulate data in key-value pairs. As your projects grow in complexity, the need to merge or combine multiple dictionaries often arises. Whether you’re aggregating data from various sources, consolidating configurations, or simply looking to streamline your code, understanding how to effectively combine dictionaries is an essential skill for any Python programmer.
Combining dictionaries in Python can be approached in several ways, each with its own advantages and nuances. From using built-in methods to leveraging the power of comprehensions, Python offers a variety of techniques to achieve this goal. Depending on your specific needs—such as whether you want to handle overlapping keys or maintain the order of entries—there are tailored solutions that can help you merge dictionaries seamlessly.
As we delve deeper into this topic, you’ll discover not only the various methods available for combining dictionaries but also best practices to ensure your code remains efficient and readable. By mastering these techniques, you’ll enhance your ability to manipulate data structures in Python, paving the way for more robust and dynamic applications. So, let’s explore the different strategies for merging dictionaries and find the one that best fits your coding style and project requirements.
Using the Update Method
The `update()` method is a straightforward way to merge two dictionaries in Python. This method modifies the dictionary in place, adding key-value pairs from another dictionary. If a key already exists in the original dictionary, its value will be updated with the value from the second dictionary.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
dict1.update(dict2)
print(dict1) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
In this example, the value for key `’b’` in `dict1` is updated to `3`, while keys from `dict2` that do not exist in `dict1` are added.
Using the Merge Operator
Starting from Python 3.9, the merge operator `|` can be utilized to combine dictionaries easily. This operator creates a new dictionary containing the merged contents of both dictionaries, without modifying the originals.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = dict1 | dict2
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
This method retains the values from the second dictionary for duplicate keys, similar to the `update()` method but without altering the original dictionaries.
Using Dictionary Comprehension
Another approach to combine two dictionaries is through dictionary comprehension. This method is particularly useful when you need to apply conditions or transformations while merging.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined_dict = {k: v for d in (dict1, dict2) for k, v in d.items()}
print(combined_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
In this example, the dictionary comprehension iterates over both dictionaries, merging their key-value pairs. The last dictionary’s values overwrite those from the previous one for duplicate keys.
Using the ChainMap from Collections
The `ChainMap` class from the `collections` module provides a way to group multiple dictionaries together. While it does not create a new dictionary, it allows for treating multiple dictionaries as one.
“`python
from collections import ChainMap
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined = ChainMap(dict2, dict1)
print(dict(combined)) Output: {‘b’: 3, ‘c’: 4, ‘a’: 1}
“`
This approach gives priority to the first dictionary when keys overlap, allowing for controlled visibility of keys.
Comparison Table of Methods
Method | In-place Modification | Returns New Dictionary | Python Version |
---|---|---|---|
update() | Yes | No | All versions |
Merge Operator (|) | No | Yes | 3.9+ |
Dictionary Comprehension | No | Yes | All versions |
ChainMap | No | No (views only) | 3.3+ |
Each method has its own use cases and benefits, allowing developers to choose the most suitable approach based on their specific requirements and the Python version they are using.
Methods to Combine Two Dictionaries in Python
In Python, there are several efficient ways to combine two dictionaries. Each method has its own use cases and advantages. Below are the most common approaches:
Using the `update()` Method
The `update()` method allows you to merge one dictionary into another. This method modifies the first dictionary in place.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
dict1.update(dict2)
dict1 is now {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
- If keys overlap, the values from the second dictionary will overwrite those from the first.
Using Dictionary Unpacking (Python 3.5+)
Dictionary unpacking is a concise way to create a new dictionary by merging two or more dictionaries.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined = {dict1, dict2}
combined is {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
- This method is particularly useful when you want to retain the original dictionaries unchanged.
Using the `|` Operator (Python 3.9+)
Python 3.9 introduced a new syntax for merging dictionaries using the `|` operator.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined = dict1 | dict2
combined is {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
- This method is both clear and efficient, allowing for a more readable syntax when combining dictionaries.
Using a Dictionary Comprehension
A dictionary comprehension provides a flexible way to combine dictionaries, especially if you want to apply custom logic to the merging process.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined = {k: v for d in (dict1, dict2) for k, v in d.items()}
combined is {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
- This approach allows you to control how keys and values are combined, such as summing values for overlapping keys.
Handling Duplicate Keys
When combining dictionaries, handling duplicate keys may be necessary. The following strategies can be applied:
Method | Description |
---|---|
Overwrite | The last dictionary’s value is used (default behavior). |
Sum Values | Combine values by summing them if they are numeric. |
Custom Function | Use a custom merge function to define how to combine values. |
Example of summing values for duplicate keys:
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
combined = {}
for d in (dict1, dict2):
for k, v in d.items():
combined[k] = combined.get(k, 0) + v
combined is {‘a’: 1, ‘b’: 5, ‘c’: 4}
“`
This method provides flexibility for more complex merging logic.
Expert Insights on Merging Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Combining two dictionaries in Python can be efficiently accomplished using the `update()` method, which allows you to merge one dictionary into another. Alternatively, Python 3.9 introduced the merge (`|`) operator, which provides a more intuitive syntax for this operation.”
Michael Chen (Data Scientist, AI Solutions Group). “When merging dictionaries, it is crucial to consider how to handle key collisions. The `collections.ChainMap` class can be a useful tool for maintaining the original dictionaries while providing a unified view of their contents.”
Sarah Thompson (Python Developer, CodeCraft Academy). “For those looking for a concise approach, dictionary comprehensions offer a powerful way to merge dictionaries while applying transformations to the keys or values. This method is particularly useful when working with larger datasets.”
Frequently Asked Questions (FAQs)
How can I combine two dictionaries in Python?
You can combine two dictionaries in Python using the `update()` method, the `` unpacking operator, or the `dict` constructor. For example, `dict1.update(dict2)` modifies `dict1` in place, while `combined = {dict1, **dict2}` creates a new dictionary.
What happens if there are duplicate keys when combining dictionaries?
When combining dictionaries with duplicate keys, the values from the second dictionary will overwrite those from the first. For example, if both dictionaries have the same key, the value from the second dictionary will be retained in the combined result.
Can I combine dictionaries with different data types for keys and values?
Yes, you can combine dictionaries with different data types for keys and values in Python. The keys must be hashable, but values can be of any data type, including lists, tuples, or other dictionaries.
Is there a method to combine dictionaries in Python 3.9 and later?
Yes, in Python 3.9 and later, you can use the merge operator (`|`) to combine dictionaries. For example, `combined = dict1 | dict2` creates a new dictionary that merges both.
How do I combine multiple dictionaries at once?
You can combine multiple dictionaries using the `` unpacking operator in a single expression, like `combined = {dict1, dict2, dict3}`. Alternatively, you can use the `ChainMap` from the `collections` module for a more dynamic approach.
Are there performance considerations when combining large dictionaries?
Yes, performance can be a consideration when combining large dictionaries. Using methods like `update()` modifies the dictionary in place and may be more memory efficient, while creating a new dictionary with unpacking may require additional memory.
Combining two dictionaries in Python can be accomplished using several methods, each suited for different scenarios and Python versions. The most common approaches include using the `update()` method, the unpacking operator `**`, and the `dict` constructor. Each method has its own advantages, depending on whether you want to modify an existing dictionary or create a new one.
The `update()` method directly modifies the first dictionary by adding key-value pairs from the second dictionary. This is a straightforward approach but does not create a new dictionary. In contrast, using the unpacking operator `**` allows for the creation of a new dictionary that merges both dictionaries without altering the originals. This method is particularly useful when immutability is a concern.
For those using Python 3.9 or later, the `|` operator provides a concise and intuitive way to combine dictionaries. This operator allows for an elegant syntax that enhances code readability. Regardless of the method chosen, it is important to consider how duplicate keys are handled, as the latter dictionary will overwrite values from the former in cases of key collisions.
In summary, understanding the various techniques for combining dictionaries in Python is essential for effective programming. Each method offers unique benefits, and the choice
Author Profile
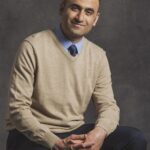
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?