How Can You Effectively Comment a Block of Code in Python?
In the world of programming, clarity and communication are just as vital as the code itself. Whether you’re collaborating with a team or revisiting your own work after some time, well-placed comments can make all the difference. Among the various programming languages, Python stands out for its simplicity and readability, yet even the most straightforward code can benefit from thoughtful commentary. If you’ve ever wondered how to effectively comment a block of code in Python, you’re not alone. This article will guide you through the nuances of commenting, helping you enhance your code’s readability and maintainability.
Commenting in Python is not merely a matter of adding notes; it’s an art that can elevate your coding practice. By learning how to comment a block of code effectively, you can provide context, explain complex logic, and clarify the purpose of your functions. This practice not only aids others who may read your code but also serves as a helpful reminder for yourself when you return to your projects after a break.
In this article, we will explore the various methods of commenting in Python, focusing on how to comment out blocks of code efficiently. We’ll discuss the differences between single-line and multi-line comments, and how to use them strategically to enhance your code. Whether you’re a beginner or a seasoned programmer
Commenting Multiple Lines in Python
In Python, there is no direct syntax for block comments as seen in some other programming languages. However, there are effective methods to achieve the same result by using either multi-line strings or repeated single-line comments.
To comment a block of code, you can utilize triple quotes. While triple quotes are primarily intended for multi-line strings, they can also serve as comments when not assigned to a variable. Here’s how you can do it:
python
“””
This is a block of code that is commented out.
The code below will not execute.
print(“This will not run.”)
“””
Although this method is common, it is essential to note that using triple quotes for commenting can lead to confusion since they are not true comments but rather string literals that are not used. A clearer approach is to use the pound sign (#) for single-line comments.
Using Single-Line Comments
For commenting out multiple lines, simply prefix each line with the pound sign. This method is straightforward and widely accepted among Python developers. Here’s an example:
python
# This is the first line of the comment
# This is the second line of the comment
# This code will not execute
# print(“This will not run.”)
This approach allows for a more explicit indication of comments, making it clear to anyone reading the code.
Best Practices for Commenting Code
When commenting blocks of code in Python, consider the following best practices:
- Clarity: Comments should clearly explain why the code exists and what it does.
- Relevance: Keep comments relevant to the code they describe. Avoid redundant comments that merely restate what the code does.
- Brevity: Be concise. Long comments can be overwhelming and reduce code readability.
- Updates: Regularly update comments to reflect changes in the codebase to prevent misinformation.
Here is a table summarizing the different comment methods:
Method | Syntax | Use Case |
---|---|---|
Triple Quotes | “”” comment “”” | Multi-line comments (not recommended for production) |
Single-Line Comments | # comment | Best practice for commenting individual lines |
By adhering to these guidelines, developers can maintain a clean and understandable codebase, allowing for easier collaboration and maintenance.
Using Triple Quotes for Block Comments
In Python, one common method for commenting out blocks of code is by using triple quotes. This approach is particularly effective for multi-line comments. Here’s how it works:
- You can use either triple single quotes (`”’`) or triple double quotes (`”””`).
- The text enclosed within these triple quotes is ignored by the Python interpreter.
Example:
python
”’
This is a block of code that will not be executed.
It can span multiple lines.
”’
print(“This line will run.”)
Using the Hash Symbol for Individual Lines
Another method for commenting on multiple lines of code is to prefix each line with the hash symbol (`#`). This is suitable for shorter comments or when you want to comment out specific lines within a block of code.
Example:
python
# This line is commented out
# print(“This line will not run.”)
print(“This line will run.”)
Creating a Commenting Function
For scenarios where you may want to toggle comments on and off in a block of code, you can create a simple function that utilizes string literals. Though not conventional, it can be useful for larger blocks.
Example:
python
def comment_out():
“””
This entire block of code is treated as a comment.
You can return to it later or modify it as needed.
“””
print(“This line is commented out as part of the function.”)
Commenting Best Practices
When using comments to enhance code readability, consider the following best practices:
- Clarity: Ensure comments are clear and provide meaningful explanations of the code.
- Consistency: Use a consistent commenting style throughout the codebase.
- Relevance: Comment on why a certain piece of code exists, especially if it’s not immediately obvious.
- Avoid Redundancy: Do not restate what the code itself is doing, focus on why it is doing it.
Commenting Method | Pros | Cons |
---|---|---|
Triple Quotes | Easy for multi-line comments | Can be misinterpreted as string literals |
Hash Symbol | Simple and straightforward | Requires each line to be prefixed |
Custom Comment Function | Useful for large blocks | Not a standard practice |
Using IDE Features for Commenting
Many Integrated Development Environments (IDEs) provide features to comment or uncomment multiple lines of code easily. These features typically involve keyboard shortcuts:
- Visual Studio Code: Select the lines and press `Ctrl + /` to toggle comments.
- PyCharm: Use `Ctrl + /` for single-line comments or `Ctrl + Shift + /` for block comments.
- Jupyter Notebooks: Use `#` for individual lines; comments within markdown cells can also be utilized.
Utilizing these IDE features can greatly enhance your efficiency when managing comments in your code.
Expert Insights on Commenting Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Commenting a block of code in Python can be effectively achieved using triple quotes. This method not only allows for multi-line comments but also enhances code readability, making it easier for developers to understand the purpose of the code.”
Michael Chen (Lead Python Developer, Tech Innovations Inc.). “Utilizing triple quotes for commenting is a best practice in Python. It serves as a clear indication of non-executable code, which is particularly useful during the debugging process or when collaborating with a team.”
Sarah Johnson (Technical Writer, Python Programming Journal). “In Python, while single-line comments are created using the hash symbol, for block comments, triple quotes are preferred. This technique allows developers to encapsulate a large section of code, providing context without cluttering the codebase.”
Frequently Asked Questions (FAQs)
How do I comment a block of code in Python?
To comment a block of code in Python, you can use triple quotes (`”’` or `”””`). This method allows you to create multi-line comments that can span several lines.
Can I use the hash symbol (#) for multi-line comments?
While the hash symbol (`#`) is used for single-line comments, you can use it at the beginning of each line to create multi-line comments. However, this is less efficient than using triple quotes.
What is the purpose of commenting code?
Commenting code serves to explain the logic and functionality, making it easier for others (and yourself) to understand the code’s intent and structure when revisiting it later.
Are comments ignored during the execution of a Python program?
Yes, comments are entirely ignored by the Python interpreter during execution. They do not affect the program’s performance or output.
Is there a difference between inline comments and block comments?
Yes, inline comments are placed on the same line as a statement and typically provide brief explanations, while block comments are used to describe larger sections of code and can span multiple lines.
What are best practices for writing comments in Python?
Best practices include writing clear and concise comments, avoiding obvious statements, and ensuring comments are updated alongside code changes to maintain accuracy and relevance.
In Python, commenting a block of code can be effectively achieved using multi-line string literals or the hash symbol. While Python does not have a dedicated syntax for multi-line comments like some other programming languages, developers often utilize triple quotes (”’ or “””) to create comments that span multiple lines. This method allows for clear documentation of complex code sections and enhances code readability.
Another approach is to use the hash symbol (#) at the beginning of each line for single-line comments. Although this method requires more effort for larger blocks of code, it is a straightforward technique that many developers are familiar with. Combining both methods can provide flexibility depending on the context and the amount of commentary needed.
Overall, effective commenting practices in Python are essential for maintaining code clarity and facilitating collaboration among developers. By using multi-line string literals or hash symbols appropriately, programmers can ensure that their code is well-documented and easier to understand for themselves and others who may work on the code in the future.
Author Profile
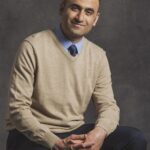
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?