How Can You Effectively Comment a Block of Code in Python?
### Introduction
In the world of programming, clarity is key. As developers, we often find ourselves revisiting our code after days, weeks, or even months, and the last thing we want is to decipher our own logic. This is where commenting comes into play, serving as a vital tool for enhancing code readability and maintainability. Among the various languages, Python stands out for its simplicity and elegance, but even in its straightforward syntax, knowing how to effectively comment a block of code can significantly impact your workflow and collaboration with others.
Commenting in Python isn’t just about leaving notes for yourself; it’s about creating a clear narrative that guides anyone who might read your code in the future. Whether you’re working on a personal project or contributing to a larger team effort, understanding how to comment blocks of code allows you to convey complex ideas succinctly and efficiently. In this article, we will explore the different methods of commenting in Python, focusing on how to effectively block comment sections of your code.
By mastering the art of commenting, you can enhance your programming practices, making your code not only functional but also accessible and easy to understand. As we delve deeper, you’ll discover the nuances of Python’s commenting capabilities, ensuring that your code communicates its purpose clearly and effectively. Prepare to elevate
Using Multi-line Strings for Block Comments
In Python, a common way to create block comments is by using multi-line strings. This method allows you to comment out multiple lines of code effectively. You can use triple quotes (either single `”’` or double `”””`) to encapsulate your comments. Although these are technically string literals, when not assigned to a variable, they function as comments.
Example:
python
”’
This is a block comment.
It can span multiple lines.
”’
print(“Hello, World!”)
This approach is particularly useful for temporarily disabling sections of code during testing or debugging.
Utilizing the Hash Symbol for Single-line Comments
For single-line comments, Python employs the hash symbol (`#`). Each line that you wish to comment out must begin with this symbol. While this does not create a traditional block comment, it is often used for shorter comments or annotations within the code.
Example:
python
# This is a single-line comment
print(“Hello, World!”) # This prints a greeting
For longer comments, you can stack multiple single-line comments on top of each other:
python
# This is the first line of a comment
# This is the second line of a comment
# This is the third line of a comment
print(“Hello, World!”)
Best Practices for Commenting Code
When commenting your code, consider the following best practices to ensure clarity and maintainability:
- Be Descriptive: Comments should explain what the code is doing, why a particular approach was taken, or any important notes regarding the functionality.
- Keep Comments Up-to-date: As the code changes, ensure that comments are updated to reflect any modifications.
- Avoid Redundant Comments: Comments that restate the obvious can clutter the code. Instead, aim for insights that add value to the reader.
Commenting Styles in Python
Different developers may have varying styles for commenting. Below is a comparison of common styles:
Style | Description | Example |
---|---|---|
Multi-line Strings | Utilizes triple quotes for longer explanations. | python ”’ This is a multi-line comment. ”’ |
Single-line Comments | Uses `#` for short comments, usually one per line. | python # Comment here |
Docstrings | Special multi-line strings for documenting functions/classes. | python def func(): ”’This is a docstring.”’ |
By adhering to these practices and styles, you can ensure that your code remains readable and maintainable for yourself and others who may work with it in the future.
Commenting Single Lines of Code
In Python, you can comment a single line of code using the hash symbol (`#`). Any text following the `#` on that line will be ignored by the Python interpreter. This is useful for adding explanations or notes to your code.
Example:
python
# This is a single line comment
print(“Hello, World!”) # This will print a greeting
Commenting Multiple Lines of Code
To comment out multiple lines in Python, you have a couple of options. However, Python does not have a built-in multi-line comment feature like some other programming languages. The most common methods include:
- Using multiple single-line comments:
python
# This is the first line of the comment
# This is the second line of the comment
print(“Hello, World!”)
- Using triple quotes (though primarily for docstrings):
python
“””
This is a multi-line comment
using triple quotes.
It can span multiple lines.
“””
print(“Hello, World!”)
While triple quotes are typically used for string literals, they can serve to comment out blocks of code, as the interpreter will ignore them if they are not assigned to a variable.
Best Practices for Commenting Code
When commenting your code, consider the following best practices to enhance readability and maintainability:
- Be concise and clear: Comments should explain the “why” behind the code, not restate the obvious.
- Keep comments up to date: Always revise comments when you change the associated code to avoid confusion.
- Use comments to explain complex logic: If a piece of code is not immediately understandable, provide a comment to clarify its purpose.
- Avoid excessive commenting: Too many comments can clutter the code. Aim for a balance.
Example of Effective Commenting
Consider the following code snippet that calculates the factorial of a number:
python
def factorial(n):
# Return 1 if n is 0 or 1 (base case)
if n == 0 or n == 1:
return 1
else:
# Recursive case: n * factorial of n-1
return n * factorial(n – 1)
In this example, comments are used effectively to explain the base case and the recursive case of the factorial function, making the code easier to understand for future readers.
Common Mistakes to Avoid
When commenting code, be mindful of these common pitfalls:
Mistake | Description |
---|---|
Commenting obvious code | Avoid stating the obvious; focus on the logic instead. |
Outdated comments | Keep comments synchronized with code changes. |
Over-commenting | Too many comments can detract from readability. |
Misleading comments | Ensure comments accurately reflect the code’s functionality. |
By adhering to these guidelines, you will create a more maintainable codebase that is easier for both you and others to understand.
Expert Insights on Commenting Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting blocks of code in Python is essential for maintaining readability and collaboration. Utilizing triple quotes for multi-line comments is a common practice, as it allows developers to encapsulate explanations or disable sections of code without affecting execution.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “In Python, while there is no built-in syntax for block comments, developers can effectively use multi-line strings as comments. This approach not only documents the code but also helps in debugging by allowing sections to be easily commented out during development.”
Lisa Patel (Technical Writer, Python Programming Journal). “Effective commenting practices are crucial for code maintenance. In Python, using the hash symbol for single-line comments and triple quotes for larger blocks can significantly improve code clarity, making it easier for teams to understand the logic behind complex implementations.”
Frequently Asked Questions (FAQs)
How do I comment a single line of code in Python?
To comment a single line of code in Python, use the hash symbol `#` at the beginning of the line. Everything following the `#` on that line will be treated as a comment and ignored by the interpreter.
What is the syntax for multi-line comments in Python?
Python does not have a specific multi-line comment syntax. However, you can use triple quotes (`”’` or `”””`) to create a string that is not assigned to a variable, effectively acting as a multi-line comment.
Can I use the `#` symbol for multi-line comments?
While you can use `#` for each line to create multi-line comments, this is not efficient. It is recommended to use triple quotes for better readability and organization.
Are comments ignored by the Python interpreter?
Yes, comments are ignored by the Python interpreter. They serve only as annotations or explanations for the code, making it easier for developers to understand the logic.
What is the purpose of commenting code in Python?
Commenting code enhances readability, provides context for complex logic, and aids in collaboration by allowing other developers to understand the code’s intent without deciphering it.
Can I comment out a block of code temporarily in Python?
Yes, you can comment out a block of code temporarily by placing `#` before each line or using triple quotes to enclose the block. This allows you to disable code without deleting it.
In Python, commenting a block of code can be accomplished through several techniques, which enhance code readability and maintainability. The most common method is to use the hash symbol (#) for single-line comments. However, for multi-line or block comments, Python does not have a specific syntax like some other programming languages. Instead, developers often use triple quotes (”’ or “””) to create a string that is not assigned to a variable, effectively serving as a comment.
Utilizing triple quotes is particularly useful for documenting larger sections of code or providing detailed explanations. While this method does not technically create a comment, it is a widely accepted practice among Python developers. Additionally, developers can also use the built-in `pydoc` or docstring conventions to provide documentation for functions, classes, and modules, which can be beneficial for both the developer and users of the code.
In summary, while Python does not have a dedicated syntax for block comments, using triple quotes is an effective workaround. It is essential to prioritize code clarity through proper commenting practices, as this can significantly improve collaboration and code maintenance in any programming project. By adhering to these conventions, developers can ensure that their code is not only functional but also understandable to others who may work
Author Profile
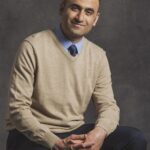
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?