How Can You Effectively Comment Out a Block of Code in Python?
### Introduction
In the world of programming, clarity is key. Whether you’re collaborating with a team or revisiting your own code after a long break, being able to effectively comment out sections of your code can make all the difference. In Python, this practice not only enhances readability but also allows developers to debug and test their code more efficiently. If you’ve ever found yourself tangled in a web of code, unsure of how to temporarily disable certain parts without deleting them, you’re in the right place. This article will guide you through the essential techniques for commenting out blocks of code in Python, ensuring your coding experience is as smooth and productive as possible.
Commenting out code is an invaluable skill for any programmer. It serves multiple purposes, from making notes for future reference to preventing specific lines from executing during testing. In Python, the syntax for comments is straightforward, but knowing how to apply it effectively can elevate your coding practices. Understanding the difference between single-line and multi-line comments is crucial, as each serves its own unique function in the development process.
As we delve deeper into this topic, you’ll discover various methods to comment out blocks of code, along with best practices to keep your codebase clean and understandable. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to
Commenting Out Code in Python
In Python, commenting out a block of code is a straightforward process, but it’s essential to understand the methods available to achieve this effectively. Commenting is crucial for making code more readable and for temporarily disabling parts of your code during debugging or development.
Using the Hash Symbol
The most common way to comment in Python is by using the hash symbol (`#`). This method allows you to comment out single lines or add inline comments. However, if you want to comment out multiple lines, you’ll need to prepend the hash symbol to each line individually.
Example:
python
# This is a single line comment
# This is a comment
# that spans multiple
# lines.
Using Triple Quotes
While Python doesn’t have a specific syntax for multi-line comments, you can use triple quotes (`”’` or `”””`) to achieve a similar effect. Triple quotes are primarily used for multi-line strings, but if they are not assigned to a variable, they effectively act as comments.
Example:
python
”’
This is a block of text
that is treated as a comment
by Python.
”’
Note: While using triple quotes can serve the purpose of commenting out code, it is not the preferred method for this specific task since it creates a string object.
Best Practices for Commenting
When commenting code, consider the following best practices:
- Be clear and concise: Write comments that are easy to understand.
- Avoid obvious comments: Do not state the obvious; focus on explaining the “why” rather than the “what.”
- Update comments: Ensure comments are updated when the code changes to maintain accuracy.
- Use docstrings for functions and classes: Utilize triple quotes to describe the purpose and usage of functions and classes.
Commenting Techniques Comparison
The following table summarizes the differences between the two main commenting techniques:
Technique | Use Case | Example |
---|---|---|
Hash Symbol (`#`) | Single-line comments or multiple lines (each line must start with `#`) | # This is a comment |
Triple Quotes (`”’` or `”””`) | Multi-line comments, typically used for documentation | '''This is a comment''' |
By following these guidelines and utilizing the appropriate techniques, you can effectively manage comments in your Python code, enhancing both readability and maintainability.
Commenting Out a Block of Code in Python
In Python, there are no native multiline comment syntax options like those found in some other programming languages. However, there are effective ways to comment out blocks of code using either multiple single-line comments or multiline strings.
Using Multiple Single-Line Comments
The most straightforward method to comment out a block of code is to prepend each line with the hash symbol (`#`). This approach is simple and widely used.
Example:
python
# print(“This line will not execute”)
# print(“This is another line that is commented out”)
To make commenting more efficient, especially in larger code blocks, many text editors and IDEs offer shortcuts to comment out multiple lines at once. Here are common keyboard shortcuts:
Editor/IDE | Windows Shortcut | Mac Shortcut |
---|---|---|
Visual Studio Code | Ctrl + / | Cmd + / |
PyCharm | Ctrl + / | Cmd + / |
Jupyter Notebook | Ctrl + / | Cmd + / |
Using Multiline Strings
Another method to comment out a block of code is by using triple quotes (`”’` or `”””`). Although this is technically a string and not a comment, if the string is not assigned to a variable, Python will ignore it during execution.
Example:
python
”’
print(“This line will not execute”)
print(“This is another line that is commented out”)
”’
### Important Considerations
- Readability: Using multiple single-line comments can improve code readability, especially for others reviewing your code.
- Code Execution: Strings created with triple quotes are still part of the code structure; thus, they can be inadvertently executed if used incorrectly. Therefore, they should be used cautiously.
- Documentation: If you intend to provide a block of documentation rather than a comment, triple quotes are more appropriate as they can be utilized for docstrings.
Best Practices for Commenting
When commenting out blocks of code, consider the following best practices:
- Clarity: Use comments to clearly indicate why a block of code is commented out. This aids in future debugging and enhances collaboration.
- Consistent Style: Maintain a consistent commenting style throughout your codebase.
- Temporary Comments: If you are commenting out code temporarily during debugging, consider adding a note on what you plan to revisit.
By utilizing single-line comments or multiline strings, Python developers can effectively comment out blocks of code, ensuring maintainability and clarity in their programming practices.
Expert Insights on Commenting Out Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). “In Python, the most straightforward way to comment out a block of code is to use the hash symbol (#) before each line. However, for larger blocks, using triple quotes (”’ or “””) can also be effective, as it allows you to temporarily disable a section of code without needing to prefix each line individually.”
James Liu (Lead Python Developer, Tech Solutions Group). “While the hash symbol is the conventional method for single-line comments, it’s important to note that Python does not have a built-in multi-line comment feature. Instead, developers often utilize triple quotes, which are typically reserved for docstrings, to comment out multiple lines during the development phase.”
Sarah Thompson (Python Programming Instructor, Code Academy). “When teaching Python, I emphasize the importance of clarity in code. Commenting out blocks of code using triple quotes can serve as a temporary solution, but I advise students to remove them once they finalize their code to maintain readability and avoid confusion in collaborative environments.”
Frequently Asked Questions (FAQs)
How do you comment out a single line of code in Python?
To comment out a single line of code in Python, simply place a hash symbol (`#`) at the beginning of the line. This will prevent the interpreter from executing that line.
Is there a way to comment out multiple lines of code in Python?
Python does not have a built-in multi-line comment feature. However, you can comment out multiple lines by placing a `#` at the beginning of each line or by using triple quotes (`”’` or `”””`) to create a string that is not assigned to any variable.
What happens if you use triple quotes for comments?
Using triple quotes creates a string literal that is not assigned to a variable, effectively acting as a comment. However, this approach is not technically a comment and can lead to unintended behavior if the string is inadvertently used or if it appears in a function.
Can you use a block comment in Python?
Python does not support block comments in the same way some other languages do. You can simulate a block comment by using multiple single-line comments or triple quotes, but the latter should be used with caution.
Are there any best practices for commenting code in Python?
Best practices include writing clear, concise comments that explain the purpose of the code, avoiding obvious comments, and keeping comments up to date with code changes. Use comments to clarify complex logic or to provide context for future readers.
Can comments affect the performance of a Python program?
Comments do not affect the performance of a Python program, as they are ignored by the interpreter during execution. However, excessive or poorly written comments can make the code harder to read and maintain, which may impact development efficiency.
In Python, commenting out a block of code is essential for improving code readability and maintaining clean code practices. While Python does not have a built-in multi-line comment feature like some other programming languages, developers can achieve this by using triple quotes or by employing the hash symbol (#) for individual lines. Triple quotes allow for the creation of multi-line strings that can serve as comments, while the hash symbol is used to comment out single lines or each line of a block of code individually.
It is important to note that using triple quotes for comments is not a conventional practice, as they are primarily intended for docstrings. Therefore, the preferred method for commenting out multiple lines is to place a hash symbol at the beginning of each line. This method ensures clarity and aligns with Python’s design philosophy, which emphasizes readability and simplicity.
In summary, effectively commenting out blocks of code in Python can be accomplished through careful use of the hash symbol or triple quotes. Understanding these techniques not only aids in debugging and maintaining code but also fosters collaboration among developers by providing context and explanations for complex sections of code. Adopting best practices in commenting will ultimately lead to more maintainable and comprehensible codebases.
Author Profile
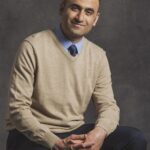
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?