How Can You Comment Out an Entire Section in Python?
In the world of programming, clarity and organization are paramount. As developers, we often find ourselves needing to temporarily disable sections of code or leave notes for future reference. This is where the art of commenting comes into play, particularly in Python, a language renowned for its readability and simplicity. Understanding how to comment out a whole section of code not only enhances the maintainability of your projects but also streamlines collaboration with fellow programmers. In this article, we will explore effective techniques for commenting out blocks of code in Python, ensuring your scripts remain clean and comprehensible.
When working with Python, it’s essential to know that comments are an integral part of code documentation. They allow you to annotate your code, making it easier for others (and yourself) to understand the intent behind your logic. Commenting out sections of code can be particularly useful during debugging or when you want to test different functionalities without deleting existing code. Python provides a few straightforward methods to achieve this, catering to both single-line and multi-line comments.
In the following sections, we will delve into the various strategies for commenting out entire sections in Python, highlighting best practices and common pitfalls to avoid. Whether you’re a seasoned developer or just starting your programming journey, mastering these techniques will empower you to write cleaner, more
Commenting Out Code in Python
In Python, commenting out a whole section of code can be accomplished using various techniques, each suited for different scenarios. Python does not have a built-in multi-line comment syntax like some other programming languages, but there are effective methods to achieve the same result.
One common approach is to use the hash symbol (“) at the beginning of each line. This method is straightforward but can be cumbersome if you have many lines to comment out.
For example:
“`python
This is a comment
This is another comment
print(“This line is commented out”)
“`
To make this process easier in some code editors, you can often select multiple lines and use a keyboard shortcut to comment them all at once. For instance:
- In Visual Studio Code, you can use `Ctrl + /` (or `Cmd + /` on macOS).
- In PyCharm, the same shortcut applies.
Another method to comment out a block of code is to use triple quotes (`”’` or `”””`). While primarily used for multi-line strings or docstrings, enclosing a section of code in triple quotes effectively prevents it from executing. However, this technique is generally discouraged for commenting out code since it can lead to unintended behavior if the enclosed code is interpreted as a string.
Example using triple quotes:
“`python
”’
print(“This will not run”)
print(“This will also not run”)
”’
“`
Best Practices for Commenting
When commenting out sections of code, it is advisable to follow best practices to maintain code readability and clarity. Consider the following guidelines:
- Use Comments Judiciously: Avoid excessive commenting. Focus on complex logic that may not be immediately clear to others.
- Be Descriptive: If you need to comment out code, explain why it is being done. This helps others (or your future self) understand the context.
- Temporary Comments: If the code is temporarily disabled for testing purposes, indicate that it is a temporary measure.
Comparison of Commenting Methods
The table below summarizes the different methods for commenting out code in Python, highlighting their advantages and disadvantages.
Method | Advantages | Disadvantages |
---|---|---|
Hash Symbol (“) | Simple and clear; widely understood. | Time-consuming for large blocks of code. |
Triple Quotes (`”’` or `”””`) | Easy to comment out multiple lines. | Can lead to confusion if interpreted as a string; not intended for comments. |
Editor Shortcuts | Efficient for large blocks; quick toggle. | Requires familiarity with the editor; may not work in all environments. |
By understanding these methods and their implications, developers can effectively manage code comments, ensuring that their code remains clean and maintainable.
Commenting Out a Section of Code in Python
In Python, there is no built-in multi-line comment feature like in some other programming languages. However, you can effectively comment out a whole section of code using several methods. The most common approaches include using the hash symbol (“), multi-line strings, or code editors that support commenting out blocks.
Using the Hash Symbol
The simplest way to comment out multiple lines in Python is to place a “ at the beginning of each line. This method is straightforward but can be cumbersome for larger sections of code.
Example:
“`python
This is a comment
This is another comment
The following line is commented out
print(“This line won’t execute”)
“`
Using Multi-line Strings
Another effective method is to use triple quotes (`”’` or `”””`). While this is technically for defining strings, it can be used to comment out code since Python ignores these strings if they are not assigned to a variable.
Example:
“`python
”’
print(“This line won’t execute”)
print(“This line also won’t execute”)
”’
“`
It’s important to note that using this method can lead to unintended behavior if the triple-quoted string appears in a context where it is executed, like within a function.
Using Code Editors and IDEs
Most modern code editors and Integrated Development Environments (IDEs) provide functionality to comment out blocks of code quickly. The methods vary by editor:
- VSCode: Select the lines you want to comment out and press `Ctrl + /` (Windows/Linux) or `Cmd + /` (Mac).
- PyCharm: Highlight the lines and press `Ctrl + /` (Windows/Linux) or `Cmd + /` (Mac) to toggle comments.
- Jupyter Notebook: Highlight the lines and press `Ctrl + /` to comment out the selected lines.
Table of Commenting Methods
Method | Description | Example |
---|---|---|
Hash Symbol () | Prepend to each line. |
print("Line 1")
|
Triple Quotes | Use triple quotes to enclose code. |
'''
|
IDE/Editor Shortcuts | Use built-in shortcuts for commenting. |
Ctrl + / (or Cmd + /)
|
By employing these methods, you can effectively comment out sections of code in Python, enhancing the readability and maintainability of your scripts.
Expert Insights on Commenting Out Sections in Python
Dr. Emily Carter (Senior Software Developer, CodeCraft Solutions). “In Python, the most effective way to comment out a whole section of code is to use triple quotes. This method allows you to encapsulate multiple lines without affecting the execution of the code, making it a practical choice for temporarily disabling large blocks.”
Michael Tran (Lead Python Instructor, Tech Academy). “While single-line comments using the hash symbol are common, for larger sections, I recommend using triple quotes. This approach not only keeps your code clean but also allows for easy reactivation of the commented section when needed.”
Sarah Lopez (Python Software Engineer, Innovatech). “Commenting out entire sections in Python can be efficiently done with triple quotes. However, developers should be cautious, as this method can sometimes lead to confusion if not properly documented, especially in collaborative environments.”
Frequently Asked Questions (FAQs)
How can I comment out a whole section in Python?
To comment out a whole section in Python, you can use triple quotes (`”’` or `”””`). Enclose the section of code you want to comment out within these quotes, and Python will treat it as a multi-line string, effectively ignoring it during execution.
Is there a specific syntax for single-line comments in Python?
Yes, single-line comments in Python are created using the hash symbol (“). Any text following this symbol on the same line will be considered a comment and will not be executed.
Can I use the hash symbol to comment out multiple lines?
While you can use the hash symbol for each line individually, it is not efficient for commenting out multiple lines. Using triple quotes is a more effective approach for larger sections of code.
Are there any tools or IDE features that help with commenting out code in Python?
Many Integrated Development Environments (IDEs) and code editors, such as PyCharm, Visual Studio Code, and Jupyter Notebook, offer features to comment and uncomment selected lines of code quickly, typically through keyboard shortcuts.
What happens if I use triple quotes incorrectly?
If you use triple quotes incorrectly, such as leaving them unclosed, Python will raise a syntax error. Ensure that every opening triple quote has a corresponding closing quote.
Can I use comments to document my code effectively?
Yes, comments are an essential part of documenting code. They help explain the purpose of code sections, making it easier for others (or yourself) to understand the logic and functionality in the future.
In Python, commenting out a whole section of code can be achieved using several methods, each with its own advantages. The most common approach is to use the hash symbol () at the beginning of each line you wish to comment out. This method is straightforward and allows for easy identification of commented lines, making it ideal for short sections of code.
For larger blocks of code, Python does not have a built-in multi-line comment syntax like some other programming languages. However, developers often use triple quotes (”’ or “””) as a workaround. While this is primarily intended for multi-line strings, it can effectively serve as a comment when not assigned to a variable. This technique is particularly useful for temporarily disabling larger sections of code during debugging or development.
It is essential to understand the context in which comments are used. Properly commenting code improves readability and maintainability, allowing other developers (or your future self) to understand the purpose and functionality of the code. Therefore, choosing the appropriate method for commenting out code sections is crucial for effective collaboration and code management.
Author Profile
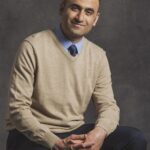
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?