How Can You Easily Comment Out a Block of Code in Python?
In the world of programming, clarity and organization are paramount. As developers, we often find ourselves in situations where we need to temporarily disable a section of code without deleting it. This is where the concept of commenting comes into play, serving as a powerful tool for enhancing code readability and managing complex projects. If you’ve ever wondered how to comment out a block of code in Python, you’re not alone. Mastering this skill can significantly streamline your coding process and improve collaboration with others.
Commenting out code is not just about hiding lines; it’s about creating a narrative within your code. It allows you to annotate your thought process, explain complex logic, or simply mark sections for future reference. In Python, the approach to commenting out blocks of code is straightforward, yet it can vary depending on the context and the desired outcome. Whether you’re debugging, experimenting with new features, or collaborating with teammates, knowing how to effectively comment out code can save you time and prevent confusion.
As we delve deeper into the mechanics of commenting in Python, we will explore the various methods available to you. From single-line comments to multi-line strategies, understanding these techniques will empower you to write cleaner, more maintainable code. So, let’s unlock the secrets of commenting in Python and elevate your
Commenting Out Code in Python
In Python, there are two primary methods to comment out blocks of code: using the hash symbol () and using multi-line string literals. Each method serves distinct purposes and can be utilized effectively depending on the situation.
Using the Hash Symbol
The most common way to comment out a single line of code in Python is by prefixing the line with the hash symbol (). This method is straightforward and ideal for brief comments.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a greeting message
“`
To comment out multiple lines of code using the hash symbol, you must place a hash symbol at the beginning of each line. This can be somewhat tedious for longer blocks of code.
Example:
“`python
This is the first line of a comment
This is the second line of a comment
This is the third line of a comment
print(“Hello, World!”)
“`
Using Multi-line String Literals
An alternative method to comment out multiple lines of code is to use multi-line string literals. By enclosing text in triple quotes (either `”’` or `”””`), you can create a block of text that is ignored by the Python interpreter, effectively acting as a comment.
Example:
“`python
“””
This is a multi-line comment.
It can span multiple lines.
“””
print(“Hello, World!”)
“`
This method is especially useful for longer comments or for temporarily disabling blocks of code without modifying each line individually.
Comparison of Commenting Methods
The following table summarizes the differences between the two commenting methods:
Method | Syntax | Use Case |
---|---|---|
Single-line Comment | Quick comments or disabling single lines | |
Multi-line Comment | ”’ or “”” | Longer comments or disabling multiple lines |
Best Practices
When commenting code, consider the following best practices to enhance code readability and maintainability:
- Be Clear and Concise: Ensure comments are straightforward and to the point. Avoid unnecessary verbosity.
- Update Comments: Regularly review and update comments to reflect any changes in the code logic.
- Avoid Redundant Comments: Do not comment on self-explanatory code. Comments should add value and clarification where necessary.
- Use Comments to Explain Why: Instead of explaining what the code does, focus on the reasoning behind complex logic or decisions.
By adhering to these practices, you can create well-documented code that is easier to understand and maintain over time.
Commenting Out Blocks of Code in Python
In Python, commenting out a block of code is essential for debugging, documentation, and maintaining code readability. There are several methods to accomplish this, each with its specific use cases.
Using the Hash Symbol ()
The most common way to comment out a single line or a block of code is by using the hash symbol (“). However, when it comes to multi-line comments, each line must begin with a hash symbol.
Example:
“`python
This is a single-line comment
This is another line of comment
print(“This line is commented out”)
print(“This line is also commented out”)
“`
For longer blocks, this can become tedious, but it is straightforward.
Using Triple Quotes
Another method to comment out a block of code is by using triple quotes (`”’` or `”””`). While primarily used for multi-line strings, Python ignores these strings when they are not assigned to a variable or used in any expression.
Example:
“`python
“””
print(“This line is commented out”)
print(“This line is also commented out”)
“””
This line will execute
print(“This line will run”)
“`
Note: This approach is not technically a comment but effectively ignores the code within the triple quotes.
Using IDE Features
Many Integrated Development Environments (IDEs) and code editors provide built-in features for commenting out blocks of code. Here are some common shortcuts:
IDE/Editor | Windows Shortcut | Mac Shortcut |
---|---|---|
PyCharm | `Ctrl + /` | `Cmd + /` |
Visual Studio Code | `Ctrl + /` | `Cmd + /` |
Sublime Text | `Ctrl + /` | `Cmd + /` |
These shortcuts allow you to comment or uncomment selected lines quickly, enhancing productivity.
Best Practices for Commenting Code
When commenting out code, consider the following best practices:
- Clarity: Ensure comments clearly state the intention behind the code.
- Consistency: Use the same commenting style throughout the codebase to maintain uniformity.
- Necessity: Avoid excessive comments. Only comment on complex logic or sections that may confuse other developers.
- Maintenance: Regularly review and update comments to ensure they remain relevant to the code’s functionality.
Mastering the techniques for commenting out blocks of code is crucial for effective Python programming. By using the hash symbol, triple quotes, and leveraging IDE features, developers can enhance code readability and maintainability.
Expert Insights on Commenting Out Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, CodeWise Solutions). Commenting out blocks of code in Python is essential for debugging and maintaining code clarity. Utilizing the triple quotes method allows developers to easily disable sections of code without removing them, which is particularly useful during testing phases.
Michael Chen (Lead Python Developer, Tech Innovations Inc.). In Python, the most common way to comment out multiple lines of code is by using triple quotes. However, it is important to note that this method is technically a string literal and not a comment. For true comments, developers should use the hash symbol () at the beginning of each line.
Lisa Patel (Python Programming Instructor, Code Academy). Understanding how to comment out blocks of code effectively can greatly enhance code readability and collaboration among team members. I recommend adopting a consistent commenting style, such as using a dedicated comment block for large sections of code, to improve overall code management.
Frequently Asked Questions (FAQs)
How can I comment out a block of code in Python?
To comment out a block of code in Python, you can use triple quotes (either `”’` or `”””`). This method allows you to effectively ignore multiple lines of code during execution.
Is there a shortcut to comment out multiple lines in Python?
Yes, many code editors and IDEs offer shortcuts for commenting out multiple lines. For example, in Visual Studio Code, you can select the lines and press `Ctrl + /` (Windows) or `Cmd + /` (Mac) to toggle comments.
Can I use the hash symbol to comment out multiple lines?
While you can use the hash symbol (“) to comment out multiple lines, it requires placing “ at the beginning of each line. This is less efficient than using triple quotes for larger blocks of code.
What happens if I use triple quotes for comments in Python?
Using triple quotes for comments creates a string that is not assigned to any variable, effectively making it a comment. However, it is treated as a string object, which means it may still consume memory.
Are there best practices for commenting code in Python?
Best practices include keeping comments concise, using them to explain why code exists rather than what it does, and avoiding excessive commenting that can clutter the code.
Can I uncomment a block of code easily in Python?
Yes, you can uncomment a block of code by removing the comment markers. If you used triple quotes, simply delete them. If you used “, remove the “ from the beginning of each line.
In Python, commenting out a block of code can be achieved primarily through the use of the hash symbol (), which allows for single-line comments. However, for multi-line comments or to comment out larger sections of code, developers often utilize triple quotes (”’ or “””). This method is not technically a comment but is commonly used for creating docstrings or temporarily disabling code without deleting it.
It is essential to understand that while using the hash symbol is straightforward for single lines, triple quotes can be more versatile for larger blocks. However, one must be cautious, as using triple quotes inappropriately may lead to unintended behavior if the quotes are interpreted as strings rather than comments. Thus, ensuring clarity in code is vital for maintainability and readability.
Key takeaways include the importance of commenting in code for documentation and collaboration purposes. Clear comments help other developers (and future you) understand the intent behind the code, which can significantly enhance the overall quality and maintainability of a project. Therefore, mastering the techniques for commenting out blocks of code is a fundamental skill for any Python programmer.
Author Profile
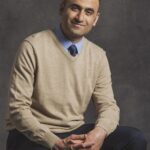
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?