How Can You Effectively Comment Out Blocks of Code in Python?
In the world of programming, clarity and organization are paramount. As developers, we often find ourselves needing to temporarily disable sections of code or annotate our work for future reference. This is where the art of commenting comes into play, particularly in Python, a language celebrated for its simplicity and readability. Whether you’re a seasoned coder or just embarking on your programming journey, understanding how to effectively comment out blocks of code is an essential skill that can enhance your workflow and improve collaboration with others.
When it comes to commenting in Python, the approach is straightforward yet powerful. Unlike some programming languages that require complex syntax, Python provides a clean and intuitive way to manage comments. This not only helps in debugging and testing but also allows developers to leave notes and explanations that can be invaluable for both themselves and their peers. In this article, we will explore the various methods of commenting out code, highlighting best practices and common pitfalls to avoid.
As you dive deeper into the nuances of commenting in Python, you’ll discover the flexibility it offers in managing your codebase. From single-line comments to multi-line annotations, each method serves a unique purpose that can streamline your coding process. By mastering these techniques, you’ll not only write cleaner code but also foster better communication within your development team. Join us
Commenting Out Code in Python
In Python, commenting out blocks of code can be achieved through a couple of methods. While single-line comments are straightforward using the “ symbol, commenting out multiple lines requires a different approach since Python does not have a built-in multi-line comment feature like some other programming languages. Below are the common techniques used to comment out blocks of code effectively.
Using Triple Quotes
One common technique to comment out multiple lines in Python is using triple quotes (`”’` or `”””`). Although primarily intended for multi-line strings, they can effectively serve as comments when not assigned to a variable. Here’s how it works:
“`python
”’
This is a comment.
It spans multiple lines.
”’
“`
This method is useful for temporarily disabling code during development or for leaving detailed explanations in the code. However, be cautious: if the triple-quoted string is not assigned, it may still be interpreted by the interpreter, albeit as a no-op.
Using “ for Each Line
The most straightforward method to comment out multiple lines is by placing a “ at the beginning of each line. This technique is simple but can become tedious with larger blocks of code. Here is an example:
“`python
This is a comment.
Each line needs a hash mark.
This method is explicit and clear.
“`
While this method is clear and easily understood, it can be cumbersome for extensive code sections.
Best Practices for Code Commenting
When commenting out blocks of code, it is essential to adhere to best practices to maintain code readability and functionality. Here are some recommendations:
- Use comments to explain why certain code exists, not just what it does.
- Avoid cluttering your code with excessive comments; aim for clarity in your code itself.
- Keep commented-out code to a minimum. If code is no longer needed, consider removing it entirely instead of commenting it out.
- Utilize version control systems (like Git) to maintain historical versions of your code, rather than leaving large blocks commented out.
Commenting Method | Advantages | Disadvantages |
---|---|---|
Triple Quotes | Easy to comment multiple lines at once | May be interpreted as a string if not used carefully |
Using “ for Each Line | Clear and explicit; easy to read | Time-consuming for large blocks |
By understanding and utilizing these methods, Python developers can efficiently manage their code, enhancing both readability and maintainability while minimizing potential errors during development.
Commenting Out Code in Python
In Python, commenting out blocks of code can be accomplished using several methods, allowing developers to temporarily disable code without deleting it. This practice is essential for debugging and improving code readability.
Using the Hash Symbol
The most straightforward method to comment out a single line of code is by using the hash symbol (“). Any text following this symbol on the same line will be ignored by the Python interpreter. For example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a greeting
“`
For multiple lines, you would need to place a hash symbol at the beginning of each line:
“`python
This is the first line of a comment
This is the second line of a comment
“`
Using Triple Quotes
While not a traditional comment, triple quotes (`”’` or `”””`) can be used to create multi-line strings that are not assigned to any variable, effectively acting as comments. This method is often used for larger blocks of text:
“`python
”’
This is a block of text
that acts as a comment.
It can span multiple lines.
”’
print(“This will run.”)
“`
It is important to note that using triple quotes this way is more commonly associated with docstrings, which serve as documentation for functions, classes, and modules.
Commenting Out Code in Integrated Development Environments (IDEs)
Many IDEs and text editors provide shortcuts for commenting out blocks of code. These features can enhance productivity by allowing developers to comment or uncomment multiple lines quickly. Below are some common shortcuts:
IDE/Text Editor | Comment Shortcut | Uncomment Shortcut |
---|---|---|
PyCharm | `Ctrl + /` | `Ctrl + /` |
Visual Studio Code | `Ctrl + /` | `Ctrl + /` |
Jupyter Notebook | `Ctrl + /` (for selected lines) | `Ctrl + /` (for selected lines) |
Atom | `Ctrl + /` | `Ctrl + /` |
Sublime Text | `Ctrl + /` | `Ctrl + /` |
Using these shortcuts can significantly streamline the coding process, especially when working on larger projects.
Best Practices for Commenting
When commenting out code, adhere to best practices to maintain code clarity:
- Be Consistent: Use the same commenting style throughout your project.
- Keep Comments Relevant: Ensure that comments accurately describe the code’s purpose or the reason for being commented out.
- Avoid Over-commenting: Excessive comments can clutter code. Aim for clarity and conciseness.
- Remove Unused Comments: Once code is finalized, consider removing commented-out code that is no longer needed to enhance readability.
By following these guidelines, developers can effectively manage code comments, improving both the development process and the final product’s maintainability.
Expert Insights on Commenting Out Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out blocks of code in Python is essential for maintaining code readability and organization. The most common method is to use the hash symbol () for single-line comments. However, for multi-line comments, developers often use triple quotes (”’ or “””) to effectively comment out larger sections of code, which is particularly useful during debugging.”
Michael Chen (Lead Python Developer, CodeMaster Solutions). “In Python, while there is no built-in syntax specifically for block comments, utilizing triple quotes is a widely accepted practice. This approach allows developers to temporarily disable sections of code while preserving the original structure, making it easier to test various implementations without losing context.”
Lisa Patel (Technical Instructor, Python Academy). “Understanding how to comment out blocks of code is fundamental for any Python programmer. Using the hash symbol for single lines is straightforward, but for larger blocks, I recommend triple quotes. This not only comments out the code but also serves as a documentation tool, allowing for clearer explanations of complex logic.”
Frequently Asked Questions (FAQs)
How can I comment out a single line of code in Python?
You can comment out a single line of code in Python by placing a hash symbol (“) at the beginning of the line. This tells the interpreter to ignore that line during execution.
Is there a way to comment out multiple lines of code in Python?
Python does not have a specific syntax for multi-line comments like some other languages. However, you can use triple quotes (`”’` or `”””`) to create a string that is not assigned to a variable, effectively commenting out multiple lines.
Can I use the “ symbol for inline comments in Python?
Yes, you can use the “ symbol for inline comments. Place the “ after the code on the same line, and everything following it will be treated as a comment.
What happens if I forget to close a multi-line comment using triple quotes?
If you forget to close a multi-line comment using triple quotes, Python will raise a syntax error, as it will interpret the following code as part of the comment until it encounters a closing triple quote.
Are comments in Python ignored during execution?
Yes, comments in Python are completely ignored during execution. They are intended solely for documentation purposes to make the code easier to understand for developers.
Is there a best practice for commenting code in Python?
Best practices for commenting in Python include writing clear and concise comments, avoiding redundant comments, and using comments to explain the “why” behind complex logic rather than the “what,” which should be evident from the code itself.
In Python, commenting out blocks of code is an essential practice for improving code readability and maintainability. While Python does not have a built-in multi-line comment feature like some other programming languages, developers can utilize several techniques to achieve the same effect. The most common method is to use the hash symbol () at the beginning of each line of code that needs to be commented out. This approach allows for clear identification of comments, as Python ignores any text following the hash symbol.
Another effective technique for commenting out larger blocks of code is to use triple quotes (”’ or “””). While primarily intended for multi-line strings, they can also serve as a way to comment out sections of code, as Python will treat the enclosed text as a string literal and ignore it during execution. However, it is important to note that this method is not a true comment and can lead to unintended consequences if the string is inadvertently used elsewhere in the code.
In summary, understanding how to comment out blocks of code in Python is crucial for maintaining clean and understandable code. By using the hash symbol for single lines or triple quotes for larger sections, developers can effectively manage their codebase. Adopting these practices not only aids in collaboration but also enhances the overall
Author Profile
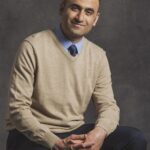
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?