How Can You Effectively Comment Out Code in Python?
Introduction
In the world of programming, clarity and organization are paramount, and Python is no exception. Whether you’re a seasoned developer or a newcomer to the coding scene, understanding how to effectively comment out code is a crucial skill that can enhance your coding experience. Comments serve as a powerful tool for documentation, allowing you to annotate your code, explain complex logic, and make your scripts more readable for yourself and others. In this article, we will delve into the various methods of commenting in Python, ensuring you have the knowledge to keep your code clean and comprehensible.
When you write Python code, you often find yourself needing to leave notes or temporarily disable certain lines without deleting them. This is where comments come into play. They allow you to provide context for your code, making it easier to revisit and understand later. Moreover, comments can be invaluable during collaborative projects, as they help team members grasp the intent behind specific code segments. Understanding the different types of comments available in Python will empower you to communicate your ideas more effectively.
In addition to enhancing readability, comments can also aid in debugging and testing. By commenting out sections of code, you can isolate issues without permanently altering your codebase. This flexibility not only streamlines your workflow but also fosters a more efficient coding environment.
Commenting in Python
In Python, commenting is essential for improving code readability and maintainability. It allows developers to annotate their code, providing explanations for complex logic or outlining the purpose of specific sections. Python supports two types of comments: single-line comments and multi-line comments.
Single-line Comments
Single-line comments in Python begin with the hash symbol (`#`). Anything following this symbol on the same line is considered a comment and will be ignored by the Python interpreter. This type of comment is typically used for brief explanations or notes.
Example:
python
# This is a single-line comment
print(“Hello, World!”) # This prints a greeting
Multi-line Comments
Python does not have a specific syntax for multi-line comments like some other programming languages. However, a common practice is to use triple quotes (`”’` or `”””`) to create a multi-line comment. The content enclosed in triple quotes will also be ignored by the interpreter, and it can be useful for longer explanations or documentation.
Example:
python
“””
This is a multi-line comment.
It can span multiple lines and is often used
to describe the purpose of a function or module.
“””
def greet():
print(“Hello, World!”)
Best Practices for Commenting
To ensure effective commenting, consider the following best practices:
- Be Concise: Keep comments brief yet informative. Avoid unnecessary verbosity that may clutter the code.
- Stay Relevant: Ensure comments are relevant to the code they describe. Outdated comments can lead to confusion.
- Use Consistent Formatting: Maintain a uniform style for comments throughout the codebase for better readability.
- Avoid Obvious Comments: Do not state the obvious. Comments should add value by explaining why something is done, not what is done if it’s already clear from the code.
Commenting Practices in Documentation
In addition to inline comments, documenting functions, classes, and modules is crucial for maintaining code quality. Python uses docstrings, which are a type of multi-line comment, to describe the purpose and usage of a component.
Example:
python
def add(a, b):
“””
Add two numbers and return the result.
Parameters:
a (int or float): The first number.
b (int or float): The second number.
Returns:
int or float: The sum of the two numbers.
“””
return a + b
Summary of Commenting Methods
Comment Type | Syntax | Usage |
---|---|---|
Single-line Comment | # comment | For brief explanations or notes. |
Multi-line Comment | ”’ comment ”’ or “”” comment “”” | For longer explanations or documentation. |
Docstring | def function_name(): “”” comment “”” | To describe the purpose and usage of functions, classes, or modules. |
By following these guidelines, developers can enhance the clarity and effectiveness of their Python code through proper commenting practices.
Commenting in Python
In Python, comments are essential for making code more understandable by providing explanations or notes for the programmer. They are ignored during the execution of the program, which allows developers to annotate their code without affecting functionality.
Single-Line Comments
Single-line comments in Python begin with the hash symbol (`#`). Everything following the `#` on that line will be treated as a comment. For example:
python
# This is a single-line comment
print(“Hello, World!”) # This prints a greeting
- Usage: Use single-line comments to explain a specific line of code or to leave notes for yourself and other developers.
- Best Practices:
- Keep comments concise and relevant.
- Avoid stating the obvious; focus on clarifying the intent.
Multi-Line Comments
While Python does not have a specific syntax for multi-line comments, there are effective ways to achieve this. The most common method is to use triple quotes (`”’` or `”””`).
python
“””
This is a multi-line comment.
It can span several lines.
“””
print(“Hello, World!”)
Alternatively, you can use multiple single-line comments:
python
# This is a comment
# that spans multiple
# lines
print(“Hello, World!”)
- Usage: Multi-line comments are useful for providing detailed explanations or documentation for complex sections of code.
- Best Practices:
- Use triple quotes for longer explanations to improve readability.
- Ensure that multi-line comments are appropriately indented to match the surrounding code.
Docstrings
Docstrings, short for documentation strings, are a specific type of multi-line comment used to describe modules, classes, methods, or functions. They are defined using triple quotes and can be accessed via the `__doc__` attribute.
python
def greet():
“””This function prints a greeting.”””
print(“Hello, World!”)
- Usage: Use docstrings to document your functions and classes, providing necessary information on parameters, return values, and usage.
- Best Practices:
- Start the docstring with a brief description of the function’s purpose.
- Include parameter types and return types for clarity.
Commenting Best Practices
Effective commenting is vital for maintaining code quality. Consider the following best practices:
Best Practice | Description |
---|---|
Keep Comments Up-to-Date | Ensure comments reflect the current state of the code. |
Avoid Redundant Comments | Do not comment on code that is self-explanatory. |
Use Consistent Style | Maintain a uniform commenting style throughout your code. |
Document the Why, Not Just the How | Explain the reasoning behind complex logic instead of just what the code does. |
By adhering to these principles, code becomes more maintainable, understandable, and collaborative, facilitating smoother development processes.
Expert Insights on Commenting in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). Commenting in Python is essential for maintaining code readability and facilitating collaboration among developers. Using the hash symbol (#) allows for single-line comments, while triple quotes can be utilized for multi-line comments. This practice not only clarifies the code’s intent but also aids in debugging and future code modifications.
Michael Chen (Lead Python Developer, Tech Solutions Group). In Python, comments are an integral part of writing clean and maintainable code. They serve as documentation for others who may read the code later. I recommend using comments to explain the ‘why’ behind complex logic rather than the ‘what,’ as the code itself should convey the latter. This approach enhances understanding and reduces the cognitive load for future developers.
Sarah Patel (Python Programming Instructor, Code Academy). Mastering the art of commenting in Python is crucial for both novice and experienced programmers. Comments should be concise yet informative. I encourage my students to adopt a habit of commenting not just for their own benefit but also for the benefit of the entire development team. Properly commented code can significantly reduce onboarding time for new team members.
Frequently Asked Questions (FAQs)
How do I comment out a single line in Python?
To comment out a single line in Python, use the hash symbol (`#`) at the beginning of the line. Everything following the `#` on that line will be treated as a comment and ignored during execution.
Can I comment out multiple lines in Python?
Yes, to comment out multiple lines in Python, you can use the hash symbol (`#`) at the beginning of each line. Alternatively, you can use triple quotes (`”’` or `”””`) to create a multi-line string, which effectively serves as a comment if not assigned to a variable.
What is the purpose of comments in Python?
Comments in Python serve to explain and clarify code for human readers. They help improve code readability and maintainability by providing context and rationale for code decisions without affecting program execution.
Are comments ignored by the Python interpreter?
Yes, comments are completely ignored by the Python interpreter. They do not affect the execution of the program and are solely for the benefit of developers who read the code.
Can I use comments in Python functions and classes?
Absolutely, comments can be used within functions and classes in Python. They are commonly used to describe the purpose of the function or class, as well as to document parameters and return values.
Is there a difference between comments and docstrings in Python?
Yes, comments are created using the hash symbol (`#`) and are meant for brief explanations, while docstrings are multi-line strings placed at the beginning of functions, classes, or modules, and are used to describe their purpose and usage in detail. Docstrings can be accessed through the `__doc__` attribute.
In Python, commenting out code is an essential practice that enhances code readability and maintainability. Comments are used to explain the purpose of code segments, making it easier for others (or oneself) to understand the logic behind the code at a later time. There are two primary ways to comment in Python: single-line comments and multi-line comments. Single-line comments begin with the hash symbol (#), while multi-line comments can be created using triple quotes (”’ or “””).
Single-line comments are straightforward and are often used for brief explanations or notes. They are placed on their own line or at the end of a line of code. Multi-line comments, on the other hand, are useful for providing detailed descriptions or for temporarily disabling blocks of code during testing or debugging. Both methods are crucial for effective documentation and can significantly improve collaboration in team environments.
In summary, mastering the use of comments in Python is vital for any programmer. It not only aids in the understanding of the code but also fosters better practices in coding standards. By utilizing comments effectively, developers can create more robust, maintainable, and collaborative codebases.
Author Profile
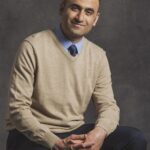
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?