How Can You Effectively Compare Dates in Python?
In the world of programming, managing and manipulating dates is a fundamental skill that can significantly enhance the functionality of your applications. Whether you’re building a calendar application, analyzing time-series data, or simply tracking events, understanding how to compare dates in Python is essential. Python’s rich ecosystem provides various tools and libraries that make date manipulation not only efficient but also intuitive. If you’ve ever found yourself puzzled by the intricacies of date comparisons, fear not! This article will guide you through the essential concepts and techniques to master this crucial aspect of programming.
At its core, comparing dates in Python involves understanding how to represent dates and times accurately. Python’s built-in `datetime` module offers a robust framework for working with dates and times, allowing you to create, manipulate, and compare date objects seamlessly. Whether you need to determine if one date is earlier than another, check for equality, or calculate the difference between two dates, Python provides straightforward methods to achieve these tasks.
Moreover, as you delve deeper into the topic, you’ll discover that Python’s rich ecosystem extends beyond the built-in capabilities. Libraries like `pandas` and `dateutil` offer advanced functionalities that can simplify complex date comparisons, especially when working with large datasets or specific time zones. By the end of this
Using the datetime Module
To compare dates in Python, the most common approach is to utilize the built-in `datetime` module. This module provides the necessary classes for manipulating dates and times. The primary classes used for comparison are `date`, `time`, and `datetime`.
Here’s how you can perform date comparisons:
- Creating Date Objects: You can create date objects using the `datetime.date(year, month, day)` method.
- Comparison Operators: Once you have date objects, you can use standard comparison operators such as `<`, `>`, `==`, `!=`, `<=`, and `>=`.
Example:
“`python
from datetime import date
date1 = date(2023, 10, 1)
date2 = date(2023, 10, 15)
if date1 < date2:
print("Date1 is earlier than Date2")
elif date1 > date2:
print(“Date1 is later than Date2”)
else:
print(“Date1 is the same as Date2”)
“`
Comparing DateTime Objects
When working with specific times in addition to dates, the `datetime` class is more appropriate. The `datetime` class allows you to create objects that include both date and time.
Example:
“`python
from datetime import datetime
datetime1 = datetime(2023, 10, 1, 12, 0)
datetime2 = datetime(2023, 10, 1, 15, 30)
if datetime1 < datetime2: print("Datetime1 is earlier than Datetime2") ```
Handling Timezones
When comparing dates and times, it is crucial to consider time zones, especially if the dates originate from different time zones. The `pytz` library can be used alongside `datetime` to handle time zone-aware date comparisons.
Example:
“`python
from datetime import datetime
import pytz
timezone1 = pytz.timezone(‘America/New_York’)
timezone2 = pytz.timezone(‘Europe/London’)
datetime1 = timezone1.localize(datetime(2023, 10, 1, 12, 0))
datetime2 = timezone2.localize(datetime(2023, 10, 1, 17, 0))
if datetime1 < datetime2: print("Datetime1 is earlier than Datetime2") ```
Using Timedelta for Date Arithmetic
The `timedelta` class allows for date arithmetic, which can be beneficial when comparing dates relative to one another. This can be used to check if a date is a certain number of days away from another date.
Example:
“`python
from datetime import date, timedelta
date1 = date(2023, 10, 1)
date2 = date1 + timedelta(days=10)
if date2 > date1:
print(“Date2 is 10 days after Date1”)
“`
Comparison Summary Table
Comparison Method | Example Code | Notes |
---|---|---|
Using date | date1 < date2 |
Compares only the date |
Using datetime | datetime1 < datetime2 |
Compares date and time |
Timezone-aware comparison | datetime1.astimezone() < datetime2 |
Adjusts for time zones |
Using timedelta | date1 + timedelta(days=10) |
Adds/subtracts days from a date |
By leveraging the capabilities of Python's `datetime` module, you can effectively compare dates with precision and clarity in your applications.
Comparing Dates Using Built-in Python Libraries
Python provides several modules for date manipulation, with `datetime` being the most commonly used. The `datetime` module allows you to work with dates and times effectively, making date comparisons straightforward.
To compare dates, you first need to create `datetime` objects. The following example demonstrates how to do this:
```python
from datetime import datetime
Define two dates
date1 = datetime(2023, 10, 1)
date2 = datetime(2023, 10, 15)
Comparison
if date1 < date2:
print("date1 is earlier than date2")
elif date1 > date2:
print("date1 is later than date2")
else:
print("date1 is the same as date2")
```
In this code snippet:
- `datetime(2023, 10, 1)` creates a `datetime` object for October 1, 2023.
- The comparison operators `<`, `>`, and `==` are used to compare the two dates directly.
Using the `date` Class for Date Comparisons
For date-only comparisons without time, the `date` class from the `datetime` module can be utilized. This approach simplifies handling dates when the time component is irrelevant.
Example:
```python
from datetime import date
Create date objects
date1 = date(2023, 10, 1)
date2 = date(2023, 10, 15)
Comparison
if date1 < date2:
print("date1 is earlier than date2")
```
The `date` class supports similar comparison operators as `datetime`, making it easy to evaluate which date comes first.
Handling Date Strings
When working with date strings, you can convert them into `datetime` or `date` objects using the `strptime` method. This is essential for comparisons when dates are provided in string format.
Example:
```python
from datetime import datetime
Define date strings
date_string1 = "2023-10-01"
date_string2 = "2023-10-15"
Convert to datetime objects
date1 = datetime.strptime(date_string1, "%Y-%m-%d")
date2 = datetime.strptime(date_string2, "%Y-%m-%d")
Comparison
if date1 < date2:
print("date1 is earlier than date2")
```
In this example:
- `strptime(date_string1, "%Y-%m-%d")` parses the date string into a `datetime` object.
Comparing Dates with `pandas`
For data analysis tasks, the `pandas` library provides a powerful way to handle date comparisons, especially when working with DataFrames.
Example:
```python
import pandas as pd
Create a DataFrame
data = {'dates': ['2023-10-01', '2023-10-15']}
df = pd.DataFrame(data)
Convert the 'dates' column to datetime
df['dates'] = pd.to_datetime(df['dates'])
Compare dates
df['is_earlier'] = df['dates'] < pd.Timestamp('2023-10-10')
print(df)
```
This will produce a DataFrame indicating which dates are earlier than October 10, 2023. The `pd.to_datetime` function is crucial for converting strings to datetime objects, allowing for efficient comparisons.
Date | Is Earlier Than 2023-10-10 |
---|---|
2023-10-01 | True |
2023-10-15 |
Best Practices for Date Comparisons
- Always ensure date formats are consistent when comparing.
- Use `datetime` or `date` objects for comparisons instead of strings.
- Utilize libraries like `pandas` for large datasets to streamline operations.
By adhering to these practices, you can efficiently manage and compare dates within your Python applications.
Expert Insights on Comparing Dates in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). "When comparing dates in Python, the most efficient approach is to utilize the built-in `datetime` module. This module allows for straightforward date manipulation and comparison, enabling developers to easily determine the chronological order of dates."
James Liu (Software Engineer, CodeCraft Solutions). "For accurate date comparisons, it is essential to ensure that the dates are in the same format. Using `strptime` to parse date strings into `datetime` objects can help avoid common pitfalls associated with string comparisons."
Maria Gonzalez (Python Developer, Open Source Community). "I recommend using the `pandas` library for more complex date comparisons, especially when dealing with large datasets. Its `Timestamp` objects and vectorized operations make it easier to handle and compare multiple dates efficiently."
Frequently Asked Questions (FAQs)
How can I compare two dates in Python?
You can compare two dates in Python using the comparison operators (`<`, `>`, `==`, `!=`, `<=`, `>=`). The `datetime` module allows you to create date objects that can be compared directly.
What module is commonly used for date manipulation in Python?
The `datetime` module is the standard library module used for manipulating dates and times in Python. It provides classes for working with dates, times, and intervals.
How do I convert a string to a date in Python?
You can convert a string to a date using the `strptime` method from the `datetime` class. For example, `datetime.strptime(date_string, format)` allows you to specify the format of the input string.
Can I compare dates with different formats in Python?
No, dates must be in the same format for comparison. You should convert all dates to a common format using `strptime` or similar methods before comparing them.
What happens if I compare a date with a string in Python?
Comparing a date with a string will raise a `TypeError` in Python. Ensure both values are of the same type (either both dates or both strings) before performing a comparison.
Is it possible to calculate the difference between two dates in Python?
Yes, you can calculate the difference between two date objects using subtraction, which returns a `timedelta` object. This object can be used to retrieve the difference in days, seconds, and other time units.
In Python, comparing dates is a straightforward process that can be accomplished using the built-in `datetime` module. This module provides a robust framework for handling dates and times, allowing users to create date objects and perform various comparisons. The primary methods for comparing dates involve using relational operators such as `<`, `>`, `==`, and `!=`, which can be directly applied to `datetime` objects. This functionality enables users to determine the chronological order of dates easily.
When working with dates, it is essential to ensure that the dates being compared are in the same format. The `datetime` module allows for the parsing of date strings into `datetime` objects, which can then be compared. Additionally, the module provides the `date` and `time` classes for more specific date and time manipulations. Understanding how to convert between different formats and types is crucial for accurate comparisons.
Another important aspect of date comparison in Python is the handling of time zones. The `pytz` library can be integrated with the `datetime` module to manage time zone differences effectively. This is particularly useful when comparing dates from different geographical locations. Properly accounting for time zones ensures that comparisons are valid and reflect the intended chronological order.
Author Profile
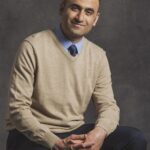
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?