How Can You Effectively Compare Lists in Python?
In the world of programming, data manipulation is a fundamental skill, and Python, with its versatile data structures, offers a plethora of ways to handle and compare lists. Whether you’re analyzing data sets, checking for duplicates, or merging information from different sources, understanding how to compare lists is crucial for efficient coding and data management. This article will delve into the various techniques and methods available in Python to compare lists, equipping you with the knowledge to tackle a range of programming challenges.
When it comes to comparing lists in Python, there are multiple approaches you can take, each suited for different scenarios. From simple equality checks to more complex operations like finding the intersection or differences between lists, Python provides built-in functionalities and libraries that streamline these processes. Understanding the nuances of these methods not only helps in achieving accurate results but also enhances the performance of your code.
As we explore the various techniques for list comparison, you’ll discover how to leverage Python’s powerful features, such as list comprehensions and set operations, to make your comparisons more efficient. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your skills, this guide will provide you with valuable insights and practical examples to elevate your Python programming capabilities.
Using Set Operations
One of the most efficient ways to compare lists in Python is by utilizing set operations. Sets are unordered collections of unique elements, making them ideal for comparisons. You can easily determine which elements are common, unique, or missing between two lists using set operations like intersection, union, and difference.
- Intersection: Elements that are present in both lists.
- Union: All unique elements from both lists.
- Difference: Elements that are present in one list but not in the other.
Example:
“`python
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
set1 = set(list1)
set2 = set(list2)
intersection = set1 & set2
union = set1 | set2
difference = set1 – set2
“`
In this example, `intersection` would yield `{3, 4}`, `union` would yield `{1, 2, 3, 4, 5, 6}`, and `difference` would yield `{1, 2}`.
Using List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. They can also be effectively used to compare lists by filtering elements based on conditions.
For instance, to find elements that are present in the first list but not in the second, you can use:
“`python
only_in_list1 = [item for item in list1 if item not in list2]
“`
This will give you a new list containing elements that are unique to `list1`.
Using the `collections` Module
The `collections` module in Python provides a `Counter` class that can be used to compare lists by counting the occurrences of each element. This is particularly useful when you need to account for duplicate values.
Example:
“`python
from collections import Counter
list1 = [‘a’, ‘b’, ‘c’, ‘a’]
list2 = [‘a’, ‘b’, ‘d’, ‘e’]
counter1 = Counter(list1)
counter2 = Counter(list2)
common_elements = counter1 & counter2 Intersection
unique_to_list1 = counter1 – counter2 Elements in list1 not in list2
unique_to_list2 = counter2 – counter1 Elements in list2 not in list1
“`
The resulting `common_elements`, `unique_to_list1`, and `unique_to_list2` will help you understand the distribution of elements across both lists.
Comparing Lists for Order and Content
When comparing lists, it is essential to consider both the content and the order of elements. You can achieve this by using the equality operator `==`, which checks if the lists are identical in terms of both order and content.
Example:
“`python
list1 = [1, 2, 3]
list2 = [1, 2, 3]
list3 = [3, 2, 1]
are_equal = list1 == list2 True
are_equal_ordered = list1 == list3
“`
If you want to compare the lists regardless of the order, you can convert them to sets:
“`python
are_equal_unordered = set(list1) == set(list3) True
“`
Comparison Summary Table
Method | Use Case | Example |
---|---|---|
Set Operations | Find common/unique elements efficiently | set(list1) & set(list2) |
List Comprehensions | Filter based on conditions | [item for item in list1 if item not in list2] |
Counter | Count occurrences for duplicates | Counter(list1) & Counter(list2) |
Equality Operator | Check for identical lists | list1 == list2 |
Comparing Lists in Python
When comparing lists in Python, several methods are available depending on the specific requirements of the comparison. Below are common techniques to compare lists effectively.
Using the Equality Operator
The simplest way to compare two lists for equality is by using the `==` operator. This checks if both lists have the same elements in the same order.
“`python
list1 = [1, 2, 3]
list2 = [1, 2, 3]
list3 = [3, 2, 1]
are_equal = list1 == list2 True
are_not_equal = list1 == list3
“`
Checking for Subset or Superset
To determine if one list is a subset or superset of another, you can convert the lists to sets and use the `issubset()` and `issuperset()` methods.
“`python
list_a = [1, 2, 3]
list_b = [1, 2, 3, 4, 5]
is_subset = set(list_a).issubset(set(list_b)) True
is_superset = set(list_b).issuperset(set(list_a)) True
“`
Finding Common Elements
To find the intersection of two lists, you can use the `set` data structure, which provides an efficient way to retrieve common elements.
“`python
list_x = [1, 2, 3, 4]
list_y = [3, 4, 5, 6]
common_elements = list(set(list_x) & set(list_y)) [3, 4]
“`
Identifying Differences
To identify elements present in one list but not in the other, you can also utilize sets. The difference can be calculated as follows:
“`python
list_m = [1, 2, 3]
list_n = [2, 3, 4]
only_in_m = list(set(list_m) – set(list_n)) [1]
only_in_n = list(set(list_n) – set(list_m)) [4]
“`
Comparing Lists with Order and Duplicates
If you need to consider order and duplicates, the `collections.Counter` class is useful for counting occurrences of elements.
“`python
from collections import Counter
list_p = [1, 2, 2, 3]
list_q = [1, 2, 3, 3]
are_counter_equal = Counter(list_p) == Counter(list_q)
“`
Using List Comprehensions for Custom Comparisons
You can also employ list comprehensions for more complex comparisons, such as finding elements that differ between two lists.
“`python
list_r = [1, 2, 3]
list_s = [2, 3, 4]
differences = [item for item in list_r if item not in list_s] [1]
“`
Comparing Nested Lists
For nested lists, you may need to recursively compare elements. This can be achieved using a custom function.
“`python
def compare_nested_lists(list1, list2):
if len(list1) != len(list2):
return
return all(compare_nested_lists(i, j) if isinstance(i, list) and isinstance(j, list) else i == j for i, j in zip(list1, list2))
nested_list1 = [[1, 2], [3, 4]]
nested_list2 = [[1, 2], [3, 4]]
are_nested_equal = compare_nested_lists(nested_list1, nested_list2) True
“`
Performance Considerations
When comparing large lists, consider the following:
- Using sets for membership tests is generally faster than lists due to O(1) average time complexity for lookups.
- List comprehensions and generator expressions can improve performance when filtering elements.
- Avoid redundant conversions between lists and sets when possible to minimize overhead.
By employing these methods and strategies, you can effectively compare lists in Python based on your specific requirements.
Expert Insights on Comparing Lists in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When comparing lists in Python, utilizing built-in functions such as `set()` can be highly effective for identifying unique elements across lists. This approach not only simplifies the comparison process but also enhances performance, especially with larger datasets.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For more complex comparisons, employing list comprehensions can provide a concise and readable way to filter and compare lists. This method allows developers to write more Pythonic code while maintaining clarity in their logic.”
Sarah Patel (Python Developer, Data Insights Group). “In scenarios where order matters, using libraries like `numpy` or `pandas` can facilitate efficient list comparisons. These libraries offer robust functionalities that handle multidimensional data and provide powerful tools for analysis.”
Frequently Asked Questions (FAQs)
How can I compare two lists for equality in Python?
You can compare two lists for equality using the equality operator `==`. If both lists contain the same elements in the same order, the result will be `True`; otherwise, it will be “.
What methods can I use to find the differences between two lists in Python?
You can use the `set` data structure to find differences. The expressions `set(list1) – set(list2)` and `set(list2) – set(list1)` will yield the elements that are unique to each list.
How do I check if one list is a subset of another in Python?
You can use the `issubset()` method of the `set` data structure. For example, `set(list1).issubset(set(list2))` returns `True` if all elements of `list1` are present in `list2`.
What is the best way to find common elements between two lists in Python?
To find common elements, you can use the intersection of sets: `set(list1) & set(list2)`. This will return a set containing elements that appear in both lists.
Can I compare lists of different lengths in Python?
Yes, you can compare lists of different lengths. However, if you use the equality operator `==`, the comparison will return “ unless both lists are identical in elements and order.
How can I ignore the order when comparing two lists in Python?
To compare two lists while ignoring the order, convert them to sets using `set(list1)` and `set(list2)`. Then, check for equality: `set(list1) == set(list2)`. This will return `True` if both lists contain the same elements, regardless of their order.
In Python, comparing lists can be accomplished through various methods, each suited for different scenarios. The most straightforward approach is to use the equality operator (`==`), which checks if two lists are identical in terms of order and content. However, if the order of elements is not a concern, one can utilize sets to compare lists, as sets inherently disregard order and duplicate entries. This method is beneficial when determining if two lists contain the same unique elements.
Another powerful technique involves leveraging list comprehensions and built-in functions such as `all()` or `any()`. These allow for more complex comparisons, such as checking for the presence of common elements or validating specific conditions across lists. Additionally, libraries like NumPy and Pandas provide optimized functions for comparing lists, especially when dealing with large datasets or requiring advanced operations like element-wise comparisons.
In summary, Python offers a variety of methods for comparing lists, each with its own advantages depending on the requirements of the task. Understanding these methods enables developers to choose the most efficient and appropriate approach for their specific needs, whether it be simple equality checks, set comparisons, or utilizing specialized libraries for more complex data manipulations.
Author Profile
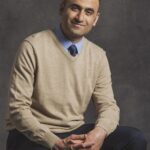
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?