How Can You Effectively Compare Output Files in C?
When developing software in C, the ability to compare output files is a crucial skill that can streamline debugging and enhance code efficiency. Whether you’re working on a complex application or a simple program, ensuring that your output matches expected results is essential for validating your work. As you dive into the world of C programming, understanding how to effectively compare output files can save you time and help you identify discrepancies that may arise during development.
Comparing output files in C involves more than just a side-by-side visual inspection; it requires a systematic approach to identify differences in data, format, and structure. This process can be particularly useful when testing algorithms, verifying data integrity, or even conducting regression tests after changes are made to your code. By employing various techniques and tools, programmers can automate the comparison process, making it more efficient and less prone to human error.
In this article, we will explore the various methods and tools available for comparing output files in C, from simple command-line utilities to more sophisticated programming techniques. Whether you’re a novice programmer looking to enhance your debugging skills or an experienced developer seeking to optimize your workflow, understanding how to effectively compare output files will undoubtedly elevate your programming capabilities and contribute to your overall success in C development.
Understanding File Comparison
When comparing output files in C, it is essential to assess both the content and the structure of the files. This can involve checking for differences in data, formatting, or even metadata, depending on the application requirements. The comparison can be implemented using various techniques, including line-by-line comparison, checksum validation, and using external tools.
Methods for Comparing Files
There are several methods to compare output files in C. Here are some common techniques:
- Line-by-Line Comparison: This method reads both files line by line and compares each line for differences.
- Checksum Comparison: By calculating a checksum (e.g., MD5 or SHA-1) for each file, you can determine whether the files are identical without needing to read through each byte.
- Using External Tools: Tools like `diff` in Unix/Linux can be called from C programs to perform file comparisons.
Implementing Line-by-Line Comparison
To perform a line-by-line comparison, you can use the following approach:
- Open both files for reading.
- Read each line from both files until the end of either file is reached.
- Compare the lines:
- If they differ, record the difference and continue.
- If they are the same, proceed to the next line.
- Close both files after the comparison is complete.
Here is a basic code example demonstrating this method:
“`c
include
include
void compareFiles(const char *file1, const char *file2) {
FILE *f1 = fopen(file1, “r”);
FILE *f2 = fopen(file2, “r”);
char line1[256], line2[256];
int lineNum = 1;
if (!f1 || !f2) {
perror(“Error opening file”);
return;
}
while (fgets(line1, sizeof(line1), f1) && fgets(line2, sizeof(line2), f2)) {
if (strcmp(line1, line2) != 0) {
printf(“Difference at line %d:\nFile1: %sFile2: %s”, lineNum, line1, line2);
}
lineNum++;
}
fclose(f1);
fclose(f2);
}
“`
Checksum Comparison Example
Using checksums can be a more efficient way to compare large files. Here’s a sample function that computes an MD5 checksum using an external library:
“`c
include
include
void computeMD5(const char *filename, unsigned char *digest) {
FILE *file = fopen(filename, “rb”);
MD5_CTX mdContext;
char buffer[1024];
int bytesRead;
if (file == NULL) {
perror(“Error opening file”);
return;
}
MD5_Init(&mdContext);
while ((bytesRead = fread(buffer, 1, sizeof(buffer), file)) != 0) {
MD5_Update(&mdContext, buffer, bytesRead);
}
MD5_Final(digest, &mdContext);
fclose(file);
}
“`
Comparison Summary Table
The following table summarizes the different methods of file comparison:
Method | Advantages | Disadvantages |
---|---|---|
Line-by-Line | Simple to implement; clear output | Slow for large files; memory usage |
Checksum | Fast; low memory usage | positives possible; requires additional libraries |
External Tools | Robust; handles various formats | Dependency on external programs; additional complexity |
By utilizing these methods, you can effectively compare output files in C, ensuring that your applications maintain the expected functionality and data integrity.
File Comparison Techniques in C
When comparing output files in C, there are several methods to achieve this, depending on the specific requirements and contexts. Below are some effective approaches:
Using Standard Library Functions
The C Standard Library provides functions that can be utilized for reading files and comparing their contents. The most common approach involves using `fopen()`, `fgetc()`, and `feof()`.
Steps to Compare Files:
- Open both files using `fopen()`.
- Read the files character by character using `fgetc()`.
- Compare each character until the end of either file is reached.
- Check for differences and handle them accordingly.
Sample Code:
“`c
include
int compareFiles(const char *file1, const char *file2) {
FILE *f1 = fopen(file1, “r”);
FILE *f2 = fopen(file2, “r”);
if (!f1 || !f2) {
perror(“Error opening file”);
return -1;
}
int ch1, ch2;
int position = 0;
while ((ch1 = fgetc(f1)) != EOF && (ch2 = fgetc(f2)) != EOF) {
if (ch1 != ch2) {
printf(“Files differ at position %d: ‘%c’ != ‘%c’\n”, position, ch1, ch2);
fclose(f1);
fclose(f2);
return 1; // Files are different
}
position++;
}
if (ch1 != ch2) {
printf(“Files differ in length.\n”);
fclose(f1);
fclose(f2);
return 1; // Files are different
}
fclose(f1);
fclose(f2);
return 0; // Files are identical
}
“`
Using `diff` Command via System Call
For a more comprehensive comparison, especially with larger files, you may utilize the `diff` command available in Unix-like systems. This can be done by invoking it from within your C program using `system()`.
Code Example:
“`c
include
void compareFilesWithDiff(const char *file1, const char *file2) {
char command[256];
snprintf(command, sizeof(command), “diff %s %s”, file1, file2);
system(command);
}
“`
Using Hashing for Quick Comparison
For large files, computing a hash for each file can provide a quick comparison method. If the hashes are different, the files are different; if the hashes are the same, further investigation may be needed.
Steps:
- Read the file in chunks and compute a hash (e.g., MD5 or SHA).
- Compare the resulting hashes.
Sample Code Using MD5:
“`c
include
void computeMD5(const char *filename, unsigned char *result) {
FILE *file = fopen(filename, “rb”);
MD5_CTX md5Context;
MD5_Init(&md5Context);
unsigned char buffer[1024];
size_t bytesRead;
while ((bytesRead = fread(buffer, 1, sizeof(buffer), file)) != 0) {
MD5_Update(&md5Context, buffer, bytesRead);
}
MD5_Final(result, &md5Context);
fclose(file);
}
int compareFilesByHash(const char *file1, const char *file2) {
unsigned char hash1[MD5_DIGEST_LENGTH], hash2[MD5_DIGEST_LENGTH];
computeMD5(file1, hash1);
computeMD5(file2, hash2);
return memcmp(hash1, hash2, MD5_DIGEST_LENGTH);
}
“`
Visualizing Differences with External Libraries
For advanced comparison, consider using libraries such as `libdiff`. These libraries provide sophisticated algorithms for comparing text files, including line-by-line and word-by-word comparisons.
Integration Steps:
- Install the library.
- Include the necessary headers in your C program.
- Use the library’s API to compare files and visualize differences.
This approach is useful for applications requiring detailed analysis of file differences, especially in text files.
Expert Insights on Comparing Output Files in C Programming
Dr. Emily Carter (Senior Software Engineer, CodeTech Solutions). “When comparing output files in C, utilizing the `diff` command in conjunction with file redirection can be highly effective. This allows developers to quickly identify discrepancies between expected and actual outputs, streamlining the debugging process.”
James Lin (Lead Developer, OpenSource Innovations). “Incorporating checksum algorithms within your C programs can provide a reliable method for comparing output files. By generating and comparing checksums, developers can efficiently verify file integrity and ensure that outputs match expected results.”
Sarah Thompson (Technical Writer, C Programming Digest). “For more complex comparisons, writing a custom comparison function in C can be invaluable. This approach allows for tailored comparison logic that can handle specific data structures or formats, offering greater control over the comparison process.”
Frequently Asked Questions (FAQs)
How can I compare two output files in C?
You can compare two output files in C by reading both files line by line and checking for differences using functions like `fopen`, `fgets`, and `strcmp`. Alternatively, you can use external tools like `diff` in Unix/Linux environments.
What libraries can assist in comparing files in C?
The standard C library provides functions for file handling, but for more advanced comparisons, libraries like `libdiff` or using system calls to external diff tools can be helpful.
Is there a built-in function in C for file comparison?
C does not have a built-in function specifically for file comparison. You must implement the comparison logic using file I/O functions and string comparison functions.
How do I handle binary files when comparing in C?
To compare binary files, open the files in binary mode using `fopen` with the `”rb”` mode. Read the files in chunks and compare the bytes directly to check for differences.
What is the best approach to compare large files in C?
For large files, read and compare the files in smaller chunks or buffers to minimize memory usage. Use a loop to read fixed-size blocks and compare them iteratively.
Can I automate file comparison in C using scripts?
Yes, you can automate file comparison by writing a C program that takes file paths as input and outputs the differences. Additionally, you can integrate this with shell scripts for batch processing.
Comparing output files in C can be approached through various methods, each suited to different requirements and contexts. The most common techniques involve using file handling functions to read the contents of the files, followed by comparisons of the data read. This can be done character by character, line by line, or even by utilizing specialized libraries designed for file comparison. Understanding the structure and format of the output files is crucial, as it can influence the choice of comparison method.
One effective way to perform comparisons is by leveraging the standard library functions such as `fopen`, `fgets`, and `strcmp`. These functions allow developers to open files, read their contents, and compare strings efficiently. Additionally, implementing a checksum or hash function can provide a quick way to determine if two files are identical without needing to compare them in detail. For larger files or more complex data structures, using data structures like arrays or linked lists to store file contents before comparison can enhance performance and organization.
Another significant consideration is handling discrepancies between files. It is essential to define what constitutes a difference, whether it be variations in whitespace, case sensitivity, or formatting. Tools and libraries that provide diff functionalities can automate this process, making it easier to identify and report differences. Ultimately
Author Profile
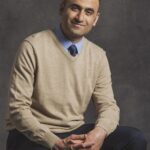
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?