How Can You Effectively Compare Strings in Python?
In the world of programming, strings are one of the most fundamental data types, serving as the backbone for text manipulation and data representation. Whether you’re developing a simple application or a complex software system, the ability to compare strings effectively is crucial. Python, known for its simplicity and readability, offers a variety of methods to compare strings, making it a favorite among both novice and experienced developers. But how do you ensure that your string comparisons are accurate and efficient?
When comparing strings in Python, there are several approaches you can take, each suited for different scenarios. From basic equality checks to more complex comparisons that take into account case sensitivity and locale, Python provides a range of built-in functions and operators to facilitate these tasks. Understanding the nuances of string comparison can help you avoid common pitfalls and optimize your code for better performance.
In this article, we will delve into the various methods available for string comparison in Python, exploring their syntax, functionality, and best practices. Whether you’re looking to compare strings for equality, order, or specific patterns, you’ll find the insights you need to enhance your programming skills and tackle string-related challenges with confidence. So, let’s unravel the intricacies of string comparison in Python and unlock the potential of your coding projects!
String Comparison Operators
In Python, string comparison can be performed using various operators. The most common operators used for comparing strings include:
- Equality (`==`): Checks if two strings are identical.
- Inequality (`!=`): Checks if two strings are not identical.
- Less than (`<`): Determines if one string is lexicographically smaller than another.
– **Greater than (`>`)**: Determines if one string is lexicographically larger than another.
- Less than or equal to (`<=`): Checks if one string is less than or equal to another.
– **Greater than or equal to (`>=`)**: Checks if one string is greater than or equal to another.
For example:
“`python
str1 = “apple”
str2 = “banana”
print(str1 == str2) Output:
print(str1 < str2) Output: True
```
String Comparison Functions
Python also provides built-in functions that can be used to compare strings. These functions can be especially useful when working with case sensitivity or specific conditions.
- `str.lower()`: Converts a string to lowercase, allowing for case-insensitive comparisons.
- `str.upper()`: Converts a string to uppercase, also supporting case-insensitive comparisons.
- `str.casefold()`: A more aggressive version of `lower()` designed for case-insensitive comparisons.
Example of using these functions:
“`python
str1 = “Hello”
str2 = “hello”
print(str1.lower() == str2.lower()) Output: True
“`
Using the `in` Keyword
The `in` keyword is another powerful tool for string comparison in Python. It checks for the presence of a substring within a string.
Example:
“`python
main_str = “Hello, welcome to the world of Python.”
sub_str = “welcome”
if sub_str in main_str:
print(f”‘{sub_str}’ is found in the main string.”)
“`
Comparing Strings with the `startswith()` and `endswith()` Methods
Python strings come equipped with methods that facilitate specific types of comparisons:
- `startswith(prefix)`: Returns `True` if the string starts with the specified prefix.
- `endswith(suffix)`: Returns `True` if the string ends with the specified suffix.
Example:
“`python
filename = “example.txt”
if filename.endswith(“.txt”):
print(“This is a text file.”)
“`
Comparing Strings: A Summary Table
Here is a summary table of different methods and operators for comparing strings in Python:
Method/Operator | Description | Example |
---|---|---|
`==` | Checks if two strings are equal | `str1 == str2` |
`!=` | Checks if two strings are not equal | `str1 != str2` |
`<`, `>`, `<=`, `>=` | Lexicographical comparison | `str1 < str2` |
`in` | Checks for substring presence | `sub_str in main_str` |
`startswith(prefix)` | Checks if string starts with a prefix | `str.startswith(‘H’)` |
`endswith(suffix)` | Checks if string ends with a suffix | `str.endswith(‘.txt’)` |
Comparing Strings in Python
String comparison in Python is a fundamental operation that can be performed using several methods. The most common techniques include direct comparison operators, string methods, and built-in functions.
Using Comparison Operators
Python provides several built-in comparison operators that can be utilized for string comparison:
– **Equality (`==`)**: Checks if two strings are identical.
– **Inequality (`!=`)**: Checks if two strings are different.
– **Greater than (`>`)**: Determines if one string is lexicographically greater than another.
- Less than (`<`): Determines if one string is lexicographically less than another.
– **Greater than or equal to (`>=`)**: Checks if one string is greater than or equal to another.
- Less than or equal to (`<=`): Checks if one string is less than or equal to another.
Example:
“`python
str1 = “apple”
str2 = “banana”
print(str1 == str2) Output:
print(str1 < str2) Output: True
```
Using the `str` Methods
Python’s string methods offer additional functionalities to compare strings based on specific conditions:
- `str.startswith(prefix)`: Checks if a string starts with a specified prefix.
- `str.endswith(suffix)`: Checks if a string ends with a specified suffix.
- `str.find(sub)`: Returns the lowest index of the substring if found; otherwise, returns -1.
- `str.count(sub)`: Returns the number of non-overlapping occurrences of the substring.
Example:
“`python
text = “Hello, World!”
print(text.startswith(“Hello”)) Output: True
print(text.endswith(“World!”)) Output: True
print(text.find(“o”)) Output: 4
print(text.count(“o”)) Output: 2
“`
Case Insensitive Comparison
To compare strings without considering the case, you can convert both strings to the same case using `str.lower()` or `str.upper()`.
Example:
“`python
str1 = “Python”
str2 = “python”
print(str1.lower() == str2.lower()) Output: True
“`
Using the `locale` Module for Localized Comparison
For locale-aware string comparisons, the `locale` module can be used. This is particularly useful in applications that need to respect local language rules.
Example:
“`python
import locale
locale.setlocale(locale.LC_COLLATE, ‘en_US.UTF-8’)
str1 = “resume”
str2 = “résumé”
print(locale.strcoll(str1, str2)) Output may vary based on the locale
“`
Sorting Strings
When sorting a list of strings, you can use the `sorted()` function or the `list.sort()` method. Both methods support key functions to customize comparisons.
Example:
“`python
strings = [“banana”, “apple”, “cherry”]
sorted_strings = sorted(strings) Default: lexicographical order
print(sorted_strings) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Conclusion on String Comparison Techniques
Understanding how to compare strings in Python is crucial for data manipulation and processing. By leveraging the built-in operators, methods, and libraries, developers can implement robust string comparison logic that suits various applications.
Expert Insights on Comparing Strings in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When comparing strings in Python, it is essential to understand the nuances of the equality operator (==) versus the identity operator (is). The former checks for value equality, while the latter checks for reference equality, which can lead to unexpected results if not properly understood.”
James Liu (Data Scientist, Analytics World). “For more complex string comparisons, such as case-insensitive comparisons, utilizing the .lower() or .upper() methods can be invaluable. This ensures that the comparison is not affected by the casing of the strings, which is a common pitfall in data processing.”
Sarah Thompson (Python Developer, CodeCraft Solutions). “In scenarios where performance is critical, especially with large datasets, leveraging the built-in string methods like str.startswith() and str.endswith() can provide efficient ways to compare strings without the overhead of creating new string objects.”
Frequently Asked Questions (FAQs)
How can I compare two strings for equality in Python?
You can compare two strings for equality using the `==` operator. For example, `string1 == string2` will return `True` if both strings are identical.
What is the difference between case-sensitive and case-insensitive string comparison?
Case-sensitive comparison considers uppercase and lowercase letters as different, while case-insensitive comparison treats them as equivalent. Use `string1.lower() == string2.lower()` for case-insensitive comparisons.
How do I check if one string contains another string in Python?
You can use the `in` keyword to check for substring presence. For example, `if substring in string:` evaluates to `True` if `substring` is found within `string`.
Can I compare strings using the `cmp()` function in Python?
The `cmp()` function is not available in Python 3. Instead, you can use comparison operators like `<`, `>`, and `==` to compare strings lexicographically.
How do I compare strings based on their length in Python?
To compare strings by length, use the `len()` function. For example, `len(string1) < len(string2)` checks if `string1` is shorter than `string2`.
Is there a way to sort a list of strings in Python?
Yes, you can sort a list of strings using the `sort()` method or the `sorted()` function. Both will arrange the strings in alphabetical order by default.
In Python, comparing strings is a fundamental operation that can be accomplished using various methods. The most straightforward approach is to use the equality operators, such as `==` for checking if two strings are identical, and `!=` for determining if they are different. Additionally, Python provides comparison operators like `<`, `>`, `<=`, and `>=`, which can be used to compare strings lexicographically based on their Unicode values.
Another important aspect of string comparison in Python is case sensitivity. By default, string comparisons are case-sensitive, meaning that ‘apple’ and ‘Apple’ would not be considered equal. To perform case-insensitive comparisons, one can convert both strings to the same case using methods like `.lower()` or `.upper()` before performing the comparison. This ensures that the comparison is based solely on the content of the strings, disregarding any differences in case.
For more complex string comparison scenarios, such as checking for substring presence or similarity, Python offers built-in functions and libraries. The `in` keyword can be used to check if one string is a substring of another. Additionally, libraries like `difflib` can be employed to compare strings and generate similarity ratios, which are particularly useful in applications like
Author Profile
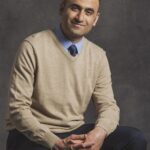
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?