How Can You Concatenate a String in JavaScript?
Introduction
In the dynamic world of web development, mastering the art of string manipulation is essential for creating robust and interactive applications. Among the various operations you can perform on strings, concatenation stands out as a fundamental technique that allows developers to seamlessly combine multiple strings into a single, cohesive unit. Whether you’re crafting user-friendly messages, building dynamic HTML content, or processing data inputs, knowing how to concatenate strings in JavaScript can significantly enhance your coding efficiency and creativity.
In JavaScript, concatenation is a straightforward yet powerful concept that enables you to merge strings using a variety of methods. From the classic use of the plus operator to more modern approaches like template literals, each method offers unique advantages that cater to different programming scenarios. Understanding these techniques not only helps in writing cleaner code but also boosts your ability to manipulate and display data effectively.
As you delve deeper into the nuances of string concatenation, you’ll discover how these methods can be applied in practical situations, transforming your development workflow. Whether you are a novice coder or an experienced programmer looking to refine your skills, mastering string concatenation in JavaScript is a stepping stone toward creating more dynamic and responsive web applications. Prepare to unlock the full potential of your coding capabilities as we explore the various ways to concatenate strings in JavaScript
Using the Concatenation Operator
In JavaScript, the simplest way to concatenate strings is by using the `+` operator. This operator allows you to combine two or more strings into a single string. Here are some key points about using the concatenation operator:
- It can concatenate literals, variables, or a combination of both.
- You can concatenate multiple strings in a single statement.
For example:
javascript
let str1 = “Hello, “;
let str2 = “world!”;
let result = str1 + str2;
console.log(result); // Output: Hello, world!
Template Literals
Another modern approach to string concatenation is using template literals, which are enclosed by backticks (“ ` “). This method allows for easier string interpolation and multiline strings. You can embed expressions directly within the string by using `${expression}` syntax.
Example:
javascript
let name = “Alice”;
let greeting = `Hello, ${name}! Welcome to our site.`;
console.log(greeting); // Output: Hello, Alice! Welcome to our site.
String.concat() Method
JavaScript also provides the `String.concat()` method, which can concatenate multiple strings. While this method is less commonly used compared to the `+` operator and template literals, it can still be useful in certain scenarios.
Example usage:
javascript
let str1 = “Good “;
let str2 = “morning”;
let result = str1.concat(str2);
console.log(result); // Output: Good morning
Comparative Overview of Concatenation Methods
The following table summarizes the different methods of string concatenation in JavaScript, highlighting their key features.
Method | Syntax | Key Features |
---|---|---|
Concatenation Operator | `string1 + string2` | Simple, widely used, supports multiple strings. |
Template Literals | “ `string ${expression}` “ | Supports interpolation, multiline strings, clearer syntax. |
String.concat() | `string1.concat(string2)` | Less common, but useful for method chaining. |
Considerations for Performance
When choosing a method for string concatenation, it is important to consider performance, especially in scenarios involving large strings or extensive concatenation operations. The following points provide insights into performance considerations:
- Concatenation Operator: Generally performs well for small to medium-sized strings. However, excessive use in loops may lead to performance degradation.
- Template Literals: Offers a cleaner syntax and can be more readable, but performance is comparable to the concatenation operator.
- String.concat(): This method may have slight overhead due to function calls and is typically less performant than the direct concatenation operator.
while there are multiple ways to concatenate strings in JavaScript, the choice of method should depend on the specific use case and the readability of the code.
Concatenation Methods in JavaScript
In JavaScript, there are several ways to concatenate strings. Each method has its own use cases and benefits.
Using the `+` Operator
The most straightforward method for concatenating strings is by using the `+` operator. This allows for easy and readable string combinations.
javascript
let str1 = “Hello, “;
let str2 = “World!”;
let result = str1 + str2; // “Hello, World!”
Using the `concat()` Method
JavaScript strings have a built-in `concat()` method that can also be used to join multiple strings together.
javascript
let str1 = “Hello, “;
let str2 = “World!”;
let result = str1.concat(str2); // “Hello, World!”
Template Literals
Introduced in ES6, template literals provide a more powerful way to concatenate strings, especially when including variables or expressions.
javascript
let name = “World”;
let result = `Hello, ${name}!`; // “Hello, World!”
This method supports multiline strings and can embed expressions seamlessly.
Using `Array.join()` Method
For concatenating multiple strings efficiently, especially in loops or large datasets, using an array with the `join()` method can be advantageous.
javascript
let parts = [“Hello”, “World”];
let result = parts.join(“, “); // “Hello, World”
Performance Considerations
The choice of concatenation method can impact performance, particularly with a large number of strings. Here is a brief overview:
Method | Performance | Use Case |
---|---|---|
`+` Operator | Generally fast | Simple concatenation |
`concat()` | Similar to `+` | When clarity is preferred |
Template Literals | Very efficient | When including variables/expression |
`Array.join()` | Optimal for many | When combining multiple strings |
Practical Examples
Here are some practical scenarios where string concatenation is commonly used:
- Building URLs:
javascript
let baseUrl = “https://api.example.com/users/”;
let userId = 123;
let url = baseUrl + userId; // “https://api.example.com/users/123”
- Dynamic Messages:
javascript
let userName = “Alice”;
let greeting = `Welcome back, ${userName}!`; // “Welcome back, Alice!”
- Creating CSV Strings:
javascript
let headers = [“Name”, “Age”, “City”];
let data = [“John”, 30, “New York”];
let csv = headers.join(“, “) + “\n” + data.join(“, “); // “Name, Age, City\nJohn, 30, New York”
Understanding the various methods for string concatenation in JavaScript allows for more efficient and readable code, tailored to specific scenarios and performance needs.
Expert Insights on String Concatenation in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “Concatenating strings in JavaScript can be achieved using the `+` operator or the `concat()` method. The `+` operator is the most straightforward approach, while `concat()` can be useful for chaining multiple strings together in a more readable manner.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “When working with string concatenation in JavaScript, I recommend using template literals, which allow for interpolation and multi-line strings. This method enhances code readability and maintainability compared to traditional concatenation methods.”
Sarah Lee (JavaScript Educator, WebDev Academy). “It is essential to understand the implications of string concatenation in terms of performance, especially when dealing with large datasets. Using `Array.join()` to concatenate multiple strings can often yield better performance than repeated use of the `+` operator.”
Frequently Asked Questions (FAQs)
How do I concatenate strings in JavaScript?
To concatenate strings in JavaScript, you can use the `+` operator or the `concat()` method. For example, `let result = “Hello, ” + “world!”;` or `let result = “Hello, “.concat(“world!”);`.
Can I concatenate strings using template literals?
Yes, you can use template literals for string concatenation. By enclosing your strings in backticks (“ ` “), you can include variables and expressions directly. For example, “let result = `Hello, ${name}!`;“.
Is there a performance difference between using `+` and `concat()`?
In most cases, there is no significant performance difference for small strings. However, using the `+` operator is generally preferred for its simplicity and readability.
Can I concatenate multiple strings at once?
Yes, you can concatenate multiple strings using the `+` operator or `concat()` method. For example, `let result = “Hello, ” + “world!” + ” How are you?”;` or `let result = “Hello, “.concat(“world!”, ” How are you?”);`.
What happens if I concatenate a string with a number?
When you concatenate a string with a number, JavaScript converts the number to a string and combines them. For example, `”The answer is ” + 42` results in `”The answer is 42″`.
Are there any alternatives to concatenating strings in JavaScript?
Yes, you can use the `Array.join()` method to concatenate strings. For example, `let result = [‘Hello’, ‘world’].join(‘, ‘);` produces `”Hello, world”`.
In JavaScript, concatenating strings can be achieved through several methods, each with its own advantages. The most common methods include using the `+` operator, the `concat()` method, and template literals. The `+` operator is straightforward and widely used for combining strings, while the `concat()` method offers a more explicit approach. Template literals, introduced in ES6, provide a modern and flexible way to create strings, allowing for embedded expressions and multi-line strings.
One of the key takeaways is the versatility of string concatenation methods in JavaScript. The choice of method can depend on the specific needs of the application, such as readability, performance, or the need for dynamic content. Template literals, for instance, are particularly useful when dealing with variables and expressions, as they enhance code clarity and maintainability.
Additionally, it is important to consider potential performance implications when concatenating strings, especially in scenarios involving large amounts of data or frequent operations. While the differences may be negligible for small strings, using template literals or array joins can be more efficient in performance-critical applications. Understanding these nuances can lead to better coding practices and improved application performance.
Author Profile
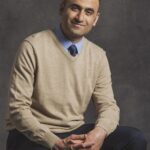
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?