How Can You Connect to Snowflake Using Python?
In the age of data-driven decision-making, organizations are increasingly turning to cloud-based data warehousing solutions to harness the power of their information. Snowflake, a leader in this domain, offers a robust platform that enables businesses to store, analyze, and share data seamlessly. However, to fully leverage Snowflake’s capabilities, you need to connect to it using the right tools and languages. For Python enthusiasts, this opens up a world of possibilities for data manipulation and analytics. In this article, we will guide you through the essential steps to connect to Snowflake using Python, empowering you to unlock the full potential of your data.
Connecting to Snowflake with Python is a straightforward process that allows developers and data analysts to interact with their data warehouse efficiently. By utilizing libraries such as `snowflake-connector-python`, users can establish a connection, execute SQL queries, and retrieve results directly within their Python environment. This integration not only streamlines workflows but also enhances the ability to perform complex data operations and analytics with ease.
As we delve deeper into this topic, we will explore the prerequisites for setting up your environment, the necessary configurations, and best practices for maintaining a secure and efficient connection. Whether you are a seasoned Python developer or a newcomer eager to explore the world of data
Establishing a Connection to Snowflake
To connect to Snowflake using Python, you need to utilize the `snowflake-connector-python` library, which provides a robust way to interact with your Snowflake instance.
First, ensure that you have the required library installed. You can do this using pip:
“`bash
pip install snowflake-connector-python
“`
Once the library is installed, you can establish a connection using the following parameters:
- user: Your Snowflake username.
- password: Your Snowflake password.
- account: The account identifier, which may include the region and cloud provider.
- warehouse: The name of the warehouse you want to use.
- database: The name of the database you will connect to.
- schema: The schema within the database.
The connection can be established with the following code snippet:
“`python
import snowflake.connector
Establishing the connection
conn = snowflake.connector.connect(
user=’YOUR_USERNAME’,
password=’YOUR_PASSWORD’,
account=’YOUR_ACCOUNT’,
warehouse=’YOUR_WAREHOUSE’,
database=’YOUR_DATABASE’,
schema=’YOUR_SCHEMA’
)
“`
Executing Queries
Once a connection is established, you can execute SQL queries using the cursor object. Here’s how you can do this:
“`python
Create a cursor object
cur = conn.cursor()
Execute a query
try:
cur.execute(“SELECT CURRENT_VERSION()”)
Fetch the result
version = cur.fetchone()
print(“Current Snowflake version:”, version[0])
finally:
cur.close() Always close the cursor
“`
Managing Connection Parameters
When connecting to Snowflake, it’s important to manage your connection parameters properly to ensure security and efficiency. Below are some key practices:
- Environment Variables: Store sensitive information like passwords in environment variables rather than hardcoding them.
- Connection Pooling: If your application requires multiple connections, consider using connection pooling for better performance.
- Secure Connection: Ensure SSL is enabled for secure data transmission.
Here’s a simple table summarizing connection parameters:
Parameter | Description |
---|---|
user | Your Snowflake account username. |
password | Your Snowflake account password. |
account | Your Snowflake account identifier. |
warehouse | The virtual warehouse for query execution. |
database | The database you want to connect to. |
schema | The schema within the database. |
Closing the Connection
After completing your operations, it is crucial to close the connection to free up resources. You can do this with the following code:
“`python
Close the connection
conn.close()
“`
By following these steps, you can effectively connect to Snowflake using Python and manage your database interactions efficiently.
Prerequisites
To connect to Snowflake using Python, ensure that you have the following prerequisites in place:
- Snowflake Account: You must have access to a Snowflake account.
- Python Environment: A working Python environment (Python 3.6 or later is recommended).
- Snowflake Connector for Python: This can be installed via pip.
Installation of Snowflake Connector
Install the Snowflake Connector for Python using the following command in your terminal or command prompt:
“`bash
pip install snowflake-connector-python
“`
This command fetches the latest version of the Snowflake connector from the Python Package Index (PyPI) and installs it in your Python environment.
Basic Connection Setup
To connect to Snowflake, you will need to provide several parameters, including your account information, username, password, and warehouse details. Below is an example of how to set up a basic connection:
“`python
import snowflake.connector
Establish the connection
conn = snowflake.connector.connect(
user=’YOUR_USERNAME’,
password=’YOUR_PASSWORD’,
account=’YOUR_ACCOUNT’,
warehouse=’YOUR_WAREHOUSE’,
database=’YOUR_DATABASE’,
schema=’YOUR_SCHEMA’
)
“`
Connection Parameters
The connection requires the following parameters:
Parameter | Description |
---|---|
user | Your Snowflake username |
password | Your Snowflake password |
account | Your Snowflake account name (e.g., ‘xy12345.us-east-1’) |
warehouse | The name of the warehouse to use |
database | The name of the database to connect to |
schema | The name of the schema to use |
Executing Queries
Once connected, you can execute SQL queries using the following approach:
“`python
Create a cursor object
cur = conn.cursor()
Execute a query
cur.execute(“SELECT CURRENT_VERSION()”)
Fetch the results
result = cur.fetchone()
print(result)
Close the cursor
cur.close()
“`
This example demonstrates how to create a cursor, execute a SQL query, fetch the result, and close the cursor afterward.
Handling Connection Errors
When connecting to Snowflake, it is essential to handle potential connection errors gracefully. Use a try-except block as shown below:
“`python
try:
conn = snowflake.connector.connect(
user=’YOUR_USERNAME’,
password=’YOUR_PASSWORD’,
account=’YOUR_ACCOUNT’
)
Perform operations
except snowflake.connector.errors.Error as e:
print(f”Error connecting to Snowflake: {e}”)
finally:
conn.close()
“`
This code will catch any connection errors and print an appropriate error message while ensuring that the connection is closed in the end.
Closing the Connection
It is crucial to close the connection to Snowflake after completing your database operations to free up resources. This can be done simply by calling:
“`python
conn.close()
“`
This step ensures that all resources are properly released and avoids potential memory leaks.
Connecting to Snowflake with Python: Expert Insights
Dr. Emily Chen (Data Scientist, Cloud Analytics Group). “To connect to Snowflake using Python, it is essential to utilize the Snowflake Connector for Python. This library simplifies the authentication process and allows for seamless data operations. Ensure that you have the correct credentials and network configurations to establish a secure connection.”
Michael Thompson (Senior Database Engineer, Tech Innovations Inc.). “When connecting to Snowflake with Python, leveraging environment variables for storing sensitive information like user credentials is a best practice. This approach enhances security and makes your code cleaner and more maintainable.”
Lisa Patel (Cloud Solutions Architect, DataBridge Solutions). “I recommend using the ‘snowflake-connector-python’ package, which can be easily installed via pip. Additionally, familiarize yourself with the connection parameters such as account, user, password, and warehouse to ensure a successful connection to your Snowflake instance.”
Frequently Asked Questions (FAQs)
How do I install the Snowflake Connector for Python?
To install the Snowflake Connector for Python, use the following pip command: `pip install snowflake-connector-python`. Ensure that you have Python and pip installed on your system.
What are the prerequisites for connecting to Snowflake using Python?
You need to have a Snowflake account, the Snowflake Connector for Python installed, and valid credentials (username, password, account identifier) to establish a connection.
How can I establish a connection to Snowflake in Python?
You can establish a connection using the following code snippet:
“`python
import snowflake.connector
conn = snowflake.connector.connect(
user=’YOUR_USERNAME’,
password=’YOUR_PASSWORD’,
account=’YOUR_ACCOUNT’
)
“`
Replace `YOUR_USERNAME`, `YOUR_PASSWORD`, and `YOUR_ACCOUNT` with your actual credentials.
How do I execute a query in Snowflake using Python?
After establishing a connection, you can execute a query using the `cursor` object as follows:
“`python
cursor = conn.cursor()
cursor.execute(“SELECT * FROM your_table”)
for row in cursor:
print(row)
“`
What should I do if I encounter connection errors?
Check your network settings, verify your credentials, ensure that your Snowflake account is active, and confirm that your IP address is whitelisted in Snowflake’s settings.
How can I close the connection to Snowflake in Python?
To close the connection, use the `close()` method on the connection object:
“`python
conn.close()
“`
This ensures that all resources are released properly.
Connecting to Snowflake using Python is a straightforward process that primarily involves utilizing the Snowflake Connector for Python. This library allows developers to easily establish a connection to their Snowflake data warehouse and execute SQL queries directly from their Python applications. The steps typically include installing the connector, configuring the connection parameters such as account, user, password, and database, and then using the connection to perform various database operations.
One of the key takeaways from the discussion is the importance of securely managing credentials. It is advisable to use environment variables or configuration files to store sensitive information like passwords, rather than hardcoding them into the application. This practice enhances security and helps in maintaining best practices in software development.
Additionally, leveraging the features of the Snowflake Connector can significantly enhance data handling capabilities. The connector supports various functionalities such as executing queries, fetching results, and handling large datasets efficiently. By understanding and utilizing these features, developers can optimize their data workflows and improve overall performance when interacting with Snowflake.
connecting to Snowflake using Python is an essential skill for data professionals looking to leverage cloud data warehousing capabilities. By following the outlined steps and adhering to best practices for security and performance, users can effectively integrate Snowflake
Author Profile
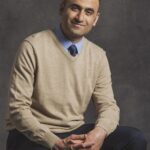
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?