How Can You Continue a Line in Python for Better Code Readability?
When diving into the world of Python programming, one may encounter situations where lines of code become too lengthy or complex to fit neatly within the confines of a single line. Whether you are writing a function with multiple parameters, constructing a long string, or simply trying to maintain readability in your code, knowing how to effectively continue a line is an essential skill for any programmer. This practice not only enhances the clarity of your code but also adheres to the principles of clean coding, making your scripts easier to read and maintain.
In Python, line continuation can be achieved through various methods, each serving a unique purpose depending on the context of your code. Understanding these techniques can help you manage the flow of your programming logic without sacrificing readability. For instance, you might find yourself using implicit line continuation within parentheses, brackets, or braces, which allows you to break lines naturally without the need for additional syntax. Alternatively, explicit line continuation can be employed using the backslash character, providing a clear indication of continuation for the reader.
As you explore the nuances of line continuation in Python, you’ll discover that these techniques are not merely about aesthetics; they are vital for writing efficient, professional-grade code. By mastering how to continue a line, you will not only improve your coding practices but also enhance your overall
Using Backslashes for Line Continuation
In Python, one straightforward method to continue a line is by using a backslash (`\`). This character tells the interpreter that the line is not finished and continues onto the next line. It is particularly useful for breaking long statements into more readable segments.
Example:
“`python
total = 1 + 2 + 3 + 4 + 5 + \
6 + 7 + 8 + 9 + 10
“`
While the backslash is effective, it is essential to ensure there are no trailing spaces after it, as this can lead to syntax errors.
Using Parentheses, Brackets, or Braces
Another approach to continuing a line is by using parentheses `()`, brackets `[]`, or braces `{}`. When an expression is enclosed within these symbols, Python implicitly allows line continuation.
Example with parentheses:
“`python
result = (1 + 2 + 3 +
4 + 5 + 6)
“`
Example with brackets:
“`python
my_list = [
1, 2, 3,
4, 5, 6
]
“`
Example with braces:
“`python
my_dict = {
‘name’: ‘Alice’,
‘age’: 30,
‘city’: ‘New York’
}
“`
This method is often preferred for its readability and clarity, especially in complex expressions.
Using Triple Quotes for Multiline Strings
When working with strings that span multiple lines, you can use triple quotes (`”’` or `”””`). This allows you to create a string that includes line breaks without needing any special characters.
Example:
“`python
multiline_string = “””This is a string
that spans multiple lines
without any issues.”””
“`
This method is particularly useful for documentation strings (docstrings) or when you want to include formatted text.
Comparison of Line Continuation Methods
The following table summarizes the different methods of line continuation in Python:
Method | Description | Example |
---|---|---|
Backslash | Explicitly tells Python to continue the line | total = 1 + 2 + 3 + \ 6 + 7 + 8 |
Parentheses | Implicit continuation for expressions | result = (1 + 2 + 3 + 4) |
Brackets | Use for lists or arrays over multiple lines | my_list = [1, 2, 3, 4] |
Braces | Used for dictionaries or sets across lines | my_dict = {‘key’: ‘value’} |
Triple Quotes | For multiline strings | “””This is a string””” |
Each method has its suitable context, and choosing the right one can enhance the readability and maintainability of your code.
Using Backslash for Line Continuation
In Python, one of the simplest methods to continue a line of code onto the next line is by using a backslash (`\`). This character serves as an explicit line continuation indicator, allowing you to break long statements into more manageable parts.
Example:
“`python
total = 1 + 2 + 3 + \
4 + 5 + 6
“`
In this example, the backslash at the end of the first line tells Python that the statement continues on the next line. It is important to ensure there are no spaces after the backslash, as this will cause a syntax error.
Implicit Line Continuation with Parentheses
Python also allows implicit line continuation when using parentheses, brackets, or braces. This approach is often preferred as it enhances readability and does not require a backslash.
Example:
“`python
total = (1 + 2 + 3 +
4 + 5 + 6)
“`
Here, the parentheses allow the expression to extend over multiple lines naturally. The same implicit continuation can be used with lists, dictionaries, and function calls:
Example with a list:
“`python
my_list = [
1, 2, 3,
4, 5, 6
]
“`
Continuation in Function Calls
When calling functions with many arguments, you can also break the call into multiple lines for better readability. This is done using either parentheses or by separating arguments appropriately.
Example using parentheses:
“`python
result = my_function(
argument1, argument2,
argument3, argument4
)
“`
Alternatively, if the function takes a long list of arguments, you might choose to format them like this:
“`python
result = my_function(argument1,
argument2,
argument3)
“`
Using Triple Quotes for Multiline Strings
When dealing with long strings, Python allows the use of triple quotes (`”’` or `”””`) for multiline strings. This feature is particularly useful for creating long textual content without needing explicit line continuation.
Example:
“`python
long_string = “””This is a long string
that spans multiple lines
without requiring any special
line continuation characters.”””
“`
This method is effective for documentation strings (docstrings) or any context where you need to maintain the format of a multi-line string.
Best Practices for Line Continuation
When utilizing line continuation, consider the following best practices:
- Readability: Aim to keep lines under a certain length (e.g., 79 or 100 characters) for better readability.
- Consistent Style: Choose between explicit (using backslashes) and implicit (using parentheses) line continuations, and remain consistent throughout your code.
- Commenting: If breaking lines in complex expressions, adding comments can help clarify the logic for future reference.
By applying these methods and practices, you can effectively manage long lines of code in Python, enhancing both readability and maintainability.
Expert Insights on Continuing Lines in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, you can continue a line using a backslash (\) at the end of the line, which indicates that the statement continues on the next line. This is particularly useful for improving code readability, especially when dealing with long expressions or function calls.”
James Liu (Lead Software Engineer, CodeCraft Solutions). “Another effective method to continue a line in Python is to use parentheses, brackets, or braces. By enclosing expressions within these structures, you can split long lines without needing a backslash, which enhances clarity and maintains the code’s logical flow.”
Sarah Patel (Python Instructor, Coding Academy). “When teaching Python, I emphasize the importance of using implicit line continuation through parentheses for multi-line statements. It not only makes the code cleaner but also helps beginners avoid common pitfalls associated with the backslash method.”
Frequently Asked Questions (FAQs)
What does it mean to continue a line in Python?
Continuing a line in Python refers to splitting a single logical line of code across multiple physical lines for better readability, especially when dealing with long expressions or statements.
How can I continue a line using a backslash?
You can continue a line by placing a backslash (`\`) at the end of the line. This indicates that the statement continues on the next line, allowing for clearer formatting without breaking the code’s logic.
Are there other ways to continue a line in Python?
Yes, you can use parentheses `()`, brackets `[]`, or braces `{}` to continue a line. When an expression is enclosed in these symbols, Python automatically understands that the statement continues on the next line.
Can I continue a line with a string literal?
Yes, string literals can be continued across lines by using triple quotes (`”’` or `”””`). This allows for multi-line strings without needing to use backslashes.
What happens if I forget to use a line continuation character?
If you forget to use a line continuation character when needed, Python will raise a `SyntaxError`, indicating that it encountered an unexpected end of the line.
Is there a limit to how many times I can continue a line in Python?
There is no explicit limit to how many times you can continue a line in Python. However, excessive continuation may lead to decreased readability, which is generally discouraged in coding best practices.
In Python, continuing a line of code is essential for maintaining readability and organization, especially when dealing with lengthy statements or complex expressions. There are several methods to achieve this, including the use of the backslash (`\`) as a line continuation character, utilizing parentheses, brackets, or braces, and employing implicit line continuation within multi-line constructs. Each method serves to ensure that the code remains clear and manageable, allowing developers to structure their code effectively.
One of the most common practices is to use the backslash, which explicitly indicates that the line of code is not complete and continues on the next line. However, using parentheses, brackets, or braces is often preferred as it enhances readability without the need for a backslash. This method allows for natural breaks in the code, particularly in function calls or when defining lists and dictionaries. Understanding these techniques is crucial for writing clean and maintainable Python code.
In summary, mastering line continuation in Python is a key skill for developers aiming to write efficient and readable code. By utilizing the appropriate methods for line continuation, programmers can avoid syntax errors and improve the overall structure of their scripts. Emphasizing clarity in code not only benefits the original author but also aids others who may read or maintain the
Author Profile
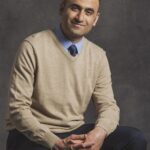
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?