How Can You Convert a List to a Dictionary in Python?
In the world of Python programming, data manipulation is a fundamental skill that every developer should master. Among the various data structures available, lists and dictionaries are two of the most commonly used. While lists are excellent for storing ordered collections of items, dictionaries shine when it comes to associating keys with values for quick lookups. But what happens when you find yourself with a list and you need to convert it into a dictionary? This transformation can open up new possibilities for organizing and accessing your data efficiently. In this article, we will explore the methods and techniques to seamlessly convert lists into dictionaries, empowering you to enhance your coding capabilities.
Understanding how to convert a list to a dictionary in Python is not just about syntax; it’s about grasping the underlying principles of data structures. Lists are typically used to hold sequences of items, while dictionaries provide a way to map unique keys to corresponding values. This conversion process can be particularly useful in scenarios where you want to create a more structured representation of your data, such as when you have a list of pairs or a list of items that need to be indexed by a specific attribute.
As we delve deeper into this topic, we will examine various approaches to achieve this conversion, highlighting the flexibility and power of Python’s built-in functions. Whether you’re
Using the `dict()` Constructor
One straightforward method to convert a list to a dictionary is by utilizing the built-in `dict()` constructor. This method is particularly effective when dealing with a list of tuples, where each tuple contains a key-value pair.
For example:
“`python
list_of_tuples = [(‘a’, 1), (‘b’, 2), (‘c’, 3)]
dictionary = dict(list_of_tuples)
“`
This approach directly transforms the list of tuples into a dictionary, resulting in the following output:
“`python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
“`
Using Dictionary Comprehension
Dictionary comprehension provides a concise way to create dictionaries from lists. This technique is especially useful for generating dictionaries from lists that contain a series of elements, allowing you to define both the keys and values in a single expression.
Consider the following example:
“`python
keys = [‘a’, ‘b’, ‘c’]
values = [1, 2, 3]
dictionary = {keys[i]: values[i] for i in range(len(keys))}
“`
This creates a dictionary as follows:
“`python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
“`
Alternatively, if you have a list of values and want to assign them sequentially to keys, you can use `enumerate()`:
“`python
values = [1, 2, 3]
dictionary = {f’key_{i}’: value for i, value in enumerate(values)}
“`
This results in:
“`python
{‘key_0’: 1, ‘key_1’: 2, ‘key_2’: 3}
“`
Using `zip()` Function
The `zip()` function can also be utilized to convert two lists into a dictionary. This is particularly useful when you have one list of keys and another list of corresponding values.
Here’s how it can be done:
“`python
keys = [‘a’, ‘b’, ‘c’]
values = [1, 2, 3]
dictionary = dict(zip(keys, values))
“`
The resulting dictionary will be:
“`python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
“`
The `zip()` function pairs elements from the two lists together, and the `dict()` constructor then converts these pairs into a dictionary.
Example Comparison Table
To illustrate the different methods for converting lists to dictionaries, consider the following table:
Method | Code Example | Output |
---|---|---|
dict() Constructor | dict([(‘a’, 1), (‘b’, 2)]) | {‘a’: 1, ‘b’: 2} |
Dictionary Comprehension | {keys[i]: values[i] for i in range(len(keys))} | {‘a’: 1, ‘b’: 2, ‘c’: 3} |
zip() Function | dict(zip(keys, values)) | {‘a’: 1, ‘b’: 2, ‘c’: 3} |
Each of these methods has its own advantages depending on the specific structure of the list and the desired outcome.
Methods to Convert a List to a Dictionary in Python
In Python, there are several efficient methods to convert a list into a dictionary. The approach you choose will depend on the structure of your list and the desired outcome for your dictionary.
Using Dictionary Comprehension
Dictionary comprehension offers a concise way to create dictionaries from lists. This method is particularly useful when you have pairs of elements in the list that can be treated as key-value pairs.
“`python
my_list = [(‘a’, 1), (‘b’, 2), (‘c’, 3)]
my_dict = {key: value for key, value in my_list}
“`
Example:
- Given a list of tuples `my_list = [(‘name’, ‘Alice’), (‘age’, 25), (‘city’, ‘New York’)]`, the resulting dictionary will be:
“`python
{‘name’: ‘Alice’, ‘age’: 25, ‘city’: ‘New York’}
“`
Using the `dict()` Constructor
The `dict()` constructor can transform lists of tuples directly into dictionaries. This method is straightforward and works well when the list structure is clear.
“`python
my_list = [(‘x’, 10), (‘y’, 20)]
my_dict = dict(my_list)
“`
Key Points:
- The list must contain pairs of elements (key, value).
- Any duplicate keys will result in the last value being used.
Using `zip()` Function
When you have two separate lists—one for keys and another for values—you can use the `zip()` function in conjunction with the `dict()` constructor.
“`python
keys = [‘name’, ‘age’, ‘city’]
values = [‘Bob’, 30, ‘Los Angeles’]
my_dict = dict(zip(keys, values))
“`
Resulting Dictionary:
- The above code produces:
“`python
{‘name’: ‘Bob’, ‘age’: 30, ‘city’: ‘Los Angeles’}
“`
Using `enumerate()` for Index-based Keys
If you want to convert a list into a dictionary with indices as keys, `enumerate()` can be particularly useful.
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_dict = {index: value for index, value in enumerate(my_list)}
“`
Example Output:
- This will generate:
“`python
{0: ‘apple’, 1: ‘banana’, 2: ‘cherry’}
“`
Handling Duplicate Keys
When converting a list to a dictionary, it’s crucial to consider how to handle duplicate keys. Python dictionaries do not allow duplicate keys, meaning the last occurrence will be retained.
Example:
“`python
my_list = [(‘a’, 1), (‘b’, 2), (‘a’, 3)]
my_dict = dict(my_list)
“`
Result:
- The resulting dictionary will be:
“`python
{‘a’: 3, ‘b’: 2}
“`
Strategies for Avoiding Overwrites:
- Use a list of values for each key if duplicates are expected.
- Implement logic to aggregate values into lists or other data structures.
Utilizing these methods, you can effectively convert lists into dictionaries tailored to your specific needs, enabling better data organization and manipulation in Python programming tasks.
Expert Insights on Converting Lists to Dictionaries in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Converting a list to a dictionary in Python can be efficiently accomplished using dictionary comprehensions or the built-in `dict()` function. This flexibility allows developers to create key-value pairs dynamically, enhancing code readability and performance.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When working with lists of tuples, the `dict()` constructor is particularly useful. It transforms a list of pairs into a dictionary seamlessly, which is a common requirement in data processing tasks.”
Sarah Patel (Python Developer Advocate, Open Source Community). “Utilizing the `zip()` function in combination with lists can yield a powerful method for dictionary creation. This approach allows for the pairing of two lists into a dictionary, which is especially beneficial when managing related datasets.”
Frequently Asked Questions (FAQs)
How can I convert a list of tuples into a dictionary in Python?
You can convert a list of tuples into a dictionary using the `dict()` constructor. For example, `dict([(key1, value1), (key2, value2)])` will create a dictionary with the specified key-value pairs.
What is the syntax for converting a list of lists into a dictionary?
To convert a list of lists into a dictionary, you can use a dictionary comprehension. For instance, `{item[0]: item[1] for item in list_of_lists}` will create a dictionary where the first element of each sub-list is the key and the second element is the value.
Can I convert a list with unique elements into a dictionary with default values?
Yes, you can use a dictionary comprehension to achieve this. For example, `{key: default_value for key in list}` will create a dictionary where each unique element in the list is a key and all keys have the same default value.
Is it possible to convert a list of keys into a dictionary without values?
Yes, you can create a dictionary with keys from the list and assign a default value, such as `None`. For example, `{key: None for key in list_of_keys}` will create a dictionary with the list elements as keys and `None` as their values.
How do I handle duplicate keys when converting a list to a dictionary?
When converting a list to a dictionary, if there are duplicate keys, only the last occurrence will be retained. To handle duplicates, consider using a `defaultdict` or aggregating values into a list for each key.
What method can I use to convert two lists into a dictionary?
You can use the `zip()` function combined with the `dict()` constructor. For example, `dict(zip(list_of_keys, list_of_values))` will create a dictionary pairing elements from the two lists as key-value pairs.
Converting a list to a dictionary in Python can be achieved through various methods, depending on the structure of the list and the desired outcome. Common approaches include using dictionary comprehensions, the `dict()` constructor, and the `zip()` function. Each method allows for flexibility in how the list elements are organized into key-value pairs, making it essential to choose the most suitable approach based on the specific requirements of the task.
One of the most straightforward methods is to use dictionary comprehensions, which provide a concise way to create dictionaries from lists. This method is particularly useful when transforming a list of tuples or a list of lists into a dictionary. Alternatively, the `zip()` function can be employed when you have two separate lists—one for keys and another for values—allowing for a clean and efficient pairing of elements.
In summary, understanding the structure of your data and the desired output is crucial when converting a list to a dictionary in Python. By leveraging the appropriate methods, developers can efficiently organize data and enhance the functionality of their applications. Mastering these techniques not only improves code readability but also optimizes performance in data manipulation tasks.
Author Profile
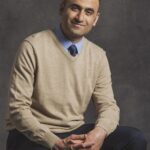
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?