How Can You Convert a String to a Float in Python?
In the realm of programming, data types serve as the foundation upon which we build our applications. Among these types, strings and floats hold significant importance, often requiring conversion from one to the other to facilitate calculations and data processing. If you’ve ever found yourself grappling with the need to transform a string representation of a number into a float for mathematical operations in Python, you’re not alone. This seemingly simple task can be a crucial step in ensuring your code runs smoothly and efficiently.
Converting a string to a float in Python is a common requirement that arises in various scenarios, from parsing user input to processing data from external sources. Understanding the mechanics behind this conversion not only enhances your coding skills but also empowers you to handle numerical data with confidence. Python provides straightforward methods to achieve this transformation, making it accessible even for those who are new to programming.
As we delve deeper into the nuances of this conversion process, we’ll explore the functions and techniques available in Python, along with best practices to avoid common pitfalls. Whether you’re looking to refine your coding abilities or simply seeking to understand how to manipulate data types effectively, this guide will equip you with the knowledge you need to seamlessly convert strings to floats in your Python projects.
Converting Strings to Floats
To convert a string to a float in Python, the primary function used is `float()`. This function takes a string (or a number) as an argument and converts it into a floating-point number. The conversion is straightforward, but there are some considerations to keep in mind, especially when dealing with different formats of numeric strings.
Basic Conversion
The simplest form of conversion can be demonstrated as follows:
“`python
string_value = “3.14”
float_value = float(string_value)
print(float_value) Output: 3.14
“`
In this example, the string `”3.14″` is converted to the float `3.14`.
Handling Different Formats
When converting strings that represent numbers, ensure that they are formatted correctly. Common issues that can arise include:
- Strings with commas (e.g., `”1,234.56″`).
- Strings that include currency symbols (e.g., `”$100.00″`).
- Strings that are not valid representations of numbers (e.g., `”abc”`).
To handle these cases, you may need to preprocess the string before conversion. Here are some methods for common scenarios:
- Removing Commas: You can use the `replace()` method to remove commas.
- Stripping Currency Symbols: Use slicing or string methods to remove unwanted characters.
Example of Preprocessing
Here’s how you can handle strings with commas and currency symbols:
“`python
def preprocess_and_convert(string_value):
Remove commas and currency symbols
cleaned_string = string_value.replace(“,”, “”).replace(“$”, “”)
return float(cleaned_string)
Example usage
string_value = “$1,234.56”
float_value = preprocess_and_convert(string_value)
print(float_value) Output: 1234.56
“`
Error Handling
When converting strings that may not represent valid float values, it is prudent to handle potential errors. The `ValueError` exception can be caught to manage invalid inputs gracefully.
“`python
def safe_convert(string_value):
try:
return float(string_value)
except ValueError:
return None or handle the error as needed
Example usage
result = safe_convert(“abc”)
print(result) Output: None
“`
Summary of Conversion Methods
The following table summarizes the methods discussed for converting strings to floats:
Method | Description |
---|---|
float() | Directly converts a properly formatted string to a float. |
replace() | Used for removing unwanted characters like commas or currency symbols. |
try-except | Catches conversion errors and manages invalid inputs. |
Using these methods, you can effectively convert strings to floats in Python, ensuring that your data is processed accurately and robustly.
Converting Strings to Floats
In Python, converting a string to a float is a straightforward process using the built-in `float()` function. This function takes a string representation of a number and converts it into a floating-point number.
Basic Usage of the `float()` Function
To convert a string to a float, you can simply pass the string to the `float()` function:
“`python
string_value = “3.14”
float_value = float(string_value)
“`
In this example, `float_value` will hold the value `3.14` as a float.
Handling Different String Formats
The `float()` function can handle various string formats, including:
- Standard decimal numbers: `”123.45″`
- Scientific notation: `”1e-3″` (equivalent to `0.001`)
- Leading and trailing spaces: `” 2.71 “` (will be converted correctly)
Example:
“`python
values = [“3.14”, “1e-3″, ” 2.71 “]
float_values = [float(value) for value in values]
“`
This will result in `float_values` containing `[3.14, 0.001, 2.71]`.
Handling Invalid Inputs
Attempting to convert a string that does not represent a valid float will raise a `ValueError`. To handle such cases gracefully, it is advisable to use a try-except block:
“`python
def safe_float_conversion(string_value):
try:
return float(string_value)
except ValueError:
return None or handle it in a way that suits your application
Example usage
result = safe_float_conversion(“invalid_number”)
“`
In this case, `result` will be `None`.
Examples of Conversion
Here are a few examples illustrating the conversion of various string inputs to floats:
Input String | Resulting Float |
---|---|
`”42.0″` | `42.0` |
`”0.001″` | `0.001` |
`”-3.14″` | `-3.14` |
`”1e4″` | `10000.0` |
`”NaN”` | `nan` |
Performance Considerations
Converting strings to floats is generally efficient, but performance may degrade with very large datasets or when done repeatedly in a loop. For bulk conversions, consider using libraries like NumPy, which can handle arrays of strings and convert them to floats more efficiently.
Example with NumPy:
“`python
import numpy as np
string_array = np.array([“1.0”, “2.5”, “3.14”])
float_array = string_array.astype(float)
“`
This method provides better performance for large datasets.
Converting strings to floats in Python is simple and effective with the `float()` function. Proper error handling ensures robustness, and utilizing libraries like NumPy can enhance performance in data-intensive applications.
Expert Insights on Converting Strings to Floats in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Converting a string to a float in Python can be accomplished effortlessly using the built-in `float()` function. This method is straightforward and handles various string formats, including those with decimal points, making it an essential tool for data manipulation.”
Mark Thompson (Data Scientist, Analytics Hub). “It is crucial to ensure that the string being converted is in a valid float format. Utilizing error handling with `try` and `except` blocks can prevent runtime errors and provide a more robust solution when dealing with user input or external data sources.”
Linda Martinez (Software Engineer, CodeCrafters). “When converting strings to floats, one must be aware of locale settings that can affect the decimal separator. For instance, some locales use commas instead of periods. Utilizing the `locale` module can help manage these discrepancies effectively.”
Frequently Asked Questions (FAQs)
How can I convert a string to a float in Python?
You can convert a string to a float in Python using the `float()` function. For example, `float(“3.14”)` will return `3.14`.
What happens if the string cannot be converted to a float?
If the string cannot be converted to a float, Python will raise a `ValueError`. For instance, trying to convert `float(“abc”)` will result in an error.
Can I convert a string with commas to a float?
No, the `float()` function does not accept strings with commas. You must remove the commas first, for example, by using `string.replace(“,”, “”)`.
Is it possible to convert a string representing a scientific notation to a float?
Yes, Python can convert strings in scientific notation to floats. For example, `float(“1e3”)` will return `1000.0`.
Are there any alternatives to using the float() function for conversion?
While `float()` is the most common method, you can also use libraries like `numpy` which provide additional functionality for handling numerical data types.
How can I handle exceptions when converting strings to floats?
You can use a `try-except` block to handle exceptions. For example:
“`python
try:
value = float(“your_string”)
except ValueError:
print(“Conversion failed.”)
“`
Converting a string to a float in Python is a straightforward process that can be accomplished using the built-in `float()` function. This function takes a string representation of a number and converts it into a floating-point number. It is important to ensure that the string is formatted correctly; otherwise, a `ValueError` will be raised. Common formats include standard decimal notation and scientific notation.
When performing this conversion, developers should be aware of potential issues such as leading or trailing whitespace, as well as the presence of non-numeric characters. Utilizing the `strip()` method can help eliminate unwanted spaces, while error handling can be implemented using try-except blocks to manage exceptions gracefully. This approach enhances the robustness of the code, especially when dealing with user input or data from external sources.
In summary, converting strings to floats in Python is a critical skill for developers working with numerical data. By understanding the proper use of the `float()` function and implementing best practices for error handling and data validation, programmers can ensure their applications handle numeric conversions effectively and reliably.
Author Profile
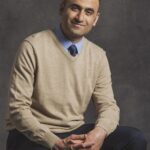
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?