How Can You Create a 2-Dimensional Array in Python?
In the world of programming, data organization is key to building efficient and effective applications. Among the various structures available to developers, arrays stand out for their simplicity and versatility. When it comes to handling data in a more complex manner, 2-dimensional arrays become an invaluable tool, allowing for the representation of data in a grid-like format. Whether you’re working on a game, a scientific simulation, or data analysis, mastering 2-dimensional arrays in Python can significantly enhance your coding capabilities.
Creating a 2-dimensional array in Python is not only straightforward but also opens up a realm of possibilities for data manipulation and visualization. This structure is particularly useful when you need to represent matrices, tables, or any data that can be logically organized in rows and columns. Python’s built-in lists provide a simple way to create these arrays, while libraries like NumPy offer advanced functionalities that make working with multidimensional data even easier.
As we delve deeper into the mechanics of creating and utilizing 2-dimensional arrays in Python, you’ll discover various methods, from basic list comprehension to leveraging powerful libraries. We’ll explore how to initialize, access, and manipulate these arrays, equipping you with the knowledge to effectively manage complex datasets in your projects. Whether you are a beginner or looking to refresh your skills, understanding
Creating a 2 Dimensional Array Using Lists
In Python, a simple way to create a two-dimensional array is by using a list of lists. Each inner list represents a row in the 2D array. This method is straightforward and allows for dynamic resizing.
“`python
Creating a 2D array with predefined values
array_2d = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
“`
You can access elements using two indices: the first for the row and the second for the column. For example, `array_2d[0][1]` retrieves the value `2`.
Creating a 2 Dimensional Array Using NumPy
NumPy, a powerful library for numerical computations, provides a more efficient way to create and manipulate two-dimensional arrays. To use NumPy, ensure you have it installed in your environment. You can create a 2D array with the `numpy.array()` function.
“`python
import numpy as np
Creating a 2D array using NumPy
array_2d_numpy = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
“`
NumPy arrays support various operations, including slicing, reshaping, and mathematical operations.
Creating a 2 Dimensional Array Using List Comprehensions
List comprehensions in Python provide a concise way to create a 2D array. This method is particularly useful for generating arrays with sequential or patterned values.
“`python
Creating a 3×3 2D array using list comprehension
array_2d_comprehension = [[j for j in range(3)] for i in range(3)]
“`
The above code generates a 2D array filled with values from 0 to 2 in each row.
Example of a 2 Dimensional Array
To illustrate how different methods can generate the same two-dimensional array, consider the following comparison:
Method | Code Example | Output |
---|---|---|
List of Lists | array_2d = [[1, 2], [3, 4]] | [[1, 2], [3, 4]] |
NumPy | array_2d_numpy = np.array([[1, 2], [3, 4]]) | array([[1, 2], [3, 4]]) |
List Comprehension | array_2d_comprehension = [[i + j for j in range(2)] for i in range(2)] | [[0, 1], [1, 2]] |
Each method has its advantages depending on the context of use. Using lists is intuitive, while NumPy offers extensive functionality for scientific computations. List comprehensions provide a succinct way to generate arrays programmatically.
Creating a 2 Dimensional Array in Python
Python does not have built-in support for 2D arrays in the same way that some other programming languages do. However, there are several methods to create 2D arrays using lists or libraries like NumPy.
Using Nested Lists
A straightforward way to create a 2D array is by using nested lists. Each inner list represents a row in the 2D array.
“`python
Creating a 2D array using nested lists
array_2d = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
“`
You can access elements using two indices, where the first index refers to the row and the second to the column:
“`python
element = array_2d[1][2] Accesses the element ‘6’
“`
Using NumPy Library
For more advanced operations and better performance, the NumPy library is widely used in Python. It provides an efficient way to create and manipulate 2D arrays.
Installation of NumPy
If you haven’t installed NumPy yet, you can do so using pip:
“`bash
pip install numpy
“`
Creating a 2D Array with NumPy
To create a 2D array in NumPy, use the `numpy.array()` function:
“`python
import numpy as np
Creating a 2D array using NumPy
array_2d_np = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
“`
You can also create a 2D array filled with zeros or ones:
“`python
Creating a 2D array of zeros
zero_array = np.zeros((3, 3))
Creating a 2D array of ones
ones_array = np.ones((3, 3))
“`
Accessing Elements in NumPy Arrays
Similar to nested lists, you can access elements in a NumPy array using indices:
“`python
element_np = array_2d_np[1, 2] Accesses the element ‘6’
“`
Manipulating 2D Arrays
With NumPy, you can perform various operations efficiently. Here are some common manipulations:
- Addition: Adding two arrays element-wise.
- Multiplication: Multiplying arrays element-wise.
- Transposition: Flipping an array over its diagonal.
For example, to add two arrays:
“`python
array_a = np.array([[1, 2], [3, 4]])
array_b = np.array([[5, 6], [7, 8]])
result = array_a + array_b Result: [[6, 8], [10, 12]]
“`
Creating and manipulating 2D arrays in Python can be efficiently handled using either nested lists or the NumPy library, depending on your needs for performance and functionality.
Expert Insights on Creating 2D Arrays in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Creating a 2-dimensional array in Python can be efficiently accomplished using the NumPy library. This library provides a powerful array object that allows for multidimensional data manipulation, making it ideal for scientific computing and data analysis.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For beginners, utilizing nested lists is a straightforward way to create 2D arrays in Python. However, for more complex applications, I recommend leveraging NumPy, as it offers enhanced performance and a plethora of functions tailored for array operations.”
Sarah Thompson (Python Developer, DevMasters). “When creating 2-dimensional arrays, understanding the difference between lists and NumPy arrays is crucial. While lists are flexible, NumPy arrays provide significant advantages in terms of speed and functionality, especially when handling large datasets.”
Frequently Asked Questions (FAQs)
How do I create a 2-dimensional array in Python?
You can create a 2-dimensional array in Python using nested lists or by utilizing libraries like NumPy. For nested lists, define a list containing other lists, e.g., `array = [[1, 2], [3, 4]]`. With NumPy, use `import numpy as np` followed by `array = np.array([[1, 2], [3, 4]])`.
What is the difference between a list of lists and a NumPy array?
A list of lists is a native Python data structure that can hold heterogeneous data types, while a NumPy array is a specialized data structure designed for numerical computations, offering better performance and functionality for mathematical operations.
Can I create a 2D array with specific dimensions in Python?
Yes, you can create a 2D array with specific dimensions using list comprehensions or NumPy. For example, `array = [[0 for _ in range(columns)] for _ in range(rows)]` creates a 2D list filled with zeros. Using NumPy, you can use `array = np.zeros((rows, columns))` to achieve the same.
How can I access elements in a 2-dimensional array?
To access elements in a 2D array, use indexing. For a list of lists, use `element = array[row][column]`. For a NumPy array, use `element = array[row, column]`.
Can I modify elements in a 2-dimensional array?
Yes, you can modify elements in both a list of lists and a NumPy array. For a list, assign a new value using `array[row][column] = new_value`. For a NumPy array, use `array[row, column] = new_value` for the same effect.
What are some common use cases for 2-dimensional arrays in Python?
Common use cases include representing matrices, storing pixel values in images, handling tabular data, and performing mathematical operations in data science and machine learning applications.
Creating a two-dimensional array in Python can be accomplished using various methods, with the most common approaches being the use of nested lists or leveraging libraries such as NumPy. A nested list is a straightforward way to represent a 2D array, where each element of the outer list is itself a list, thus forming rows and columns. This method is particularly useful for simple applications where performance is not a critical factor.
For more advanced applications, especially those requiring efficient numerical computations, the NumPy library is highly recommended. NumPy provides a powerful array object called ndarray, which is optimized for performance and offers a wide range of functionalities for mathematical operations. Creating a 2D array with NumPy is as simple as using the `numpy.array()` function, which can convert a nested list into a 2D array, allowing for more efficient data manipulation and analysis.
In summary, the choice between using nested lists or NumPy arrays depends on the specific requirements of the task at hand. For basic needs, nested lists suffice, while NumPy is advantageous for performance-intensive applications. Understanding these options enables Python developers to select the most appropriate method for creating and managing two-dimensional arrays in their projects.
Author Profile
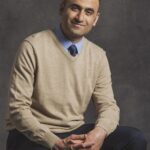
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?