How Can You Create a File in JavaScript? A Step-by-Step Guide
Creating files in JavaScript opens up a world of possibilities for developers, enabling them to manage data dynamically and improve user experiences in web applications. Whether you’re building a simple note-taking app or a complex data management system, understanding how to create files programmatically can enhance your project’s functionality and interactivity. In this article, we’ll explore the various methods available in JavaScript for file creation, delving into both client-side and server-side techniques that cater to different use cases.
At its core, file creation in JavaScript involves generating content and saving it in a format that can be accessed later. On the client side, developers can leverage the capabilities of the File API and Blob objects to create files directly in the browser, allowing users to download or manipulate data seamlessly. Meanwhile, server-side JavaScript, particularly with Node.js, offers robust file system modules that enable developers to create, read, and write files on the server, paving the way for more complex data handling.
As we delve deeper into the topic, we’ll examine practical examples and best practices for file creation in JavaScript. Whether you’re looking to enhance your web applications or streamline server-side processes, mastering file creation techniques is an essential skill that will elevate your programming prowess and open new avenues for innovation.
Creating a File in JavaScript on the Client Side
To create a file in JavaScript on the client side, developers typically leverage the Blob and File APIs. This process is primarily applicable in web browsers where users can download files directly generated by scripts.
A Blob (Binary Large Object) represents data that can be stored in a file-like object. The `Blob` constructor allows you to create a new Blob object, which can then be converted into a downloadable file.
Here’s how you can create a file using JavaScript:
- Create a Blob: Use the Blob constructor to create a new Blob object containing the data you wish to include in the file.
javascript
const data = new Blob([“Hello, world!”], { type: ‘text/plain’ });
- Create a URL for the Blob: Utilize the `URL.createObjectURL` method to create a temporary URL for the Blob object.
javascript
const url = URL.createObjectURL(data);
- Create a link and trigger download: Create an anchor (``) element, set its `href` attribute to the Blob URL, and use the `download` attribute to specify the desired filename.
javascript
const a = document.createElement(‘a’);
a.href = url;
a.download = ‘hello.txt’;
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
- Revoke the Blob URL: Clean up by revoking the Blob URL once the download has been triggered.
javascript
URL.revokeObjectURL(url);
Creating a File in JavaScript on the Server Side
When working in a Node.js environment, file creation is handled using the built-in `fs` (file system) module. This module provides an API for interacting with the file system in a way that is consistent across different operating systems.
To create a file on the server side, follow these steps:
- **Import the fs module:**
javascript
const fs = require(‘fs’);
- **Write to a file:** Use the `fs.writeFile` method to create a new file or overwrite an existing file.
javascript
fs.writeFile(‘example.txt’, ‘Hello, world!’, (err) => {
if (err) throw err;
console.log(‘File has been saved!’);
});
- **Append to a file:** If you want to add data to an existing file instead of overwriting it, use `fs.appendFile`.
javascript
fs.appendFile(‘example.txt’, ‘\nAppending some text.’, (err) => {
if (err) throw err;
console.log(‘Data has been appended!’);
});
Method | Description |
---|---|
`writeFile` | Creates a new file or overwrites an existing file. |
`appendFile` | Adds data to the end of an existing file. |
By following these methods, developers can effectively create files in both client-side and server-side JavaScript environments, allowing for flexibility in how data is managed and stored.
Creating a File in JavaScript in the Browser
In modern web applications, you can create files in the user’s browser using the Blob API and the URL.createObjectURL method. This allows developers to generate files on the client side without needing server-side interaction.
To create a file in the browser, follow these steps:
- Generate Content: Prepare the content you want to save.
- Create a Blob: Use the Blob constructor to create a file-like object.
- Create a Download Link: Use the URL.createObjectURL method to create a link to the Blob.
- Initiate Download: Programmatically create an anchor tag and trigger a click event.
Here’s a sample code snippet demonstrating these steps:
javascript
function createFile(content, fileName) {
// Step 1: Create a Blob object
const blob = new Blob([content], { type: ‘text/plain’ });
// Step 2: Create a URL for the Blob
const url = URL.createObjectURL(blob);
// Step 3: Create an anchor element
const a = document.createElement(‘a’);
a.href = url;
a.download = fileName;
// Step 4: Append to the body and trigger a click
document.body.appendChild(a);
a.click();
// Clean up: Remove the anchor from the document and revoke the URL
document.body.removeChild(a);
URL.revokeObjectURL(url);
}
// Usage
createFile(‘Hello, World!’, ‘hello.txt’);
Creating a File in Node.js
In Node.js, file creation is straightforward using the built-in `fs` (File System) module. This module provides methods to interact with the file system, allowing you to create, read, and write files easily.
To create a file in Node.js, follow these steps:
- **Require the fs Module**: Include the File System module in your script.
- **Use Write Methods**: Use methods like `fs.writeFile` or `fs.appendFile`.
Here’s an example using `fs.writeFile`:
javascript
const fs = require(‘fs’);
const content = ‘Hello, World!’;
const fileName = ‘hello.txt’;
// Step 1: Create or overwrite a file
fs.writeFile(fileName, content, (err) => {
if (err) {
console.error(‘Error creating file:’, err);
return;
}
console.log(‘File created successfully!’);
});
File Creation Options
When creating files, various options can be configured based on the context (browser or Node.js). Here’s a comparison of methods:
Context | Method | Description |
---|---|---|
Browser | `Blob` + `URL.createObjectURL` | Creates a file-like object in memory. |
Node.js | `fs.writeFile` | Writes data to a file, creating it if it doesn’t exist. |
Node.js | `fs.appendFile` | Appends data to a file, creating it if it doesn’t exist. |
This distinction is important for selecting the appropriate approach based on your application’s environment and requirements.
Handling Errors
When working with file creation, error handling is crucial, especially in Node.js. Always check for errors in your callbacks or use try-catch blocks with promises.
Example with error handling in Node.js:
javascript
const fs = require(‘fs’);
const content = ‘Hello, World!’;
const fileName = ‘hello.txt’;
fs.writeFile(fileName, content, (err) => {
if (err) {
console.error(‘Error:’, err.message);
} else {
console.log(‘File created successfully!’);
}
});
This ensures that any issues encountered during file creation are captured and can be addressed accordingly.
Expert Insights on Creating Files in JavaScript
Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Creating files in JavaScript can be efficiently achieved using the File System API in Node.js. This allows developers to read, write, and manipulate files directly on the server side, providing a seamless experience for file management.”
Michael Thompson (JavaScript Developer Advocate, Tech Innovations). “For front-end applications, leveraging the Blob and URL.createObjectURL methods is essential. This approach enables users to generate files dynamically in the browser, enhancing user interactivity without requiring server-side processing.”
Sarah Lee (Full Stack Developer, Web Solutions Inc.). “When working with file creation in JavaScript, it is crucial to consider the environment. While Node.js provides robust file handling capabilities, browser-based solutions require a different approach, such as utilizing the File API and ensuring compatibility across various browsers.”
Frequently Asked Questions (FAQs)
How can I create a text file using JavaScript?
You can create a text file in JavaScript by utilizing the Blob API. First, create a Blob object with the desired content, then use the URL.createObjectURL method to generate a URL for the file, and finally create an anchor element to trigger the download.
Is it possible to create files on the server using JavaScript?
JavaScript running in the browser cannot create files directly on the server. You need to use server-side technologies like Node.js, where you can use the `fs` module to create and manipulate files on the server.
Can I create a file in JavaScript without user interaction?
No, due to security restrictions, browsers require user interaction (like clicking a button) to initiate file downloads. This is to prevent unauthorized file creation or downloads.
What is the role of the FileSaver.js library in file creation?
FileSaver.js is a popular library that simplifies the process of saving files on the client side. It abstracts the Blob API and provides a straightforward API for creating files and triggering downloads with minimal code.
How do I create a CSV file in JavaScript?
To create a CSV file, format your data as a string with comma-separated values, create a Blob from that string, and then use the same method as mentioned above to generate a download link for the user.
Can I create files in a web application using the File System Access API?
Yes, the File System Access API allows web applications to read and write files directly to the user’s file system with their permission. This API provides a more seamless experience for file manipulation compared to traditional methods.
Creating a file in JavaScript can be achieved through various methods depending on the environment in which the JavaScript code is executed. In a web browser, the File API and Blob objects are commonly used to generate files dynamically. This allows developers to create text files, images, and other types of files directly from the browser, which can then be downloaded by users. The process typically involves creating a Blob from the data, generating a URL for it, and then triggering a download action.
In a Node.js environment, the process of creating files is straightforward, as it provides built-in modules like ‘fs’ (file system) that allow for file manipulation. Developers can use methods such as ‘fs.writeFile’ or ‘fs.appendFile’ to create and write to files on the server. This capability is essential for server-side applications that require data persistence, such as logging or storing user-generated content.
Overall, understanding how to create files in JavaScript is crucial for both client-side and server-side development. It enables developers to enhance user experiences by allowing file downloads in the browser and managing data effectively on the server. By leveraging the appropriate APIs and modules, developers can implement file creation seamlessly in their applications.
Author Profile
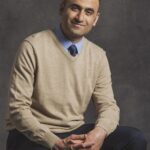
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?