How Can You Easily Create a Script in Python?
Creating a script in Python opens the door to a world of automation, data manipulation, and creative problem-solving. Whether you’re a seasoned programmer or a curious beginner, Python’s simplicity and versatility make it an ideal choice for scripting. With its clear syntax and powerful libraries, you can quickly transform your ideas into functional code that can perform a wide range of tasks, from automating mundane processes to analyzing complex datasets. In this article, we’ll guide you through the essentials of crafting your own Python scripts, equipping you with the knowledge to unleash your programming potential.
At its core, a Python script is a collection of commands that the Python interpreter executes in sequence. This allows you to automate repetitive tasks, manage files, or even build applications that can run on various platforms. Understanding how to create a script involves familiarizing yourself with Python’s syntax, data structures, and control flow, which all play a crucial role in how your code operates. As you delve deeper, you’ll discover how to leverage Python’s extensive libraries to enhance your scripts, making them more efficient and powerful.
Whether you’re looking to streamline your workflow, analyze data, or simply explore the world of programming, learning how to create a script in Python is an invaluable skill. With the right guidance and practice, you’ll be able to write
Understanding Python Scripts
A Python script is a file containing Python code that can be executed directly by the Python interpreter. This allows developers to automate tasks, manipulate data, or develop applications. Scripts are typically saved with a `.py` extension and can be run from the command line or within an integrated development environment (IDE).
To create a basic Python script, follow these essential steps:
- Choose a text editor or an IDE. Popular options include:
- Visual Studio Code
- PyCharm
- Jupyter Notebook
- Sublime Text
- Write your Python code in the chosen editor.
Here is a simple example of a Python script:
“`python
This is a sample Python script
print(“Hello, World!”)
“`
To save the script, use a descriptive filename, such as `hello_world.py`.
Executing Your Python Script
Once the script is saved, you can execute it using the command line or terminal. To run your script, navigate to the directory where the script is saved and use the following command:
“`bash
python hello_world.py
“`
This command invokes the Python interpreter to execute the script. If you’re using Python 3, you might need to use `python3` instead.
Basic Structure of a Python Script
A Python script can include various components:
- Comments: Lines that are not executed, used for documentation.
- Variables: Containers for storing data values.
- Functions: Blocks of reusable code that perform specific tasks.
- Control Structures: Conditional statements (if-else) and loops (for, while) that control the flow of the script.
Here is a simple table outlining these components:
Component | Description |
---|---|
Comments | Used to explain code; starts with “ |
Variables | Store data values; e.g., `x = 5` |
Functions | Reusable code blocks; defined using `def` |
Control Structures | Manage script flow; includes `if`, `for`, and `while` |
Best Practices for Writing Python Scripts
Adhering to best practices enhances the readability and maintainability of your scripts. Consider the following recommendations:
- Use meaningful variable and function names that convey their purpose.
- Keep your code DRY (Don’t Repeat Yourself) by avoiding redundancy.
- Organize your code into functions to improve clarity and modularity.
- Comment your code adequately to explain complex logic.
- Follow PEP 8, the Python style guide, to ensure consistency.
By following these steps and best practices, you can create effective and efficient Python scripts that are easy to read and maintain.
Understanding the Basics of Python Scripts
A Python script is a file containing Python code that can be executed to perform tasks or solve problems. Python scripts typically end with the `.py` extension. The core components of a Python script include:
- Variables: Used to store data values.
- Data Types: Such as integers, floats, strings, and lists.
- Functions: Reusable pieces of code that perform specific tasks.
- Control Structures: Such as loops and conditionals to control the flow of execution.
Setting Up Your Environment
Before you start creating a Python script, ensure that you have the necessary environment set up:
- Install Python: Download and install the latest version of Python from the official website (https://www.python.org/downloads/).
- Choose an IDE or Text Editor: Popular options include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Sublime Text
- Verify the Installation: Open a command prompt or terminal and type:
“`bash
python –version
“`
This command should return the installed version of Python.
Creating Your First Python Script
To create a simple Python script, follow these steps:
- Open your chosen IDE or text editor.
- Create a new file and name it `hello.py`.
- Write the following code in the file:
“`python
print(“Hello, World!”)
“`
- Save the file.
To run the script, open your command prompt or terminal, navigate to the directory where the file is saved, and execute:
“`bash
python hello.py
“`
Adding Functionality to Your Script
Once you have a basic script, you can enhance its functionality. Below are common operations you can include:
- Input and Output: Use the `input()` function to get user input.
“`python
name = input(“Enter your name: “)
print(f”Hello, {name}!”)
“`
- Conditionals: Control the flow using `if`, `elif`, and `else`.
“`python
age = int(input(“Enter your age: “))
if age < 18:
print("You are a minor.")
else:
print("You are an adult.")
```
- Loops: Automate repetitive tasks using `for` or `while` loops.
“`python
for i in range(5):
print(i)
“`
- Functions: Define functions to encapsulate reusable code.
“`python
def greet(name):
return f”Hello, {name}!”
print(greet(“Alice”))
“`
Debugging and Error Handling
Errors can occur in scripts, and handling them effectively is crucial. Common error types include syntax errors and runtime errors. Use `try` and `except` blocks to manage exceptions:
“`python
try:
number = int(input(“Enter a number: “))
print(10 / number)
except ValueError:
print(“Please enter a valid number.”)
except ZeroDivisionError:
print(“Division by zero is not allowed.”)
“`
Organizing Your Script
For larger scripts, organization is key. Follow these best practices:
- Comments: Use “ to add comments explaining code functionality.
- Function Definitions: Group related functions together.
- Module Imports: Keep all imports at the top of the script.
- Structure: Follow a logical flow, starting with imports, then function definitions, followed by the main execution code.
“`python
import math
def calculate_area(radius):
return math.pi * radius ** 2
if __name__ == “__main__”:
r = float(input(“Enter radius: “))
print(f”Area: {calculate_area(r)}”)
“`
Executing and Sharing Your Script
To share your Python script, consider the following methods:
- Direct Sharing: Share the `.py` file directly.
- Version Control: Use Git and platforms like GitHub to manage and share code.
- Packaging: Create a package if your script has multiple files or dependencies.
This structured approach to creating Python scripts will facilitate the development of effective and efficient code.
Expert Insights on Creating Scripts in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a script in Python begins with understanding the problem you want to solve. Start by defining your objectives clearly, then outline the steps your script needs to take. This structured approach will guide you in writing clean, efficient code.”
Michael Chen (Python Developer, CodeCraft Solutions). “To create a script in Python, one must first ensure that Python is properly installed on your system. Utilize a text editor or an Integrated Development Environment (IDE) to write your code. Familiarize yourself with basic syntax and libraries, which are essential for effective scripting.”
Sarah Patel (Data Scientist, Analytics Hub). “When scripting in Python, always prioritize readability and maintainability. Use comments liberally to explain complex sections of your code, and adhere to the PEP 8 style guide. This practice not only aids in your own understanding but also makes collaboration with others smoother.”
Frequently Asked Questions (FAQs)
How do I start writing a script in Python?
To start writing a script in Python, open a text editor or an Integrated Development Environment (IDE) such as PyCharm or Visual Studio Code. Create a new file with a `.py` extension and begin coding using Python syntax.
What is the basic structure of a Python script?
The basic structure of a Python script includes importing necessary libraries, defining functions, and executing code in a main block. A simple script may start with `import` statements, followed by function definitions, and end with a conditional `if __name__ == “__main__”:` to execute the main functionality.
How can I run my Python script?
You can run your Python script by opening a terminal or command prompt, navigating to the directory where your script is located, and executing the command `python script_name.py`, replacing `script_name.py` with your actual script file name.
What are some common errors to watch for when creating a Python script?
Common errors include syntax errors, indentation errors, and runtime errors. Syntax errors occur when the code does not follow Python’s rules, indentation errors arise from inconsistent spacing, and runtime errors occur during execution due to issues like type mismatches or missing files.
How can I debug my Python script?
To debug a Python script, you can use built-in debugging tools such as `pdb`, print statements to track variable values, or IDE features that allow you to set breakpoints and step through the code line by line to identify issues.
What are best practices for writing Python scripts?
Best practices include writing clear and concise code, using meaningful variable names, adhering to PEP 8 style guidelines, adding comments for clarity, and organizing code into functions or classes for better modularity and reusability.
Creating a script in Python involves several key steps that are essential for both beginners and experienced programmers. First, it is crucial to have a clear understanding of the problem you aim to solve with your script. This involves defining the objectives and the functionality required. Once the objectives are established, you can begin writing the code using a text editor or an Integrated Development Environment (IDE) that supports Python.
Next, structuring your script is important for readability and maintainability. This includes organizing your code into functions and classes where applicable, which helps in breaking down complex tasks into manageable segments. Additionally, incorporating comments and documentation within your script enhances its clarity, making it easier for others (and yourself) to understand the logic behind your code in the future.
Testing and debugging your script are critical steps that should not be overlooked. By running your script and checking for errors, you can ensure that it performs as intended. Utilizing Python’s built-in debugging tools and writing unit tests can significantly aid in identifying and resolving issues. Finally, once your script is functioning correctly, consider sharing it through version control systems like Git, which facilitates collaboration and version management.
In summary, creating a Python script requires a systematic approach that includes understanding the problem
Author Profile
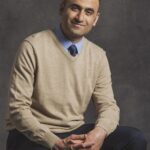
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?