How Can You Create an Engaging Slideshow Using JavaScript?
Creating a slideshow in JavaScript is an exciting way to enhance your web projects, allowing you to present images, text, and other content in a dynamic and visually appealing manner. Whether you’re looking to showcase a portfolio, share memorable moments from a recent event, or simply display information in a more engaging format, a well-crafted slideshow can make all the difference. In this article, we will explore the fundamentals of building a slideshow using JavaScript, guiding you through the essential concepts and techniques that will bring your ideas to life.
At its core, a slideshow is a sequence of images or content that transitions smoothly from one to the next, often accompanied by navigation controls for user interaction. JavaScript plays a crucial role in this process, enabling developers to manipulate the Document Object Model (DOM) and create animations that enhance the user experience. By leveraging JavaScript’s capabilities, you can implement features such as automatic transitions, manual controls, and even responsive designs that adapt to various screen sizes.
In this article, we will delve into the various components involved in creating a slideshow, including HTML structure, CSS styling, and the JavaScript functions that tie everything together. You’ll learn how to set up the basic framework, incorporate essential features, and customize your slideshow to match your unique style. Whether you’re a
Setting Up the HTML Structure
To create a slideshow in JavaScript, you first need to establish a solid HTML foundation. The structure typically consists of a container for the slides, individual slide elements, and navigation controls. Here’s a simple example of how you can set up your HTML:
In this structure:
- The `
- Each slide is contained within a `
`, where the `fade` class can be used for transition effects.
- Navigation arrows are implemented using anchor tags with onclick events to control the slideshow.
Styling the Slideshow
CSS plays a crucial role in enhancing the visual appeal and functionality of your slideshow. Below is a basic CSS snippet to style the slideshow:
css
.slideshow-container {
position: relative;
max-width: 1000px;
margin: auto;
}
.mySlides {
display: none; /* Hide all slides by default */
}
img {
width: 100%; /* Make images responsive */
}
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
}
This CSS ensures that:
- The slideshow is centered and responsive.
- Slides are hidden initially, and images take the full width of their container.
- Navigation buttons are styled for better visibility and usability.
Implementing the JavaScript Functionality
With the HTML and CSS set, it’s time to add the JavaScript that will control the slideshow’s behavior. Below is a simple implementation:
javascript
let slideIndex = 1;
showSlides(slideIndex);
function plusSlides(n) {
showSlides(slideIndex += n);
}
function showSlides(n) {
let i;
let slides = document.getElementsByClassName(“mySlides”);
if (n > slides.length) { slideIndex = 1 }
if (n < 1) { slideIndex = slides.length }
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
slides[slideIndex - 1].style.display = "block";
}
In this JavaScript code:
- `slideIndex` keeps track of the current slide.
- `plusSlides(n)` adjusts the slide index based on user interaction.
- `showSlides(n)` displays the appropriate slide while hiding others.
Enhancing with Automatic Slide Transition
To make the slideshow more dynamic, you can add an automatic transition feature. This can be achieved with the following modification to your JavaScript:
javascript
let slideInterval = setInterval(() => plusSlides(1), 3000); // Change slide every 3 seconds
This code snippet ensures that slides automatically transition every three seconds, enhancing user experience without requiring manual input.
Table of Key Functionality
Function | Description |
---|---|
plusSlides(n) | Increases or decreases the slide index. |
showSlides(n) | Displays the current slide based on the slide index. |
Implementing these components will create a fully functional and visually appealing slideshow using JavaScript.
Creating a Basic Slideshow
To create a basic slideshow in JavaScript, you will need to structure your HTML, apply CSS for styling, and implement JavaScript for functionality. Below is a simple example demonstrating these components.
HTML Structure
The first step is to set up the HTML structure for the slideshow. This typically involves a container for the images and navigation controls.
CSS Styling
The next step involves styling the slideshow to ensure it appears visually appealing. Below is a basic CSS example:
css
.slideshow-container {
position: relative;
max-width: 1000px;
margin: auto;
}
.mySlides {
display: none;
}
img {
width: 100%;
}
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
user-select: none;
}
JavaScript Functionality
To add functionality to the slideshow, implement the following JavaScript code. This will control the slide transitions and navigation.
javascript
let slideIndex = 0;
showSlides();
function showSlides() {
let i;
const slides = document.getElementsByClassName(“mySlides”);
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
slideIndex++;
if (slideIndex > slides.length) {slideIndex = 1}
slides[slideIndex – 1].style.display = “block”;
setTimeout(showSlides, 2000); // Change image every 2 seconds
}
function plusSlides(n) {
slideIndex += n;
if (slideIndex > slides.length) {slideIndex = 1}
if (slideIndex < 1) {slideIndex = slides.length}
showSlides();
}
Explanation of the Code
- HTML:
- Each image is wrapped in a `div` with the class `mySlides`.
- Navigation arrows are included to allow users to move through the slides.
- CSS:
- The slideshow container is centered, and images are set to be responsive.
- Navigation arrows are styled and positioned.
- JavaScript:
- The `showSlides` function hides all slides and displays the current slide based on the `slideIndex`.
- The `plusSlides` function adjusts the index based on user input from navigation arrows.
Enhancements
To improve the slideshow experience, consider the following enhancements:
- Indicators: Add dots at the bottom to indicate the current slide.
- Autoplay Control: Allow users to start or pause the slideshow.
- Keyboard Navigation: Enable arrow key navigation for accessibility.
Each enhancement can be integrated into the existing code structure with minor adjustments.
Expert Insights on Creating a Slideshow in JavaScript
Jessica Lin (Senior Front-End Developer, Tech Innovations Inc.). “Creating a slideshow in JavaScript requires a solid understanding of the Document Object Model (DOM). I recommend using event listeners to manage transitions and ensuring that your code is modular for easier maintenance and scalability.”
Michael Chen (JavaScript Framework Specialist, CodeCraft Academy). “Utilizing libraries like jQuery can simplify the process of creating a slideshow, but understanding the underlying JavaScript principles is crucial. This knowledge allows developers to customize functionalities beyond what libraries offer.”
Sarah Patel (UX/UI Designer, Creative Solutions Group). “When designing a slideshow, consider the user experience. Implementing smooth animations and responsive design principles ensures that the slideshow is engaging across all devices. JavaScript’s CSS manipulation capabilities are invaluable in this regard.”
Frequently Asked Questions (FAQs)
What are the basic components needed to create a slideshow in JavaScript?
To create a slideshow in JavaScript, you need HTML for the structure, CSS for styling, and JavaScript for functionality. The HTML will contain the images and navigation elements, CSS will handle the layout and transitions, and JavaScript will manage the logic for displaying images and user interactions.
How can I implement automatic transitions in a JavaScript slideshow?
Automatic transitions can be implemented using the `setInterval` function in JavaScript. This function repeatedly calls a function that changes the currently displayed image at specified intervals, creating an automatic slideshow effect.
What event listeners should be used for user navigation in a slideshow?
You should use event listeners for click events on navigation buttons, such as “Next” and “Previous.” This allows users to manually navigate through the slideshow by changing the displayed image based on their interactions.
How do I handle image loading in a JavaScript slideshow?
To handle image loading, you can preload images by creating new `Image` objects in JavaScript. This ensures that images are loaded before being displayed, improving the user experience by preventing delays during transitions.
What libraries can simplify the process of creating a slideshow in JavaScript?
Several libraries can simplify slideshow creation, including jQuery, Swiper, and Slick. These libraries provide pre-built components and features, allowing developers to implement complex slideshows with minimal code.
How can I add captions to images in a JavaScript slideshow?
To add captions, include a caption element within the HTML structure of each slide. Use JavaScript to update the caption text dynamically based on the currently displayed image, ensuring that captions correspond to the correct images.
Creating a slideshow in JavaScript involves several key steps, including setting up the HTML structure, styling with CSS, and implementing the functionality through JavaScript. The basic structure typically consists of a container for the slides, individual slide elements, and navigation controls such as next and previous buttons. Properly organizing these components ensures that the slideshow operates smoothly and is visually appealing.
In terms of functionality, JavaScript plays a crucial role in managing the display of slides. This includes writing functions to handle slide transitions, timing for automatic slideshows, and event listeners for user interactions. A common approach is to use an array to store the slide images or content, enabling easy access and manipulation as the user navigates through the slideshow. Additionally, employing CSS transitions can enhance the visual experience by providing smooth animations between slides.
Key takeaways from the discussion on creating a slideshow in JavaScript include the importance of a well-structured HTML layout, the necessity of clear and efficient JavaScript code for functionality, and the role of CSS in enhancing user experience. By following best practices in these areas, developers can create dynamic and responsive slideshows that improve engagement on their websites. Ultimately, a well-executed slideshow can serve as an effective tool for showcasing
Author Profile
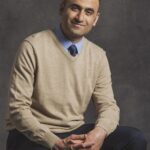
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?