How Can You Create an In-Memory Cache in GoLang?
In the fast-paced world of software development, performance optimization is a critical factor that can make or break an application. One effective strategy that has gained traction among developers is the use of in-memory caching. By temporarily storing frequently accessed data in memory, applications can significantly reduce latency and improve response times, ultimately leading to a smoother user experience. In the Go programming language, or Golang, creating an in-memory cache is not only straightforward but also highly efficient, making it an appealing choice for developers looking to enhance their applications.
In-memory caching in Golang allows developers to leverage the speed of RAM to store and retrieve data quickly, bypassing the need for slower disk I/O operations. This technique is particularly beneficial for applications that require rapid access to data, such as web servers or microservices. By understanding the fundamental principles of caching and how to implement them in Go, developers can build robust applications that handle increased loads with ease.
As we delve deeper into the mechanics of creating an in-memory cache in Golang, we will explore various strategies and best practices that will empower you to harness the full potential of caching. Whether you are building a simple application or a complex system, mastering in-memory caching can provide a significant boost to your application’s performance and scalability. Get ready
Understanding In-Memory Caching
In-memory caching is a technique used to store data temporarily in a fast-access memory location to enhance the performance of applications. By keeping frequently accessed data in memory, applications can avoid the overhead of fetching data from slower storage solutions, such as disk drives or remote databases. In Go, implementing an in-memory cache can be done effectively using various data structures, particularly maps combined with synchronization mechanisms to ensure thread safety.
Implementing a Simple In-Memory Cache
To create a basic in-memory cache in Go, one can utilize a map to store the data, along with a mutex for concurrent access. Below is a simple implementation:
“`go
package main
import (
“sync”
“time”
)
// Cache struct represents the in-memory cache
type Cache struct {
items map[string]interface{}
mu sync.RWMutex
}
// NewCache creates a new instance of Cache
func NewCache() *Cache {
return &Cache{
items: make(map[string]interface{}),
}
}
// Set adds an item to the cache
func (c *Cache) Set(key string, value interface{}) {
c.mu.Lock()
defer c.mu.Unlock()
c.items[key] = value
}
// Get retrieves an item from the cache
func (c *Cache) Get(key string) (interface{}, bool) {
c.mu.RLock()
defer c.mu.RUnlock()
value, exists := c.items[key]
return value, exists
}
// Delete removes an item from the cache
func (c *Cache) Delete(key string) {
c.mu.Lock()
defer c.mu.Unlock()
delete(c.items, key)
}
“`
In this implementation, we define a `Cache` struct that holds a map for storing items and a mutex for managing concurrent access. The `Set`, `Get`, and `Delete` methods allow for basic operations on the cache.
Adding Expiration to Cache Entries
To enhance the cache functionality, adding expiration to cache entries can be beneficial. This ensures that stale data is removed after a specified duration. Here’s how to implement expiration:
“`go
type CacheItem struct {
Value interface{}
Expiration int64
}
func (c *Cache) SetWithExpiration(key string, value interface{}, duration time.Duration) {
c.mu.Lock()
defer c.mu.Unlock()
c.items[key] = CacheItem{
Value: value,
Expiration: time.Now().Add(duration).Unix(),
}
}
func (c *Cache) GetWithExpiration(key string) (interface{}, bool) {
c.mu.RLock()
defer c.mu.RUnlock()
item, exists := c.items[key].(CacheItem)
if !exists || time.Now().Unix() > item.Expiration {
return nil,
}
return item.Value, true
}
“`
In this code, a `CacheItem` struct is introduced, holding both the value and its expiration time. The `SetWithExpiration` and `GetWithExpiration` methods manage the addition and retrieval of items with expiration logic.
Performance Considerations
When implementing an in-memory cache, consider the following performance aspects:
- Concurrency: Use proper synchronization mechanisms (like `sync.RWMutex`) to handle concurrent access.
- Memory Usage: Monitor memory consumption to avoid excessive memory usage due to large datasets.
- Cache Size: Implement a size limit and eviction strategy (e.g., LRU) to remove the least recently used items when the cache reaches its capacity.
Cache Eviction Strategies
Implementing an eviction strategy can help maintain optimal cache performance. Below is a table summarizing common cache eviction strategies:
Strategy | Description |
---|---|
LRU (Least Recently Used) | Evicts the least recently accessed items first. |
LFU (Least Frequently Used) | Evicts items that are used the least often. |
FIFO (First In, First Out) | Evicts items in the order they were added. |
By considering these strategies and implementing them appropriately, you can significantly enhance the performance and efficiency of your in-memory cache in Go.
Creating an In-Memory Cache in Golang
To implement an in-memory cache in Go, you can use various data structures and approaches. The following outlines a simple yet effective way to create your own cache using a map and synchronization primitives.
Basic Cache Implementation
The simplest form of an in-memory cache can be achieved using a Go map to store key-value pairs. To ensure thread safety, you should utilize a `sync.RWMutex`. Below is an example of a basic cache structure:
“`go
package main
import (
“sync”
“time”
)
type Cache struct {
items map[string]cacheItem
mu sync.RWMutex
}
type cacheItem struct {
value interface{}
expiration time.Time
}
func NewCache() *Cache {
return &Cache{
items: make(map[string]cacheItem),
}
}
“`
Setting Values in the Cache
To store values in the cache, you can create a method that accepts a key, value, and an expiration duration. The following method demonstrates how to do this:
“`go
func (c *Cache) Set(key string, value interface{}, duration time.Duration) {
c.mu.Lock()
defer c.mu.Unlock()
c.items[key] = cacheItem{
value: value,
expiration: time.Now().Add(duration),
}
}
“`
Getting Values from the Cache
Retrieving values requires checking if a key exists and if the item has expired. Implement the following method:
“`go
func (c *Cache) Get(key string) (interface{}, bool) {
c.mu.RLock()
defer c.mu.RUnlock()
item, found := c.items[key]
if !found || time.Now().After(item.expiration) {
return nil,
}
return item.value, true
}
“`
Deleting Cache Entries
You may also want to remove entries from the cache. The following method allows you to delete a key:
“`go
func (c *Cache) Delete(key string) {
c.mu.Lock()
defer c.mu.Unlock()
delete(c.items, key)
}
“`
Cache Cleanup
It’s essential to implement a mechanism for cleaning up expired items from the cache. One strategy is to periodically run a cleanup function:
“`go
func (c *Cache) Cleanup() {
c.mu.Lock()
defer c.mu.Unlock()
for key, item := range c.items {
if time.Now().After(item.expiration) {
delete(c.items, key)
}
}
}
“`
Usage Example
Here’s how you can use the cache in a sample application:
“`go
func main() {
cache := NewCache()
cache.Set(“foo”, “bar”, 5*time.Second)
value, found := cache.Get(“foo”)
if found {
fmt.Println(“Found:”, value)
}
time.Sleep(6 * time.Second)
_, found = cache.Get(“foo”)
if !found {
fmt.Println(“Value expired.”)
}
}
“`
This example demonstrates creating a cache, adding an item, retrieving it, and handling expiration correctly. Adjust the cache’s behavior as needed based on your application requirements.
Expert Insights on Creating In-Memory Cache in Golang
Dr. Emily Carter (Senior Software Engineer, Cloud Solutions Inc.). “When implementing an in-memory cache in Golang, it is crucial to leverage the built-in `sync` package for safe concurrent access. This ensures that your cache can handle multiple goroutines without running into race conditions, thus maintaining data integrity.”
Mark Thompson (Lead Developer, GoLang Innovations). “Utilizing Go’s native data structures, such as maps, is a straightforward approach to creating an in-memory cache. However, for larger applications, consider implementing a more sophisticated caching strategy, like LRU (Least Recently Used) eviction, to optimize memory usage and performance.”
Sara Kim (Technical Architect, Software Development Group). “Monitoring cache performance is essential. Integrating metrics collection within your in-memory cache implementation allows you to analyze hit rates and latency, which can guide further optimizations and ensure that your caching strategy aligns with application requirements.”
Frequently Asked Questions (FAQs)
What is an in-memory cache in Golang?
An in-memory cache in Golang is a storage mechanism that keeps data in the system’s RAM for quick access, significantly improving the performance of applications by reducing the need to fetch data from slower persistent storage.
How can I implement a simple in-memory cache in Golang?
To implement a simple in-memory cache in Golang, use a map to store key-value pairs and synchronize access using the `sync` package to avoid race conditions. You can define methods to set, get, and delete items from the cache.
What libraries are available for creating an in-memory cache in Golang?
Several libraries are available, including `groupcache`, `bigcache`, and `go-cache`. These libraries provide additional features such as expiration, eviction policies, and concurrency support.
How do I handle cache expiration in Golang?
To handle cache expiration, you can store the expiration time along with the value in the cache and check this timestamp when retrieving items. If the current time exceeds the expiration time, the item should be considered expired and removed from the cache.
Is it safe to use a map for caching in a concurrent environment?
Using a map directly in a concurrent environment is not safe due to potential race conditions. It is advisable to use synchronization mechanisms such as `sync.Mutex` or `sync.RWMutex` to ensure safe concurrent access.
Can I use an in-memory cache for distributed applications?
An in-memory cache is typically not suitable for distributed applications, as each instance would have its own cache. For distributed caching, consider using solutions like Redis or Memcached that can be accessed by multiple instances across different servers.
Creating an in-memory cache in Golang is a practical approach to enhance application performance by reducing the need for repeated data retrieval from slower storage systems. The implementation typically involves using data structures such as maps or specialized libraries that provide caching mechanisms. By leveraging these tools, developers can store frequently accessed data in memory, which significantly decreases latency and improves response times for applications.
Key considerations when designing an in-memory cache include determining the appropriate cache size, implementing eviction policies, and ensuring thread safety. Developers often choose between various eviction strategies, such as Least Recently Used (LRU) or Time-To-Live (TTL), to manage cache entries effectively. Additionally, utilizing synchronization techniques, such as mutexes or channels, is essential for maintaining data integrity in concurrent environments.
In summary, an in-memory cache in Golang can be efficiently created using native data structures or third-party libraries. By carefully considering cache management strategies and concurrency control, developers can build robust caching solutions that significantly enhance application performance. This approach not only optimizes resource utilization but also improves user experience by delivering faster data access.
Author Profile
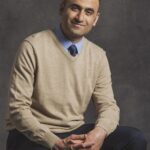
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?