How Can You Create a Dynamic Menu in Python? A Step-by-Step Guide!
Creating a menu in Python can be an exciting venture for both novice and experienced programmers alike. Whether you’re developing a simple command-line application or a more complex graphical user interface, the ability to design a user-friendly menu is crucial for enhancing the overall experience. Menus not only guide users through your application but also help organize functionality in a coherent manner, making it easier for users to navigate and utilize the features you’ve built. In this article, we’ll explore the various methods and techniques to create dynamic and interactive menus in Python, empowering you to elevate your projects to new heights.
At its core, a menu in Python serves as a structured interface that allows users to select options and execute commands. The beauty of Python lies in its versatility; you can create menus using simple text-based prompts in the console or leverage libraries like Tkinter or PyQt for more sophisticated graphical interfaces. Each approach has its own set of advantages, depending on the complexity of your application and the user experience you wish to create.
In addition to the technical aspects of menu creation, it’s essential to consider usability and design principles. A well-organized menu not only improves navigation but also enhances the overall functionality of your application. As we delve deeper into this topic, we will cover practical examples and best practices that will
Creating a Basic Menu in Python
To create a basic menu in Python, you can utilize a combination of functions and control flow statements. A menu typically allows users to select from several options, which can lead to different functionalities within your program. Below is an example of how you can structure a simple text-based menu using functions and a loop.
“`python
def display_menu():
print(“1. Option One”)
print(“2. Option Two”)
print(“3. Exit”)
def option_one():
print(“You selected Option One.”)
def option_two():
print(“You selected Option Two.”)
while True:
display_menu()
choice = input(“Enter your choice: “)
if choice == ‘1’:
option_one()
elif choice == ‘2’:
option_two()
elif choice == ‘3’:
print(“Exiting the program.”)
break
else:
print(“Invalid choice. Please try again.”)
“`
In this example, the `display_menu` function prints the menu options, while the user input determines which option is executed.
Using Dictionaries for Menu Options
A more scalable approach to creating a menu in Python is to use a dictionary to map user choices to functions. This method enhances readability and maintainability of your code. Below is an example:
“`python
def option_one():
print(“You selected Option One.”)
def option_two():
print(“You selected Option Two.”)
def exit_program():
print(“Exiting the program.”)
menu_options = {
‘1’: option_one,
‘2’: option_two,
‘3’: exit_program
}
while True:
print(“\nMenu:”)
for key in menu_options.keys():
print(f”{key}. {menu_options[key].__name__.replace(‘_’, ‘ ‘).title()}”)
choice = input(“Enter your choice: “)
if choice in menu_options:
if choice == ‘3’:
menu_options[choice]()
break
menu_options[choice]()
else:
print(“Invalid choice. Please try again.”)
“`
This structure allows for easy modifications and the addition of new options without altering the control flow significantly.
Advanced Menu with Submenus
For more complex applications, you may need to implement submenus. This can be achieved by nesting functions and maintaining separate dictionaries for each menu. Below is an example of a menu with a submenu:
“`python
def main_menu():
print(“\nMain Menu:”)
print(“1. Go to Submenu”)
print(“2. Exit”)
def submenu():
print(“\nSubmenu:”)
print(“1. Sub Option One”)
print(“2. Sub Option Two”)
print(“3. Back to Main Menu”)
def run_submenu():
while True:
submenu()
choice = input(“Enter your choice: “)
if choice == ‘1’:
print(“You selected Sub Option One.”)
elif choice == ‘2’:
print(“You selected Sub Option Two.”)
elif choice == ‘3’:
break
else:
print(“Invalid choice. Please try again.”)
while True:
main_menu()
choice = input(“Enter your choice: “)
if choice == ‘1’:
run_submenu()
elif choice == ‘2’:
print(“Exiting the program.”)
break
else:
print(“Invalid choice. Please try again.”)
“`
This example demonstrates how to create a submenu that can be accessed from the main menu, enhancing user interaction.
Menu Option | Function |
---|---|
1 | Option One |
2 | Option Two |
3 | Exit Program |
Utilizing these techniques allows for the development of a flexible and user-friendly menu system in Python applications.
Creating a Text-Based Menu in Python
To create a simple text-based menu in Python, you can utilize basic control structures such as loops and conditionals. The following steps outline the process:
- Define Menu Options: Create a list of options that will be displayed to the user.
- Display the Menu: Use a loop to present the menu until the user selects an option to exit.
- Handle User Input: Capture user input and execute corresponding actions based on the selection.
Here’s a sample implementation:
“`python
def display_menu():
print(“Menu:”)
print(“1. Option 1”)
print(“2. Option 2”)
print(“3. Option 3”)
print(“4. Exit”)
def main():
while True:
display_menu()
choice = input(“Please select an option (1-4): “)
if choice == ‘1’:
print(“You selected Option 1.”)
elif choice == ‘2’:
print(“You selected Option 2.”)
elif choice == ‘3’:
print(“You selected Option 3.”)
elif choice == ‘4’:
print(“Exiting the menu.”)
break
else:
print(“Invalid choice. Please try again.”)
if __name__ == “__main__”:
main()
“`
Creating a Graphical User Interface (GUI) Menu
For a more sophisticated menu, you can use libraries such as Tkinter, which is included with Python. Tkinter allows you to create GUI applications with menus.
- Install Tkinter: Usually pre-installed, but ensure it’s available in your Python environment.
- Set Up the Main Window: Initialize the main application window.
- Create Menu Bar: Use the `Menu` widget to create a menu bar and add options.
Example code for a simple Tkinter menu:
“`python
import tkinter as tk
from tkinter import messagebox
def option1():
messagebox.showinfo(“Selection”, “You selected Option 1”)
def option2():
messagebox.showinfo(“Selection”, “You selected Option 2”)
def option3():
messagebox.showinfo(“Selection”, “You selected Option 3”)
app = tk.Tk()
app.title(“Menu Example”)
menu = tk.Menu(app)
app.config(menu=menu)
submenu = tk.Menu(menu)
menu.add_cascade(label=”Options”, menu=submenu)
submenu.add_command(label=”Option 1″, command=option1)
submenu.add_command(label=”Option 2″, command=option2)
submenu.add_command(label=”Option 3″, command=option3)
submenu.add_separator()
submenu.add_command(label=”Exit”, command=app.quit)
app.mainloop()
“`
Creating a Menu with Command-Line Arguments
You can also create a menu that utilizes command-line arguments, allowing users to select options when running the script.
- Import Required Modules: Use `argparse` for handling command-line inputs.
- Define Available Options: Set up the options using the `ArgumentParser`.
Example implementation:
“`python
import argparse
def main(option):
if option == ‘1’:
print(“You selected Option 1.”)
elif option == ‘2’:
print(“You selected Option 2.”)
elif option == ‘3’:
print(“You selected Option 3.”)
else:
print(“Invalid option selected.”)
if __name__ == “__main__”:
parser = argparse.ArgumentParser(description=”Select an option.”)
parser.add_argument(“option”, choices=[‘1’, ‘2’, ‘3’], help=”Choose an option (1, 2, or 3).”)
args = parser.parse_args()
main(args.option)
“`
Menu Design Considerations
When designing menus, consider the following best practices:
- Clarity: Ensure options are clearly labeled.
- Accessibility: Allow users to easily navigate and select options.
- Feedback: Provide immediate feedback upon selection.
- Scalability: Design menus that can be easily expanded as new options are added.
Utilizing these approaches will enhance user interaction with your Python applications.
Expert Insights on Creating Menus in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Creating menus in Python can enhance user interaction significantly. Utilizing libraries like Tkinter or PyQt allows developers to build intuitive graphical user interfaces, making the application more accessible to users who may not be familiar with command-line operations.”
James Liu (Lead Python Instructor, Code Academy). “When designing a menu in Python, it is essential to consider the user experience. A well-structured menu not only improves navigation but also organizes functionality in a logical manner, which can be achieved through clear labeling and grouping of related options.”
Sarah Thompson (Software Architect, Tech Innovations Corp). “For console applications, implementing a text-based menu using simple loops and conditionals can be effective. This approach allows for quick modifications and scalability as the application grows, ensuring that the menu remains relevant to the evolving needs of the user.”
Frequently Asked Questions (FAQs)
How can I create a simple text-based menu in Python?
You can create a simple text-based menu in Python by using a loop and conditional statements. Utilize the `input()` function to capture user choices and `print()` statements to display menu options.
What libraries can assist in creating a more advanced menu in Python?
Libraries such as `curses` for terminal handling or `tkinter` for graphical user interfaces can enhance menu creation. These libraries provide additional features for more interactive and visually appealing menus.
How do I handle user input in a Python menu?
You can handle user input by using the `input()` function, which captures the user’s choice. Implement error handling with `try-except` blocks to manage invalid inputs effectively.
Can I create a menu that calls different functions based on user selection?
Yes, you can define functions for each menu option and call them based on the user’s selection. This modular approach improves code organization and readability.
What is the best way to structure a menu in Python for scalability?
For scalability, structure your menu using functions or classes. This allows for easy modifications and additions of new options without disrupting existing code.
Is it possible to create a menu that runs indefinitely until the user chooses to exit?
Yes, you can implement an infinite loop that continues to display the menu until the user selects an exit option. Use a `while True` loop combined with a break statement to exit the loop when needed.
Creating a menu in Python can be accomplished through various methods, depending on the desired complexity and user interface. For simple command-line applications, using basic input functions to display options and capture user choices is effective. This method allows for straightforward navigation and interaction, making it suitable for beginner projects or scripts.
For more advanced applications, especially those requiring a graphical user interface (GUI), libraries such as Tkinter or PyQt can be utilized. These libraries provide tools to create visually appealing menus that enhance user experience. Developers can design menus with buttons, dropdowns, and other interactive elements, allowing for a more sophisticated and engaging interface.
Overall, the approach to creating a menu in Python should align with the application’s requirements and the target audience. Whether opting for a simple text-based menu or a complex GUI, Python offers the flexibility to implement a wide range of menu designs. Understanding the available tools and libraries is crucial for effectively developing a user-friendly menu system.
Author Profile
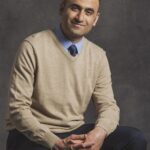
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?