How Can You Create a Python Script from Scratch?
In today’s digital age, the ability to automate tasks and manipulate data using programming is more valuable than ever. Python, with its simplicity and versatility, has emerged as a favorite among beginners and seasoned developers alike. Whether you’re looking to streamline your workflow, analyze data, or even create a game, knowing how to create a Python script can open up a world of possibilities. This article will guide you through the essentials of crafting your very own Python scripts, empowering you to harness the full potential of this powerful programming language.
Creating a Python script is not just about writing code; it’s about problem-solving and bringing your ideas to life. At its core, a Python script is a collection of instructions that the Python interpreter executes sequentially. This means that with just a few lines of code, you can automate repetitive tasks, manipulate files, or even interact with web applications. The beauty of Python lies in its readability and the extensive libraries available, making it accessible for anyone eager to learn.
As you embark on your journey to create a Python script, you’ll discover that the process involves understanding the basic structure of Python code, utilizing functions, and leveraging libraries to extend functionality. From setting up your development environment to writing your first script, each step is a building block toward mastering this dynamic
Setting Up Your Development Environment
To create a Python script, you must first set up your development environment. This involves ensuring that Python is installed on your machine, and optionally, selecting a code editor that suits your workflow.
- Install Python: Download and install the latest version of Python from the official website (https://www.python.org/downloads/). During installation, ensure that you check the box that adds Python to your system PATH.
- Choose a Code Editor: While you can use any text editor, some popular choices for Python development include:
- Visual Studio Code
- PyCharm
- Sublime Text
- Atom
Once you have your editor set up, you’re ready to create your first Python script.
Creating Your First Python Script
To create a Python script, follow these steps:
- Open your code editor.
- Create a new file: Use the `File` menu to create a new file, and save it with a `.py` extension, such as `myscript.py`.
- Write Your Code: Begin coding your script. For example, you can start with a simple print statement:
“`python
print(“Hello, World!”)
“`
- Save the File: Regularly save your changes to avoid losing your work.
Running Your Python Script
To execute your Python script, you can use the command line or terminal. Here’s how:
- Using Command Line:
- Open your command line interface (CLI).
- Navigate to the directory where your script is saved using the `cd` command.
- Run the script by typing:
“`bash
python myscript.py
“`
- Using Integrated Terminal in Code Editors: Many code editors have an integrated terminal. You can run your script directly from there, following the same command as above.
Debugging Your Script
Debugging is an essential part of programming. Here are some common debugging techniques:
- Print Statements: Use print statements to output variable values and track the flow of execution.
- Python Debugger (pdb): You can use Python’s built-in debugger by importing it in your script:
“`python
import pdb; pdb.set_trace()
“`
- Integrated Debugging Tools: Many IDEs provide built-in debugging tools that allow you to set breakpoints and step through your code.
Debugging Technique | Description |
---|---|
Print Statements | Inserting print statements to display variable states at certain points. |
pdb | Using Python’s debugger to inspect variables and control execution. |
IDE Debugger | Utilizing built-in tools to step through code and monitor execution. |
With these tools and techniques, you can effectively create and debug your Python scripts.
Choosing a Development Environment
Selecting the right development environment is crucial for creating Python scripts efficiently. A development environment can range from simple text editors to full-fledged Integrated Development Environments (IDEs).
- Text Editors: Lightweight and quick to use, suitable for small scripts.
- Examples: Notepad++, Sublime Text, Visual Studio Code
- IDEs: Offer advanced features like debugging tools, code completion, and integrated terminal.
- Examples: PyCharm, Jupyter Notebook, Spyder
Setting Up Your Environment
After choosing your development environment, the next step is to set it up properly.
- Install Python: Download the latest version of Python from the official website (python.org) and follow the installation instructions.
- Verify Installation: Open your command line interface and type:
“`bash
python –version
“`
This command should display the installed Python version.
- Install Necessary Packages: Use pip, Python’s package manager, to install additional libraries you may need:
“`bash
pip install package_name
“`
Writing Your First Python Script
Creating a Python script involves writing code in a text file and saving it with a `.py` extension.
- Create a New File: Open your chosen text editor or IDE, and create a new file.
- Write Code: Begin writing your Python code. For example:
“`python
print(“Hello, World!”)
“`
- Save the File: Save the file with a `.py` extension, such as `hello.py`.
Running Your Python Script
To execute your Python script, follow these steps based on your operating system.
- Windows:
- Open Command Prompt.
- Navigate to the script’s directory:
“`bash
cd path\to\your\script
“`
- Run the script:
“`bash
python hello.py
“`
- Mac/Linux:
- Open Terminal.
- Navigate to the script’s directory:
“`bash
cd /path/to/your/script
“`
- Run the script:
“`bash
python3 hello.py
“`
Debugging Your Script
Debugging is an essential part of script development. Here are some common debugging techniques:
- Print Statements: Insert print statements to track variable values and program flow.
- Use a Debugger: Most IDEs come with built-in debuggers that allow you to step through code and inspect variables.
- Error Messages: Pay attention to error messages. They often indicate the line number and nature of the issue.
Enhancing Your Script
As you become more comfortable with Python, consider enhancing your scripts with additional features:
- Functions: Organize your code into reusable blocks.
“`python
def greet(name):
print(f”Hello, {name}!”)
“`
- Modules: Import external libraries to extend functionality.
“`python
import math
print(math.sqrt(16))
“`
- User Input: Make your script interactive by accepting user input.
“`python
name = input(“Enter your name: “)
greet(name)
“`
Best Practices
Adhering to best practices improves code readability and maintainability:
- Comment Your Code: Use comments to explain complex sections of code.
- Follow Naming Conventions: Use descriptive variable and function names (e.g., `calculate_area`).
- Keep Code Organized: Structure your code logically with consistent indentation and spacing.
Resources for Further Learning
Utilize the following resources to deepen your Python knowledge:
Resource Type | Examples |
---|---|
Online Courses | Coursera, Udemy, edX |
Documentation | Official Python docs (docs.python.org) |
Community Forums | Stack Overflow, Reddit (r/learnpython) |
Books | “Automate the Boring Stuff with Python,” “Python Crash Course” |
By following these guidelines, you will be well on your way to creating effective and efficient Python scripts.
Expert Insights on Creating Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To create an effective Python script, one must first define the problem clearly. Understanding the requirements and desired outcomes will guide the structure and logic of your code, ensuring that the script is both efficient and maintainable.”
Michael Tran (Lead Data Scientist, Data Insights Lab). “When beginning to write a Python script, it is crucial to leverage libraries and frameworks that can simplify complex tasks. Utilizing established modules not only speeds up development but also enhances the script’s functionality and reliability.”
Sarah Johnson (Python Instructor, Code Academy). “Testing is an essential part of creating a Python script. Implementing unit tests during the development process can help identify bugs early and ensure that the code behaves as expected, ultimately leading to a more robust final product.”
Frequently Asked Questions (FAQs)
What are the basic steps to create a Python script?
To create a Python script, start by installing Python on your computer. Open a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode. Write your Python code in the editor, then save the file with a `.py` extension. Finally, run the script using the command line or terminal by typing `python filename.py`.
Do I need to install any special software to write Python scripts?
You need to install Python, which includes the interpreter necessary to run your scripts. Additionally, using an IDE or text editor that supports Python, such as PyCharm, VSCode, or even simple editors like Notepad++, can enhance your coding experience.
How can I run a Python script from the command line?
To run a Python script from the command line, open your terminal or command prompt, navigate to the directory where your script is saved using the `cd` command, and then type `python filename.py` or `python3 filename.py`, depending on your Python installation.
What are some common errors encountered when creating Python scripts?
Common errors include syntax errors, indentation errors, and runtime errors. Syntax errors occur when the code violates Python’s grammar rules. Indentation errors arise from incorrect spacing, while runtime errors happen during execution, often due to logic mistakes or invalid operations.
How can I debug a Python script?
You can debug a Python script using print statements to track variable values or by utilizing debugging tools available in IDEs like PyCharm or VSCode. Additionally, Python’s built-in `pdb` module allows for step-by-step execution and inspection of the code.
What resources are available for learning Python scripting?
Numerous resources are available, including online courses on platforms like Coursera and Udemy, official Python documentation, and community forums such as Stack Overflow. Books like “Automate the Boring Stuff with Python” also provide practical examples for beginners.
Creating a Python script involves several essential steps that cater to both beginners and experienced programmers. First, it is crucial to have a clear understanding of the problem you want to solve or the task you wish to automate. This clarity will guide the development process and help in structuring the script effectively. Once the objective is established, the next step is to set up the programming environment, which includes installing Python and any necessary libraries or frameworks that will aid in the script’s functionality.
After setting up the environment, writing the actual code is the next significant phase. This process includes defining functions, utilizing control structures, and implementing error handling to ensure the script runs smoothly. It is also important to follow best practices in coding, such as maintaining readability through proper indentation and commenting, which will facilitate future modifications and debugging. Testing the script thoroughly is vital to identify and resolve any issues before deployment.
Finally, once the script is complete and tested, documenting the code and its usage is essential for both personal reference and for others who may use or modify the script in the future. This documentation can include usage instructions, examples, and explanations of the code’s logic. By following these steps, anyone can create a functional and efficient Python script that meets their specific
Author Profile
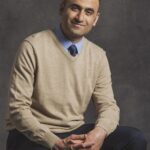
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?