How Can You Easily Create a Table in Python?
Creating tables in Python is a fundamental skill that can greatly enhance your data manipulation and presentation capabilities. Whether you’re working with simple datasets or complex databases, knowing how to structure and display your information in a tabular format is essential. Python offers a variety of libraries and tools that make this process not only straightforward but also highly efficient. From data analysis to web development, the ability to create tables can transform the way you interact with data, making it more accessible and easier to understand.
In this article, we will explore the various methods available for creating tables in Python, catering to different needs and scenarios. You’ll learn about popular libraries such as Pandas, which provides powerful data structures and functions for handling tabular data, as well as other options like PrettyTable and tabulate that focus on displaying data in a visually appealing way. Each method has its own strengths, and understanding these can help you choose the right approach for your project.
Additionally, we will touch on practical applications of table creation, from data analysis tasks to generating reports and visualizations. By the end of this article, you will have a solid foundation in creating tables in Python, empowering you to present your data clearly and effectively. Whether you’re a beginner or looking to sharpen your skills, this guide will equip you with the knowledge
Creating Tables with Pandas
One of the most efficient ways to create and manipulate tables in Python is by using the Pandas library. Pandas provides a powerful DataFrame object that can be thought of as a two-dimensional table, similar to a spreadsheet or SQL table.
To create a DataFrame, you can utilize various methods, including from dictionaries, lists, or even reading from external files such as CSV. Here are some common methods for creating a DataFrame:
- From a dictionary: Each key corresponds to a column in the DataFrame, while the values are lists representing the data for that column.
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
“`
- From a list of lists: You can create a DataFrame by passing a list of lists to the DataFrame constructor and specifying column names.
“`python
data = [[‘Alice’, 25, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]]
df = pd.DataFrame(data, columns=[‘Name’, ‘Age’, ‘City’])
“`
- From CSV files: If your data is stored in a CSV file, you can easily read it into a DataFrame.
“`python
df = pd.read_csv(‘data.csv’)
“`
Creating Tables with PrettyTable
Another library for creating tables in Python is PrettyTable, which is particularly useful for displaying data in a formatted way in the console.
To use PrettyTable, follow these steps:
- Install the PrettyTable library if you haven’t already:
“`bash
pip install prettytable
“`
- Create a table by defining the columns and adding rows:
“`python
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
“`
The output will be a neatly formatted table in the console:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
Creating Tables with Tabulate
The Tabulate library is another excellent choice for creating formatted tables in Python. It allows you to display data in a variety of formats, including plain text, HTML, and LaTeX.
To create a table using Tabulate:
- Install the library:
“`bash
pip install tabulate
“`
- Create and print a table:
“`python
from tabulate import tabulate
data = [[“Alice”, 25, “New York”],
[“Bob”, 30, “Los Angeles”],
[“Charlie”, 35, “Chicago”]]
print(tabulate(data, headers=[“Name”, “Age”, “City”], tablefmt=”grid”))
“`
This will produce the following output:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
By leveraging these libraries, you can create, manipulate, and display tables in Python effectively.
Creating Tables with Pandas
Pandas is one of the most popular libraries for data manipulation and analysis in Python. It provides powerful data structures like DataFrames that can be used to create and manipulate tables easily.
To create a table (DataFrame) in Pandas, follow these steps:
- Install Pandas: If you haven’t already, install the Pandas library using pip.
“`bash
pip install pandas
“`
- Import Pandas: Begin by importing the library in your Python script.
“`python
import pandas as pd
“`
- Create a DataFrame: You can create a DataFrame using various methods, including from dictionaries, lists, or existing data files.
Example using a dictionary:
“`python
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
print(df)
“`
This will output:
“`
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
“`
Creating Tables with SQLite
SQLite provides a lightweight database that allows you to create and manage tables. The following steps illustrate how to create a table using SQLite in Python.
- Install SQLite: SQLite comes pre-installed with Python, but if you need to install it, you can use pip.
“`bash
pip install sqlite3
“`
- Import SQLite: Import the SQLite module in your script.
“`python
import sqlite3
“`
- Connect to a Database: Create a connection to the database. If the database does not exist, it will be created.
“`python
conn = sqlite3.connect(‘example.db’)
cursor = conn.cursor()
“`
- Create a Table: Execute a SQL command to create a table.
“`python
cursor.execute(”’
CREATE TABLE employees (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
age INTEGER,
city TEXT
)
”’)
“`
- Commit Changes and Close: Make sure to commit your changes and close the connection.
“`python
conn.commit()
conn.close()
“`
Creating Tables with PrettyTable
PrettyTable is a Python library used to display tabular data in a visually appealing ASCII table format. Here’s how you can create a table using PrettyTable.
- Install PrettyTable: Use pip to install the library.
“`bash
pip install prettytable
“`
- Import PrettyTable: Import the library in your script.
“`python
from prettytable import PrettyTable
“`
- Create a Table: Define the table and add columns.
“`python
table = PrettyTable()
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
“`
The output will be a neatly formatted table:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
Creating Tables in Jupyter Notebooks
In Jupyter Notebooks, you can create tables using Pandas, or you can display HTML tables directly.
- Using Pandas in Jupyter: Simply create a DataFrame and display it. The notebook will render it as a table.
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
df
“`
- Using HTML: You can create tables in HTML format and render them in Jupyter.
“`python
from IPython.core.display import HTML
html_table = “””
Name | Age | City |
---|---|---|
Alice | 25 | New York |
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
“””
display(HTML(html_table))
“`
Expert Insights on Creating Tables in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Creating tables in Python can be efficiently accomplished using libraries such as Pandas, which provides powerful data manipulation capabilities. By utilizing the DataFrame structure, users can easily create, modify, and analyze tabular data, making it an essential tool for data analysis.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For those looking to create simple tables in Python without external libraries, the built-in `prettytable` module offers a straightforward approach. It allows for the creation of ASCII tables that are easy to read and can be customized according to user preferences.”
Sarah Johnson (Python Developer, Data Insights Group). “When creating tables for web applications, leveraging libraries like Flask or Django in conjunction with HTML tables is crucial. This method not only enhances the presentation of data but also integrates seamlessly with backend databases, ensuring dynamic data handling.”
Frequently Asked Questions (FAQs)
How can I create a simple table using Python?
You can create a simple table in Python using libraries like Pandas or PrettyTable. For example, using Pandas, you can create a DataFrame and display it as a table.
What libraries are commonly used to create tables in Python?
Common libraries include Pandas for data manipulation, PrettyTable for simple ASCII tables, and Matplotlib for visualizing tables as plots.
Can I create a table in Python without using external libraries?
Yes, you can create a table using built-in data structures like lists or dictionaries and format the output manually using string formatting techniques.
How do I display a table in a Jupyter Notebook?
In a Jupyter Notebook, you can display a Pandas DataFrame directly, and it will render as a table. Use the `display()` function from IPython.display for enhanced formatting.
Is it possible to export a table created in Python to a file?
Yes, you can export tables created with Pandas to various file formats, including CSV, Excel, and HTML, using the `to_csv()`, `to_excel()`, or `to_html()` methods.
Can I style tables created in Python?
Yes, libraries like Pandas provide options for styling DataFrames. Additionally, you can use libraries like Plotly or Dash for interactive and visually appealing table presentations.
Creating a table in Python can be accomplished using various libraries, each offering unique features and functionalities suited to different needs. The most common libraries for table creation include Pandas, PrettyTable, and tabulate. Pandas is particularly powerful for data manipulation and analysis, allowing users to create DataFrames that can be easily converted into tables. PrettyTable is ideal for generating simple ASCII tables, while tabulate provides a flexible way to format tables in various styles.
When using Pandas, one can create a table by defining a DataFrame with structured data, which can then be displayed in a tabular format. PrettyTable allows for the addition of rows and columns dynamically, making it suitable for quick table generation. Tabulate, on the other hand, is useful for converting lists and dictionaries into well-formatted tables, enhancing the presentation of data in console applications.
In summary, the choice of library depends on the specific requirements of the project. For data analysis and manipulation, Pandas is the go-to option, while PrettyTable and tabulate are excellent for simpler, more visually appealing outputs. Understanding the strengths of each library will enable users to create effective tables tailored to their needs in Python.
Author Profile
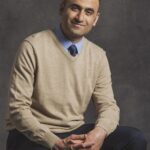
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?