How Can You Create a Website Using Python?
Creating a website can seem like a daunting task, especially for those who are new to programming or web development. However, with the rise of Python as a versatile and powerful language, building a website has never been more accessible. Whether you’re looking to launch a personal blog, an e-commerce platform, or a portfolio site, Python offers a range of frameworks and tools that can simplify the process and enhance your development experience. In this article, we will explore the steps and resources needed to create a website using Python, empowering you to bring your online vision to life.
At its core, creating a website with Python involves understanding the fundamentals of web development, including front-end and back-end technologies. Python frameworks such as Flask and Django provide robust structures that streamline the development process, allowing you to focus on functionality and design rather than getting bogged down by the complexities of coding from scratch. By leveraging these frameworks, developers can efficiently manage databases, handle user authentication, and create dynamic web pages that respond to user interactions.
In addition to frameworks, Python’s extensive libraries and community support offer a wealth of resources for aspiring web developers. From integrating APIs to enhancing user experience with interactive elements, Python’s versatility enables you to implement a wide array of features that can elevate your website. As we delve deeper
Frameworks for Web Development
To create a website using Python, choosing the right framework is crucial. Python offers several frameworks that streamline the web development process, each suited for different types of projects. Here are some of the most popular frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It includes an ORM (Object-Relational Mapping), authentication, and admin panel out of the box.
- Flask: A micro-framework that is lightweight and easy to use. It is particularly suitable for smaller applications or services and allows for more flexibility.
- FastAPI: Designed for building APIs quickly with automatic interactive documentation. It is based on standard Python type hints and is one of the fastest frameworks available.
- Pyramid: A flexible framework that can scale from simple to complex applications. It allows developers to choose their components and can be used for both small and large applications.
Setting Up Your Development Environment
Before starting the development process, it is essential to set up your development environment. Here are the steps you should follow:
- Install Python: Ensure you have the latest version of Python installed on your machine. You can download it from the official website.
- Set Up a Virtual Environment: It is good practice to use virtual environments to manage dependencies. You can create a virtual environment by running:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment: Depending on your operating system, use the following commands:
- For Windows:
“`bash
myenv\Scripts\activate
“`
- For macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Required Packages: Use pip to install the necessary packages based on the framework you choose. For example, for Django:
“`bash
pip install django
“`
Creating Your First Web Application
Once your environment is set up, you can start building your web application. Here’s a basic outline using Django as an example:
- Create a New Project:
“`bash
django-admin startproject myproject
“`
- Navigate into the Project Directory:
“`bash
cd myproject
“`
- Run the Development Server:
“`bash
python manage.py runserver
“`
You can now access your application at `http://127.0.0.1:8000/`.
- Create an App within Your Project:
“`bash
python manage.py startapp myapp
“`
- Define Models: Open `models.py` in your app directory and define your data models. Example:
“`python
from django.db import models
class Item(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
“`
- Migrate the Database:
“`bash
python manage.py makemigrations
python manage.py migrate
“`
Basic Routing and Views
Routing is a critical component of web applications. It determines how URLs are mapped to views. Here’s how to set up routing in Django:
- Open `urls.py` and define your URL patterns:
“`python
from django.urls import path
from . import views
urlpatterns = [
path(”, views.index, name=’index’),
]
“`
- Create the corresponding view in `views.py`:
“`python
from django.shortcuts import render
def index(request):
return render(request, ‘index.html’)
“`
Database Integration
Python web applications often require database integration. Django comes with built-in support for various databases. Here’s a simplified table of some common databases and the corresponding Django settings:
Database | Settings Configuration |
---|---|
SQLite |
“`python DATABASES = { ‘default’: { ‘ENGINE’: ‘django.db.backends.sqlite3’, ‘NAME’: BASE_DIR / “db.sqlite3”, } } “` |
PostgreSQL |
“`python DATABASES = { ‘default’: { ‘ENGINE’: ‘django.db.backends.postgresql’, ‘NAME’: ‘mydatabase’, ‘USER’: ‘myuser’, ‘PASSWORD’: ‘mypassword’, ‘HOST’: ‘localhost’, ‘PORT’: ‘5432’, } } “` |
Choosing the Right Framework
When creating a website using Python, selecting an appropriate web framework is crucial for efficiency and scalability. Popular frameworks include:
- Django: A high-level framework that promotes rapid development and clean design. It’s ideal for larger applications.
- Flask: A micro-framework that is lightweight and easy to get started with, suitable for smaller applications and APIs.
- FastAPI: Known for its speed and efficiency, this framework is excellent for building APIs with automatic documentation.
Each framework has its strengths and is suited for different project types. Consider the scale and requirements of your project when choosing.
Setting Up the Development Environment
To create a website using Python, you’ll need to set up your development environment. Follow these steps:
- Install Python: Ensure you have Python installed. Use the command:
“`bash
python –version
“`
If it’s not installed, download it from [python.org](https://www.python.org/downloads/).
- Set Up a Virtual Environment: This helps manage dependencies.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
“`
- Install Framework: Depending on your choice, install the necessary package.
- For Django:
“`bash
pip install django
“`
- For Flask:
“`bash
pip install flask
“`
- For FastAPI:
“`bash
pip install fastapi uvicorn
“`
Creating a Simple Web Application
Once your environment is set up, you can create a simple web application using your chosen framework.
Django Example:
- Create a new project:
“`bash
django-admin startproject myproject
cd myproject
“`
- Start the development server:
“`bash
python manage.py runserver
“`
Flask Example:
- Create a new Python file (e.g., `app.py`) and add:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the application:
“`bash
python app.py
“`
FastAPI Example:
- Create a new Python file (e.g., `main.py`) and add:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
async def read_root():
return {“Hello”: “World”}
“`
- Run the application:
“`bash
uvicorn main:app –reload
“`
Managing Dependencies
Effective dependency management is essential for maintaining your web application. Utilize a `requirements.txt` file to list your dependencies:
- Create the file:
“`bash
pip freeze > requirements.txt
“`
- To install dependencies from the file:
“`bash
pip install -r requirements.txt
“`
This practice helps ensure that your project can be easily set up in different environments or shared with collaborators.
Deploying Your Web Application
Deployment is the final step to make your website accessible. Common platforms for deploying Python applications include:
- Heroku: A platform-as-a-service (PaaS) that supports Python applications.
- AWS Elastic Beanstalk: A robust solution for deploying and managing applications in the cloud.
- DigitalOcean: Offers virtual private servers that can be configured for your Python application.
Basic Deployment Steps:
- Prepare your application for production, including setting up a production server.
- Choose a hosting platform and create an account.
- Follow the platform-specific documentation to deploy your application.
Ensure you test your application thoroughly in the production environment to confirm that everything functions as intended.
Expert Insights on Creating Websites with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a website using Python is increasingly popular due to the language’s simplicity and versatility. Frameworks like Django and Flask allow developers to build robust applications quickly, making it an excellent choice for both beginners and experienced programmers.”
Michael Chen (Web Development Instructor, Code Academy). “When considering how to create a website using Python, it is crucial to understand the architecture of web applications. Utilizing Python’s libraries for handling requests, such as Requests and Beautiful Soup, can streamline the development process significantly.”
Sarah Thompson (Lead Developer, Digital Solutions Group). “The choice of Python for web development is not just about coding ease; it also offers strong community support and extensive documentation. Leveraging tools like Jinja2 for templating and SQLAlchemy for database interactions can elevate your project to a professional level.”
Frequently Asked Questions (FAQs)
What are the basic steps to create a website using Python?
To create a website using Python, start by selecting a web framework such as Flask or Django. Set up your development environment, create a project directory, define the application structure, and write your application code. Finally, run the application locally and deploy it to a web server.
Do I need to know HTML and CSS to create a website using Python?
Yes, knowledge of HTML and CSS is essential for creating a website with Python. HTML is used for structuring the content, while CSS is used for styling the appearance of the website. Understanding these technologies will enhance your web development skills.
Which Python web frameworks are recommended for beginners?
For beginners, Flask is highly recommended due to its simplicity and flexibility. Django is also a great choice for those looking for a more feature-rich framework that includes built-in functionalities such as authentication and database management.
Can I use Python for both frontend and backend development?
Python is primarily used for backend development, but it can be integrated with frontend technologies. For frontend development, you can utilize JavaScript, HTML, and CSS, while Python can handle server-side logic and data processing.
How can I deploy my Python web application?
You can deploy your Python web application using platforms like Heroku, AWS, or DigitalOcean. Each platform provides documentation for deploying Python applications, which typically involves setting up a server, configuring the environment, and uploading your code.
Is it possible to create a dynamic website using Python?
Yes, Python is well-suited for creating dynamic websites. By utilizing frameworks like Flask or Django, you can build applications that interact with databases, handle user input, and generate content dynamically based on user interactions or other criteria.
Creating a website using Python involves several key steps that leverage the language’s versatility and the power of various frameworks. The process typically begins with selecting an appropriate web framework, such as Flask or Django, which provides the necessary tools and libraries to streamline development. Flask is known for its simplicity and flexibility, making it ideal for small to medium-sized projects, while Django offers a more robust structure suitable for larger applications with built-in features like authentication and an admin panel.
Once the framework is chosen, developers need to set up their development environment, which includes installing Python, the selected framework, and any additional libraries required for the project. This setup is crucial for ensuring that the development process is efficient and organized. After the environment is ready, the next step involves designing the website’s architecture, including defining the URL routes, creating views, and setting up templates for rendering HTML content.
Testing and deployment are also critical components of the website creation process. Developers should implement unit tests to ensure that the application functions correctly and is free of bugs. Once testing is complete, the website can be deployed to a web server using platforms like Heroku, AWS, or DigitalOcean, making it accessible to users. Overall, Python provides a powerful and flexible option for
Author Profile
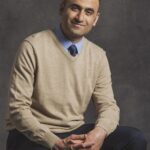
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?