How Can You Decompile a Compiled Python File?
In the world of programming, the ability to decompile a compiled Python file can be a game-changer, whether you’re a developer looking to recover lost source code or a curious learner eager to understand the inner workings of a Python application. Compiled Python files, typically with a `.pyc` extension, are the result of the Python interpreter transforming your human-readable code into bytecode that the machine can execute. However, this transformation often leaves developers wondering how to reverse the process and regain access to the original source code.
Decompiling Python files involves a series of steps and tools designed to convert bytecode back into a more understandable format. While the process may seem daunting at first, it opens up a world of possibilities for debugging, learning, and enhancing existing applications. Understanding the nuances of bytecode and the tools available for decompilation can empower developers to troubleshoot issues, recover lost work, or even analyze third-party libraries.
As we delve deeper into the methods and tools for decompiling Python files, you’ll discover the different approaches available, the legal and ethical considerations to keep in mind, and the best practices for ensuring a smooth decompilation process. Whether you’re a seasoned programmer or just starting your coding journey, mastering the art of decompilation can significantly enhance your
Understanding Compiled Python Files
Compiled Python files, typically with a `.pyc` or `.pyo` extension, are bytecode representations of Python source code. These files are generated by the Python interpreter to improve loading times. However, due to their compiled nature, they are not easily readable. To reverse this process, decompilation is necessary, transforming bytecode back into a human-readable format.
Tools for Decompiling Python Files
Several tools are available for decompiling Python files. Each has its own features and compatibility with different Python versions. Below are some commonly used decompilers:
- Uncompyle6: Suitable for Python 2.5 to 3.7, this tool converts `.pyc` files back into `.py` files.
- decompyle3: Designed for Python 3.7 and later, it focuses on decompiling Python 3 bytecode.
- pycdc: A more advanced option, pycdc can decompile both Python 2 and Python 3 bytecode.
Tool | Python Version Compatibility | Usage |
---|---|---|
Uncompyle6 | 2.5 – 3.7 | Command-line based |
decompyle3 | 3.7+ | Command-line based |
pycdc | 2.x and 3.x | Graphical interface |
Steps to Decompile a Compiled Python File
To decompile a compiled Python file, follow these general steps. The process may vary slightly depending on the tool you choose.
- Install the Decompiler: Use pip to install the decompiler. For example, to install Uncompyle6, run:
bash
pip install uncompyle6
- Locate the Compiled File: Identify the path of the `.pyc` or `.pyo` file you wish to decompile.
- Run the Decompiler:
- For Uncompyle6, use the command:
bash
uncompyle6 -o OUTPUT_DIR INPUT_FILE.pyc
- Replace `OUTPUT_DIR` with the directory where you want the decompiled file to be saved, and `INPUT_FILE.pyc` with the path to your compiled file.
- Review the Output: After running the decompiler, check the output directory for the generated `.py` file. Open it in a text editor to review the decompiled code.
Considerations and Limitations
While decompiling can be useful, it is essential to consider the following:
- Quality of Decompiled Code: The decompiled output may not perfectly match the original source code. Variable names and comments are often lost during compilation.
- Legal Implications: Ensure you have the right to decompile the code, as it may violate copyright or licensing agreements.
- Compatibility Issues: Some decompilers may not support all features of the Python version used for compilation, leading to incomplete or incorrect decompilation.
By following these steps and considerations, users can effectively decompile Python files to access their underlying code.
Understanding Compiled Python Files
Compiled Python files typically have a `.pyc` or `.pyo` extension, containing bytecode that is executed by the Python interpreter. These files are generated to enhance performance by avoiding the need to recompile source code every time a script runs. However, there may be instances when developers need to decompile these files to retrieve the original source code or to analyze the code for security or debugging purposes.
Tools for Decompiling Python Files
Several tools can assist in decompiling compiled Python files. Below is a list of popular options:
- uncompyle6
- Supports Python versions 2.7 and 3.0 to 3.7.
- Command: `uncompyle6
`
- decompyle3
- Designed for Python 3.7+.
- Command: `decompyle3
`
- pycdc
- A cross-platform decompiler for Python bytecode.
- Command: Run the executable with the bytecode file as an argument.
- PyInstaller Extractor
- Useful for extracting files from PyInstaller packages.
- Command: `pyinstxtractor.py
`
Step-by-Step Decompilation Process
The process of decompiling a compiled Python file can vary slightly depending on the tool used. Below is a general guideline using `uncompyle6`.
- Install uncompyle6
Use pip to install the tool:
bash
pip install uncompyle6
- Locate the Compiled File
Ensure you have the `.pyc` file you wish to decompile. This file is usually found in the `__pycache__` directory.
- Run the Decompiler
Use the command line to navigate to the directory containing the `.pyc` file and run:
bash
uncompyle6
- Review the Output
The decompiler will output the original source code to the console. You can redirect this output to a file by using:
bash
uncompyle6
- Edit and Save the Decompiled Code
Open the `output.py` file in a text editor for further analysis or modification.
Common Considerations and Limitations
When decompiling Python files, be mindful of the following:
- Code Obfuscation
If the original code was obfuscated, the decompiled output may be difficult to read.
- Compatibility Issues
Ensure the decompiler supports the Python version of the compiled file.
- Legal and Ethical Implications
Decompiling proprietary or copyrighted code without permission may violate legal agreements. Always ensure compliance with relevant laws.
While decompiling Python files can be a useful skill for developers, it is essential to approach this task responsibly and ethically. Using the right tools and following the outlined steps will facilitate a successful decompilation process.
Expert Insights on Decompiling Compiled Python Files
Dr. Emily Carter (Senior Software Engineer, CodeSecure Solutions). “Decompiling a compiled Python file, typically a .pyc file, involves using tools like `uncompyle6` or `decompyle3`. These tools can reverse the bytecode back into a readable Python source code, but it is essential to understand the legal implications of decompiling software that you do not own.”
Mark Thompson (Cybersecurity Analyst, TechDefend Inc.). “When attempting to decompile Python files, one must consider the version compatibility. Different Python versions produce different bytecode formats, so using the correct decompilation tool that matches the Python version used for compilation is crucial for successful recovery of the source code.”
Linda Nguyen (Open Source Software Advocate, FreeCode Foundation). “While decompiling can be a useful technique for recovering lost code or understanding third-party libraries, it is important to respect intellectual property rights. Always ensure that you have permission to decompile any software, as unauthorized decompilation can lead to legal issues.”
Frequently Asked Questions (FAQs)
What is a compiled Python file?
A compiled Python file is typically a file with a `.pyc` or `.pyo` extension, which contains bytecode that the Python interpreter can execute. This bytecode is generated from the original Python source code files (`.py`) to improve performance.
Why would someone want to decompile a compiled Python file?
Decompiling a compiled Python file allows developers to recover the original source code for debugging, analysis, or educational purposes. It can also be useful for understanding third-party libraries when source code is not available.
What tools can be used to decompile Python bytecode?
Common tools for decompiling Python bytecode include `uncompyle6`, `decompyle3`, and `pycdc`. These tools can convert `.pyc` files back into human-readable Python source code.
Is decompiling a compiled Python file legal?
The legality of decompiling a compiled Python file varies by jurisdiction and depends on the specific circumstances, including copyright laws and the terms of use of the software. It is advisable to consult legal counsel if unsure.
What are the limitations of decompiling Python bytecode?
Decompilation may not perfectly reconstruct the original source code, especially in cases where optimizations or obfuscation techniques were applied. Additionally, comments and certain variable names may be lost during compilation.
How can I decompile a `.pyc` file using `uncompyle6`?
To decompile a `.pyc` file using `uncompyle6`, install the tool via pip (`pip install uncompyle6`), then run the command `uncompyle6
Decompiling a compiled Python file, typically with a .pyc or .pyo extension, involves converting the bytecode back into a more human-readable format, such as source code. This process is essential for developers who may need to recover lost source code or analyze the functionality of third-party libraries. Tools such as `uncompyle6`, `decompyle3`, and `pycdc` are commonly used for this purpose, each offering varying levels of support for different Python versions. Understanding the underlying bytecode structure is also beneficial, as it can help in troubleshooting and enhancing the decompilation process.
It is crucial to note that decompiling software can raise legal and ethical considerations. Users should ensure they have the right to decompile the code in question, as doing so without permission may violate copyright laws or licensing agreements. Additionally, while decompilation can often recover a significant portion of the original source code, the output may not be identical to the original due to optimizations made during compilation.
In summary, decompiling a compiled Python file is a valuable skill for developers, allowing for code recovery and analysis. By utilizing appropriate tools and being mindful of legal implications, developers can effectively navigate the decompilation
Author Profile
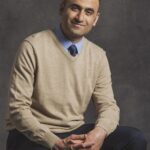
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?