How Can You Effectively Implement Delays in Python?
In the world of programming, timing can be everything. Whether you’re creating an interactive game, automating tasks, or managing data flows, the ability to introduce delays in your code can enhance user experience and improve functionality. Python, a versatile and widely-used programming language, offers several methods to implement delays, allowing developers to control the pace at which their programs execute. In this article, we will explore the various ways to introduce pauses in Python, ensuring that you have the tools necessary to manage timing effectively in your projects.
Delaying execution in Python can serve multiple purposes, from pacing animations in a game to waiting for a resource to become available before proceeding with a task. The language’s simplicity and readability make it an excellent choice for both beginners and seasoned developers looking to fine-tune their applications. By understanding the different techniques available, you can make your code more efficient and user-friendly, while also learning how to handle timing-related challenges that may arise.
As we delve deeper into the topic, we’ll cover various methods to introduce delays, including built-in functions and libraries that can help you achieve your desired timing effects. Whether you need a simple sleep function for a quick pause or a more sophisticated approach for complex applications, this guide will equip you with the knowledge to implement delays seamlessly
Using the time Module
The simplest way to introduce a delay in Python is by utilizing the built-in `time` module. This module provides various time-related functions, and the `sleep()` function is particularly useful for delaying execution.
To use the `sleep()` function, you need to import the `time` module and call `time.sleep(seconds)`, where `seconds` is a floating-point number indicating the number of seconds to delay.
Example code:
“`python
import time
print(“This message will appear first.”)
time.sleep(5) Delay for 5 seconds
print(“This message appears after a 5-second delay.”)
“`
Using the threading Module
Another method for creating delays is through the `threading` module. This is especially useful when you want to run multiple threads simultaneously and incorporate delays within a specific thread without blocking the main program.
The `threading.Timer` class allows you to schedule a function to be called after a specified interval.
Example code:
“`python
import threading
def delayed_function():
print(“This message is delayed by 3 seconds.”)
timer = threading.Timer(3.0, delayed_function)
timer.start() This will execute after 3 seconds
“`
Using Asyncio for Asynchronous Delays
For asynchronous programming, Python’s `asyncio` library provides a way to introduce delays without blocking the event loop. The `asyncio.sleep()` function is used in asynchronous functions to pause execution for a specified duration.
Example code:
“`python
import asyncio
async def main():
print(“Starting the delay…”)
await asyncio.sleep(2) Non-blocking delay for 2 seconds
print(“Delay completed.”)
asyncio.run(main())
“`
Comparison of Delay Methods
The choice of delay method depends on the specific requirements of your application. Below is a comparison table highlighting the key attributes of each method:
Method | Blocking | Use Case |
---|---|---|
time.sleep() | Yes | Simple scripts where blocking behavior is acceptable. |
threading.Timer | No | When you need to run delays in separate threads. |
asyncio.sleep() | No | Asynchronous applications where non-blocking delays are required. |
By understanding these methods, you can effectively manage delays in your Python applications based on your specific needs.
Using the `time` Module
In Python, one of the simplest ways to introduce a delay is by utilizing the built-in `time` module, which provides a straightforward function for pausing execution.
- `time.sleep(seconds)`: This function takes a single argument, which is the number of seconds you want the program to pause. It can accept both integer and floating-point values.
Example:
“`python
import time
print(“This will print first.”)
time.sleep(5) Delays for 5 seconds
print(“This will print after a 5-second delay.”)
“`
Using the `threading` Module
For more complex scenarios where you might need to delay execution in a multithreaded environment, the `threading` module offers the `Timer` class.
- `threading.Timer(interval, function, args=None, kwargs=None)`: This creates a timer that will wait for a specified interval before executing a function.
Example:
“`python
import threading
def delayed_action():
print(“This prints after a delay using threading.”)
Set a timer for 3 seconds
timer = threading.Timer(3, delayed_action)
timer.start() Starts the timer
“`
Asynchronous Delays with `asyncio`
In asynchronous programming, the `asyncio` library allows for non-blocking delays, making it ideal for I/O-bound applications.
- `await asyncio.sleep(seconds)`: This function pauses the execution of the coroutine for the specified number of seconds without blocking the entire program.
Example:
“`python
import asyncio
async def main():
print(“Starting async delay…”)
await asyncio.sleep(2) Non-blocking delay
print(“Finished after 2 seconds.”)
asyncio.run(main())
“`
Delays in GUI Applications
When developing GUI applications, introducing delays may require special handling to avoid freezing the interface. Utilizing the `after` method in libraries like Tkinter can effectively manage this.
Example:
“`python
import tkinter as tk
def delayed_function():
print(“This prints after a delay in a GUI application.”)
root = tk.Tk()
root.after(3000, delayed_function) Delay for 3000 milliseconds
root.mainloop()
“`
Using `timeit` for Performance Measurement
When measuring execution time, the `timeit` module can be used to benchmark how long certain operations take, which indirectly can help in analyzing delays.
Example:
“`python
import timeit
Measure the time it takes to execute a simple loop
execution_time = timeit.timeit(‘for i in range(100): pass’, number=1000)
print(f”Execution time: {execution_time} seconds.”)
“`
Additional Delay Techniques
Other methods to introduce delays might include:
- Busy-waiting: Continuously checking for a condition, which is generally inefficient.
- Using external libraries: Libraries like `sched` can schedule events with delays.
Example using `sched`:
“`python
import sched
import time
scheduler = sched.scheduler(time.time, time.sleep)
def scheduled_action():
print(“This action is scheduled with a delay.”)
Schedule the action to be executed after 5 seconds
scheduler.enter(5, 1, scheduled_action)
scheduler.run() Run the scheduler
“`
Expert Insights on Delaying Execution in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the most straightforward way to introduce a delay is by using the `time.sleep()` function. This method is effective for pausing execution for a specified number of seconds, making it ideal for scenarios such as rate limiting in API calls or creating timed delays in user interfaces.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “For more complex scenarios where you need to delay execution without blocking the entire program, utilizing the `asyncio` library is highly recommended. This allows for asynchronous programming, enabling you to handle other tasks while waiting, which is particularly beneficial in web applications and real-time data processing.”
Sarah Johnson (Python Instructor, Code Academy). “When teaching beginners about delays in Python, I often emphasize the importance of understanding the context in which delays are used. Whether it’s for creating animations, simulating real-world processes, or managing task scheduling, choosing the right method—be it `time.sleep()`, `threading`, or `asyncio`—is crucial for effective programming.”
Frequently Asked Questions (FAQs)
How can I introduce a delay in Python?
You can introduce a delay in Python using the `time.sleep()` function from the `time` module. This function takes a single argument, which is the number of seconds to pause execution.
What is the purpose of using time.sleep() in Python?
The `time.sleep()` function is used to pause the execution of a program for a specified duration. This is useful for rate limiting, waiting for resources, or creating timed sequences in applications.
Can I use time.sleep() with fractional seconds?
Yes, `time.sleep()` accepts floating-point numbers, allowing you to specify delays in fractions of a second, such as `time.sleep(0.5)` for a half-second delay.
Are there alternatives to time.sleep() for delaying execution in Python?
Yes, alternatives include using `threading.Event().wait()` for more complex scenarios or employing asynchronous programming with `asyncio.sleep()` in asynchronous functions.
Is it possible to create a delay in a loop using Python?
Yes, you can create a delay in a loop by calling `time.sleep()` within the loop body. This will pause execution for the specified duration on each iteration.
Does using time.sleep() block the entire program?
Yes, `time.sleep()` blocks the thread in which it is called, pausing all operations in that thread. For non-blocking delays, consider using asynchronous programming techniques.
Delaying execution in Python can be achieved through various methods, with the most common being the use of the `time` module. The `time.sleep()` function allows developers to pause the execution of a program for a specified number of seconds, making it a straightforward solution for introducing delays. This method is particularly useful in scenarios such as rate limiting, pacing tasks, or creating timed intervals between actions.
In addition to the `time` module, other techniques for implementing delays include using asynchronous programming with the `asyncio` library. This approach is beneficial for non-blocking delays, allowing other tasks to run concurrently while waiting. Additionally, libraries such as `threading` can be employed to create delays in a separate thread, providing more flexibility in managing execution flow without freezing the main program.
Overall, understanding the different methods available for introducing delays in Python is crucial for effective program control and optimization. By selecting the appropriate technique based on the specific requirements of a project, developers can enhance the performance and responsiveness of their applications. This knowledge not only aids in managing execution timing but also contributes to the overall efficiency of Python programming.
Author Profile
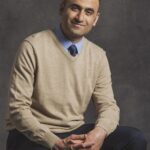
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?