How Can You Effectively Delete a Task Row in JavaScript?
In the realm of web development, managing dynamic content is a fundamental skill that every developer must master. One common scenario is the need to delete a task row from a list, whether it’s in a to-do application, a project management tool, or any interactive web interface. This seemingly simple action can significantly enhance user experience, allowing for a cleaner and more efficient interface. But how exactly do you implement this functionality using JavaScript? In this article, we will explore the various methods and best practices for deleting task rows, ensuring your application remains responsive and user-friendly.
Deleting a task row in JavaScript involves more than just removing an element from the DOM; it requires an understanding of event handling, data management, and user interface updates. When a user decides to delete a task, it’s essential to provide immediate feedback and ensure that the underlying data structure reflects this change. This process typically includes listening for user interactions, such as button clicks, and executing a function that modifies the task list accordingly.
Furthermore, we’ll delve into different approaches to achieve this, from manipulating the Document Object Model (DOM) directly to utilizing frameworks and libraries that streamline the process. Whether you’re building a simple JavaScript application or working within a more complex framework, understanding how to effectively delete task rows will empower
Identifying the Task Row
To delete a task row in JavaScript, the first step is identifying the specific row you intend to remove. Typically, task rows are represented as elements in the Document Object Model (DOM). They might be contained in a table or a list, and each row or item should have a unique identifier, such as an `id` attribute or a specific class.
You can use various methods to select the element, such as `document.getElementById`, `document.querySelector`, or `document.getElementsByClassName`. Here’s an example of selecting a task row using `querySelector`:
javascript
const taskRow = document.querySelector(‘.task-row[data-id=”1″]’);
This code snippet selects a task row with a class of `task-row` and a data attribute of `data-id` equal to `1`.
Removing the Task Row
Once you have identified the task row, the next step is to remove it from the DOM. The `remove()` method is straightforward and effective for this purpose. You can execute the following code:
javascript
if (taskRow) {
taskRow.remove();
}
This code checks if the task row exists and, if so, removes it from the DOM.
Handling Event Listeners
If the task rows are associated with event listeners (for example, a delete button for each task), you should ensure that these listeners are appropriately managed. You can create a function to handle the deletion process:
javascript
function deleteTask(event) {
const taskRow = event.target.closest(‘.task-row’);
if (taskRow) {
taskRow.remove();
}
}
In this example, `event.target.closest(‘.task-row’)` finds the nearest task row relative to the button clicked, allowing for dynamic deletion based on user interaction.
Example of Task Row Deletion
Here’s a complete example that demonstrates how to delete a task row from an HTML table:
Task 1 | |
Task 2 |
In this example, when the delete button is clicked, the corresponding task row is removed from the table.
Best Practices for Deleting Task Rows
When implementing functionality to delete task rows, consider the following best practices:
- User Confirmation: Before deletion, confirm with the user to prevent accidental deletions.
- Error Handling: Implement error handling to manage any issues that might arise during the deletion process.
- Dynamic Updates: Ensure that any related data structures are updated accordingly to reflect the deletion.
- Accessibility: Ensure buttons are accessible to all users, including those using screen readers.
Best Practice | Description |
---|---|
User Confirmation | Ask users to confirm before deleting a task. |
Error Handling | Handle potential errors during the deletion process. |
Dynamic Updates | Update data structures to reflect changes. |
Accessibility | Ensure deletion buttons are accessible for all users. |
Identifying the Task Row to Delete
To delete a task row in JavaScript, the first step is to properly identify the specific row that needs to be removed. This can be achieved by using unique identifiers such as IDs or classes. Here’s how to approach this:
- Using ID: If each task row has a unique ID, you can easily select it for deletion.
- Using Classes: If multiple rows share the same class, you can loop through them to find the correct one.
- Using Data Attributes: Custom data attributes can help in uniquely identifying rows.
Removing the Task Row from the DOM
Once you have identified the row, you can remove it from the Document Object Model (DOM) using JavaScript. Here are some methods to achieve this:
- Using `remove()` Method: This method directly removes the element from the DOM.
javascript
const taskRow = document.getElementById(‘task-row-id’); // Replace with your row’s ID
taskRow.remove();
- Using `parentNode.removeChild()`: If you need to target a specific child node within a parent element, this method is effective.
javascript
const taskRow = document.getElementById(‘task-row-id’); // Replace with your row’s ID
taskRow.parentNode.removeChild(taskRow);
Handling Event Listeners
When dealing with task rows, it’s essential to manage any event listeners associated with the task row to prevent memory leaks. Consider the following:
- Removing Event Listeners: If you’ve attached any event listeners to the task row, make sure to remove them before deleting the row.
javascript
function handleClick() {
// Handle click event
}
taskRow.removeEventListener(‘click’, handleClick);
- Using Delegated Events: Instead of adding event listeners directly to each task row, consider using event delegation on a parent element to simplify management.
Example Code for Deleting a Task Row
Here is a complete example demonstrating how to delete a task row using a button click:
Task 1 | |
Task 2 |
Updating the Data Model
If your task rows are backed by a data model (e.g., an array of tasks), it’s important to update this model after deletion to maintain consistency. Here’s how:
- Locate the Index: Find the index of the task to be deleted.
- Remove the Task: Use `splice()` to remove the task from the array.
javascript
let tasks = [‘Task 1’, ‘Task 2’];
function deleteTask(taskIndex) {
tasks.splice(taskIndex, 1); // Remove the task from the array
}
By following these guidelines, you can effectively delete task rows in JavaScript while ensuring that your application remains efficient and well-structured.
Expert Insights on Deleting Task Rows in JavaScript
Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “To effectively delete a task row in JavaScript, it is crucial to first identify the specific row element within the DOM. Utilizing methods such as `removeChild()` on the parent node of the target row can ensure a clean deletion without leaving residual elements.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Implementing event listeners for delete buttons associated with each task row can streamline the deletion process. By using the `dataset` attribute, developers can easily access the unique identifier of the row to be deleted, enhancing both efficiency and maintainability of the code.”
Sarah Thompson (Full Stack Developer, Web Solutions Group). “It’s essential to consider user experience when deleting task rows. Implementing a confirmation dialog before executing the deletion can prevent accidental removals. This practice not only improves usability but also safeguards against unintended data loss.”
Frequently Asked Questions (FAQs)
How can I delete a specific task row in a JavaScript array?
To delete a specific task row in a JavaScript array, use the `splice()` method. For example, if you want to remove the task at index `i`, use `tasks.splice(i, 1);`, where `tasks` is your array of tasks.
What is the best way to remove a task from the DOM in JavaScript?
To remove a task from the DOM, first select the element using methods like `getElementById()` or `querySelector()`, then call the `remove()` method on that element. For example, `document.getElementById(‘taskId’).remove();` will remove the element with the specified ID.
Can I delete a task row using an event listener?
Yes, you can delete a task row using an event listener. Attach a click event listener to the delete button associated with the task row, and within the event handler, use the `remove()` method to delete the corresponding DOM element.
How do I ensure the task is deleted both from the UI and the data structure?
To ensure the task is deleted from both the UI and the data structure, first remove the task from the data structure (e.g., using `splice()`), then update the UI by removing the corresponding DOM element. This ensures consistency between your data and the displayed tasks.
What should I do if I want to confirm deletion before removing a task?
To confirm deletion before removing a task, use the `confirm()` method to prompt the user. If the user confirms, proceed with the deletion logic; otherwise, cancel the operation. For example: `if (confirm(‘Are you sure you want to delete this task?’)) { /* delete logic */ }`.
Is there a way to undo a delete action in JavaScript?
Yes, you can implement an undo feature by storing the deleted task in a temporary variable or an array. After deletion, provide an option for the user to restore the task within a specified time frame, using methods like `push()` to re-add it to the original array and updating the UI accordingly.
In JavaScript, deleting a task row typically involves manipulating the Document Object Model (DOM) to remove an element representing the task. This process often requires selecting the specific row or element that needs to be deleted, and then utilizing methods such as `remove()` or `parentNode.removeChild()` to effectively eliminate it from the interface. Understanding event handling is also crucial, as user interactions, such as clicking a delete button, often trigger the deletion process.
Moreover, it is essential to consider the underlying data structure that holds the tasks. If the tasks are stored in an array or an object, deleting a task from the UI should be accompanied by an update to the data structure to maintain consistency. This ensures that the application state accurately reflects the current tasks, preventing potential discrepancies between the displayed data and the underlying logic.
In summary, deleting a task row in JavaScript is a straightforward process that involves DOM manipulation and careful consideration of the data structure. By effectively managing both the user interface and the underlying data, developers can create a seamless experience for users when managing tasks. This approach not only enhances functionality but also contributes to better application performance and user satisfaction.
Author Profile
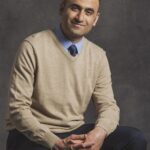
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?