How Can You Effectively Determine Check/Uncheck States of Checkboxes in a Tree Structure Using Angular?
In the world of web development, user interfaces play a pivotal role in enhancing user experience and interaction. One of the common UI elements that developers often implement is the checkbox, particularly within tree structures that allow for hierarchical data representation. Imagine an application where users can manage complex data sets effortlessly, selecting or deselecting items with a simple click. This is where mastering the art of check/uncheck checkboxes in a tree structure becomes essential for Angular developers.
Understanding how to effectively manage checkboxes in a tree can significantly improve the usability of your application. Angular, with its robust framework and powerful data binding capabilities, provides developers with the tools needed to create dynamic and interactive components. By leveraging Angular’s features, you can implement a system that not only reflects the user’s selections but also maintains the integrity of the hierarchical data structure.
As you delve deeper into this topic, you will discover various techniques and best practices for managing checkbox states within a tree. From handling parent-child relationships to ensuring that changes propagate correctly throughout the tree, mastering these concepts will empower you to create intuitive interfaces that respond seamlessly to user interactions. Whether you’re building a complex data management tool or a simple checklist application, understanding how to determine the check/uncheck states of checkboxes in a tree structure will elevate your Angular development
Understanding Checkbox States in Angular Tree Structures
In Angular applications, managing checkboxes within a tree structure can be quite complex, particularly when dealing with nested items. The checkbox state should reflect the state of its parent items, and vice versa, ensuring a seamless user experience.
To achieve this, you can utilize Angular’s two-way data binding and event handling features. The primary steps involved in determining the check or uncheck state of checkboxes in a tree structure include:
- Data Structure: Define a recursive data model that includes properties for the checkbox state.
- Template Binding: Use Angular’s binding features to connect checkbox states to your data model.
- Event Handling: Implement event listeners to handle user interactions and update the state accordingly.
Defining the Data Model
A well-structured data model is crucial for managing checkbox states. Each node in the tree should maintain its own state, as well as references to its child nodes. Below is an example of a data structure that can be used:
“`typescript
export interface TreeNode {
id: number;
name: string;
checked: boolean;
children?: TreeNode[];
}
“`
This model allows each node to maintain its own `checked` status and potentially hold an array of `children` nodes.
Binding Checkboxes in the Template
To bind checkboxes in the Angular template, you can utilize `ngModel` for two-way data binding. Below is an example of how to bind the checkbox state to the tree nodes in an Angular component’s template:
“`html
-
{{ node.name }}-
{{ child.name }}
-
“`
Handling Checkbox Changes
When a user checks or unchecks a checkbox, you need to update the state of the parent node and potentially the children. This can be achieved through a method that recursively updates the states based on user interactions.
“`typescript
onCheckboxChange(node: TreeNode) {
node.checked = !node.checked; // Toggle the checked state
if (node.children) {
node.children.forEach(child => {
child.checked = node.checked; // Set child states based on parent
this.onCheckboxChange(child); // Recursively update children
});
}
}
“`
Visualizing Checkbox States
To clearly communicate the state of checkboxes, you might want to implement a visual representation. Below is a table summarizing the expected checkbox states based on different scenarios:
Parent State | Child State | Checkbox Indication |
---|---|---|
Checked | Checked | Checked |
Checked | Unchecked | Indeterminate |
Unchecked | Unchecked | Unchecked |
This table can help developers understand how to implement the logic for setting the checkbox states correctly as they work with tree structures in their Angular applications.
Understanding the Checkbox States in Angular Tree Components
In Angular applications, managing the checked and unchecked states of checkboxes in tree structures requires a clear strategy. This can be achieved by utilizing Angular’s reactive forms or template-driven forms. Each node of the tree can have a boolean property representing its checked state.
Setting Up the Tree Structure
Define the tree data structure, ensuring each node includes a property for the checkbox state:
“`typescript
interface TreeNode {
id: number;
name: string;
checked: boolean;
children?: TreeNode[];
}
const treeData: TreeNode[] = [
{ id: 1, name: ‘Parent 1’, checked: , children: [{ id: 2, name: ‘Child 1’, checked: }] },
{ id: 3, name: ‘Parent 2’, checked: , children: [] }
];
“`
Checkbox State Management
To manage the checkbox states effectively, implement functions to check and uncheck nodes recursively. This ensures that checking a parent node updates its children accordingly.
“`typescript
checkNode(node: TreeNode, checked: boolean): void {
node.checked = checked;
if (node.children) {
node.children.forEach(child => this.checkNode(child, checked));
}
}
“`
Handling User Interaction
Utilize Angular’s binding features to update the model when a checkbox is checked or unchecked:
“`html
-
{{ node.name }}-
{{ child.name }}
-
“`
Implementing Indeterminate State
To visually represent a partially checked state (indeterminate), a method to calculate the checkbox state of parent nodes based on their children is necessary.
“`typescript
updateParentNodeState(node: TreeNode): void {
if (node.children) {
const allChecked = node.children.every(child => child.checked);
const anyChecked = node.children.some(child => child.checked);
node.checked = allChecked;
node.indeterminate = !allChecked && anyChecked;
// Update parent recursively
// Call this function on the parent node if applicable
}
}
“`
Styling Indeterminate Checkbox
To reflect the indeterminate state visually, style the checkbox accordingly:
“`css
input[type=”checkbox”]:indeterminate {
background-color: orange; /* Example color */
}
“`
Testing Checkbox Functionality
Ensure to test the functionality across different scenarios, such as:
- Checking a parent node.
- Unchecking a parent node.
- Checking/unchecking child nodes.
- Verifying the indeterminate state of parent nodes.
Utilizing tools like Jasmine and Karma for unit tests can streamline this process, ensuring that all edge cases are handled effectively.
This structure allows for a comprehensive approach to managing checkbox states in tree components within Angular applications. Implementing these strategies will lead to an intuitive user experience and maintainable code.
Strategies for Managing Checkbox States in Angular Tree Structures
Dr. Emily Carter (Senior Frontend Developer, Tech Innovations Inc.). “To effectively manage the check/uncheck states of checkboxes in an Angular tree structure, it is crucial to implement a recursive function that traverses the tree nodes. This function should update the state of each parent node based on the states of its child nodes, ensuring that the UI accurately reflects the hierarchical relationships.”
Michael Chen (Angular Specialist, CodeCraft Academy). “Utilizing Angular’s reactive forms can significantly simplify checkbox state management in tree structures. By leveraging FormGroups and FormArrays, developers can create a dynamic model that reflects the checkbox states, allowing for easier updates and validations as users interact with the tree.”
Sarah Thompson (UI/UX Engineer, Digital Solutions Group). “When designing a tree with checkboxes in Angular, it is essential to consider user experience. Implementing visual indicators for indeterminate states can enhance clarity. Additionally, ensuring that check/uncheck actions are intuitive and responsive will lead to a more engaging user interface.”
Frequently Asked Questions (FAQs)
How can I programmatically check or uncheck checkboxes in an Angular tree?
You can programmatically check or uncheck checkboxes in an Angular tree by manipulating the data model that drives the tree structure. Update the checked property of the relevant nodes in your data source and ensure that the tree component reflects these changes through Angular’s change detection.
What are the best practices for managing checkbox states in a tree structure?
Best practices include maintaining a centralized state management approach, such as using services or NgRx for state management. This ensures consistent checkbox states across the application. Additionally, use a recursive function to traverse the tree and update the states efficiently.
How do I handle checkbox state changes in an Angular tree component?
You can handle checkbox state changes by binding the checkbox input to a method that updates the corresponding node’s state in your data model. Use Angular’s two-way data binding with `[(ngModel)]` or reactive forms to facilitate this interaction.
What libraries can assist with creating tree structures with checkboxes in Angular?
Libraries such as Angular Material, PrimeNG, and ngx-treeview provide pre-built components for creating tree structures with checkboxes. These libraries come with built-in functionality to manage checkbox states, making implementation easier.
Can I implement a “select all” feature for checkboxes in an Angular tree?
Yes, you can implement a “select all” feature by creating a method that toggles the checked state of all child nodes when the parent checkbox is checked or unchecked. Ensure to update the parent checkbox state based on the individual child checkbox states as well.
How can I synchronize checkbox states between parent and child nodes in an Angular tree?
To synchronize checkbox states, you should implement a logic that checks whether all child nodes are checked or unchecked when a parent node’s state changes. Conversely, update the parent node’s state based on the collective state of its children whenever any child checkbox is toggled.
Determining the check and uncheck states of checkboxes in a tree structure within an Angular application involves understanding the hierarchical nature of the data. Angular provides various ways to manage state, including reactive forms and template-driven forms. By leveraging Angular’s data binding features, developers can effectively synchronize the checkbox states with the underlying data model, ensuring that changes in the UI are accurately reflected in the data structure.
One effective approach is to implement a recursive function that traverses the tree structure. This function can be used to check or uncheck all child nodes when a parent checkbox is toggled. Additionally, maintaining a state variable to track whether all children are checked or unchecked can help in determining the state of the parent checkbox. This two-way data binding allows for a seamless user experience, where the UI responds dynamically to user interactions.
Moreover, utilizing Angular’s Change Detection strategy can optimize performance by minimizing unnecessary re-renders. It is also essential to consider edge cases, such as partially checked states, where a parent checkbox should reflect the mixed state of its children. By implementing these strategies, developers can create a robust and user-friendly tree component that effectively manages checkbox states in Angular applications.
Author Profile
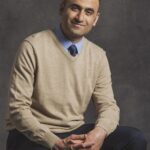
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?