How Can You Develop a Website Using Python?
In today’s digital landscape, having a website is essential for individuals and businesses alike. While there are numerous tools and platforms available for building websites, Python has emerged as a powerful and versatile programming language that can simplify the development process. Whether you’re a seasoned developer or a curious beginner, learning how to develop a website using Python opens up a world of possibilities, allowing you to create dynamic, interactive, and robust web applications. This article will guide you through the essentials of web development with Python, empowering you to transform your ideas into reality.
Python’s popularity in web development stems from its readability, simplicity, and a rich ecosystem of frameworks and libraries. With tools like Flask and Django, developers can quickly set up a web application, manage databases, and create user-friendly interfaces. These frameworks not only streamline the development process but also provide built-in features that enhance security and scalability. As you embark on your web development journey, understanding these frameworks and their capabilities will be crucial in building effective and efficient websites.
Moreover, the flexibility of Python allows for seamless integration with various technologies and platforms, making it an ideal choice for projects of any scale. Whether you’re looking to create a personal blog, an e-commerce site, or a complex web application, Python provides the resources and
Choosing a Framework
When developing a website using Python, selecting an appropriate framework is crucial. The choice of framework can significantly impact development speed, complexity, and scalability. Here are some popular frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It includes built-in features like an ORM, authentication, and an admin panel.
- Flask: A micro-framework that is lightweight and easy to extend. It is suitable for small to medium-sized applications where simplicity is desired.
- FastAPI: An emerging framework focused on performance and ease of use for building APIs. It utilizes Python type hints for automatic validation.
The table below summarizes the key features of each framework:
Framework | Type | Key Features |
---|---|---|
Django | Full-Stack | ORM, Admin Interface, Built-in Auth |
Flask | Micro | Lightweight, Extensible, Simple |
FastAPI | API Framework | Fast, Type Hints, Async Support |
Setting Up Your Environment
Establishing a proper development environment is essential for efficiency and organization. Follow these steps to set up your Python environment:
- Install Python: Ensure you have the latest version of Python installed. Use a package manager like `pip` for installations.
- Create a Virtual Environment: This isolates your project dependencies. Use the following command:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- On Windows: `myenv\Scripts\activate`
- On macOS/Linux: `source myenv/bin/activate`
- Install Required Packages: Use `pip` to install the chosen framework and other dependencies. For example, to install Django:
“`bash
pip install django
“`
Building the Application
Once your environment is set up, you can start building your application. The process generally includes the following steps:
- Create a Project: Use the framework’s command-line tool to create a new project.
- For Django, run:
“`bash
django-admin startproject myproject
“`
- Create an App: Inside your project, create apps that encapsulate specific functionalities.
- For example, to create an app in Django:
“`bash
python manage.py startapp myapp
“`
- Define Models: In your app, define data models that represent your database tables.
- Set Up Views and URLs: Create views that handle requests and define URL patterns to route traffic to the appropriate views.
- Templates: Use templates to render dynamic HTML pages.
- Static Files: Manage static files like CSS and JavaScript for styling and functionality.
Testing Your Application
Testing is an integral part of web development. Ensure that your application works as intended by writing and running tests. Frameworks like Django come with built-in testing tools. Here are some testing strategies:
- Unit Testing: Test individual components for expected behavior.
- Integration Testing: Verify that different parts of your application work together seamlessly.
- End-to-End Testing: Simulate user interactions to ensure the application behaves as expected.
To run tests in Django, use the command:
“`bash
python manage.py test
“`
By following these guidelines, you will be well on your way to developing a robust website using Python.
Choosing a Framework
Selecting the right framework is crucial for developing a website using Python. Several popular frameworks cater to different needs, each with its own set of features and benefits.
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. Ideal for complex, data-driven websites.
- Flask: A micro-framework that is lightweight and flexible, suitable for smaller applications or when you want more control over components.
- FastAPI: Designed for building APIs quickly and efficiently, with automatic interactive documentation.
Framework | Best For | Key Features |
---|---|---|
Django | Large projects | Batteries included, ORM, Admin interface |
Flask | Small projects | Simplicity, modularity, customizable |
FastAPI | APIs | Asynchronous support, automatic docs |
Setting Up the Development Environment
Setting up a Python development environment is essential for creating a website. Follow these steps:
- Install Python: Ensure Python (preferably 3.x) is installed. You can download it from the [official Python website](https://www.python.org/downloads/).
- Create a Virtual Environment: This helps manage dependencies for your projects.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
“`
- Install Required Packages: Use pip to install your chosen framework.
“`bash
pip install django for Django
pip install flask for Flask
pip install fastapi for FastAPI
“`
Building the Website
Once your environment is set up, start building your website. Here are the general steps:
- Create a Project: Initialize a new project using the framework’s command-line tools.
- For Django:
“`bash
django-admin startproject myproject
“`
- For Flask, create a new Python file (e.g., `app.py`) and set up a basic application.
- Define Models: If using Django, define your models in `models.py`. For Flask, you can use an ORM like SQLAlchemy.
- Create Views: Set up routes to handle requests and define what data is returned.
- Django example:
“`python
from django.http import HttpResponse
def home(request):
return HttpResponse(“Hello, World!”)
“`
- Flask example:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
“`
- Design Templates: Use HTML templates to create the user interface. Django uses its templating engine, while Flask can utilize Jinja2.
- Static Files: Serve CSS, JavaScript, and image files. Ensure they are correctly linked in your templates.
Testing the Application
Testing your web application is vital for ensuring functionality and performance. Use the following approaches:
- Unit Testing: Write tests for individual components.
- Integration Testing: Test how different parts of your application work together.
- User Acceptance Testing: Validate the application against user requirements.
Framework-specific testing tools include:
- Django: Built-in test framework.
- Flask: Use `pytest` or `unittest`.
“`bash
Example command to run tests
python manage.py test For Django
pytest For Flask
“`
Deployment
Deploying your website involves making it accessible over the internet. Consider these options:
- Cloud Hosting: Use platforms like Heroku, AWS, or DigitalOcean.
- Containerization: Docker can help package your application with all dependencies.
Steps for deployment generally include:
- Prepare Configuration: Set environment variables and configuration files.
- Deploy Code: Push your code to the chosen platform.
- Set Up Database: If applicable, configure the database settings.
- Run Migrations: For frameworks that support them, ensure database schemas are up-to-date.
By following these structured steps, you can efficiently develop and deploy a website using Python.
Expert Insights on Developing a Website Using Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When developing a website using Python, it is essential to choose the right framework. Django and Flask are two popular options, each offering distinct advantages. Django is ideal for larger applications due to its robust features, while Flask provides flexibility for smaller projects.”
Michael Chen (Web Development Instructor, Code Academy). “Understanding the fundamentals of HTML, CSS, and JavaScript is crucial when using Python for web development. Python handles the backend logic, but a solid grasp of frontend technologies ensures a seamless integration and enhances user experience.”
Sarah Patel (Lead Developer, Web Solutions Group). “Testing and deployment are often overlooked in the web development process. Utilizing tools like pytest for testing your Python code and services like Heroku or AWS for deployment can significantly streamline the development cycle and improve application reliability.”
Frequently Asked Questions (FAQs)
What are the basic requirements to develop a website using Python?
To develop a website using Python, you need a basic understanding of Python programming, knowledge of web frameworks like Flask or Django, familiarity with HTML, CSS, and JavaScript, and a development environment set up with Python installed.
Which Python frameworks are best for web development?
The most popular Python frameworks for web development are Django, which is feature-rich and ideal for large applications, and Flask, which is lightweight and suitable for smaller projects. Other options include FastAPI and Pyramid.
How do I set up a local development environment for Python web development?
To set up a local development environment, install Python from the official website, choose a framework (like Flask or Django), create a virtual environment using `venv`, and install necessary packages using `pip`.
Can I use Python for front-end development?
Python is primarily used for back-end development. However, you can integrate Python with front-end technologies using frameworks like Brython or Transcrypt, but it is more common to use JavaScript for front-end tasks.
How do I deploy a Python web application?
To deploy a Python web application, you can use platforms like Heroku, AWS, or DigitalOcean. The process typically involves setting up a server, configuring the environment, and using a web server like Gunicorn or Nginx to serve your application.
What databases can I use with Python web applications?
You can use several databases with Python web applications, including relational databases like PostgreSQL and MySQL, as well as NoSQL databases like MongoDB. Django and Flask both provide ORM support for easy database interactions.
Developing a website using Python involves several key steps and considerations that leverage the language’s versatility and the power of various frameworks. The process typically begins with selecting a suitable web framework, such as Flask or Django, which provide the necessary tools and libraries to streamline development. Flask is ideal for smaller applications due to its lightweight nature, while Django is better suited for larger projects requiring a more robust structure and built-in features.
Once the framework is chosen, developers must focus on designing the website’s architecture, including the database setup, routing, and templates. This stage is crucial as it lays the foundation for how users will interact with the site. Integrating a database, such as SQLite or PostgreSQL, is essential for managing data efficiently. Additionally, understanding HTML, CSS, and JavaScript is vital, as these technologies work in tandem with Python to create a fully functional and visually appealing website.
Testing and deployment are the final stages of the development process. It is important to rigorously test the website for bugs and performance issues to ensure a smooth user experience. Once testing is complete, deploying the website to a server, such as Heroku or AWS, makes it accessible to users. Continuous maintenance and updates are also necessary to keep the website
Author Profile
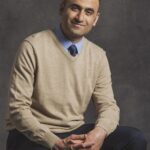
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?