How Can You Display ViewData[Title] in HTML?
In the world of web development, effectively displaying data is crucial for creating dynamic and engaging user experiences. One common scenario developers encounter is the need to present data stored in a server-side structure, such as ViewData, within an HTML view. Whether you’re building a robust web application or a simple website, understanding how to seamlessly integrate data into your HTML can significantly enhance the functionality and appeal of your project. In this article, we will explore the techniques and best practices for displaying `ViewData[title]` in HTML, empowering you to create more interactive and informative web pages.
When working with ASP.NET MVC or similar frameworks, `ViewData` serves as a convenient way to pass data from the controller to the view. This lightweight dictionary allows developers to share information, such as titles, messages, or any other data type, without tightly coupling the model and view. However, simply passing data isn’t enough; knowing how to render it effectively in HTML is essential for ensuring that users can easily access and understand the information presented to them.
As we delve deeper into this topic, we will cover various methods to display `ViewData[title]`, including syntax, context, and practical examples. By mastering these techniques, you’ll be equipped to create cleaner, more
Utilizing ViewData in HTML
To display data stored in the ViewData dictionary in an HTML view, you can use Razor syntax, which is integral to ASP.NET MVC applications. ViewData allows you to pass data from a controller to the view, making it accessible for rendering dynamic content.
Accessing a value from ViewData is straightforward. You simply use the key associated with the value you want to retrieve. For example, if you have stored a title in ViewData with the key `”title”`, you can display it in your HTML as follows:
“`html
@ViewData[“title”]
“`
This syntax allows the title stored in ViewData to be rendered directly in an `
` HTML element.
Best Practices for Using ViewData
When working with ViewData, it’s essential to follow best practices to maintain code readability and ensure data integrity. Here are some key guidelines:
- Use Strongly Typed Models: Whenever possible, utilize strongly typed models instead of ViewData. This approach enhances type safety and IntelliSense support in your IDE.
- Limit ViewData Usage: Use ViewData sparingly, primarily for small amounts of data that do not warrant a full model. Overusing ViewData can make your views harder to maintain.
- Check for Null Values: Always check for null when accessing ViewData to avoid runtime errors. You can do this using a conditional statement:
“`html
@if (ViewData[“title”] != null)
{
@ViewData[“title”]
}
else
{
Default Title
}
“`
Example of Displaying Multiple ViewData Entries
If you need to display multiple entries from ViewData, you can do so by iterating through the keys. Below is an example that assumes you have set multiple entries in your controller.
“`html
-
@foreach (var key in ViewData.Keys)
- @key: @ViewData[key]
{
}
“`
This code snippet creates an unordered list of all the keys and their corresponding values stored in ViewData.
Creating a Table with ViewData
To present data in a more structured format, you can utilize an HTML table. Here’s an example that displays a list of products using ViewData, assuming you have stored a list in the ViewData dictionary.
“`html
Product Name | Price |
---|---|
@product.Name | @product.Price.ToString(“C”) |
“`
In this example, we assume that `ViewData[“products”]` contains a list of product objects. Each product’s name and price are displayed in a table format.
Conclusion on ViewData Usage
By effectively utilizing ViewData, you can enhance the interactivity and dynamism of your web applications. Following best practices ensures that your code remains clean and maintainable while leveraging the powerful capabilities of the ASP.NET MVC framework.
Displaying ViewData in HTML
To display `ViewData` in an HTML view within an ASP.NET MVC application, you typically access the data stored in `ViewData` using the keys assigned to it. The key for the title is often defined in the controller and then accessed in the view.
Accessing ViewData in Your View
In your Razor view, you can access the `ViewData` object directly by using the appropriate key. For example, if your key for the title is `title`, you would use the following syntax:
“`html
@ViewData[“title”]
“`
This line of code takes the value associated with the key `title` from the `ViewData` dictionary and renders it as an `
` heading in your HTML output.
Setting ViewData in Controller
In your ASP.NET MVC controller, you set the `ViewData` value like this:
“`csharp
public ActionResult Index()
{
ViewData[“title”] = “Welcome to My Application”;
return View();
}
“`
This snippet defines the title that will be displayed in the view. When the `Index` action is invoked, the title will be accessible in the associated view.
Handling Null Values
When using `ViewData`, it’s essential to ensure that the key exists to prevent runtime errors. You can do this by checking if the key is present:
“`html
@if (ViewData[“title”] != null)
{
@ViewData[“title”]
}
else
{
Default Title
}
“`
This approach provides a default title if the `ViewData` key does not exist, enhancing the robustness of your application.
Example Implementation
Here is a complete example demonstrating how to set and display `ViewData` in a simple ASP.NET MVC application:
Controller Code:
“`csharp
public class HomeController : Controller
{
public ActionResult Index()
{
ViewData[“title”] = “Welcome to My Application”;
return View();
}
}
“`
View Code (`Index.cshtml`):
“`html
@{
ViewBag.Title = “Home Page”;
}
@ViewData[“title”]
This is the main content of the page.
“`
Best Practices
When using `ViewData`, consider the following best practices:
- Use Strongly Typed Views: Whenever possible, prefer using strongly typed views over `ViewData` for better type safety.
- Limit Usage: Use `ViewData` sparingly, as over-reliance can lead to code that is hard to maintain.
- Naming Conventions: Use clear and consistent naming conventions for keys to enhance readability and manageability.
By following these guidelines, you can effectively manage and display data within your ASP.NET MVC views, ensuring a clean and maintainable codebase.
Best Practices for Displaying ViewData in HTML
Jordan Matthews (Web Development Specialist, CodeCraft Academy). “To effectively display ViewData in HTML, it is crucial to ensure that the data is properly passed from the controller to the view. Utilizing Razor syntax allows for seamless integration, making the code cleaner and more maintainable.”
Lisa Chen (Senior Software Engineer, Tech Innovations Inc.). “When rendering ViewData in HTML, developers should consider using HTML helpers to enhance security and prevent XSS attacks. This approach not only improves data handling but also ensures a better user experience.”
Michael Thompson (Lead Frontend Developer, Digital Solutions Group). “Incorporating proper data binding techniques is essential when displaying ViewData in HTML. This allows for dynamic updates and ensures that the view reflects the most current state of the data.”
Frequently Asked Questions (FAQs)
How can I display the value of ViewData in an HTML page?
To display the value of ViewData in an HTML page, you can use Razor syntax in your view file. For example, use `@ViewData[“title”]` to render the value stored in ViewData under the key “title”.
What is the purpose of ViewData in ASP.NET MVC?
ViewData is a dictionary object that allows you to pass data from a controller to a view. It is useful for sharing data that does not require a strongly typed model.
Can I use ViewData to pass complex objects to a view?
Yes, you can use ViewData to pass complex objects. However, it is recommended to use strongly typed models for better maintainability and type safety.
What happens if the key does not exist in ViewData?
If you attempt to access a key that does not exist in ViewData, it will return null. It is advisable to check for null before using the value to avoid runtime errors.
Is there a performance difference between ViewData and ViewBag?
Both ViewData and ViewBag serve similar purposes, but ViewBag uses dynamic properties, which may introduce a slight performance overhead. ViewData is a dictionary and is generally more efficient for accessing data.
How do I set a value in ViewData?
You can set a value in ViewData using the syntax `ViewData[“key”] = value;` in your controller action method, where “key” is the name you want to assign to the value.
Displaying data in an HTML view, particularly when using frameworks like ASP.NET MVC, involves utilizing the ViewData dictionary to pass data from the controller to the view. The ViewData object allows developers to store key-value pairs, where the key is a string and the value can be any object. To display a specific title stored in ViewData, one would typically use Razor syntax, such as @ViewData[“title”], within the HTML markup of the view.
It is essential to ensure that the key used in the ViewData dictionary matches the one referenced in the view. This consistency prevents runtime errors and ensures that the intended data is displayed correctly. Additionally, developers should consider using null checks or default values to enhance the robustness of their code, particularly when there is a possibility that the key may not exist in the ViewData.
In summary, displaying ViewData in an HTML view is a straightforward process that enhances the dynamic nature of web applications. By effectively leveraging ViewData, developers can create more interactive and responsive user interfaces. Understanding the proper syntax and best practices for using ViewData is crucial for maintaining clean and efficient code, ultimately leading to a better user experience.
Author Profile
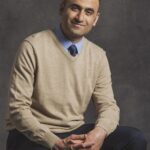
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?