How Can You Effectively Divide a Panel into Specific Dimensions in WPF?
Creating visually appealing and functional user interfaces is a crucial aspect of modern application development, especially when working with Windows Presentation Foundation (WPF). One common challenge developers face is how to effectively divide a panel into specific dimensions to accommodate various elements, ensuring a seamless user experience. Whether you’re designing a dynamic dashboard or a simple form, understanding how to manipulate panels and their dimensions can significantly enhance the layout and usability of your application. In this article, we will explore the techniques and best practices for dividing panels in WPF, enabling you to create structured and organized interfaces that cater to your specific design needs.
When working with WPF, panels serve as the backbone of your layout, providing a framework for arranging controls and other UI elements. The ability to divide a panel into specific dimensions allows developers to create responsive designs that adapt to different screen sizes and resolutions. By leveraging the built-in layout controls such as Grid, StackPanel, and WrapPanel, you can achieve precise control over how space is allocated within your application. Each panel type offers unique properties and behaviors that can be utilized to create intricate layouts, making it essential to choose the right one for your specific requirements.
Moreover, understanding the concepts of rows, columns, and margins is fundamental when dividing panels in WPF. These
Understanding Grid Layout for Panel Division
To divide a panel into specific dimensions in WPF (Windows Presentation Foundation), the Grid layout is one of the most efficient and flexible options available. The Grid allows you to define rows and columns, making it easy to control the size and position of the contained elements.
- Defining Rows and Columns: You can specify the size of each row and column using fixed sizes, star sizing (proportional sizes), or auto sizing.
- Row Definitions: Use `
` elements to set the heights of the rows. - Column Definitions: Use `
` elements to set the widths of the columns.
Example of a basic Grid definition:
“`xml
“`
Using Row and Column Definitions
Each row and column can be defined using different sizing options:
Size Type | Description | Example |
---|---|---|
Fixed | A specific pixel value. | `Height=”50″` |
Star (*) | Proportional sizing based on available space. | `Height=”*”` |
Auto | Size based on the content. | `Height=”Auto”` |
This table summarizes the three types of sizing options you can use within your Grid definitions.
Adding Elements to the Grid
Once your Grid is defined with rows and columns, you can place elements into specific cells using the `Grid.Row` and `Grid.Column` attached properties. Here’s how to do it:
“`xml
“`
In this example, the `Button` is placed in the first row and first column, while the `TextBox` occupies the second row and second column.
Using Margins and Alignments
In addition to defining the layout, you may want to control the spacing and alignment of your controls within the Grid cells. The `Margin` property allows you to add space around the elements, while the `HorizontalAlignment` and `VerticalAlignment` properties help align the elements within their respective cells.
- Margin: Defines the space around the element.
- HorizontalAlignment: Determines the alignment within the cell (e.g., Left, Center, Right).
- VerticalAlignment: Determines the vertical alignment (e.g., Top, Center, Bottom).
Example:
“`xml
“`
This button will have a margin of 5 pixels and will be centered horizontally within the cell.
Conclusion on Grid Panel Division
Utilizing the Grid layout in WPF provides a powerful way to divide panels into specific dimensions, allowing for precise control over the UI’s arrangement and appearance. By understanding row and column definitions, element placement, and alignment properties, developers can create sophisticated user interfaces that meet their design requirements.
Dividing a Panel into Specific Dimensions in WPF
To effectively divide a panel into specific dimensions in a WPF (Windows Presentation Foundation) application, developers can utilize several layout controls. The most commonly used panels for this purpose include `Grid`, `StackPanel`, and `WrapPanel`. Each panel has its unique characteristics suited for different layout needs.
Using Grid for Precise Layout
The `Grid` panel is ideal for defining rows and columns with specific dimensions. You can set fixed heights and widths or use proportional sizing with star notation.
Example of a Grid with Specific Dimensions:
“`xml
“`
Key Points:
- `Height` and `Width` can be set to fixed values (e.g., `100`, `200`).
- Use `*` to allocate space proportionally (e.g., `2*` will take twice the space of `1*`).
StackPanel for Sequential Layout
The `StackPanel` arranges child elements in a single line, either vertically or horizontally. While it does not inherently provide fixed dimensions, you can control the size of individual elements.
Example of a StackPanel:
“`xml
“`
Considerations:
- Use `Orientation` property to decide layout direction.
- Set specific dimensions for each child element to maintain consistency.
WrapPanel for Flow Layout
The `WrapPanel` allows child elements to wrap into the next line or column as space permits. This is useful for dynamic layouts where element sizes may vary.
Example of a WrapPanel:
“`xml
“`
Usage Tips:
- Adjust the size of children to ensure they fit well within the panel.
- The `WrapPanel` can adapt based on available space, but fixed sizes may help maintain visual order.
Combining Panels for Complex Layouts
For more complex layouts, combining different panels can be beneficial. For example, nesting a `StackPanel` within a `Grid` can create organized sections with flexibility.
Nesting Example:
“`xml
“`
Best Practices:
- Ensure that child elements maintain appropriate dimensions to avoid layout issues.
- Use `Margin` and `Padding` properties to enhance spacing and visual appeal.
By effectively utilizing WPF layout controls such as `Grid`, `StackPanel`, and `WrapPanel`, developers can create sophisticated interfaces with specific dimensions tailored to their design requirements.
Expert Insights on Dividing Panels into Specific Dimensions in WPF
Dr. Emily Carter (Senior Software Engineer, WPF Solutions Inc.). “When dividing a panel into specific dimensions in WPF, it is essential to utilize the Grid layout effectively. By defining rows and columns with precise measurements, developers can achieve a structured and responsive design that adapts to various screen sizes.”
Michael Tran (UI/UX Designer, Creative Interfaces). “To ensure that your panel division is visually appealing, consider using the UniformGrid. This allows for equal distribution of space among child elements, which is particularly useful when the dimensions need to be consistent across different UI components.”
Sarah Kim (WPF Application Architect, Tech Innovations). “For dynamic resizing, employing a combination of the DockPanel and StackPanel can be beneficial. This approach allows for flexible arrangements of elements while maintaining control over their dimensions, ensuring a seamless user experience.”
Frequently Asked Questions (FAQs)
How can I divide a panel into specific dimensions in WPF?
You can divide a panel into specific dimensions by using a layout container such as `Grid`, `StackPanel`, or `WrapPanel`. For precise control, `Grid` is recommended, where you can define rows and columns with specific heights and widths.
What is the difference between using a Grid and a StackPanel for layout in WPF?
A `Grid` allows for more complex layouts with defined rows and columns, enabling precise positioning and sizing of elements. A `StackPanel`, on the other hand, arranges child elements in a single line, either vertically or horizontally, without specific dimensions.
How do I set fixed dimensions for rows and columns in a WPF Grid?
You can set fixed dimensions for rows and columns in a `Grid` by specifying the `Height` and `Width` properties in the `RowDefinition` and `ColumnDefinition` elements. For example, `
Can I use percentage values to divide a panel in WPF?
Yes, you can use percentage values by setting the `Height` and `Width` properties of `RowDefinition` and `ColumnDefinition` to `*` (star sizing) or using a combination of fixed and star values. For instance, `Height=”2*”` indicates that the row will take twice the space compared to a row defined with `Height=”1*”`.
What layout options are available in WPF for dividing a panel?
WPF offers several layout options for dividing a panel, including `Grid`, `StackPanel`, `WrapPanel`, `DockPanel`, and `Canvas`. Each has unique properties suited for different layout requirements, allowing for flexible UI design.
How can I programmatically adjust the dimensions of a panel in WPF?
You can programmatically adjust the dimensions of a panel by accessing its properties in the code-behind file. For example, you can modify the `Width`, `Height`, `RowDefinitions`, or `ColumnDefinitions` of a `Grid` directly using Ccode.
Dividing a panel into specific dimensions in WPF (Windows Presentation Foundation) involves utilizing various layout containers and properties that allow for precise control over the arrangement of UI elements. Developers can achieve this by employing containers such as Grid, StackPanel, and DockPanel, each offering unique capabilities for layout management. The Grid, in particular, provides a robust structure for defining rows and columns, enabling developers to specify exact sizes and proportions for each section of the panel.
Key techniques for dividing a panel include setting the Width and Height properties directly on elements, using RowDefinitions and ColumnDefinitions in a Grid to allocate space, and leveraging the Margin and Padding properties for additional spacing adjustments. Additionally, the use of proportional sizing with star notation (e.g., “1*”, “2*”) allows for dynamic resizing based on available space, making the layout responsive to different screen sizes and resolutions.
It is essential to consider the overall design and user experience when dividing panels. Thoughtful layout decisions can enhance usability and accessibility, ensuring that the interface is intuitive and visually appealing. Understanding the behavior of different layout containers and their properties is crucial for creating effective WPF applications that meet specific dimensional requirements.
Author Profile
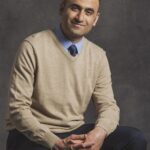
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?