How Can You Master Division in Python: A Comprehensive Guide?
Introduction
In the world of programming, mastering the art of division is essential for anyone looking to harness the power of Python. Whether you’re a novice coder or a seasoned developer, understanding how to divide numbers effectively can unlock a plethora of possibilities, from simple calculations to complex algorithms. Python, with its intuitive syntax and robust functionality, makes division not only straightforward but also versatile. In this article, we’ll explore the various methods of division in Python, ensuring you have the tools you need to tackle any mathematical challenge that comes your way.
When it comes to dividing numbers in Python, there are several approaches to consider, each suited for different scenarios. The language provides built-in operators that allow for both integer and floating-point division, giving you the flexibility to choose the method that best fits your needs. Additionally, Python’s handling of division by zero and its ability to work with complex numbers add layers of sophistication that can be crucial for more advanced applications.
As we delve deeper into the topic, you’ll discover not only the basic syntax for division but also the nuances that differentiate various types of division in Python. From understanding the implications of using the floor division operator to exploring the significance of the modulo operation, this article will equip you with a comprehensive understanding of how to divide in Python, empowering
Using the Division Operator
In Python, the primary operator for division is the forward slash (`/`). This operator performs floating-point division, meaning that it always returns a float even when the numbers are perfectly divisible. For example:
python
result = 10 / 2 # result will be 5.0
It is important to note that if you divide by zero, a `ZeroDivisionError` will be raised.
Integer Division
For scenarios requiring integer results, Python provides the double forward slash (`//`). This operator performs floor division, which rounds down the result to the nearest whole number. Here are some examples:
python
int_result = 10 // 3 # int_result will be 3
int_result = 10 // 2 # int_result will be 5
This operator is useful when you want to discard the decimal portion of the result.
Handling Division by Zero
When dividing by zero, Python will raise a `ZeroDivisionError`. To manage this gracefully, you can use a try-except block:
python
try:
result = 10 / 0
except ZeroDivisionError:
result = “Undefined (division by zero)”
This approach ensures that your program continues to run even when encountering a division by zero.
Dividing Complex Numbers
Python also supports division of complex numbers. The division operation behaves consistently with the rules of complex arithmetic. For instance:
python
a = 3 + 4j
b = 1 + 2j
result = a / b # result will be a complex number
The result will be a complex number calculated according to the division rules for complex numbers.
Using NumPy for Division
When working with arrays, the NumPy library can handle division efficiently. NumPy supports both standard and element-wise division for arrays. For instance:
python
import numpy as np
array1 = np.array([10, 20, 30])
array2 = np.array([2, 5, 3])
result = array1 / array2 # result will be array([5.0, 4.0, 10.0])
This allows for quick computations across large datasets.
Operation | Operator | Description |
---|---|---|
Floating-point division | / | Returns a float result |
Integer division | // | Returns the largest integer less than or equal to the division result |
Element-wise division (NumPy) | / | Divides each element of the array |
Incorporating these techniques will enable you to perform various division operations effectively in Python, catering to both simple and complex scenarios.
Basic Division Operation
In Python, division is primarily performed using the `/` operator, which returns a float result. Here’s a brief overview of how to use it:
python
result = 10 / 2 # result will be 5.0
This method is straightforward and can be applied to integers and floats alike. It is important to note that even when dividing two integers, the result will always be a float.
Integer Division
For cases where you need an integer result from a division, Python provides the `//` operator, which performs floor division. This operator discards the decimal portion of the result:
python
result = 10 // 3 # result will be 3
### Key Points:
- The `//` operator rounds down to the nearest whole number.
- It is useful in scenarios where only whole numbers are desired, such as indexing or counting.
Handling Division by Zero
Division by zero is a common error in programming. In Python, attempting to divide by zero using either the `/` or `//` operator will raise a `ZeroDivisionError`. To handle such situations gracefully, one can use a try-except block:
python
try:
result = 10 / 0
except ZeroDivisionError:
result = “Cannot divide by zero”
### Example Handling:
- Catch the error to prevent the program from crashing.
- Provide a fallback value or message.
Using the `divmod()` Function
Python also offers a built-in function called `divmod()`, which returns both the quotient and the remainder in a single call. This function is particularly useful when both values are needed:
python
quotient, remainder = divmod(10, 3) # quotient will be 3, remainder will be 1
### Benefits of Using `divmod()`:
- Simplifies code by reducing the number of operations needed.
- Clearly indicates the intent to obtain both quotient and remainder.
Rounding Division Results
When precise control over the number of decimal places is required, the built-in `round()` function can be applied to the result of a division operation. It allows you to specify the number of decimal places:
python
result = round(10 / 3, 2) # result will be 3.33
### Rounding Behavior:
- The `round()` function follows standard rounding rules.
- You can specify the number of digits to round to, enhancing precision in calculations.
Example Table of Division Operations
Operation | Syntax | Result |
---|---|---|
Basic Division | `10 / 2` | 5.0 |
Integer Division | `10 // 3` | 3 |
Division by Zero | `10 / 0` | Error |
Using `divmod()` | `divmod(10, 3)` | (3, 1) |
Rounded Division | `round(10 / 3, 2)` | 3.33 |
This table summarizes different division operations in Python, providing a quick reference for users. Each method serves a unique purpose, ensuring flexibility depending on the requirements of your code.
Expert Insights on Dividing in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Dividing in Python is straightforward due to its built-in operators. The use of the forward slash ‘/’ for floating-point division and the double forward slash ‘//’ for integer division allows developers to choose the appropriate method based on the required precision.”
Michael Chen (Python Developer Advocate, CodeCraft). “It is essential to handle division by zero when performing operations in Python. Utilizing try-except blocks can prevent runtime errors and ensure your application remains robust and user-friendly.”
Sarah Patel (Data Scientist, Analytics Hub). “When dividing arrays or matrices in Python, leveraging libraries like NumPy is highly efficient. NumPy’s broadcasting feature simplifies element-wise division, making it an invaluable tool for data analysis and scientific computing.”
Frequently Asked Questions (FAQs)
How do you perform division in Python?
You can perform division in Python using the `/` operator for floating-point division or the `//` operator for integer (floor) division.
What is the difference between `/` and `//` in Python?
The `/` operator performs true division, returning a float result, while the `//` operator performs floor division, returning the largest integer less than or equal to the result.
Can you divide by zero in Python?
No, dividing by zero in Python raises a `ZeroDivisionError`, which indicates that the operation is mathematically undefined.
How can I handle division by zero in Python?
You can handle division by zero using a try-except block. Catch the `ZeroDivisionError` and provide an alternative action or message.
What types of numbers can be divided in Python?
Python allows division of integers, floats, and complex numbers. The result type depends on the types of the operands involved in the division.
Is there a way to limit the number of decimal places in the result of a division?
Yes, you can use the `round()` function to limit the number of decimal places in the result of a division operation. For example, `round(a / b, 2)` will round the result to two decimal places.
In Python, division can be performed using several operators, each serving different purposes. The most commonly used operators for division are the single forward slash (/) for floating-point division and the double forward slash (//) for floor division. The single forward slash returns a float, while the double forward slash truncates the decimal and returns the largest integer less than or equal to the result. Understanding these distinctions is crucial for accurate mathematical operations in programming.
Additionally, Python’s handling of division is influenced by its dynamic typing and built-in support for large integers. This means that developers do not need to worry about integer overflow, as Python can manage large numbers seamlessly. Furthermore, when performing division by zero, Python raises a ZeroDivisionError, which is essential for maintaining the integrity of calculations and preventing runtime errors.
Overall, mastering the various division methods in Python allows for greater flexibility and precision in coding. It is important to choose the appropriate division operator based on the desired outcome, whether it be a floating-point result or an integer. By leveraging Python’s capabilities, programmers can effectively implement mathematical operations in their applications.
Author Profile
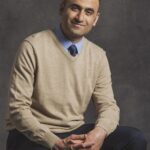
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?