How Can You Master Addition in Python: A Step-by-Step Guide?
In the world of programming, mastering the basics is essential, and few concepts are as fundamental as addition. Whether you’re a complete beginner or looking to brush up on your Python skills, understanding how to perform addition in this versatile language is a stepping stone to more complex operations. Python’s simplicity and readability make it an ideal choice for newcomers, allowing you to focus on logic rather than syntax. In this article, we will explore the various ways to perform addition in Python, equipping you with the knowledge to tackle mathematical problems with confidence.
Addition in Python is not just about combining numbers; it opens the door to a wide array of applications, from data analysis to game development. The language provides several built-in features that make arithmetic operations straightforward and intuitive. You’ll discover how to use basic operators, handle different data types, and even explore the nuances of addition within lists and other collections.
As we delve deeper into the topic, you’ll learn how to leverage Python’s capabilities to perform addition seamlessly, whether you’re working with integers, floats, or even complex data structures. By the end of this article, you’ll have a solid understanding of addition in Python, empowering you to apply these skills in your programming journey. So, let’s get started and unlock the power of addition in Python
Basic Addition in Python
In Python, performing addition is straightforward, utilizing the `+` operator. This operator can be used with various data types, including integers, floats, and even strings for concatenation.
For example, basic addition with integers can be accomplished as follows:
“`python
a = 5
b = 3
result = a + b
print(result) Output: 8
“`
Similarly, addition with floating-point numbers adheres to the same principle:
“`python
x = 2.5
y = 4.5
result = x + y
print(result) Output: 7.0
“`
When dealing with strings, the `+` operator concatenates them instead of performing numerical addition:
“`python
first_name = “John”
last_name = “Doe”
full_name = first_name + ” ” + last_name
print(full_name) Output: John Doe
“`
Using the `sum()` Function
For adding a sequence of numbers, Python provides a built-in function called `sum()`. This function is particularly useful when working with lists or tuples containing numeric values. The syntax is simple, taking an iterable as its primary argument and an optional second argument that specifies the starting value.
Here’s an example:
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) Output: 15
“`
You can also specify a starting value:
“`python
total_with_start = sum(numbers, 10)
print(total_with_start) Output: 25
“`
Performing Addition with Lists
In Python, lists can also be used to perform addition through list comprehension or by using the `map()` function.
Here’s how to add corresponding elements of two lists:
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = [x + y for x, y in zip(list1, list2)]
print(result) Output: [5, 7, 9]
“`
Alternatively, using `map()`:
“`python
result = list(map(lambda x, y: x + y, list1, list2))
print(result) Output: [5, 7, 9]
“`
Table of Addition Examples
Data Type | Example | Output |
---|---|---|
Integer | 5 + 3 | 8 |
Float | 2.5 + 4.5 | 7.0 |
String | “Hello” + ” World” | Hello World |
List | [1, 2] + [3, 4] | [1, 2, 3, 4] |
This table illustrates the versatility of addition across different data types in Python, showcasing its applications in various contexts.
Basic Addition in Python
In Python, addition is performed using the `+` operator. This operator can be used with various data types, including integers, floats, and even strings for concatenation. Below are examples illustrating the basic addition of different types.
Adding Numbers
To add two numbers in Python, simply use the `+` operator between them.
“`python
Adding two integers
a = 5
b = 10
result = a + b
print(result) Output: 15
“`
For floating-point numbers, the process is the same:
“`python
Adding two floats
x = 2.5
y = 3.5
result = x + y
print(result) Output: 6.0
“`
Adding Multiple Numbers
You can add multiple numbers by chaining the `+` operator or using the `sum()` function for an iterable.
- Chaining Operators:
“`python
result = 1 + 2 + 3 + 4 + 5
print(result) Output: 15
“`
- Using the `sum()` Function:
“`python
numbers = [1, 2, 3, 4, 5]
result = sum(numbers)
print(result) Output: 15
“`
Adding Mixed Data Types
Python allows addition of integers and floats without any issues. However, adding strings requires both operands to be strings.
- Valid Example:
“`python
int_num = 5
float_num = 10.0
result = int_num + float_num
print(result) Output: 15.0
“`
- String Concatenation:
“`python
str1 = “Hello ”
str2 = “World”
result = str1 + str2
print(result) Output: Hello World
“`
- Invalid Mixed Addition:
Attempting to add an integer and a string will result in a `TypeError`.
“`python
int_num = 5
str_num = “10”
Uncommenting the next line will raise an error
result = int_num + str_num TypeError: unsupported operand type(s) for +: ‘int’ and ‘str’
“`
Using Addition with User Input
You can also perform addition with user input by converting the input values to the appropriate data type.
“`python
Taking input from the user
num1 = input(“Enter first number: “)
num2 = input(“Enter second number: “)
Converting to float for addition
result = float(num1) + float(num2)
print(“The sum is:”, result)
“`
Table of Addition Examples
Operation | Code Example | Output |
---|---|---|
Add two integers | `5 + 10` | `15` |
Add two floats | `2.5 + 3.5` | `6.0` |
Chain multiple nums | `1 + 2 + 3 + 4 + 5` | `15` |
Use `sum()` | `sum([1, 2, 3])` | `6` |
Add int and float | `5 + 10.0` | `15.0` |
Concatenate strings | `”Hello ” + “World”` | `Hello World` |
This structured approach to addition in Python allows for versatility in handling various data types and user inputs effectively.
Expert Insights on Performing Addition in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, performing addition is straightforward and intuitive. You simply use the ‘+’ operator between two numbers, which can be integers or floats. This simplicity is one of the reasons Python is favored for educational purposes and rapid prototyping.”
Michael Chen (Lead Data Scientist, Analytics Solutions Group). “When working with addition in Python, it’s essential to understand the data types involved. Python dynamically handles type conversion, but being aware of the types can prevent unexpected results, especially when adding lists or other data structures.”
Lisa Thompson (Software Engineer, CodeCraft Academy). “For beginners, I recommend practicing addition in Python using interactive environments like Jupyter Notebooks. This allows for immediate feedback and helps solidify understanding of how addition works within the language.”
Frequently Asked Questions (FAQs)
How do I perform addition in Python?
To perform addition in Python, you can use the `+` operator. For example, `result = 5 + 3` will store the value `8` in the variable `result`.
Can I add different data types in Python?
Yes, you can add integers, floats, and strings. However, adding integers and floats will yield a float, while adding strings will concatenate them. For example, `5 + 3.0` results in `8.0`, and `’Hello’ + ‘ World’` results in `’Hello World’`.
What happens if I try to add incompatible types in Python?
If you attempt to add incompatible types, such as an integer and a string, Python will raise a `TypeError`. For instance, `5 + ‘3’` will result in an error because these types cannot be combined.
Can I use the `sum()` function for addition in Python?
Yes, the `sum()` function can be used to add elements of an iterable, such as a list or tuple. For example, `sum([1, 2, 3])` will return `6`.
Is it possible to add numbers from user input in Python?
Yes, you can add numbers from user input by using the `input()` function and converting the input to integers or floats. For example:
“`python
num1 = float(input(“Enter first number: “))
num2 = float(input(“Enter second number: “))
result = num1 + num2
“`
How can I add elements of a list in Python?
You can add elements of a list using the `sum()` function or a loop. For example, using `sum(my_list)` will return the total of all the elements in `my_list`. Alternatively, you can use a for loop to iterate through the list and accumulate the total.
In Python, performing addition is a straightforward task that can be accomplished using the plus sign (+) operator. This operator can be utilized to add integers, floats, and even strings, provided the data types are compatible. For instance, when adding two numbers, Python automatically handles the arithmetic operation, yielding a result that can be stored in a variable or printed directly to the console.
Additionally, Python’s flexibility allows for the addition of various data types. For example, when adding strings, Python concatenates them rather than performing arithmetic addition. This feature highlights the importance of understanding data types in Python to avoid type errors during operations. Furthermore, Python supports the addition of elements within data structures, such as lists and tuples, using functions like `sum()` to aggregate values efficiently.
In summary, addition in Python is a fundamental operation that can be executed with ease. By utilizing the appropriate operator and being mindful of data types, users can effectively perform addition across different contexts. This knowledge is essential for anyone looking to leverage Python for data manipulation or programming tasks.
Author Profile
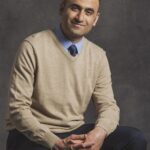
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?