How to Use ‘Not Equal’ in Python: A Comprehensive Guide
In the world of programming, comparisons are fundamental to making decisions and controlling the flow of applications. Whether you’re filtering data, validating user input, or implementing complex algorithms, understanding how to effectively compare values is crucial. In Python, one of the most common comparisons you’ll encounter is the “not equal” operation. This simple yet powerful concept can help you write cleaner, more efficient code, and mastering it can elevate your programming skills to new heights.
At its core, the “not equal” comparison in Python allows developers to determine when two values are distinct from one another. This functionality is essential for conditional statements, loops, and data manipulation, enabling you to create dynamic and responsive applications. By leveraging this operator, you can efficiently filter out unwanted data, ensure that conditions are met, and implement logic that reacts to the unique needs of your program.
As we delve deeper into the topic, we’ll explore the syntax and usage of the “not equal” operator, along with practical examples that illustrate its application in real-world scenarios. Whether you’re a beginner looking to grasp the basics or an experienced coder seeking to refine your skills, understanding how to do not equal in Python will undoubtedly enhance your programming toolkit.
Using the Not Equal Operator
In Python, the not equal comparison operator is represented by the symbols `!=`. This operator is used to compare two values or expressions, returning `True` if they are not equal and “ if they are equal. It is a fundamental aspect of control flow in Python, allowing developers to implement conditional logic based on inequality.
For example, consider the following code snippet:
“`python
a = 10
b = 20
if a != b:
print(“a and b are not equal.”)
else:
print(“a and b are equal.”)
“`
In this example, since `10` is not equal to `20`, the output will be “a and b are not equal.”
Equality vs. Inequality in Different Data Types
The not equal operator can be used across different data types, including integers, floats, strings, lists, and more. It is essential to understand how Python evaluates inequality for various data types, as the behavior may differ.
- Numbers: The comparison is straightforward; `!=` compares numerical values.
- Strings: Python compares string values lexicographically.
- Lists: Two lists are considered not equal if they contain different elements or are in a different order.
Here is a brief comparison of how the not equal operator works with various data types:
Data Type | Example | Result |
---|---|---|
Integer | 5 != 3 | True |
Float | 3.14 != 3.14 | |
String | “hello” != “world” | True |
List | [1, 2, 3] != [1, 2, 4] | True |
Common Use Cases for Not Equal
Using the not equal operator is a common practice in conditional statements, loops, and data validation scenarios. Some typical use cases include:
- Conditional Logic: Making decisions in `if` statements.
- Loops: Controlling loop execution based on conditions.
- Data Validation: Checking for unique values in data entries.
For instance, in a loop, you might want to continue processing until a certain condition is met:
“`python
value = 0
while value != 5:
print(value)
value += 1
“`
In this case, the loop will continue until `value` equals `5`, demonstrating how the not equal operator can control flow effectively.
Best Practices
When using the not equal operator, consider the following best practices:
- Use Clear Comparisons: Ensure that the expressions being compared are clear and unambiguous.
- Type Consistency: Be mindful of data types to avoid unexpected results, especially when comparing between different types.
- Readability: Maintain code readability by using descriptive variable names and avoiding overly complex comparisons.
By adhering to these guidelines, you can leverage the not equal operator effectively in your Python programming.
Using the Not Equal Operator in Python
In Python, the not equal comparison is achieved using the `!=` operator. This operator is fundamental for comparing two values and determining if they are different. If the values are not equal, the expression evaluates to `True`; otherwise, it returns “.
Examples of Not Equal Comparison
Here are some practical examples demonstrating how the `!=` operator works:
“`python
Example 1: Comparing integers
a = 5
b = 10
result = a != b True, because 5 is not equal to 10
Example 2: Comparing strings
str1 = “Hello”
str2 = “World”
result = str1 != str2 True, because “Hello” is not equal to “World”
Example 3: Comparing lists
list1 = [1, 2, 3]
list2 = [1, 2, 4]
result = list1 != list2 True, because the lists are not identical
“`
Using Not Equal in Conditional Statements
The not equal operator is often used within conditional statements to control the flow of a program. Below is an illustration of its application in an `if` statement:
“`python
user_input = input(“Enter a number: “)
if user_input != “0”:
print(“You entered a non-zero value.”)
else:
print(“You entered zero.”)
“`
In this example, the program checks if the user input is not equal to zero and responds accordingly.
Comparing Objects
The `!=` operator can also be used to compare objects. Python evaluates the inequality of objects based on their content and identity:
“`python
class Person:
def __init__(self, name):
self.name = name
def __eq__(self, other):
return self.name == other.name
Create two Person objects
person1 = Person(“Alice”)
person2 = Person(“Bob”)
Check if they are not equal
result = person1 != person2 True, because their names are different
“`
The implementation of the `__eq__` method allows for customized equality checks, which can affect the behavior of the `!=` operator.
Using Not Equal in Data Structures
When working with data structures like lists or dictionaries, the not equal operator can be beneficial for comparisons. Below are some examples:
- Lists: Check if two lists contain different elements.
“`python
list_a = [1, 2, 3]
list_b = [1, 2, 3]
result = list_a != list_b , both lists are equal
“`
- Dictionaries: Determine if two dictionaries have the same key-value pairs.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘a’: 1, ‘b’: 3}
result = dict1 != dict2 True, because the values for key ‘b’ differ
“`
Common Mistakes
When using the `!=` operator, consider the following common pitfalls:
- Using `is` instead of `!=`: The `is` operator checks for object identity, while `!=` checks for value inequality. Mixing these can lead to unexpected results.
- Comparing incompatible types: Comparing objects of different types can yield unexpected behavior. For instance, comparing a string with an integer will always return `True`.
“`python
result = “100” != 100 True, because they are different types
“`
By understanding these aspects of the not equal operator in Python, you can effectively implement it in your coding practices, ensuring more reliable and readable code.
Understanding Inequality in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the ‘!=’ operator is used to denote inequality. This operator allows developers to compare two values, returning True if they are not equal and otherwise. It is essential for control flow and data validation in programming.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “When working with data structures like lists or dictionaries, using ‘!=’ can help filter out unwanted values efficiently. Understanding how to implement this operator effectively can enhance the performance of your Python applications.”
Sarah Lopez (Data Scientist, Analytics Hub). “In data analysis, the ‘!=’ operator is crucial for cleaning datasets. It allows analysts to identify and exclude specific entries that do not meet certain criteria, thus ensuring the accuracy of their results.”
Frequently Asked Questions (FAQs)
What operator is used for not equal in Python?
The operator used for not equal in Python is `!=`. It compares two values and returns `True` if they are not equal and “ otherwise.
Can I use the `<>` operator for not equal in Python?
No, the `<>` operator is not valid in Python for not equal. Python exclusively uses `!=` for this comparison.
How do I check if two strings are not equal in Python?
To check if two strings are not equal, use the expression `string1 != string2`. This will return `True` if the strings differ and “ if they are the same.
Is there a way to perform a not equal comparison with numbers in Python?
Yes, you can use the `!=` operator to compare numbers. For example, `5 != 3` evaluates to `True`, while `5 != 5` evaluates to “.
Can I use not equal in conditional statements in Python?
Yes, you can use the not equal operator in conditional statements. For instance, `if a != b:` will execute the block of code if `a` is not equal to `b`.
Are there any alternative methods to check for inequality in Python?
While the primary method is using `!=`, you can also use the `operator` module, specifically `operator.ne(a, b)`, which serves the same purpose for checking inequality.
In Python, the concept of “not equal” is represented by the operator `!=`. This operator is used to compare two values or expressions, returning `True` if they are not equal and “ if they are equal. Understanding how to use this operator is fundamental for implementing conditional logic in programming, as it allows developers to control the flow of their code based on comparisons.
Additionally, Python provides alternative ways to express inequality, such as using the `<>` operator in older versions of Python (prior to Python 3). However, it is important to note that `<>` is deprecated in Python 3 and should be avoided in modern code. Consequently, using `!=` is the standard and recommended approach for checking inequality in current Python programming practices.
Furthermore, the `!=` operator can be applied to various data types, including integers, strings, lists, and custom objects. This versatility makes it a powerful tool in Python, enabling developers to create complex conditions and logic in their applications. Properly utilizing the not equal operator is essential for effective programming, as it enhances decision-making capabilities within the code.
Author Profile
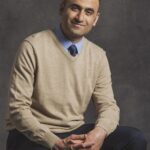
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?