How Can You Use Exponents in Python? A Beginner’s Guide
In the realm of programming, mastering the art of exponentiation is a fundamental skill that can unlock a world of possibilities. Whether you’re a budding coder or a seasoned developer, understanding how to perform calculations involving powers is essential for a variety of applications, from simple mathematical problems to complex algorithms. In Python, a language celebrated for its readability and simplicity, the process of raising numbers to a power is both straightforward and versatile.
Exponentiation in Python can be approached in several ways, each offering unique advantages depending on the context of your work. At its core, this operation allows you to manipulate numbers in a way that can lead to powerful results, whether you’re working with integers, floats, or even more complex data types. As you delve deeper into the world of Python, you’ll discover that exponentiation is not just a mathematical operation; it’s a gateway to exploring more advanced concepts such as data analysis, machine learning, and scientific computing.
In this article, we will explore the various methods to perform exponentiation in Python, highlighting the syntax and functionality that make it an indispensable tool in your programming toolkit. From built-in operators to specialized functions, you’ll learn how to harness the power of exponents effectively, setting the stage for more intricate programming challenges ahead. So, whether you’re
Using the Exponentiation Operator
In Python, the simplest way to perform exponentiation is by using the double asterisk operator `**`. This operator raises the number on the left to the power of the number on the right. Here are a few examples to illustrate its usage:
“`python
result = 2 ** 3 This calculates 2 raised to the power of 3, which equals 8
“`
You can also use negative and fractional exponents:
“`python
negative_result = 2 ** -2 This calculates 2 raised to the power of -2, which equals 0.25
fractional_result = 9 ** 0.5 This calculates the square root of 9, which equals 3
“`
Using the `pow()` Function
Another way to perform exponentiation in Python is by using the built-in `pow()` function. This function takes two or three arguments: the base, the exponent, and an optional modulus. The syntax is as follows:
“`python
pow(base, exp[, mod])
“`
- base: The number to be raised.
- exp: The exponent to raise the base to.
- mod (optional): If provided, the result will be calculated modulo this value.
Here are examples demonstrating its use:
“`python
result = pow(2, 3) This equals 8
mod_result = pow(2, 3, 3) This equals 2, as (2**3) % 3 = 2
“`
Comparative Table of Methods
The following table summarizes the two methods of exponentiation in Python:
Method | Syntax | Example | Output |
---|---|---|---|
Exponentiation Operator | base ** exp | 2 ** 3 | 8 |
Built-in pow() Function | pow(base, exp) | pow(2, 3) | 8 |
pow() with Modulus | pow(base, exp, mod) | pow(2, 3, 3) | 2 |
Performance Considerations
When it comes to performance, the `**` operator and the `pow()` function are generally equivalent for simple calculations. However, the `pow()` function can be more efficient when dealing with very large integers, especially when using the modulus argument.
- Use `**` for quick and straightforward exponentiation.
- Opt for `pow()` when performing calculations involving modular arithmetic or when working with large numbers.
By understanding these methods, you can effectively utilize exponentiation in your Python programming tasks.
Using the Exponentiation Operator
In Python, the simplest way to perform exponentiation, or raising a number to a power, is to use the exponentiation operator `**`. This operator allows for straightforward syntax and easy readability.
Syntax:
“`python
result = base ** exponent
“`
Example:
“`python
result = 2 ** 3 This will yield 8
“`
Utilizing the `pow()` Function
Another method to calculate the power of a number is through the built-in `pow()` function. This function can be especially useful when you need to include a modulus in your calculation.
Syntax:
“`python
result = pow(base, exponent, modulus)
“`
Example:
“`python
result = pow(2, 3) This will yield 8
result_mod = pow(2, 3, 3) This will yield 2 (8 mod 3)
“`
Working with Floating Point Numbers
Both the `**` operator and the `pow()` function can handle floating point numbers seamlessly. This allows for the calculation of fractional powers.
Example:
“`python
result_float = 9 ** 0.5 This will yield 3.0
result_pow_float = pow(27, 1/3) This will yield 3.0
“`
Performance Considerations
When choosing between the `**` operator and the `pow()` function, consider the following aspects:
Method | Pros | Cons |
---|---|---|
`**` | Simple and clear syntax | Cannot handle modulus |
`pow()` | Can handle modulus, more versatile | Slightly less readable |
For most applications, the difference in performance is negligible, but if you’re working in a performance-critical context, testing both methods may be warranted.
Handling Negative Exponents
Python also supports negative exponents, which will yield the reciprocal of the base raised to the absolute value of the exponent.
Example:
“`python
result_negative = 2 ** -3 This will yield 0.125
result_pow_negative = pow(2, -3) This will also yield 0.125
“`
Complex Numbers and Exponentiation
Python’s exponentiation capabilities extend to complex numbers as well. The `**` operator and `pow()` function can be used to raise complex numbers to a power.
Example:
“`python
complex_result = (1 + 2j) ** 3 This will yield (-11+2j)
“`
In summary, Python provides a robust set of tools for performing exponentiation, whether using the `**` operator or the `pow()` function. Each method has its unique advantages, allowing for flexibility depending on the specific needs of your calculations.
Understanding Exponential Operations in Python
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). “In Python, you can perform exponentiation using the `**` operator, which is a straightforward and efficient way to raise a number to a power. For instance, `2 ** 3` will yield `8`, demonstrating how Python simplifies mathematical operations.”
Mark Thompson (Data Scientist, Analytics Solutions). “Utilizing the `pow()` function is another excellent method for exponentiation in Python. This function not only raises a number to a power but also allows for an optional modulus argument, which can be particularly useful in data analysis and cryptography.”
Linda Garcia (Python Educator, Code Academy). “When teaching Python, I emphasize the importance of understanding both the `**` operator and the `pow()` function. Each has its own advantages depending on the context, and mastering these will greatly enhance a programmer’s ability to handle mathematical computations effectively.”
Frequently Asked Questions (FAQs)
How do you raise a number to a power in Python?
You can raise a number to a power in Python using the exponentiation operator ``. For example, `result = base exponent` computes the value of `base` raised to the `exponent`.
What is the alternative way to perform exponentiation in Python?
An alternative way to perform exponentiation is by using the built-in `pow()` function. For instance, `result = pow(base, exponent)` achieves the same result as using the `**` operator.
Can you use negative exponents in Python?
Yes, negative exponents are supported in Python. For example, `result = base ** -exponent` computes the reciprocal of the base raised to the positive exponent.
Is there a difference between `**` and `pow()` in Python?
Both `` and `pow()` perform exponentiation, but `pow()` can take a third argument for modulus, allowing you to compute `(base exponent) % modulus` efficiently.
How do you handle complex numbers when raising to a power in Python?
Python natively supports complex numbers. You can raise a complex number to a power using either `**` or `pow()`, and Python will handle the calculations appropriately.
What happens if you raise a number to the power of zero in Python?
In Python, any non-zero number raised to the power of zero returns `1`. This follows the mathematical principle that any non-zero number raised to zero equals one.
In Python, performing exponentiation, or raising a number to a power, can be accomplished using two primary methods: the exponentiation operator `` and the built-in `pow()` function. The `` operator is straightforward and commonly used for its simplicity, allowing users to express calculations like `base ** exponent` directly. This method is intuitive and aligns well with mathematical notation, making it easy for developers to read and understand the code.
Alternatively, the `pow()` function provides additional functionality, as it can take three arguments: `pow(base, exponent, modulus)`. This allows for modular exponentiation, which is particularly useful in scenarios involving large numbers or cryptographic applications. By using this function, developers can efficiently compute powers while also applying a modulus, thereby keeping the results manageable and within specific bounds.
Both methods are efficient and widely used in Python programming. When choosing between them, developers should consider the context of their calculations. For straightforward exponentiation, the `**` operator is often preferred for its clarity. However, when modular arithmetic is required, the `pow()` function becomes the more suitable option. Understanding these tools enables Python programmers to perform mathematical operations effectively and enhances their ability to write efficient code.
Author Profile
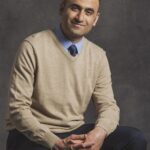
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?