How Can You Create Stunning Travel Stamps Using Python?
Travel stamps evoke a sense of adventure and nostalgia, capturing the essence of journeys taken and memories made. For artists and programmers alike, the idea of recreating these iconic symbols digitally can be both a creative challenge and a rewarding experience. If you’ve ever wanted to blend your love for travel with your programming skills, learning how to draw travel stamps using Python is an exciting way to do just that. In this article, we’ll explore the fundamentals of creating stunning travel stamp designs through the power of Python’s graphical libraries, allowing you to bring your travel experiences to life in a unique and artistic way.
Drawing travel stamps in Python involves harnessing the capabilities of various libraries that facilitate graphic design and image manipulation. From simple shapes and lines to more complex designs, Python provides a versatile platform for artists and developers to express their creativity. Whether you’re a seasoned programmer or a beginner eager to learn, the process can be both educational and enjoyable. You’ll discover how to create stamps that reflect different cultures, landmarks, and personal experiences, all while honing your coding skills.
As we delve deeper into the world of digital design, we’ll cover the essential tools and techniques needed to create your own travel stamps. You’ll learn about the various libraries available in Python, such as Turtle,
Setting Up Your Environment
To draw travel stamps in Python, you will need a suitable environment that supports graphical rendering. The most commonly used libraries for this purpose include Matplotlib and Pygame. Here’s how to set them up:
- Matplotlib: Primarily used for plotting graphs, it can also create simple graphics like stamps.
- Pygame: A more robust library for creating games and multimedia applications, allowing for more complex graphics.
You can install these libraries using pip:
“`bash
pip install matplotlib pygame
“`
Creating a Basic Stamp with Matplotlib
Using Matplotlib, you can create a simple travel stamp design by utilizing its plotting capabilities. Below is an example code snippet that demonstrates how to draw a rectangular stamp with a circular design inside.
“`python
import matplotlib.pyplot as plt
import numpy as np
Create a figure
plt.figure(figsize=(6,4))
Draw a rectangle to represent the stamp
rectangle = plt.Rectangle((0, 0), 5, 3, color=’lightblue’, ec=’blue’, lw=2)
plt.gca().add_patch(rectangle)
Draw a circle inside the rectangle
circle = plt.Circle((2.5, 1.5), 1, color=’white’, ec=’blue’, lw=2)
plt.gca().add_patch(circle)
Add text to the stamp
plt.text(2.5, 1.5, ‘Travel’, fontsize=12, ha=’center’, va=’center’, fontweight=’bold’)
Set limits and display
plt.xlim(-1, 6)
plt.ylim(-1, 4)
plt.axis(‘off’) Turn off the axis
plt.show()
“`
This code creates a basic rectangular stamp with a circular design in the middle, showcasing the word “Travel.”
Enhancing Your Travel Stamp
To make your stamp design more intricate, consider adding features such as borders, additional text, or images. Below are some techniques to enhance your stamp:
- Borders: Use multiple rectangles with varying colors and widths.
- Images: Import images using `matplotlib.image` to add logos or landmarks.
- Custom Fonts: Use libraries like `matplotlib.font_manager` to customize text styles.
Here’s an enhanced example demonstrating these concepts:
“`python
import matplotlib.pyplot as plt
import numpy as np
plt.figure(figsize=(6,4))
Draw stamp border
for i in range(3):
plt.gca().add_patch(plt.Rectangle((-0.1-i*0.1, -0.1-i*0.1), 5.2+i*0.2, 3.2+i*0.2,
color=’blue’, ec=’none’, lw=2))
Main stamp area
rectangle = plt.Rectangle((0, 0), 5, 3, color=’lightblue’, ec=’blue’, lw=2)
plt.gca().add_patch(rectangle)
Add a circle
circle = plt.Circle((2.5, 1.5), 1, color=’white’, ec=’blue’, lw=2)
plt.gca().add_patch(circle)
Add text
plt.text(2.5, 1.5, ‘Visit Paris’, fontsize=15, ha=’center’, va=’center’, fontweight=’bold’)
plt.xlim(-1, 6)
plt.ylim(-1, 4)
plt.axis(‘off’)
plt.show()
“`
Utilizing Pygame for Dynamic Stamps
Pygame allows for more dynamic and interactive designs. Here’s how you can create a simple travel stamp in Pygame:
“`python
import pygame
import sys
Initialize Pygame
pygame.init()
screen = pygame.display.set_mode((500, 300))
pygame.display.set_caption(‘Travel Stamp’)
Colors
lightblue = (173, 216, 230)
blue = (0, 0, 255)
Main loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255)) Background
pygame.draw.rect(screen, lightblue, (50, 50, 400, 200)) Stamp
pygame.draw.circle(screen, blue, (250, 150), 50) Circle
font = pygame.font.SysFont(‘Arial’, 30)
text = font.render(‘Travel’, True, blue)
screen.blit(text, (200, 135))
pygame.display.flip()
“`
This code sets up a Pygame window and draws a travel stamp with a rectangle and circle, displaying the text “Travel.”
Library | Use Case | Complexity |
---|---|---|
Matplotlib | Static graphics and simple shapes | Low |
Pygame | Dynamic graphics and games | Medium |
Setting Up Your Environment
To begin drawing travel stamps in Python, ensure you have the necessary libraries installed. The most commonly used library for drawing and graphical output in Python is `matplotlib`. Install it using pip if you haven’t done so yet:
“`bash
pip install matplotlib
“`
Basic Drawing Functions
Using `matplotlib`, you can leverage its functionality to create basic shapes that mimic travel stamps. Here are some essential functions:
- `plt.Rectangle()`: Creates rectangles for the main body of the stamp.
- `plt.Circle()`: Useful for rounded edges or seals.
- `plt.text()`: Adds text to the stamps, such as location names and dates.
Creating a Simple Travel Stamp
Here is a step-by-step example of how to create a simple travel stamp using `matplotlib`:
“`python
import matplotlib.pyplot as plt
Create a new figure
fig, ax = plt.subplots()
Draw the main rectangle of the stamp
rect = plt.Rectangle((0.1, 0.1), 0.8, 0.5, color=’lightblue’, ec=’black’, linewidth=2)
ax.add_patch(rect)
Add a circle to simulate a seal
circle = plt.Circle((0.5, 0.6), 0.1, color=’red’, ec=’black’, linewidth=2)
ax.add_patch(circle)
Add text to the stamp
ax.text(0.5, 0.65, ‘Travel Stamp’, fontsize=12, ha=’center’, color=’white’)
ax.text(0.5, 0.4, ‘Paris, France’, fontsize=10, ha=’center’, color=’black’)
Set limits and aspect
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
ax.set_aspect(‘equal’)
ax.axis(‘off’) Hide axes
Show the stamp
plt.show()
“`
Customizing the Stamp
You can enhance your travel stamp by customizing colors, fonts, and additional shapes. Here are some customization options:
- Colors: Use hex color codes or predefined color names.
- Fonts: Utilize different font styles and sizes by adding parameters in `plt.text()`.
- Shapes: Combine various shapes to create unique designs.
Advanced Features
To create more intricate designs, consider the following advanced techniques:
- Images: Add images as a background or element of the stamp using `plt.imread()`.
“`python
img = plt.imread(‘path_to_image.png’)
ax.imshow(img, extent=[0.1, 0.9, 0.1, 0.5], aspect=’auto’)
“`
- Custom Shapes: Use `matplotlib.patches` to create custom shapes, like stars or waves, enhancing the overall aesthetic.
Final Touches
To finalize your travel stamp, consider exporting it to a file format like PNG or PDF. Use the following command:
“`python
plt.savefig(‘travel_stamp.png’, bbox_inches=’tight’, dpi=300)
“`
This command ensures that the output is high quality and retains the design integrity when shared or printed.
By utilizing these techniques, you can create visually appealing travel stamps tailored to your preferences, showcasing creativity through Python programming.
Expert Insights on Drawing Travel Stamps in Python
Jessica Hartman (Lead Software Developer, Creative Coding Solutions). “To effectively draw travel stamps in Python, utilizing libraries such as Pillow for image manipulation and Matplotlib for visual representation is essential. These tools allow for high customization and facilitate the creation of unique stamp designs.”
Michael Tran (Graphic Designer and Python Enthusiast). “Incorporating vector graphics through libraries like Turtle can enhance the drawing experience. By leveraging Python’s capabilities to create shapes and patterns, one can simulate the intricate designs often found in travel stamps.”
Linda Chen (Python Programming Instructor, Tech Academy). “I recommend starting with basic shapes and gradually adding details to your travel stamps. Using object-oriented programming principles can also help in organizing your code effectively, making it easier to manage complex designs.”
Frequently Asked Questions (FAQs)
What libraries can I use to draw travel stamps in Python?
You can use libraries such as Matplotlib, Pillow, or Turtle Graphics to create and design travel stamps in Python. Each library has unique features that can help you achieve different visual styles.
How can I create a circular stamp shape using Python?
To create a circular stamp shape, you can use the Matplotlib library’s `Circle` class or the `ellipse` method in Pillow. Set the appropriate parameters for the radius and position to achieve the desired circular effect.
What are some design elements to include in a travel stamp?
Common design elements for travel stamps include iconic landmarks, country names, dates, and decorative borders. You can also incorporate symbols or graphics that represent the location.
Can I add text to my travel stamp design in Python?
Yes, you can add text to your travel stamp using the `text` function in Matplotlib or the `ImageDraw` module in Pillow. Specify the font size, type, and position to customize the text appearance.
How do I save my travel stamp design as an image file?
In Matplotlib, you can save your design using the `savefig` function. In Pillow, use the `save` method on your image object. Choose the desired file format, such as PNG or JPEG, for saving.
Is it possible to animate travel stamps in Python?
Yes, you can create simple animations of travel stamps using libraries like Matplotlib’s `FuncAnimation` or Pygame. These libraries allow you to update the stamp’s properties over time to create dynamic visual effects.
drawing travel stamps in Python involves utilizing various libraries such as Matplotlib, Pillow, or Turtle to create visually appealing graphics. Each library offers unique functionalities that cater to different aspects of graphic design, allowing users to customize shapes, colors, and textures effectively. Understanding the basic principles of graphic design, such as composition and color theory, can significantly enhance the quality of the travel stamps created.
Additionally, leveraging Python’s capabilities for image manipulation and drawing can lead to the creation of intricate designs that mimic real travel stamps. By combining geometric shapes, text, and images, users can replicate the aesthetic qualities of authentic stamps. Tutorials and resources available online can provide step-by-step guidance, making the learning process more accessible for beginners.
Ultimately, the journey of drawing travel stamps in Python not only fosters creativity but also enhances programming skills. Engaging with the community through forums and sharing creations can further inspire innovation. As users experiment with different techniques and styles, they can develop a unique artistic voice that reflects their personal experiences and adventures.
Author Profile
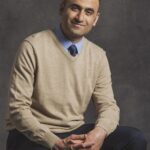
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?