How Can You Easily Drop a Column in Python?
In the world of data manipulation, the ability to efficiently manage and transform datasets is an essential skill for any aspiring data scientist or analyst. One common task that often arises when working with data is the need to drop a column. Whether you’re cleaning up a messy dataset, removing redundant information, or simply focusing on the most relevant features for your analysis, knowing how to drop a column in Python can streamline your workflow and enhance your data processing capabilities.
Python, with its robust libraries like Pandas, offers powerful tools to handle dataframes with ease. Dropping a column can be a straightforward process, but understanding the nuances of different methods and their implications can significantly impact your data analysis journey. As you delve deeper into the world of data manipulation, you’ll discover various techniques that not only allow you to remove unwanted columns but also help you maintain the integrity and structure of your dataset.
In this article, we will explore the various approaches to dropping columns in Python, highlighting best practices and potential pitfalls. Whether you’re a beginner looking to grasp the basics or an experienced user seeking to refine your skills, this guide will provide you with the knowledge and confidence to manage your data more effectively. Get ready to enhance your data manipulation toolkit and unlock new possibilities in your analytical endeavors!
Using Pandas to Drop a Column
The most common method for dropping a column in Python is by using the Pandas library. Pandas provides a straightforward way to manipulate data structures, particularly DataFrames. To drop a column, you can utilize the `drop()` method, which allows you to specify the column you wish to remove.
Here is the syntax for dropping a column:
“`python
DataFrame.drop(columns=’column_name’, inplace=True)
“`
Key parameters of the `drop()` method include:
- `columns`: Specifies the name or names of the columns to drop.
- `inplace`: If set to `True`, the operation modifies the original DataFrame without returning a new one. If “, a new DataFrame is returned.
Example usage:
“`python
import pandas as pd
Sample DataFrame
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [24, 27, 22],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
Dropping the ‘Age’ column
df.drop(columns=’Age’, inplace=True)
print(df)
“`
After executing the above code, the DataFrame will look like this:
Name | City |
---|---|
Alice | New York |
Bob | Los Angeles |
Charlie | Chicago |
Dropping Multiple Columns
To drop multiple columns simultaneously, you can pass a list of column names to the `columns` parameter. This is particularly useful when you need to clean your DataFrame by removing several unnecessary columns at once.
Example:
“`python
Dropping multiple columns
df.drop(columns=[‘City’, ‘Name’], inplace=True)
print(df)
“`
The resulting DataFrame will be empty since all columns have been dropped:
Dropping Columns with Conditions
In some cases, you may want to drop columns based on specific conditions, such as if they contain all null values or if their data type is not suitable for analysis. To achieve this, you can use boolean indexing along with the `drop()` method.
Example of dropping columns with all NaN values:
“`python
Sample DataFrame with NaN values
data_with_nan = {
‘Name’: [‘Alice’, None, ‘Charlie’],
‘Age’: [24, None, None],
‘City’: [‘New York’, None, ‘Chicago’]
}
df_nan = pd.DataFrame(data_with_nan)
Dropping columns where all values are NaN
df_nan.dropna(axis=1, how=’all’, inplace=True)
print(df_nan)
“`
This code will output the DataFrame without any columns that contain only NaN values:
Name | City |
---|---|
Alice | New York |
Charlie | Chicago |
Conclusion on Column Dropping
Dropping columns in Python using Pandas is an efficient way to manage and clean your data. Whether you need to remove one or multiple columns or filter them based on conditions, Pandas provides flexible methods to accomplish these tasks effectively.
Methods to Drop a Column in Python
In Python, particularly when working with data manipulation libraries such as Pandas, dropping a column can be accomplished through several efficient methods. Below are the most commonly used approaches:
Using Pandas `drop()` Method
The `drop()` method is one of the most straightforward ways to remove a column from a DataFrame. The syntax is as follows:
“`python
import pandas as pd
Creating a sample DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
})
Dropping a column
df_dropped = df.drop(‘B’, axis=1)
“`
- Parameters:
- `labels`: The column name(s) to drop.
- `axis`: Set to `1` for columns; `0` is for rows.
- `inplace`: If `True`, the operation is performed in place without returning a new DataFrame.
Dropping Multiple Columns
To drop multiple columns simultaneously, pass a list of column names:
“`python
df_dropped = df.drop([‘A’, ‘C’], axis=1)
“`
Using the `del` Keyword
Another method to drop a column is by using the `del` keyword, which directly deletes a specified column:
“`python
del df[‘B’]
“`
This modifies the original DataFrame without the need for an additional assignment.
Using the `pop()` Method
The `pop()` method removes a column and returns it as a Series. This can be useful if you want to keep a copy of the dropped column:
“`python
b_column = df.pop(‘B’)
“`
- After this operation, `b_column` will contain the values from column ‘B’, and it will no longer be part of `df`.
Using `loc` with Boolean Indexing
You can also drop columns by selecting the columns you want to keep. This method is particularly useful when you have a large DataFrame and want to drop multiple columns based on a condition:
“`python
df_filtered = df.loc[:, df.columns != ‘B’]
“`
This approach is flexible as it allows for dynamic selection based on conditions.
Example of Dropping Columns
Here’s a complete example showcasing different methods to drop columns from a DataFrame:
“`python
import pandas as pd
Sample DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
})
Method 1: Using drop
df1 = df.drop(‘B’, axis=1)
Method 2: Using del
del df[‘A’]
Method 3: Using pop
c_column = df.pop(‘C’)
Method 4: Using loc
df2 = df.loc[:, df.columns != ‘B’]
“`
Each of these methods can be selected based on the specific needs of your data manipulation task, providing a flexible toolkit for DataFrame management in Python.
Expert Insights on Dropping Columns in Python
Dr. Emily Chen (Data Scientist, Analytics Innovations). “When dropping a column in Python, particularly with pandas, it is crucial to ensure that the column is not essential for subsequent analysis. The method `DataFrame.drop()` allows for both in-place modification and the creation of a new DataFrame, providing flexibility depending on your workflow.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “Using the `drop()` function in pandas is straightforward, but understanding the parameters such as `axis` and `inplace` is vital. Setting `axis=1` indicates that you are dropping a column, while `inplace=True` modifies the original DataFrame directly, which can be beneficial for memory management.”
Sarah Patel (Machine Learning Engineer, Data Dynamics). “In practice, it is often a good idea to check for any dependencies before dropping a column. Utilizing the `isnull()` function can help identify if the column contains significant missing values, which may influence the decision to remove it from your dataset.”
Frequently Asked Questions (FAQs)
How do I drop a column from a pandas DataFrame in Python?
To drop a column from a pandas DataFrame, use the `drop()` method with the column name and set the `axis` parameter to 1. For example: `df.drop(‘column_name’, axis=1, inplace=True)`.
Can I drop multiple columns at once in pandas?
Yes, you can drop multiple columns by passing a list of column names to the `drop()` method. For example: `df.drop([‘column1’, ‘column2’], axis=1, inplace=True)`.
What does the `inplace` parameter do in the drop method?
The `inplace` parameter determines whether to modify the original DataFrame directly or return a new DataFrame with the specified columns removed. Setting `inplace=True` modifies the original DataFrame.
Is it possible to drop a column based on a condition?
Yes, you can drop a column based on a condition by first filtering the DataFrame and then using the `drop()` method. For example, you can check for null values and drop columns accordingly.
How can I check if a column exists before dropping it?
You can check if a column exists using the `in` operator with the DataFrame’s columns attribute. For example: `if ‘column_name’ in df.columns: df.drop(‘column_name’, axis=1, inplace=True)`.
What happens if I try to drop a column that does not exist?
If you attempt to drop a column that does not exist, pandas will raise a `KeyError`. You can avoid this by using the `errors=’ignore’` parameter in the `drop()` method.
In Python, dropping a column from a DataFrame is a common operation, particularly when using the Pandas library. The most straightforward method to achieve this is by utilizing the `drop()` function, which allows users to specify the column to be removed. This can be done by passing the column name along with the `axis` parameter set to 1, indicating that a column is being dropped rather than a row. It is also possible to drop multiple columns by providing a list of column names.
Another important aspect to consider is whether to modify the original DataFrame or create a new one. By default, the `drop()` function returns a new DataFrame without the specified column(s), leaving the original DataFrame unchanged. However, users can set the `inplace` parameter to `True` if they wish to alter the original DataFrame directly. This flexibility allows for better control over data manipulation processes.
Additionally, when dropping columns, it is crucial to ensure that the specified columns exist in the DataFrame to avoid errors. The `errors` parameter in the `drop()` function can be set to ‘ignore’ to prevent the function from raising an error if a column is not found. This feature can be particularly useful when working
Author Profile
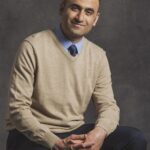
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?