How Can You Efficiently Empty an Array in JavaScript?
### Introduction
In the dynamic world of JavaScript programming, managing data efficiently is a crucial skill for developers. Arrays, as one of the fundamental data structures, play a pivotal role in storing and manipulating collections of data. However, there are times when you may find yourself needing to clear an array, whether to reset its contents for new data, optimize memory usage, or simply to ensure that old values do not interfere with new operations. Understanding how to effectively empty an array can enhance your coding practices and improve the performance of your applications.
In JavaScript, there are several methods to empty an array, each with its own nuances and implications. From leveraging built-in array methods to utilizing simple assignments, developers have a variety of tools at their disposal. It’s essential to grasp not only the mechanics of these methods but also the contexts in which they are most effective. Whether you’re a seasoned programmer or just starting your journey in coding, mastering the art of array manipulation will undoubtedly empower you to write cleaner, more efficient code.
As we delve deeper into the topic, we will explore the different approaches to emptying an array in JavaScript, examining their advantages and potential pitfalls. By the end of this article, you will have a comprehensive understanding of how to handle arrays with confidence, ensuring that your
Methods to Empty an Array
To empty an array in JavaScript, several techniques can be employed, each with its own implications. Below are the most commonly used methods, along with a description of their effects on the original array reference.
Setting Length to Zero
One of the simplest ways to empty an array is to set its length property to zero. This method effectively removes all elements from the array while maintaining the original reference.
javascript
let myArray = [1, 2, 3, 4];
myArray.length = 0; // myArray is now []
Using the Splice Method
The `splice` method can be used to remove elements from an array, allowing for more control over which elements to remove. To empty an entire array, you can specify the starting index as 0 and the number of elements to remove as the array’s current length.
javascript
let myArray = [1, 2, 3, 4];
myArray.splice(0, myArray.length); // myArray is now []
Reassigning to a New Array
Another approach is to reassign the variable to a new empty array. While this is effective for clearing the array, it does not affect any other references to the original array.
javascript
let myArray = [1, 2, 3, 4];
myArray = []; // myArray is now [] but the original reference is lost
Using Pop in a Loop
If you want to empty an array while also performing operations on each element, you can use a loop to call `pop()` until the array is empty. This method is less efficient but allows for additional processing.
javascript
let myArray = [1, 2, 3, 4];
while (myArray.length > 0) {
let element = myArray.pop();
// Perform operations with element
}
Comparison of Methods
The following table summarizes the different methods to empty an array, highlighting their effects on the original array reference.
Method | Effects on Original Reference | Complexity |
---|---|---|
Setting length to 0 | Affects all references | O(1) |
Using splice | Affects all references | O(n) |
Reassigning to a new array | Does not affect other references | O(1) |
Using pop in a loop | Affects all references | O(n) |
Each method has its advantages and use cases depending on the specific needs of the application. Choose the method that best aligns with your requirements for performance and reference handling.
Methods to Empty an Array in JavaScript
There are several effective methods to empty an array in JavaScript, each with its own characteristics and use cases. Below are the most commonly used techniques:
Using the Length Property
One of the simplest ways to empty an array is by setting its `length` property to zero. This method is efficient and straightforward.
javascript
let arr = [1, 2, 3, 4, 5];
arr.length = 0;
console.log(arr); // Output: []
Using the Splice Method
The `splice` method can also be employed to remove all elements from an array. This method modifies the original array and is particularly useful when you want to remove elements from a specific index.
javascript
let arr = [1, 2, 3, 4, 5];
arr.splice(0, arr.length);
console.log(arr); // Output: []
Using the Pop Method in a Loop
Another approach is to repeatedly call the `pop` method until the array is empty. This method is less efficient due to the iterative nature but can be useful for specific scenarios.
javascript
let arr = [1, 2, 3, 4, 5];
while (arr.length > 0) {
arr.pop();
}
console.log(arr); // Output: []
Reassigning to a New Array
You can also reassign the variable holding the array to a new empty array. This method is particularly useful if there are no other references to the original array. However, it will not affect other references pointing to the original array.
javascript
let arr = [1, 2, 3, 4, 5];
arr = [];
console.log(arr); // Output: []
Using the Filter Method
The `filter` method can be used to create a new array based on a condition. To empty the array, you can filter it with a condition that always evaluates to .
javascript
let arr = [1, 2, 3, 4, 5];
arr = arr.filter(() => );
console.log(arr); // Output: []
Comparative Overview of Methods
Method | Modifies Original Array | Performance | Use Case |
---|---|---|---|
Length Property | Yes | Fast | General-purpose emptying |
Splice | Yes | Medium | Removing elements from a range |
Pop in Loop | Yes | Slow | Specific removal scenarios |
Reassigning | No | Fast | When no references to original |
Filter | No | Medium | Creating a new empty array |
Each method has its advantages and disadvantages, and the choice of which to use depends on the specific requirements of your code, including performance considerations and whether you need to maintain references to the original array.
Expert Insights on Emptying Arrays in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “To effectively empty an array in JavaScript, one of the most efficient methods is to set its length property to zero. This approach is not only straightforward but also ensures that any references to the original array remain intact, which is crucial in maintaining data integrity.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “While there are multiple ways to empty an array, using the splice method can be particularly useful when you need to remove elements from a specific index. By calling array.splice(0, array.length), you can clear the array while also ensuring that any associated references are updated accordingly.”
Sarah Thompson (JavaScript Educator, Code Academy). “It is important to understand the implications of the method you choose to empty an array. For instance, assigning a new empty array (e.g., array = []) creates a new reference, which may lead to unexpected behavior if other variables reference the original array. Thus, developers should consider their specific use case when deciding how to empty an array.”
Frequently Asked Questions (FAQs)
How can I empty an array in JavaScript?
You can empty an array in JavaScript by setting its length property to zero, like this: `array.length = 0;`. This method is efficient and maintains the reference to the original array.
Is there a method to remove all elements from an array?
Yes, you can use the `splice()` method to remove all elements from an array. For example, `array.splice(0, array.length);` will remove all elements starting from index 0.
Can I create a new empty array instead of modifying the existing one?
Absolutely. You can create a new empty array by simply declaring it: `let newArray = [];`. This does not affect the original array.
What happens to references if I empty an array?
If you empty an array by setting its length to zero or using `splice()`, all references to that array will see the change. However, creating a new array does not affect any existing references to the original array.
Are there performance differences between methods of emptying an array?
Yes, setting the length to zero is generally the most performant method, as it directly modifies the array’s internal structure. Using `splice()` can be slower due to the overhead of element removal.
Can I use the `filter()` method to empty an array?
While `filter()` can be used to create a new array with specific conditions, it is not an efficient way to empty an array. Instead, use methods like setting length or `splice()` for this purpose.
Emptying an array in JavaScript can be achieved through several methods, each with its own implications and use cases. The most common techniques include setting the array’s length to zero, reassigning the array to a new empty array, and using the `splice` method. Each of these methods effectively removes all elements from the array but may differ in terms of performance and reference handling, particularly when the array is referenced elsewhere in the code.
One of the simplest and most efficient ways to empty an array is by setting its length property to zero. This method is straightforward and preserves the reference to the original array, making it a preferred choice when other parts of the code rely on the same array object. Alternatively, reassigning the array to a new empty array creates a new reference, which may be beneficial in certain contexts but can lead to issues if the original reference is still in use.
The `splice` method is another viable option, allowing for the removal of elements from the array starting from index zero. While this method is effective, it may be less efficient than simply setting the length to zero, especially for large arrays. Understanding these different approaches enables developers to choose the most appropriate method for their specific use case, ensuring optimal performance
Author Profile
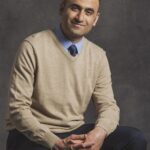
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?