How Can You Effectively Empty an Array in JavaScript?
In the dynamic world of JavaScript, arrays play a pivotal role in managing collections of data. Whether you’re developing a simple web application or a complex software solution, understanding how to manipulate these arrays is crucial for efficient coding. One common task that developers often encounter is the need to empty an array. While this may seem straightforward, there are multiple techniques to achieve this, each with its own implications for performance and memory management. In this article, we will explore the various methods available for emptying an array in JavaScript, helping you choose the best approach for your specific use case.
When it comes to emptying an array in JavaScript, there are several strategies at your disposal. From reassigning the array to a new empty array to manipulating its length property, each method has its own nuances that can affect how your code behaves. Additionally, understanding the implications of these methods on references and memory can help you avoid potential pitfalls in your applications. As we delve into this topic, we’ll also discuss best practices and considerations to keep in mind when working with arrays, ensuring that you not only know how to empty them but also when to use each technique effectively.
By the end of this article, you will have a comprehensive understanding of the various ways to clear an array in JavaScript,
Methods to Empty an Array in JavaScript
To empty an array in JavaScript, there are several methods available, each with its own characteristics and use cases. Below are the most common techniques.
Setting Length to Zero
One of the simplest and most effective ways to empty an array is to set its `length` property to zero. This method is direct and efficient.
javascript
let arr = [1, 2, 3, 4];
arr.length = 0;
console.log(arr); // Output: []
This method modifies the original array and is widely used due to its simplicity.
Using the Splice Method
The `splice` method can also be employed to remove all elements from an array. This method is particularly useful if you want to remove elements selectively or need to maintain references to the original array.
javascript
let arr = [1, 2, 3, 4];
arr.splice(0, arr.length);
console.log(arr); // Output: []
The `splice` method takes two arguments: the starting index and the number of elements to remove. By specifying `0` as the starting index and the array’s length as the number of elements to remove, the entire array is emptied.
Reassigning to a New Array
Another approach is to reassign the variable to a new empty array. This method is straightforward but note that if other references to the original array exist, they will not be affected.
javascript
let arr = [1, 2, 3, 4];
arr = []; // arr now points to a new empty array
console.log(arr); // Output: []
This method is beneficial in cases where you do not need to maintain the original reference.
Using the Filter Method
You can also use the `filter` method to create a new array based on a condition. To empty an array, you can provide a condition that is never met.
javascript
let arr = [1, 2, 3, 4];
arr = arr.filter(() => );
console.log(arr); // Output: []
This method is less common for simply emptying an array, but it demonstrates the use of functional programming techniques.
Comparison of Array Emptying Methods
The table below summarizes the various methods for emptying an array, highlighting their characteristics.
Method | Impact on Original Array | Performance |
---|---|---|
Setting Length to Zero | Yes | High |
Splice Method | Yes | Medium |
Reassigning to a New Array | No | High |
Filter Method | No | Low |
Each method has its unique advantages and should be chosen based on the specific requirements of your code.
Methods to Empty an Array in JavaScript
There are several effective techniques to empty an array in JavaScript, each suitable for different scenarios. Below are the most commonly used methods:
Using Length Property
Setting the length of an array to zero is a straightforward way to empty it. This method is efficient and modifies the original array.
javascript
let array = [1, 2, 3, 4];
array.length = 0; // Array is now empty
Using Splice Method
The `splice()` method can remove elements from an array. By specifying the start index as `0` and the count as the array’s length, you can effectively clear the array.
javascript
let array = [1, 2, 3, 4];
array.splice(0, array.length); // Array is now empty
Using Pop in a Loop
While less efficient, you can continuously use `pop()` to remove elements until the array is empty. This method is generally not recommended for large arrays due to performance concerns.
javascript
let array = [1, 2, 3, 4];
while (array.length) {
array.pop(); // Removes elements one by one
}
Assigning a New Array
You can also replace the current array with a new empty array. This approach does not affect references to the original array.
javascript
let array = [1, 2, 3, 4];
array = []; // Original reference is lost, new array is created
Comparative Summary of Methods
Method | Modifies Original Array | Performance | Use Case |
---|---|---|---|
Length Property | Yes | Fast | When you want to retain the same reference |
Splice Method | Yes | Fast | When you need to remove elements in bulk |
Pop in a Loop | Yes | Slow | For educational purposes or small arrays |
Assigning a New Array | No | Instant | When you do not need to maintain references |
Considerations
- The choice of method may depend on whether you need to maintain references to the original array.
- If performance is a concern, prefer methods that modify the array in place, such as using the length property or `splice()`.
- Be cautious with methods that involve loops for larger arrays, as they can lead to inefficiencies.
Expert Insights on Emptying Arrays in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively empty an array in JavaScript, the most straightforward method is to set its length property to zero. This approach is efficient and ensures that all references to the original array are maintained.”
Michael Chen (JavaScript Developer Advocate, CodeCraft Academy). “Using the splice method is another effective technique for emptying an array. By calling array.splice(0, array.length), you can remove all elements while also ensuring that the original array reference remains intact.”
Sarah Patel (Lead Frontend Developer, Web Solutions Group). “For modern JavaScript, utilizing the array destructuring assignment with an empty array is a clean and elegant way to clear an array. This method not only empties the array but also enhances code readability.”
Frequently Asked Questions (FAQs)
How can I empty an array in JavaScript?
You can empty an array by setting its length property to zero: `array.length = 0;`. This method effectively removes all elements from the array.
Is there a method to clear an array without modifying the original variable?
Yes, you can create a new empty array and assign it to the variable. For example: `array = [];`. This approach does not modify the original array but reassigns the variable to a new empty array.
What is the difference between using `array.length = 0` and `array.splice(0)`?
Both methods effectively empty the array, but `array.splice(0)` can also be used to remove elements from the array while returning the removed elements, whereas setting `array.length` to zero does not return any elements.
Can I use the `pop()` method in a loop to empty an array?
Yes, you can use a loop with `pop()` to remove elements one by one until the array is empty. However, this method is less efficient than setting the length to zero or using `splice()`.
Is there a performance difference between the various methods to empty an array?
Yes, setting the length to zero is generally the most efficient method, as it directly modifies the array’s length property. Using `splice()` or a loop with `pop()` may incur more overhead due to multiple operations.
Will emptying an array affect references to it in other parts of my code?
If you use `array.length = 0`, it will affect all references to that array since it modifies the original array. If you create a new array with `array = []`, only the variable pointing to that array is affected, leaving other references unchanged.
In JavaScript, there are several effective methods to empty an array, each with its own use case and implications. The most common approaches include setting the array’s length property to zero, using the splice method, and reassigning the array to a new empty array. Each of these methods can be utilized depending on the specific requirements of the code and the desired outcomes, such as whether references to the original array should be maintained or discarded.
Setting the length property of an array to zero is a straightforward and efficient way to clear an array. This method modifies the original array in place, ensuring that any references to the array remain intact. Conversely, using the splice method allows for more granular control by removing elements from the array while also maintaining references. Reassigning the array to a new empty array is another option, but it effectively breaks references to the original array, which may not be desirable in all situations.
the choice of method for emptying an array in JavaScript should be guided by the specific context and requirements of the application. Understanding the implications of each approach is crucial for effective coding practices. By selecting the appropriate method, developers can ensure that their code remains efficient, clean, and functional.
Author Profile
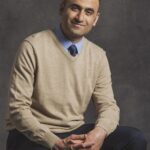
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?