How Do You Properly End a Code in Python?
In the world of programming, knowing how to effectively manage the flow of your code is crucial for creating efficient and functional applications. Python, with its elegant syntax and versatile capabilities, allows developers to write clean and readable code, but understanding how to properly end a code segment is just as important as knowing how to start one. Whether you’re wrapping up a simple script or finalizing a complex project, mastering the techniques for ending your code can significantly impact its performance and maintainability.
Ending a code in Python involves more than just hitting the stop button; it’s about ensuring that your program concludes gracefully and handles any necessary cleanup tasks. This may include closing files, releasing resources, or even returning values to the calling function. Additionally, Python provides various constructs, such as functions, classes, and exception handling, that can influence how you conclude your code effectively. By leveraging these features, you can enhance the robustness of your applications and prevent potential issues that may arise from abrupt terminations.
As you delve deeper into this topic, you’ll discover the best practices for structuring your code endings, the importance of using return statements in functions, and how to implement proper error handling to ensure your program exits smoothly. Understanding these concepts will not only improve your coding skills but also prepare you for more advanced programming challenges ahead
Using the `exit()` Function
The `exit()` function is a straightforward way to terminate a Python program. It raises the `SystemExit` exception, which can be caught by surrounding code if necessary. This function can be used to end your script gracefully. The exit status can also be specified, where a status of zero typically indicates success, while any non-zero status indicates an error.
“`python
import sys
def main():
print(“This program will exit now.”)
sys.exit(0)
main()
“`
Utilizing the `quit()` and `exit()` Commands
Similar to `exit()`, the `quit()` command can also be employed to terminate a Python program. Both functions serve the same purpose, but `quit()` is more commonly used in interactive environments, such as the Python shell. Here’s how you might see it in use:
“`python
print(“Ending the program with quit.”)
quit()
“`
Using the `os._exit()` Method
For scenarios requiring immediate termination of a program without cleanup, the `os._exit()` method can be utilized. This function is more abrupt than `exit()` or `quit()`, as it does not raise the `SystemExit` exception, and thus, does not invoke any cleanup handlers or flush standard I/O buffers.
“`python
import os
def terminate_program():
print(“Terminating immediately.”)
os._exit(1)
terminate_program()
“`
Returning from the Main Function
In scripts, simply returning from the main function will also exit the program. This is a common practice in Python, especially when the script is structured with a main function.
“`python
def main():
print(“Ending the program via return.”)
return
if __name__ == “__main__”:
main()
“`
Handling Exceptions to End a Program
Another method to conclude a Python program is through exception handling. By raising an exception, you can control the flow of your program and end it whenever necessary.
“`python
def risky_function():
raise Exception(“An error occurred. Ending the program.”)
try:
risky_function()
except Exception as e:
print(e)
“`
Comparison of Termination Methods
The following table summarizes the various methods of ending a Python program, highlighting their differences and appropriate contexts for use:
Method | Description | Use Case |
---|---|---|
exit() | Raises SystemExit | Graceful exit with optional status |
quit() | Similar to exit(), interactive use | Used in REPL environments |
os._exit() | Immediate exit, no cleanup | Critical failure scenarios |
Return from main | Ends execution naturally | Standard script execution |
Raise Exception | Triggers error handling | Controlled exit on error |
Using the `exit()` Function
The `exit()` function, part of the `sys` module, is commonly used to terminate a Python program. It takes an optional integer argument that indicates the exit status, where 0 usually signifies a successful termination, and any non-zero value indicates an error.
“`python
import sys
Terminate the program with a success status
sys.exit(0)
Terminate the program with an error status
sys.exit(1)
“`
Using the `quit()` and `exit()` Built-ins
Python also provides the `quit()` and `exit()` built-in functions, which can be used interchangeably to exit a program. These functions are primarily intended for interactive sessions and may not be suitable for production code.
“`python
Using quit() to end the program
quit()
Using exit() to end the program
exit()
“`
Raising SystemExit Exception
Another method to terminate a program is by raising the `SystemExit` exception. This approach offers more flexibility, allowing you to include a message or status code upon termination.
“`python
raise SystemExit(“Ending the program due to an error.”)
“`
Using Return Statements in Functions
When working within functions, a return statement can be used to exit the function and, consequently, the program if the function is the main entry point.
“`python
def main():
print(“Program is running.”)
return Exits the function
main()
“`
Keyboard Interrupts
In a long-running program, users can manually terminate execution using a keyboard interrupt, typically by pressing `Ctrl + C`. This raises a `KeyboardInterrupt` exception which can be caught and handled gracefully.
“`python
try:
while True:
pass Simulating a long-running task
except KeyboardInterrupt:
print(“Program terminated by user.”)
“`
Using the `os._exit()` Function
For a more immediate termination of a program, you can use `os._exit()`. This function exits the program without cleaning up, which means that it does not call any cleanup handlers or flush standard I/O buffers.
“`python
import os
Forcing an exit with no cleanup
os._exit(0)
“`
Exiting in Scripts vs. Interactive Sessions
It is important to note that the method of exiting may vary depending on whether the code is running in a script or an interactive shell. Here’s a comparison:
Context | Recommended Exit Method |
---|---|
Script | `sys.exit()` or `os._exit()` |
Interactive Session | `quit()` or `exit()` |
By understanding these various methods, you can appropriately choose how to end your Python code based on the context and requirements of your application.
Effective Strategies for Concluding Code in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the end of a code block is determined by indentation rather than explicit end statements. It is crucial to maintain consistent indentation to ensure that the code executes correctly and to avoid syntax errors.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “To effectively conclude a Python script, one should utilize the `if __name__ == ‘__main__’:` construct. This practice allows for better modularity and prevents certain code from executing when the script is imported as a module.”
Sarah Thompson (Python Educator and Author). “Ending a code in Python can also involve proper resource management. Utilizing context managers with the `with` statement ensures that files and network connections are closed appropriately, which is an essential part of writing clean and efficient Python code.”
Frequently Asked Questions (FAQs)
How do I properly end a Python script?
To properly end a Python script, ensure that all necessary cleanup operations are performed, such as closing files or releasing resources. You can use the `exit()` function or simply let the script reach its end naturally.
What is the purpose of the `exit()` function in Python?
The `exit()` function is used to terminate a Python program. It can take an optional integer argument that indicates the exit status, with `0` typically representing a successful termination.
Can I use `sys.exit()` to end a Python program?
Yes, `sys.exit()` can be used to terminate a Python program. It raises a `SystemExit` exception, which can be caught in outer layers of the program if necessary. Import the `sys` module to use this function.
What happens if I don’t end a Python script?
If a Python script does not end properly, it may leave resources open, such as files or network connections, which can lead to memory leaks or other unintended behavior.
Is it necessary to use a return statement to end a function in Python?
While it is not strictly necessary to use a return statement to end a function, doing so allows you to specify a value to return to the caller. If no return statement is provided, the function will return `None` by default.
Can I use a keyboard interrupt to stop a running Python script?
Yes, you can use a keyboard interrupt (usually Ctrl+C) to stop a running Python script. This raises a `KeyboardInterrupt` exception, which can be caught to perform cleanup operations before termination.
In Python, ending a code segment typically refers to the conclusion of a script or the termination of a program. Python does not require a specific command to end a program, as it automatically concludes when the interpreter reaches the end of the script. However, developers can use the `exit()` or `sys.exit()` functions to terminate a program explicitly. This can be particularly useful in scenarios where an early exit is needed based on certain conditions or error handling.
Additionally, when working with loops or functions, the `break` statement can be employed to exit a loop prematurely, while the `return` statement serves to exit a function and optionally pass back a value. Understanding these mechanisms allows developers to control the flow of their programs effectively, ensuring that they can terminate execution as needed based on the logic of their code.
In summary, while Python scripts conclude automatically at the end of the file, developers have various tools at their disposal to manage program termination actively. Utilizing functions like `exit()`, `break`, and `return` enhances the control over program flow, allowing for more robust and error-resilient code. Mastery of these concepts is essential for writing effective Python programs.
Author Profile
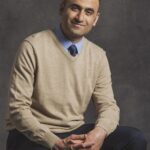
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?