How Can You Effectively End a Program in Python?
### Introduction
In the world of programming, knowing how to effectively manage your code is just as important as writing it. Whether you’re a seasoned developer or a novice just starting your coding journey, understanding how to end a program in Python is a crucial skill that can enhance your coding efficiency and prevent unnecessary errors. Just like a well-crafted story needs a satisfying conclusion, a Python program requires a clean exit to ensure that resources are properly released and that the program behaves as expected. In this article, we will explore the various methods and best practices for gracefully terminating your Python programs, ensuring that you can leave your code on a high note.
Ending a program in Python may seem straightforward, but it encompasses a variety of techniques and considerations. From using built-in functions to handling exceptions, the way you conclude your program can significantly impact its performance and reliability. You’ll discover how to use commands like `exit()` and `sys.exit()`, as well as the importance of closing files and connections to avoid resource leaks. Additionally, we’ll touch on how to manage unexpected terminations and the role of exception handling in ensuring a clean exit.
As we delve deeper into this topic, you’ll gain insights into not just the mechanics of ending a program, but also the underlying principles that guide effective coding practices. By mastering
Using the `exit()` Function
The simplest way to terminate a Python program is by using the `exit()` function from the `sys` module. This function allows you to exit from Python and can take an optional exit status, where a status of `0` typically indicates a successful termination, while any non-zero status indicates an error.
python
import sys
# Some code
sys.exit(0) # Exits the program with a status of 0
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can be used to terminate a Python program. This function is essentially a synonym for `exit()` and is commonly used in interactive sessions. It is important to note that both `quit()` and `exit()` are intended primarily for use in the interactive shell.
python
# Some code
quit() # Exits the program
Raising the `SystemExit` Exception
Another method to end a program is by raising the `SystemExit` exception. This can be useful when you want to exit from a specific point in your code, potentially after performing some cleanup or logging.
python
raise SystemExit(“Exiting the program”)
Using the `os._exit()` Function
For situations where you need to exit without cleanup, you can use the `os._exit()` function. This function terminates the process immediately, bypassing any cleanup actions such as the execution of `try`/`finally` blocks or calling the `atexit` handlers.
python
import os
# Some code
os._exit(0) # Exits immediately with status 0
Exiting from Loops and Functions
When you want to exit from nested loops or functions rather than the entire program, you can use the `break` statement or return statements, respectively.
- Breaking from a loop: The `break` statement allows you to exit the nearest enclosing loop.
python
for i in range(10):
if i == 5:
break # Exits the loop
- Returning from a function: Use the `return` statement to exit a function and optionally send a value back to the caller.
python
def my_function():
return 42 # Exits the function and returns 42
Comparison of Exit Methods
The following table summarizes the exit methods discussed above:
Method | Description | Cleanup |
---|---|---|
sys.exit() | Exits the program with optional exit status | Yes |
quit() | Exits the program (primarily for interactive sessions) | Yes |
raise SystemExit | Raises an exception to exit | Yes |
os._exit() | Immediately exits the program | No |
Each method has its specific use case, and the choice of which one to use depends on the context of your program and the desired behavior upon termination.
Using the `exit()` Function
The `exit()` function is a straightforward way to terminate a Python program. It can be used in scripts or interactive environments. When called, it raises the `SystemExit` exception, which can be caught if needed.
- Basic Usage:
python
import sys
sys.exit()
- Exiting with a Status Code:
You can provide an integer status code to indicate if the program ended successfully or with an error.
python
sys.exit(0) # Success
sys.exit(1) # Error
Using the `quit()` Function
Similar to `exit()`, the `quit()` function serves as an alternative for terminating a script. It is primarily intended for interactive use and functions in the same way, raising a `SystemExit` exception.
- Example:
python
quit() # Ends the program
Raising `SystemExit` Directly
You can also terminate a program by raising the `SystemExit` exception directly. This approach allows for more custom handling if you need to perform additional cleanup tasks before exiting.
- Example:
python
raise SystemExit(0) # Normal exit
Using `os._exit()` for Immediate Termination
In some scenarios, you may want to immediately terminate a program without calling cleanup handlers or flushing output buffers. The `os._exit()` function can be used for this purpose.
- Example:
python
import os
os._exit(0) # Exits immediately
Using Keyboard Interrupts
A Python program can be terminated manually by the user through keyboard interrupts. This typically occurs when the user presses `Ctrl + C` in the terminal.
- Catching Keyboard Interrupt:
You can handle this interrupt gracefully using a try-except block.
python
try:
# Your code here
except KeyboardInterrupt:
print(“Program interrupted.”)
sys.exit(0)
Using Conditionals to Control Program Flow
You can structure your program to exit based on specific conditions. This method allows for a more controlled shutdown of the application.
- Example:
python
if some_condition:
print(“Condition met, exiting.”)
sys.exit()
Using `atexit` Module for Cleanup Actions
The `atexit` module allows you to register cleanup functions that will be called upon normal program termination. This can be useful for resource management or logging.
- Example:
python
import atexit
def cleanup():
print(“Cleanup actions here.”)
atexit.register(cleanup)
By utilizing these methods, Python programs can be terminated gracefully or abruptly, depending on the needs of the application and the context in which it operates. Each option provides flexibility to control the shutdown process effectively.
Expert Insights on Ending a Program in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively end a program in Python, one must utilize the `sys.exit()` function from the `sys` module. This method allows for a clean termination of the program and can include an exit status code to indicate success or failure.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Using `raise SystemExit` is another robust approach to terminate a Python program. This method provides flexibility, allowing developers to raise an exception that can be caught or ignored, depending on the program’s flow.”
Sara Patel (Python Instructor, Coding Academy). “For beginners, I recommend using the `exit()` function, which is more intuitive. However, it is essential to understand that `exit()` is a convenience function that ultimately calls `sys.exit()`, so grasping the underlying mechanics is beneficial for deeper programming knowledge.”
Frequently Asked Questions (FAQs)
How can I terminate a Python program gracefully?
You can terminate a Python program gracefully by using the `sys.exit()` function from the `sys` module. This allows you to exit the program and optionally provide an exit status code.
What is the purpose of the `exit()` function in Python?
The `exit()` function is a built-in function that raises the `SystemExit` exception, which can be used to terminate the program. It can also accept an optional argument to indicate the exit status.
Can I use a keyboard interrupt to stop a Python program?
Yes, you can use a keyboard interrupt (Ctrl+C) to stop a running Python program. This raises a `KeyboardInterrupt` exception, which can be caught and handled if needed.
What happens if I do not end a Python program properly?
If a Python program does not end properly, it may leave resources open, such as files or network connections, leading to potential memory leaks or data corruption.
Is there a way to exit a Python script based on a condition?
Yes, you can use conditional statements (like `if` statements) combined with `sys.exit()` to exit a script based on specific conditions being met.
How can I stop an infinite loop in Python?
To stop an infinite loop in Python, you can use a `break` statement within the loop when a certain condition is met, or you can interrupt the program execution using Ctrl+C.
Ending a program in Python can be accomplished through various methods, each serving different purposes depending on the context of the application. The most straightforward way to terminate a program is by using the built-in `exit()` function or the `sys.exit()` method from the `sys` module. Both methods effectively stop the execution of the program and can take an optional exit status code, with `0` typically indicating a successful termination.
Another method to end a program is through the use of exceptions. By raising an exception, such as `SystemExit`, a program can be terminated gracefully, allowing for cleanup operations or logging before the program exits. Additionally, in interactive environments or when running scripts, using keyboard interrupts (like Ctrl+C) can also halt the execution of a program, providing a quick way to stop processes without modifying the code.
It is essential to choose the appropriate method for ending a program based on the specific requirements and context. For instance, using `sys.exit()` is more suitable for scripts that require a clean exit with a specific status, while exceptions can be beneficial in more complex applications where resource management is crucial. Understanding these different approaches enables developers to write more robust and maintainable Python code.
Author Profile
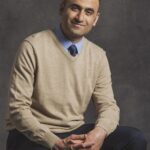
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?