How Can You Effectively End a Python Program?
Introduction
In the world of programming, knowing how to effectively manage the lifecycle of your applications is crucial. Whether you’re developing a simple script or a complex software system, there comes a time when you need to gracefully end a Python program. Understanding the various methods to terminate a program not only enhances your coding skills but also ensures that resources are properly released and that your application behaves as expected. In this article, we will explore the different techniques for concluding a Python program, providing you with the knowledge to implement them seamlessly in your projects.
When it comes to ending a Python program, there are several approaches you can take, each suited to different scenarios. From using built-in functions to handling exceptions, the methods available allow for flexibility and control over how your program exits. It’s essential to consider the implications of each approach, as they can affect the overall performance and reliability of your application. Whether you’re looking to terminate a program under normal conditions or in response to an error, understanding these techniques will empower you to write more robust code.
Additionally, we’ll touch on best practices for exiting a program, including how to ensure that any necessary cleanup operations are performed before the program closes. This is particularly important in larger applications where resources such as files, network connections, or databases may need to be properly
Using the exit() Function
The `exit()` function is a straightforward way to terminate a Python program. It raises the `SystemExit` exception, which can be caught in the outermost code if necessary. By default, `exit()` returns a status code of 0, indicating a successful termination. You can also provide a specific exit status to indicate different outcomes.
- Basic Usage:
python
exit()
- Custom Exit Status:
python
exit(1) # Indicates an error or abnormal termination
Using the sys.exit() Method
Another common method to end a Python program is by using `sys.exit()`, which is part of the `sys` module. This function behaves similarly to `exit()`, but it is often preferred in scripts and larger applications.
- Importing sys:
Before using `sys.exit()`, ensure that you import the `sys` module at the beginning of your script:
python
import sys
sys.exit()
- Custom Exit Codes:
You can also specify a custom exit code, similar to the `exit()` function:
python
sys.exit(1)
Using Exception Handling
In some scenarios, particularly within try-except blocks, raising an exception can be an effective way to end a program. The `raise` statement allows you to signal an error condition.
- Example:
python
try:
# Code that may cause an error
raise Exception(“An error occurred”)
except Exception as e:
print(e)
sys.exit(1) # Terminate the program with an error code
Using os._exit() for Immediate Termination
For scenarios requiring immediate termination without cleanup, `os._exit()` can be utilized. This function terminates the program without calling cleanup handlers or flushing standard I/O buffers.
- Usage:
python
import os
os._exit(0) # Immediate termination
Comparison of Termination Methods
Here’s a comparison table to help you understand the differences between various methods of ending a Python program:
Method | Effect on Cleanup | Default Exit Code |
---|---|---|
exit() | Runs cleanup handlers | 0 |
sys.exit() | Runs cleanup handlers | 0 |
os._exit() | No cleanup | 0 |
Understanding the various methods for terminating a Python program allows developers to choose the most appropriate one based on the context. Whether handling exceptions, performing immediate exits, or managing cleanup processes, selecting the right approach can enhance the robustness and reliability of your code.
Methods to End a Python Program
In Python, there are several ways to terminate a program, each suitable for different scenarios. The most common methods include using the built-in functions, exception handling, and external modules.
Using the `exit()` Function
The `exit()` function from the `sys` module is a straightforward way to terminate a Python script. It allows you to end the program and return an exit status.
- Syntax: `exit([status])`
- Parameters:
- `status`: An optional integer that specifies the exit status. By convention, `0` indicates a successful termination, while any non-zero value indicates an error.
Example:
python
import sys
# Some code logic
if error_condition:
sys.exit(1) # Exiting with an error status
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can also be used to terminate a program. It is primarily intended for use in the interactive interpreter.
- Syntax: `quit()`
- Behavior: Exits the program and is equivalent to calling `sys.exit()`.
Example:
python
# Some code logic
if user_input == ‘exit’:
quit() # Exiting the program
Using the `raise` Statement
You can also terminate a program by raising an exception. This method is useful for indicating error conditions.
- Syntax: `raise Exception(‘message’)`
- Behavior: The program will stop executing at the point where the exception is raised unless it is caught by a try-except block.
Example:
python
# Some code logic
if critical_error:
raise Exception(“Critical error encountered!”) # Terminating the program
Using the `os._exit()` Function
For a more abrupt termination, the `os._exit()` function can be used. This function is less common and should be used with caution, as it does not clean up resources properly.
- Syntax: `os._exit(status)`
- Parameters:
- `status`: An integer exit status code.
Example:
python
import os
# Some code logic
if severe_issue:
os._exit(1) # Immediate exit without cleanup
Using the `sys.exit()` Function
The `sys.exit()` function is often used in scripts to terminate the program cleanly. It can also accept an exit code.
- Behavior: It raises the `SystemExit` exception, which can be caught in a try-except block.
Example:
python
import sys
# Some code logic
if not_valid_input:
sys.exit(“Exiting due to invalid input”) # Exiting with a message
Handling Keyboard Interrupts
You can gracefully handle keyboard interrupts (Ctrl+C) to allow users to exit the program safely.
Example:
python
try:
while True:
# Main program logic
pass
except KeyboardInterrupt:
print(“Program terminated by user.”)
These methods provide a comprehensive toolkit for managing how a Python program can be ended, allowing developers to choose the most appropriate approach based on their needs.
Expert Insights on Terminating Python Programs
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To effectively end a Python program, one can use the built-in function `sys.exit()`, which allows for graceful termination and can also take an exit status code. This is particularly useful in larger applications where you want to indicate success or failure to the operating system.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Another method to terminate a Python program is by using the `exit()` function, which is a more user-friendly approach, especially for beginners. However, it is important to note that `exit()` is not recommended for use in scripts run in the Python interpreter, as it may lead to unexpected behavior.”
Sarah Thompson (Lead Python Instructor, Code Academy). “For interactive sessions or scripts, using a simple `break` statement in loops or `return` in functions can also effectively end the program flow. It is essential to understand the context in which you are terminating the program to choose the most appropriate method.”
Frequently Asked Questions (FAQs)
How can I end a Python program using the exit() function?
You can end a Python program by calling the `exit()` function from the `sys` module. First, import the module using `import sys`, then call `sys.exit()` to terminate the program.
What is the purpose of the quit() function in Python?
The `quit()` function serves a similar purpose as `exit()`. It is a built-in function that can be used to terminate a Python program. It is primarily intended for use in interactive sessions.
Can I use the Ctrl+C keyboard shortcut to stop a Python program?
Yes, pressing Ctrl+C in the terminal where the Python program is running will raise a KeyboardInterrupt exception, effectively stopping the program execution.
Is there a way to end a Python program based on a specific condition?
Yes, you can use conditional statements to call `sys.exit()` or `quit()` when a specific condition is met, allowing you to terminate the program programmatically.
What happens if I don’t end a Python program properly?
If a Python program does not end properly, it may leave resources open, such as file handles or network connections, which can lead to memory leaks or other unintended behavior.
Can I use return statements to end a Python program?
Return statements can be used to exit from functions, but they do not terminate the entire program. To end the program, you should use `sys.exit()` or similar termination functions.
In summary, ending a Python program can be accomplished through several methods, each suitable for different scenarios. The most straightforward approach is to use the built-in function `exit()` or `sys.exit()`, which allows for a clean termination of the program. Alternatively, raising an exception can also serve as a means to exit, especially in cases where an error condition needs to be reported. Each method provides a way to control the program flow and ensure resources are properly released.
Another important aspect to consider is the context in which the program is running. For instance, in interactive environments like Jupyter notebooks, simply reaching the end of a cell will effectively end the execution of that cell. In contrast, command-line scripts may require explicit exit commands to terminate properly. Understanding the environment can influence the choice of termination method and enhance the overall program management.
Key takeaways include the importance of choosing the appropriate exit method based on the program’s requirements and the execution context. Utilizing `exit()` or `sys.exit()` is generally recommended for standard scripts, while exceptions can be useful for error handling. Additionally, being aware of the environment in which the program operates can aid in making informed decisions about program termination.
Author Profile
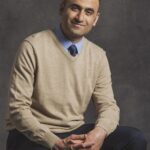
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?