How Do You Properly End an If Statement in Python?
In the world of programming, mastering the fundamentals is key to unlocking your potential as a developer. One of the foundational concepts in Python, a language celebrated for its simplicity and readability, is the use of conditional statements. Among these, the `if` statement stands out as a powerful tool that allows you to control the flow of your code based on specific conditions. But how do you effectively end an `if` statement in Python? Understanding this aspect is crucial for writing clean, efficient code that behaves as intended.
When working with `if` statements, it’s essential to grasp the structure that governs their functionality. An `if` statement begins with the keyword `if`, followed by a condition that evaluates to either true or . However, knowing how to properly conclude this statement is just as important as knowing how to initiate it. This involves understanding the indentation rules that Python enforces, which dictate how blocks of code are grouped together. The end of an `if` statement is marked not by a specific keyword, but rather by the return to a previous indentation level, signaling that the conditional block has concluded.
Additionally, Python allows for more complex conditional logic through the use of `elif` and `else` clauses, which can further extend the flow of control. Each of these components
Understanding Indentation in Python
In Python, the end of an `if` statement is determined by the indentation level. Unlike many other programming languages that use braces or keywords to denote blocks of code, Python relies on indentation to signify code structure. This means that any code that follows an `if` statement must be indented to indicate that it belongs to the conditional block.
- The standard practice is to use four spaces for indentation.
- Mixing tabs and spaces can lead to errors, so it’s advisable to stick to one method of indentation throughout your code.
When the code block for the `if` statement is complete, the next line that is not indented signifies the end of the `if` statement block.
Example of an If Statement
Below is a simple example demonstrating how to properly end an `if` statement in Python:
“`python
age = 20
if age >= 18:
print(“You are an adult.”)
print(“This line is outside the if statement.”)
“`
In this example, the `print(“You are an adult.”)` line is indented, which indicates it is part of the `if` block. The second print statement is not indented, signaling the end of the `if` block.
Multiple Conditions with Else and Elif
When an `if` statement is followed by `elif` and `else`, proper indentation is crucial for defining the relationship between these conditions. Each condition must be indented at the same level, and the block of code under each must also be consistently indented.
Here’s a table summarizing the structure of an `if` statement with multiple conditions:
Condition | Code Block |
---|---|
if condition: | code to execute if condition is True |
elif condition: | code to execute if previous conditions are and this condition is True |
else: | code to execute if all previous conditions are |
Best Practices for Ending If Statements
To ensure clarity and maintainability in your code, consider the following best practices when working with `if` statements:
- Always use a consistent number of spaces for indentation (commonly four).
- Avoid using tabs; instead, configure your text editor to convert tabs to spaces.
- Clearly comment your conditions to describe what each block does, enhancing readability.
- Keep blocks of code concise; if they grow too large, consider refactoring them into functions.
By following these guidelines, you can effectively manage `if` statements in Python, ensuring that your code is both functional and easy to understand.
Understanding the Structure of an If Statement
In Python, an `if` statement is used to evaluate a condition and execute a block of code if that condition is true. The syntax follows a simple structure:
“`python
if condition:
block of code to execute if condition is true
“`
To properly end an `if` statement, it is essential to understand how indentation signifies the scope of the statement. Python uses indentation to define blocks of code, which means the end of the `if` statement is determined by the return to a previous indentation level.
Ending an If Statement
An `if` statement is ended by the following mechanisms:
- Returning to the Previous Indentation Level: After the block of code that executes if the condition is true, any subsequent code that is not indented at the same level signals the end of the `if` block.
“`python
if condition:
This block runs if condition is true
print(“Condition is True”)
This line is outside of the if statement
print(“This line always runs”)
“`
- Using Elif and Else: An `if` statement can be extended with `elif` (else if) and `else` clauses. The end of the entire conditional structure is defined when the indentation returns to the previous level or when the structure is explicitly closed.
“`python
if condition:
print(“Condition is True”)
elif another_condition:
print(“Another condition is True”)
else:
print(“Neither condition is True”)
This line is outside of the if-elif-else structure
print(“This line always runs”)
“`
Common Mistakes to Avoid
To ensure that `if` statements are used correctly, consider the following common mistakes:
- Incorrect Indentation: Failing to maintain consistent indentation can lead to `IndentationError` or unexpected behavior. Python requires strict adherence to indentation.
- Misplaced Code: Placing code that should run unconditionally inside an `if` block can lead to logic errors.
- Lack of Else/Elif: Not utilizing `else` or `elif` when needed may lead to misunderstandings in code flow.
Example Scenarios
To illustrate the proper ending of an `if` statement, consider the following examples:
Scenario | Code Example |
---|---|
Simple If Statement | “`python if x > 10: print(“x is greater than 10”) print(“End of check”)“` |
If-Else Statement | “`python if x > 10: print(“x is greater than 10”) else: print(“x is 10 or less”) print(“End of check”)“` |
If-Elif-Else Statement | “`python if x > 10: print(“x is greater than 10”) elif x == 10: print(“x is exactly 10”) else: print(“x is less than 10”) print(“End of check”)“` |
These examples demonstrate how to properly structure an `if` statement and ensure it concludes correctly based on indentation and flow control structures.
Understanding the Conclusion of Conditional Statements in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, an if statement concludes with the indentation level returning to the previous block. This means that once the code within the if block is executed, the next line that is not indented will effectively end the if statement.”
Michael Chen (Python Programming Instructor, Code Academy). “It’s essential to remember that Python uses indentation to define the scope of an if statement. Therefore, the end is not marked by a specific keyword but rather by the return to the previous indentation level.”
Lisa Patel (Lead Developer, Python Solutions Group). “When writing Python code, the conclusion of an if statement is implicit. The block of code under the if condition will execute, and once the indentation level decreases, it signifies the end of that conditional block.”
Frequently Asked Questions (FAQs)
How do you end an if statement in Python?
An if statement in Python does not require an explicit end statement. It is concluded by the indentation level returning to the previous level or by the end of the block of code associated with the if statement.
What is the syntax for an if statement in Python?
The syntax for an if statement in Python is as follows:
“`python
if condition:
code to execute if condition is true
“`
Can you use an else statement after an if statement?
Yes, you can use an else statement after an if statement. The syntax is:
“`python
if condition:
code if condition is true
else:
code if condition is
“`
Is it possible to have multiple conditions in an if statement?
Yes, you can use `elif` to check multiple conditions. The structure is:
“`python
if condition1:
code if condition1 is true
elif condition2:
code if condition2 is true
else:
code if all conditions are
“`
What happens if there is no indentation after an if statement?
If there is no indentation after an if statement, Python will raise an `IndentationError`, as proper indentation is required to define the block of code associated with the if statement.
Can you nest if statements in Python?
Yes, you can nest if statements in Python. This allows for more complex decision-making structures. The syntax is:
“`python
if condition1:
if condition2:
code if both conditions are true
“`
In Python, an if statement is concluded simply by dedenting the code block that follows it. This means that once the indented block of code associated with the if condition is completed, any subsequent code that is not indented will be treated as outside the scope of the if statement. This structure allows for clear and organized code, making it easy to read and maintain.
It is important to note that Python uses indentation to define the blocks of code, rather than explicit keywords like ‘end’ as seen in some other programming languages. This design choice emphasizes readability and enforces a consistent coding style. Therefore, ensuring that the indentation is correct is crucial for the proper functioning of if statements and their corresponding blocks.
Key takeaways include the significance of proper indentation in Python, which not only concludes if statements but also defines the flow of control in the program. Understanding this concept is essential for writing effective Python code and avoiding common pitfalls associated with incorrect indentation. Mastery of these fundamentals will enhance your programming skills and contribute to the development of clean, efficient code.
Author Profile
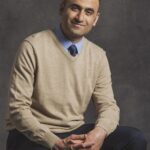
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?