How Do You Properly End a Code Block in Python?
Introduction
In the world of programming, knowing how to effectively manage the flow of your code is crucial for creating efficient and functional applications. One fundamental aspect of this is understanding how to end code in Python. Whether you’re wrapping up a simple script or concluding a complex function, knowing the right techniques can enhance your programming skills and improve the readability of your code. In this article, we will explore the various methods and best practices for signaling the end of your code, ensuring that your programs run smoothly and as intended.
When it comes to ending code in Python, it’s not just about knowing when to stop; it’s also about understanding the context in which your code operates. Python provides several mechanisms to conclude your scripts or functions gracefully, including the use of return statements, exit functions, and exception handling. Each method serves a distinct purpose and can be utilized in different scenarios, depending on your programming goals and the structure of your code.
Additionally, the way you end your code can significantly impact the overall performance and user experience of your application. By mastering the techniques for properly concluding your code, you can avoid common pitfalls such as memory leaks or incomplete processes. This article will delve into these strategies, offering insights that will empower you to write cleaner, more efficient Python code. Whether you’re a
Exiting Scripts Gracefully
To end a Python script gracefully, you can use the `sys.exit()` function from the `sys` module. This method allows you to terminate the program and can also return an exit status code to the operating system, indicating whether the program terminated successfully or encountered an error.
python
import sys
def main():
print(“Running the script…”)
# Your code logic here
sys.exit(0) # Exiting the script with a success status
if __name__ == “__main__”:
main()
The exit status codes typically follow these conventions:
- `0` – Success
- `1` – General error
- Other positive values may indicate specific error types.
Using Keyboard Interrupt
In situations where you need to stop a running script interactively, you can use the keyboard interrupt. Pressing `Ctrl + C` while the script is running will raise a `KeyboardInterrupt` exception. You can handle this exception within your code to perform any cleanup actions before exiting.
python
try:
while True:
pass # Simulating a long-running process
except KeyboardInterrupt:
print(“Script terminated by user.”)
Conditional Exit Statements
You may want to exit a script based on certain conditions. Using conditional statements allows for a more controlled exit. Here’s an example:
python
def check_condition(condition):
if condition:
print(“Condition met, exiting…”)
sys.exit(0)
else:
print(“Condition not met, continuing…”)
check_condition(True) # This will cause the script to exit
Exiting Functions Early
You can also exit from functions prematurely using the `return` statement. This is useful when you want to stop executing further lines within a function based on certain criteria.
python
def process_data(data):
if not data:
print(“No data provided, exiting function.”)
return # Exit the function early
# Continue processing data
Table of Exit Methods
The following table summarizes the methods for ending code execution in Python:
Method | Description |
---|---|
sys.exit() | Terminate the program and return an exit status code. |
KeyboardInterrupt | Manually stop the script using Ctrl + C. |
return | Exit from a function early without completing the rest of the code. |
raise | Trigger an exception to stop the program execution. |
By utilizing these methods, you can control the termination of your Python scripts effectively, ensuring that resources are freed and that your code exits in a manner that aligns with your program’s logic.
Graceful Termination of Python Code
In Python, it is often essential to end code execution gracefully to avoid data loss or corruption, especially when dealing with file operations or network connections. The following methods are commonly employed:
- Using `sys.exit()`: This function allows you to terminate a program based on conditions in your code.
python
import sys
if some_condition:
sys.exit(“Exiting the program due to a specific condition.”)
- Raising an Exception: You can raise an exception to exit from the code when an error or a specific condition is encountered.
python
raise Exception(“An error occurred, terminating the program.”)
- Using `return` Statement: In functions, the `return` statement can be used to exit the function and subsequently the program if called from the main block.
python
def main():
if some_condition:
return
if __name__ == “__main__”:
main()
Forcible Termination of Python Code
There are scenarios where a more forceful termination is required, often when dealing with infinite loops or unresponsive scripts:
- Keyboard Interrupt: You can stop execution manually by pressing `Ctrl + C`, which raises a `KeyboardInterrupt`.
- Using `os._exit()`: This method can be used to exit immediately without cleanup operations.
python
import os
os._exit(1) # Exits the program with status code 1
Exiting from Loops
When working within loops, you might need to exit based on certain conditions. The `break` statement is useful in these cases:
- Using `break`: This statement allows you to terminate the nearest enclosing loop.
python
for i in range(10):
if i == 5:
break # Exits the loop when i equals 5
- Using `continue`: This statement can skip the current iteration and continue with the next one, but it does not terminate the loop.
python
for i in range(10):
if i % 2 == 0:
continue # Skips the even numbers
print(i) # Only prints odd numbers
Exiting from Functions
Functions can return values or terminate their execution using the `return` statement. This can influence the flow of the program significantly.
- Returning Early: You can use `return` to exit a function prematurely.
python
def check_value(x):
if x < 0:
return "Negative value, exiting function."
return "Value is acceptable."
Best Practices for Ending Code
To ensure a clean and effective termination of your Python programs, consider the following best practices:
Practice | Description |
---|---|
Use `sys.exit()` | For controlled exit with messages. |
Handle exceptions gracefully | Use try-except blocks to manage errors. |
Clean up resources | Ensure file handles and network connections are closed. |
Log exit messages | Provide logging information before exiting. |
These practices will help maintain code reliability and facilitate debugging when issues arise.
Expert Insights on Properly Ending Code in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively end code in Python, it is essential to utilize the ‘return’ statement in functions, which allows for a clean exit and returns control back to the calling context, ensuring that resources are managed efficiently.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “In Python, the end of a script is implicitly defined by the completion of the last statement. However, for clarity and maintainability, it is advisable to include a ‘main’ function and call it at the end of your script, which can help in structuring your code better.”
Jessica Lin (Python Educator and Author, LearnPythonToday). “Ending your code properly in Python also involves understanding exception handling. Utilizing ‘try’ and ‘except’ blocks can ensure that your program exits gracefully, even when unexpected errors occur, thus enhancing user experience.”
Frequently Asked Questions (FAQs)
How do I properly end a Python script?
To properly end a Python script, ensure that all processes are completed, and you can use the `exit()` function or `sys.exit()` from the `sys` module to terminate the program gracefully.
What is the purpose of the `exit()` function in Python?
The `exit()` function is used to terminate the Python interpreter and can be called with an optional exit status code, where `0` typically indicates success and any non-zero value indicates an error.
Can I use `Ctrl+C` to stop a Python program?
Yes, pressing `Ctrl+C` in the terminal will raise a `KeyboardInterrupt`, which stops the execution of the Python program immediately.
What happens if I don’t end a Python script properly?
Failing to end a Python script properly may lead to resource leaks, unsaved data, or incomplete transactions, which can affect the integrity of the application and the data it processes.
Is there a difference between `exit()` and `sys.exit()`?
Yes, `exit()` is a convenience function provided by the `site` module, while `sys.exit()` is part of the `sys` module and is generally preferred for scripts, as it can handle exceptions more effectively.
How can I ensure all threads are completed before ending a Python program?
To ensure all threads are completed, use the `join()` method on each thread instance, which blocks the calling thread until the thread whose `join()` method is called is terminated.
In Python, ending code typically refers to the completion of a script or a program. Python does not require a specific command to end a script, as it will terminate automatically once the interpreter reaches the end of the file. However, there are certain practices and constructs that can be utilized to control the flow of execution and ensure that the program concludes gracefully. For instance, using the `exit()` function from the `sys` module or the built-in `quit()` function can explicitly terminate the program at any point in the code.
Additionally, it is important to handle any necessary cleanup operations before the program ends. This can include closing files, releasing resources, or ensuring that any ongoing processes are properly terminated. Utilizing context managers with the `with` statement is a best practice in Python, as it automatically handles resource management, ensuring that files and other resources are closed correctly when the block of code is exited.
In summary, while Python scripts conclude naturally when the end of the file is reached, utilizing explicit termination commands and ensuring proper cleanup are essential for writing robust and maintainable code. Understanding these concepts will aid developers in creating applications that not only function correctly but also manage resources efficiently.
Author Profile
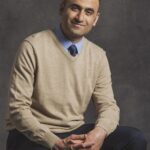
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?