How Do You Properly End a Function in Python?
In the world of programming, mastering the flow of control within your code is essential for creating efficient and effective applications. Python, with its elegant syntax and powerful capabilities, allows developers to craft functions that can perform a myriad of tasks. However, understanding how to properly conclude a function is just as critical as knowing how to begin one. Whether you’re returning a value, terminating execution early, or simply letting the function reach its natural end, the way you end a function can significantly impact the behavior of your program.
When you define a function in Python, you create a block of reusable code that can be executed whenever called upon. But what happens when it’s time to finish that function? There are various techniques and best practices to consider, each serving different purposes and scenarios. For instance, you might want to return a value to the caller, allowing the function to pass back results or data. Alternatively, you may need to implement conditional statements that dictate when a function should exit prematurely, ensuring that your code behaves as expected under various circumstances.
As you delve deeper into the nuances of function termination in Python, you’ll discover the importance of clarity and intention in your code. Understanding how to effectively conclude a function not only enhances readability but also contributes to the overall robustness of your programming. Join us as
Using Return Statements
In Python, the primary method to end a function is through the use of a `return` statement. When the interpreter encounters a return statement, it exits the function and returns control to the calling context. If a value is specified, that value is returned; otherwise, `None` is returned by default. This allows for a clean exit from the function.
Example:
“`python
def calculate_sum(a, b):
return a + b
“`
In this example, once the function `calculate_sum` is executed, it will terminate and return the sum of `a` and `b`.
Implicit Function Exit
Functions can also end implicitly when the execution flow reaches the end of the function body. This method is less explicit but still effective.
Example:
“`python
def greet(name):
print(f”Hello, {name}!”)
“`
In this case, the function `greet` ends when the print statement is executed, and control returns to the caller without a return statement.
Raising Exceptions
Another way to end a function is by raising an exception. This interrupts the normal flow of control and can be used to signify an error condition. When an exception is raised, the function exits immediately, and the control is passed to the nearest exception handler.
Example:
“`python
def divide(x, y):
if y == 0:
raise ValueError(“Cannot divide by zero”)
return x / y
“`
In this example, if `y` is zero, a `ValueError` is raised, and the function exits immediately.
Using the `break` Statement in Loops
If a function contains loops, the `break` statement can be used to exit the loop and subsequently the function. This is particularly useful when certain conditions are met, allowing for a more controlled exit from complex functions.
Example:
“`python
def find_first_even(numbers):
for num in numbers:
if num % 2 == 0:
return num
return None
“`
Here, the function will return the first even number it finds, and if no even number exists, it returns `None`.
Table of Function Exit Methods
Method | Description |
---|---|
Return Statement | Explicitly exits a function and returns a value. |
Implicit Exit | Ends when the end of the function body is reached. |
Raising Exceptions | Exits the function due to an error condition. |
Break in Loops | Exits loops within functions and consequently exits the function. |
By understanding these various methods to end a function, developers can write more effective and manageable Python code.
Returning Values from a Function
In Python, a function can be ended by using the `return` statement. This statement not only terminates the function but also sends a value back to the caller. If no value is specified, it returns `None` by default.
- Example of returning a value:
“`python
def add(a, b):
return a + b
result = add(5, 3) result will be 8
“`
- Example of returning no value:
“`python
def greet(name):
print(f”Hello, {name}!”)
return Implicitly returns None
greet(“Alice”) Output: Hello, Alice!
“`
Using `break` and `continue` in Functions
While `break` and `continue` are typically used within loops, they can also impact the flow of a function. However, they cannot be used to exit a function directly but can control loop execution.
- `break`: Exits the nearest enclosing loop.
- `continue`: Skips the rest of the code inside the loop for the current iteration and proceeds to the next iteration.
Example of using `break`:
“`python
def find_even(numbers):
for number in numbers:
if number % 2 == 0:
return number Ends function when the first even number is found
return None
“`
Raising Exceptions to End a Function
Another method to end a function is by raising an exception. This approach is useful when an error condition occurs, and you want to signal that the function cannot complete its task.
- Example of raising an exception:
“`python
def divide(a, b):
if b == 0:
raise ValueError(“Cannot divide by zero.”)
return a / b
“`
Using `pass` for Empty Functions
In some cases, you may want to define a function that does nothing. The `pass` statement allows you to create a placeholder function.
- Example of an empty function:
“`python
def placeholder_function():
pass Function does nothing and can be implemented later
“`
Ending Functions with Return Statement Variations
The `return` statement can be used in various ways to control the flow of a function, including returning multiple values using tuples.
- Example of returning multiple values:
“`python
def calculate(a, b):
sum_result = a + b
product_result = a * b
return sum_result, product_result Returns a tuple
total, product = calculate(5, 3) total = 8, product = 15
“`
Function Annotations and Return Types
Python allows for type hints, which can improve code readability by indicating the expected return type of a function.
- Example of function annotations:
“`python
def multiply(x: int, y: int) -> int:
return x * y
“`
Function Name | Parameters | Return Type |
---|---|---|
`add` | `a: int, b: int` | `int` |
`greet` | `name: str` | `None` |
`divide` | `a: float, b: float` | `float` or raises `ValueError` |
This structured approach to defining and ending functions enhances clarity and maintainability in Python programming.
Expert Insights on Ending Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, functions are concluded with the return statement, which not only exits the function but can also provide a value back to the caller. Understanding how to effectively use return statements is crucial for managing function outputs and flow control.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “To end a function in Python, one must recognize that reaching the end of the function body also implicitly returns None if no return statement is specified. This behavior is essential for developers to grasp in order to avoid unexpected results in their programs.”
Sarah Thompson (Python Educator and Author, LearnPythonNow). “When teaching how to end functions in Python, I emphasize the importance of structured programming. Using return statements not only allows for better readability but also aids in debugging by clearly defining exit points within the function.”
Frequently Asked Questions (FAQs)
How do I return a value from a function in Python?
To return a value from a function in Python, use the `return` statement followed by the value or expression you want to return. For example:
“`python
def add(a, b):
return a + b
“`
What happens if I do not use a return statement in a function?
If a function does not include a return statement, it implicitly returns `None` when the function execution completes. This means no value is returned to the caller.
Can I end a function early in Python?
Yes, you can end a function early by using the `return` statement at any point within the function. This will exit the function and return control to the caller immediately.
Is it possible to have multiple return statements in a function?
Yes, a function can have multiple return statements. The first return statement that is executed will terminate the function and return the specified value.
What is the difference between return and print in a function?
The `return` statement sends a value back to the caller, allowing it to be used later in the program. In contrast, `print` outputs a value to the console but does not return it to the caller.
Can I use return without a value in Python?
Yes, you can use `return` without a value, which will return `None` to the caller. This is useful when you want to exit a function without returning any specific data.
In Python, functions are defined using the `def` keyword, and their execution is concluded with a return statement or by reaching the end of the function block. The return statement allows a function to send back a value to the caller, effectively ending the function’s execution at that point. If a return statement is not present, the function will terminate upon reaching the end of its code block, returning `None` by default.
Understanding how to properly end a function is crucial for effective programming in Python. The return statement not only signifies the end of the function but also facilitates the transfer of data back to the calling context. This feature enables the creation of reusable and modular code, which is a fundamental principle of software development. Moreover, using return statements can enhance the clarity and maintainability of code by explicitly indicating the output of a function.
In summary, mastering the use of return statements and understanding how functions conclude in Python is essential for any programmer. It fosters better coding practices and aids in the development of efficient algorithms. By leveraging these concepts, developers can write more organized, readable, and functional code, ultimately leading to improved software quality and performance.
Author Profile
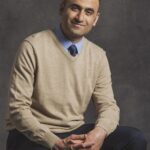
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?