How Can You Effectively End a Python Program?
When diving into the world of Python programming, one of the fundamental skills every developer must master is how to effectively control the execution of their programs. Whether you’re crafting a simple script or developing a complex application, knowing how to end a Python program gracefully is crucial. This skill not only enhances the reliability of your code but also ensures that resources are managed efficiently, preventing potential data loss or corruption. In this article, we will explore various methods to terminate a Python program, equipping you with the knowledge to handle program termination in a way that aligns with best practices.
Ending a Python program can be straightforward, but it also comes with nuances that can affect the overall performance of your application. From using built-in functions to handling exceptions and signals, there are multiple approaches to consider. Each method has its own use cases and implications, making it essential for developers to understand the context in which they are operating. Moreover, how you choose to terminate your program can influence not just the immediate outcome, but also the user experience and system stability.
As we delve deeper into this topic, we will examine the various techniques available for terminating a Python program, highlighting their advantages and potential pitfalls. By the end of this article, you’ll be equipped with the tools and insights needed to end your Python programs
Using the exit() Function
The simplest and most straightforward method to terminate a Python program is to utilize the built-in `exit()` function. This function can be called without any arguments or with an optional integer argument that indicates the exit status. By convention, an exit status of `0` signifies successful completion, while any non-zero value indicates an error.
Example usage:
python
print(“Program is ending.”)
exit(0)
The code above will print a message and then terminate the program gracefully.
Utilizing the sys Module
The `sys` module provides access to some variables used or maintained by the interpreter and allows for interaction with the interpreter. To exit a program using this module, you can use `sys.exit()`. Like `exit()`, it can also take an integer or a string as an argument.
Example usage:
python
import sys
print(“Exiting the program.”)
sys.exit(1)
This method is particularly useful in larger applications where you want to signal different exit statuses based on various conditions.
Raising SystemExit Exception
Another approach to end a Python program is by raising the `SystemExit` exception. This method provides more control, as you can handle the exception if needed.
Example usage:
python
raise SystemExit(“Program is terminating.”)
This will terminate the program and can also include a message to indicate why it was terminated.
Keyboard Interrupt
During the execution of a program, you might want to terminate it manually. This can be achieved with a keyboard interrupt, typically by pressing `Ctrl + C`. This generates a `KeyboardInterrupt` exception that can be caught and managed if desired.
Example usage:
python
try:
while True:
pass # Simulating a long-running process
except KeyboardInterrupt:
print(“Program was interrupted by the user.”)
This allows for a graceful exit while providing feedback to the user.
Exiting from Loops or Functions
In addition to terminating the entire program, you might want to exit from loops or functions prematurely. The `break` statement can be utilized within loops, and the `return` statement can be used within functions.
Example of using `break`:
python
for i in range(10):
if i == 5:
break # Exits the loop when i equals 5
print(“Loop has ended.”)
Example of using `return`:
python
def example_function():
return “Exiting function early.”
result = example_function()
print(result)
Method | Usage | Exit Status |
---|---|---|
exit() | exit() | Optional, default is 0 |
sys.exit() | sys.exit() | Optional, can be string or integer |
raise SystemExit | raise SystemExit() | Custom message or code |
Keyboard Interrupt | Ctrl + C | Generates KeyboardInterrupt |
These various methods provide flexibility in how you can terminate a Python program, allowing for both graceful exits and controlled shutdowns based on user input or program logic.
Methods to End a Python Program
In Python, there are several effective ways to terminate a program, each suitable for different scenarios. Below are the most common methods:
Using the `exit()` Function
The `exit()` function is a straightforward method to terminate a Python program. This function is provided by the `sys` module and is designed to halt the program execution immediately.
python
import sys
# Some code logic
if some_condition:
sys.exit()
- Usage: Use `sys.exit()` when you need to exit from a Python script explicitly.
- Return Codes: You can pass an optional integer argument to specify the exit status. For example, `sys.exit(0)` indicates a successful termination, while `sys.exit(1)` signals an error.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can also terminate a program. It serves primarily as an interactive shell command.
python
# Some code logic
if some_condition:
quit()
- Note: While `quit()` is convenient, it is generally recommended to use `sys.exit()` for scripts since it is more explicit and clear in intent.
Using the `raise SystemExit` Exception
Another method to end a Python program is by raising the `SystemExit` exception, which is the underlying mechanism of the `exit()` and `quit()` functions.
python
# Some code logic
if some_condition:
raise SystemExit
- Customization: Like `exit()`, you can customize the exit code by passing an integer or string to `raise SystemExit(value)`.
Using `os._exit()`
The `os._exit()` function is a low-level method that terminates a program immediately without calling cleanup handlers, flushing standard I/O buffers, etc.
python
import os
# Some code logic
if some_condition:
os._exit(0)
- Use Case: This method is typically used in child processes after a fork and is not recommended for normal program termination due to its abrupt nature.
Using Keyboard Interrupts
For interactive programs, a user can terminate execution using a keyboard interrupt. This is typically done by pressing `Ctrl+C`.
- Handling Keyboard Interrupts: You can catch this exception to perform cleanup operations before the program exits.
python
try:
# Some long-running code
while True:
pass
except KeyboardInterrupt:
print(“Program terminated by user.”)
Using Conditional Statements
In many cases, programs are structured to end based on certain conditions. This can be achieved through `if` statements.
python
# Some code logic
if condition_to_end:
print(“Ending program.”)
exit()
This approach is effective for controlling program flow and ensuring that certain conditions are met before terminating.
Comparison Table of Methods
Method | Use Case | Exit Status Customization |
---|---|---|
sys.exit() | Explicit termination | Yes |
quit() | Interactive shell | Yes |
raise SystemExit | Exception handling | Yes |
os._exit() | Child processes | No |
Keyboard Interrupt | User-initiated | No |
Effective Methods to Terminate a Python Program
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To end a Python program gracefully, one can utilize the built-in `sys.exit()` function. This method allows for an optional exit status code, providing clarity on the program’s termination state.”
Michael Chen (Python Developer, OpenSource Solutions). “For scenarios where an immediate termination is required, using `os._exit()` is effective. However, this method should be used cautiously as it bypasses cleanup handlers and may lead to resource leaks.”
Lisa Tran (Technical Writer, Python Programming Journal). “In interactive environments, such as Jupyter notebooks, the command `exit()` or `quit()` can be employed to terminate the session. These commands are user-friendly and designed for such contexts.”
Frequently Asked Questions (FAQs)
How can I terminate a Python program using a keyboard shortcut?
You can terminate a running Python program by pressing `Ctrl + C` in the terminal or command prompt where the program is executing. This sends a keyboard interrupt signal to the program.
What is the purpose of the `exit()` function in Python?
The `exit()` function is used to exit from Python. It raises the `SystemExit` exception, which can be caught in outer levels of the program, but if not caught, it will terminate the program.
How do I end a Python script from within the code?
You can use the `sys.exit()` function from the `sys` module to terminate a Python script programmatically. This function can take an optional argument to indicate the exit status.
What is the difference between `exit()` and `sys.exit()`?
`exit()` is a convenience function that is intended for use in interactive sessions, while `sys.exit()` is more appropriate for use in scripts. `sys.exit()` provides more control over the exit status.
Can I use `os._exit()` to end a Python program?
Yes, `os._exit()` can be used to terminate a program immediately without cleanup. It is generally advised to use `sys.exit()` for graceful termination, but `os._exit()` is useful in specific scenarios like terminating child processes.
What happens if I do not handle exceptions in my Python program?
If exceptions are not handled, they will propagate up the call stack and may cause the program to terminate unexpectedly. It is recommended to use try-except blocks to manage exceptions effectively.
In summary, ending a Python program can be accomplished through several methods, each suited to different scenarios. The most common way to terminate a program is by using the built-in `exit()` or `sys.exit()` functions, which allow for a graceful shutdown of the program. Additionally, raising an exception can also lead to an abrupt termination, especially in situations where an error occurs that the program cannot handle. Understanding these methods is essential for managing program flow and ensuring that resources are released properly upon termination.
Another important aspect to consider is the use of the `return` statement within functions. This statement not only exits the function but can also dictate the termination of the program if it is used in the main execution block. Moreover, utilizing control structures such as loops and conditionals can provide more nuanced control over when and how a program ends, allowing for a more tailored user experience.
Key takeaways include the importance of choosing the appropriate method for ending a Python program based on the context. For instance, using `sys.exit()` is preferable for scripts that may be run in various environments, while exceptions are useful for error handling. Ultimately, a well-structured program should incorporate clear exit strategies to enhance maintainability and user interaction.
Author Profile
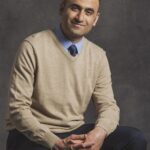
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?