How Do You Execute a Stored Procedure Effectively?
In the world of database management, efficiency and performance are paramount. As data continues to grow exponentially, the need for streamlined operations becomes increasingly critical. Enter stored procedures—powerful tools that allow developers and database administrators to encapsulate complex logic and automate repetitive tasks within the database. But how exactly do you execute a stored procedure? Understanding this fundamental process can significantly enhance your ability to manipulate and interact with your data effectively.
Stored procedures are precompiled collections of SQL statements that can be executed as a single unit. They not only improve performance by reducing the amount of information sent over the network but also enhance security by limiting direct access to the underlying data. Executing a stored procedure is a straightforward process, yet it involves various nuances depending on the database management system in use. Whether you’re working with SQL Server, MySQL, or Oracle, grasping the execution mechanics is essential for leveraging the full potential of stored procedures.
As we delve deeper into the topic, we will explore the syntax and methods for executing stored procedures across different platforms. From understanding input and output parameters to handling exceptions, mastering this skill will empower you to write more efficient code and optimize your database interactions. Join us as we unlock the secrets of executing stored procedures and elevate your database management expertise.
Executing Stored Procedures in SQL
To execute a stored procedure in SQL, the syntax generally involves the `EXEC` command, which is a straightforward way to call a stored procedure by its name. The exact method can vary depending on the database management system (DBMS) you are using. Below, we outline how to execute stored procedures in some of the most commonly used systems.
Syntax for Executing Stored Procedures
Here are examples of how to execute stored procedures across different DBMS:
- SQL Server:
“`sql
EXEC procedure_name;
“`
- MySQL:
“`sql
CALL procedure_name();
“`
- Oracle:
“`sql
BEGIN
procedure_name;
END;
“`
- PostgreSQL:
“`sql
CALL procedure_name();
“`
Passing Parameters
Stored procedures can accept parameters, which allow for more dynamic execution. When calling a stored procedure with parameters, you must specify the values to be passed. Below are examples of how to do this:
- SQL Server:
“`sql
EXEC procedure_name @param1 = value1, @param2 = value2;
“`
- MySQL:
“`sql
CALL procedure_name(value1, value2);
“`
- Oracle:
“`sql
BEGIN
procedure_name(param1, param2);
END;
“`
- PostgreSQL:
“`sql
CALL procedure_name(value1, value2);
“`
Example of Executing a Stored Procedure
Consider a stored procedure named `GetEmployeeDetails` that takes an employee ID as a parameter. Below is how you would execute this stored procedure in different systems:
DBMS | Execution Syntax |
---|---|
SQL Server | EXEC GetEmployeeDetails @EmployeeID = 101; |
MySQL | CALL GetEmployeeDetails(101); |
Oracle | BEGIN GetEmployeeDetails(101); END; |
PostgreSQL | CALL GetEmployeeDetails(101); |
Handling Return Values
Some stored procedures may return a value. The method for retrieving this value also varies by DBMS. For instance:
- SQL Server: You can define an output parameter and retrieve it after execution.
- MySQL: Use the `SELECT` statement to get the return value.
- Oracle: Use an OUT parameter.
- PostgreSQL: Use a return statement inside the procedure.
With the right syntax and an understanding of how to pass parameters, executing stored procedures can be accomplished efficiently across different database systems. Be sure to refer to the documentation specific to your DBMS for advanced features and best practices.
Executing a Stored Procedure in SQL Server
To execute a stored procedure in SQL Server, the `EXEC` or `EXECUTE` command is typically utilized. This command can be used in various ways depending on the parameters passed to the stored procedure.
Basic Syntax
“`sql
EXEC procedure_name;
“`
Example
“`sql
EXEC GetEmployeeDetails;
“`
Executing Stored Procedures with Parameters
When a stored procedure accepts parameters, you can pass them using the following syntax:
“`sql
EXEC procedure_name @parameter1 = value1, @parameter2 = value2;
“`
Example
“`sql
EXEC GetEmployeeDetails @EmployeeID = 123, @DepartmentID = 4;
“`
Using OUTPUT Parameters
If a stored procedure returns values via output parameters, you can declare variables to capture these values:
“`sql
DECLARE @OutputVar INT;
EXEC GetEmployeeCount @DepartmentID = 4, @Count = @OutputVar OUTPUT;
SELECT @OutputVar AS EmployeeCount;
“`
Executing a Stored Procedure in MySQL
In MySQL, the `CALL` statement is used to execute stored procedures. Here’s how to do it:
Basic Syntax
“`sql
CALL procedure_name();
“`
Example
“`sql
CALL GetEmployeeDetails();
“`
Executing Stored Procedures with Parameters
You can also pass parameters similarly:
“`sql
CALL procedure_name(parameter1, parameter2);
“`
Example
“`sql
CALL GetEmployeeDetails(123, 4);
“`
Executing a Stored Procedure in Oracle
Oracle uses the `EXECUTE` command, or you can use an anonymous PL/SQL block to run a stored procedure.
Basic Syntax
“`sql
EXECUTE procedure_name;
“`
Example
“`sql
EXECUTE GetEmployeeDetails;
“`
Using PL/SQL Block
For procedures with parameters, you can use a PL/SQL block:
“`sql
BEGIN
procedure_name(parameter1, parameter2);
END;
“`
Example
“`sql
BEGIN
GetEmployeeDetails(123, 4);
END;
“`
Executing a Stored Procedure in PostgreSQL
In PostgreSQL, the `CALL` statement is utilized for executing stored procedures, but note that it is available from version 11 onward.
Basic Syntax
“`sql
CALL procedure_name();
“`
Example
“`sql
CALL GetEmployeeDetails();
“`
Executing Stored Procedures with Parameters
For procedures with parameters:
“`sql
CALL procedure_name(parameter1, parameter2);
“`
Example
“`sql
CALL GetEmployeeDetails(123, 4);
“`
Executing a Stored Procedure in SQLite
SQLite does not support stored procedures in the traditional sense. Instead, you can achieve similar functionality using user-defined functions or scripts.
Creating a User-Defined Function
You can create a user-defined function with the following syntax:
“`sql
CREATE FUNCTION function_name(parameters) RETURNS return_type AS BEGIN
— function body
END;
“`
Example
“`sql
CREATE FUNCTION GetEmployeeCount(department_id INT) RETURNS INT AS BEGIN
RETURN (SELECT COUNT(*) FROM Employees WHERE DepartmentID = department_id);
END;
“`
To execute it, simply use:
“`sql
SELECT GetEmployeeCount(4);
“`
Expert Insights on Executing Stored Procedures
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “To execute a stored procedure effectively, one must first ensure that the procedure is correctly defined in the database. Utilizing the EXEC command in SQL Server or CALL in MySQL is essential, and passing the appropriate parameters is crucial for successful execution.”
Mark Thompson (Senior Database Administrator, DataGuard Corp.). “Understanding the context in which a stored procedure operates is vital. Executing it within a transaction can help maintain data integrity, especially when multiple procedures are involved. Always monitor performance metrics to optimize execution time.”
Lisa Patel (Lead Software Engineer, CodeCraft Solutions). “When executing stored procedures, consider using application-level frameworks that facilitate database interactions. This can streamline the process and enhance security by preventing SQL injection vulnerabilities through parameterized queries.”
Frequently Asked Questions (FAQs)
What is a stored procedure?
A stored procedure is a precompiled collection of SQL statements stored in the database. It can accept parameters, execute complex logic, and return results.
How do I execute a stored procedure in SQL Server?
To execute a stored procedure in SQL Server, use the `EXEC` or `EXECUTE` command followed by the procedure name. For example: `EXEC dbo.ProcedureName;`.
Can I pass parameters to a stored procedure?
Yes, you can pass parameters to a stored procedure. Use the syntax `EXEC dbo.ProcedureName @Parameter1 = Value1, @Parameter2 = Value2;` to provide the necessary arguments.
What is the difference between a stored procedure and a function?
A stored procedure performs actions and does not return a value directly, while a function returns a single value and can be used in SQL expressions.
How can I check if a stored procedure executed successfully?
You can check the execution status by examining the return value or using the `TRY…CATCH` block in SQL Server to handle errors and log messages accordingly.
Can I schedule the execution of a stored procedure?
Yes, you can schedule the execution of a stored procedure using SQL Server Agent jobs, allowing for automated execution at specified intervals or times.
Executing a stored procedure is a fundamental task in database management that allows users to encapsulate complex operations and enhance code reusability. Stored procedures are precompiled collections of SQL statements that can be executed as a single unit, providing significant performance benefits and simplifying application development. Understanding how to effectively execute these procedures is essential for database administrators and developers alike.
There are various methods to execute a stored procedure, depending on the database management system (DBMS) in use. Common approaches include using SQL commands such as `EXEC` or `CALL`, depending on whether the DBMS is SQL Server, MySQL, or Oracle. Additionally, parameters can be passed to stored procedures to customize their behavior, allowing for dynamic execution based on user input or application requirements.
Key takeaways include the importance of understanding the specific syntax and capabilities of the DBMS being used, as well as the potential for stored procedures to improve performance and maintainability of database applications. By leveraging stored procedures effectively, organizations can streamline their database operations and ensure more efficient data handling.
Author Profile
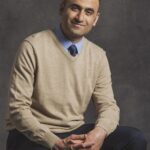
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?